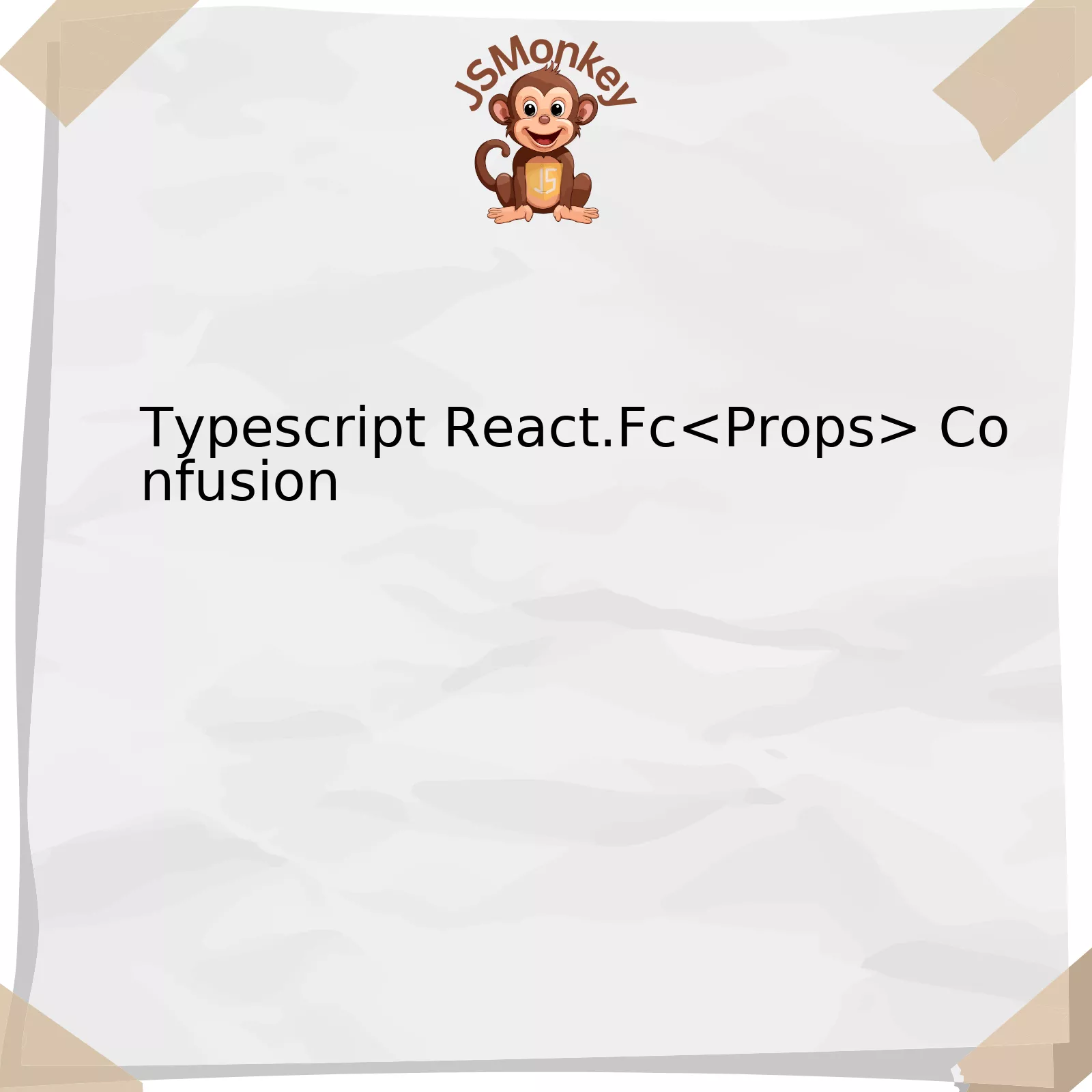
React.FC<Props>
. Let’s break down this particular idea by examining its various components:
Component | Description |
---|---|
<Props> | This symbolizes the properties or attributes that will be utilized in a specific component. They can be of any type allowed in TypeScript, providing flexibility and control over the data being passed in a React component. |
React.FC | FC stands for Function Component and it’s a type provided by React’s type definitions. By defining a component as
React.FC , you’re signaling that this particular piece is a functional component within your React application. |
<React.Fc |
Combining these two together in
React.Fc<Props> lets TypeScript know that we’re dealing with a functional component that takes incoming properties referred to as Props. |
So why might there be confusion when using this syntax? Consider a couple of scenarios:
1. **Prop Drilling:** This occurs when one intermediate part of your app requires Props purely as a passthrough towards deeper nested components. In those situations, explicit annotations with
React.FC<Props>
can become confusing, unreadable, or unwieldy.
2. **Changes on Default Props:** The way
React.FC
and
defaultProps
handle types are incompatible, meaning you would need to either type the default Props explicitly, or refrain from using
React.FC
in your component.
While powerful and useful, one should be mindful of pitfalls along
React.Fc<Props>
‘s use. An excellent way to address these challenges is by robust education and diligent practice.
As renowned coder Casey Neistat once put it:
“Every skill you acquire doubles your odds of success.”
By understanding how to use
React.FC<Props>
more effectively, one can double their odds of crafting a successful, robust React project with Typescript.
Diving into Typescript React.Fc
When it comes to integrating TypeScript with React, there can often be confusion due to the wealth of different configurations, rules, and syntax habits developers have been accustomed to. One such area of bewilderment is typically around `React.FC
The Basics of TypeScript `
React.FC<Props>
`
To put it in basic terms:
React.FC<Props> |
is a typing system that describes a functional component (FC) with defined properties (Props) typically passed from parent components. |
But where does the confusion truly lie?
– Syntax Variation:
A common challenge lies in the varied syntax that TypeScript allows for defining a functional component’s Props. This heterogeneity might result in inconsistencies in codebases maintained by multiple team members and create confusion.
For example:
jsx
const MyComponent: React.FC
is an equivalent way to :
jsx
const MyComponent = (props: Props) => {…}
– Automatic Inclusion of Children as Prop:
Yet another element of astonishment is the automatic inclusion of `children` as a prop. `React.FC
– Generic Type Argument:
The utilization of `
Dealing with such confusion
How do we deal with such complexities?
– Adopting uniform syntax conventions across teams is a good step towards mitigating confusion.
– Engage in regular code reviews to make sure the integrity of the Props’ data structures are upheld.
– And, as Bjarne Stroustrup, the original developer of C++, said: “Any fool can write code that a computer can understand, but very few can write code that humans can understand.” So, commenting and documenting code reduces misunderstandings enormously amongst team members.
With a better understanding of what lies behind these confounding aspects of `React.FC
Learn more about this topic from this source.
Clarifying Common Misconceptions: Typescript React.Fc
When dealing with TypeScript in a React context, specifically the use of `React.Fc
Misconception 1: Focusing on `React.Fc
The `
React.Fc
` generic is commonly utilized to type React functional components. While you can indeed use this format for all your functional components in a TypeScript-based React application, it’s not always necessary. Using `
React.Fc
` should be viewed as a tool you could use when additional typings are needed (like `defaultProps` or `propTypes`), rather than a blanket default across all components.
Misconception 2: Reactive Context of Props
Another misunderstanding leads to the belief that `Props` in the `React.Fc
type MyComponentProps = { name: string, age: number }
Then your component would accept an object that contains a `’name’` field of type `string`, and an `’age’` field of type `number`.
Misconception 3: FC (Functional Component) Typing Obligation
Some developers might feel obligated to use the `
React.FC
` type for every functional component. This isn’t necessarily the case, and many experienced TypeScript with ReactJS developers often recommend defining only the props of your component. The reason falls into readability and simplicity, as avoiding `
React.FC
` could make your code cleaner, especially when dealing with components that do not need child props.
As per Basarat, one of the TypeScript Deep Dive authors, “The primary reason for not using `React.FC` or `React.FunctionComponent` is its implicit definition of `children`.”
A lovely quote by Rob Pike, co-creator of the Go programming language seems apt here. He said, “Less code is better than more code.” In light of this, where it’s not necessary to include explicit typings, they may be best left out.
To encapsulate, comprehending `React.Fc
Practical Usage of Typescript in React.Fc
One of the prominent areas where TypeScript shines is when it integrates with popular Javascript libraries like React. Transitioning from writing JavaScript to TypeScript in developing a React application can be confusing, especially when dealing with TypeScript’s `React.FC
The
React.FC<Props>
(FunctionComponent) was heavily utilised in the past due to its simplicity and ability to define components that accept children or do not have props at all. However, within various development communities, the usage has now become rather controversial.
Consider this code snippet for your convenience:
type AppProps = { message: string; } const App : React.FC<AppProps> = ({message}) =>{message};
Here we see a straightforward functional component using TypeScript. But surprisingly, this approach is no more encouraged, and it may confuse many developers transitioning to TypeScript.
Let’s delve into some reasons why:
– Implicit children props: `React.FC
– Discourages functions without props: If there are no props for the component, writing `React.FC<>` becomes unnecessarily verbose.
– Unneeded render method: `React.FC
Looking into these issues, the newer school of thought suggests using regular functions instead:
type AppProps = { message: string; } function App({message}: AppProps) { return ({message}); }
In this version of the app, it does precisely the same thing, but without any unnecessary TypeScript features. This approach is less confusing and more streamlined for new TypeScript users.
In Brian Holt’s words from LinkedIn’s front-end team,”Writing TypeScript should feel like just writing JavaScript. These little things can help accomplish that.”
To make your TypeScript experience smoother, simpler syntax, such as direct function usage instead of `React.FC
Remember that, with every new technology, there can surely arise peculiarities or areas of confusion. Yet, they rarely diminish the overall value the technology provides.
Troubleshooting & Limitations: Understanding Typescript’s React.Fc
When it comes to TypeScript and React, a key point of confusion often revolves around the `React.FC
Underpinning this notion is understanding TSX, TypeScript’s syntax for embedding XML within JavaScript. This TypeScript extension adds static types to the mix and notably contributes towards confidence in code correctness.
The
React.FC
(or
React.FunctionComponent
) annotation provides an interface between TSX and functional components. It specifies that when a function component accepts props, those props are of a certain type.
One pattern could be:
type MyComponentProps = { /* define your component's props here */ } const MyComponent: React.FC<MyComponentProps> = (props) => ( /* your component render logic here */ );
Analyzing the potential limitations or points of contention with `React.FC
* Mandatory children even if not needed.
* No specificity regarding defaultProps.
Even though you might not utilise “children”, `FC` is defined as accepting children by default. This design exaggerates the number of valid properties, potentially obscuring future debugging attempts. To remedy this, consider writing out props explicitly and using proper typing to ensure understanding of what properties the function can accept.
On the flip side, when you need defaultProps, `React.FC` doesn’t alert TypeScript about their existence. As a consequence, TypeScript has no record of propTypes with optional attributes being made required via defaultProps. A workaround here is to manually type defaultProps with a conjunction operator (&):
const MyComponent: React.FC<MyComponentProps> & {defaultProps?: Partial<MyComponentProps>}> = (props) => ...
“The idea is to try to give all of the information to help others to judge the value of your contribution; not just the information that leads to judgment in one particular direction or another” – Richard Feynman, physicist and developer, even though from a different realm, his quote aptly applies to TypeScript and coding in general. This reflects our ongoing journey to fully grasp React’s interface with TypeScript and overcome any confusion surrounding it. As often experienced in programming, there might not be a ‘one-size-fits-all’ troubleshooting technique. However, by deeply understanding methodologies and their limitations, we render ourselves better equipped to handle whatever issues get thrown our way.
Analyzing the concept of Typescript React.Fc
React.FC
is an abbreviation for Function Component and is used primarily in TypeScript with React. By utilizing this particular feature, developers can create functional components that are strongly typed to receive specific properties.
Understanding the functionality of writing
React.FC
allows you to develop more robust applications:
-
React.FC
provides implicit typings for default props like children, which would otherwise have to be explicitly defined.
- By using Props in
React.FC
, developers obtain a better understanding of the types of data passed into their components, thus improving code integrity.
- With TypeScript, type-checking errors can be caught early on during development, allowing for efficient debugging and refactoring.
These benefits underly the significance of TypeScript integration within your React applications.
However, there’s been notable confusion regarding the use of
React.Fc<Props>
. Developers often face ambiguity over when to utilize it, and some argue that explicit typing is a superior approach.
A useful online reference addressing this topic is “Why I don’t use React.FC” . Here, the author suggests that there might be certain scenarios where employing
React.Fc<Props>
could result in more complications than benefits. Hence, it is crucial for developers to carefully gauge their requirements and the complexity of their application before settling for any particular tool or function.
Debates continue to brew about the pros and cons of
React.Fc<Props>
. As Don Knuth said, “We should forget about small efficiencies, say about 97% of the time: premature optimization is the root of all evil.” Whether to employ
React.Fc<Props>
or not comes down to context – your application needs, team familiarity with TypeScript and its nuances, and your project’s overall complexity and scale. Making an informed decision on this matter can help you create more flexible and maintainable code while avoiding undue confusion.
These discussions are critical for progressive growth in the JavaScript environment, leading to innovative solutions and better coding practices.