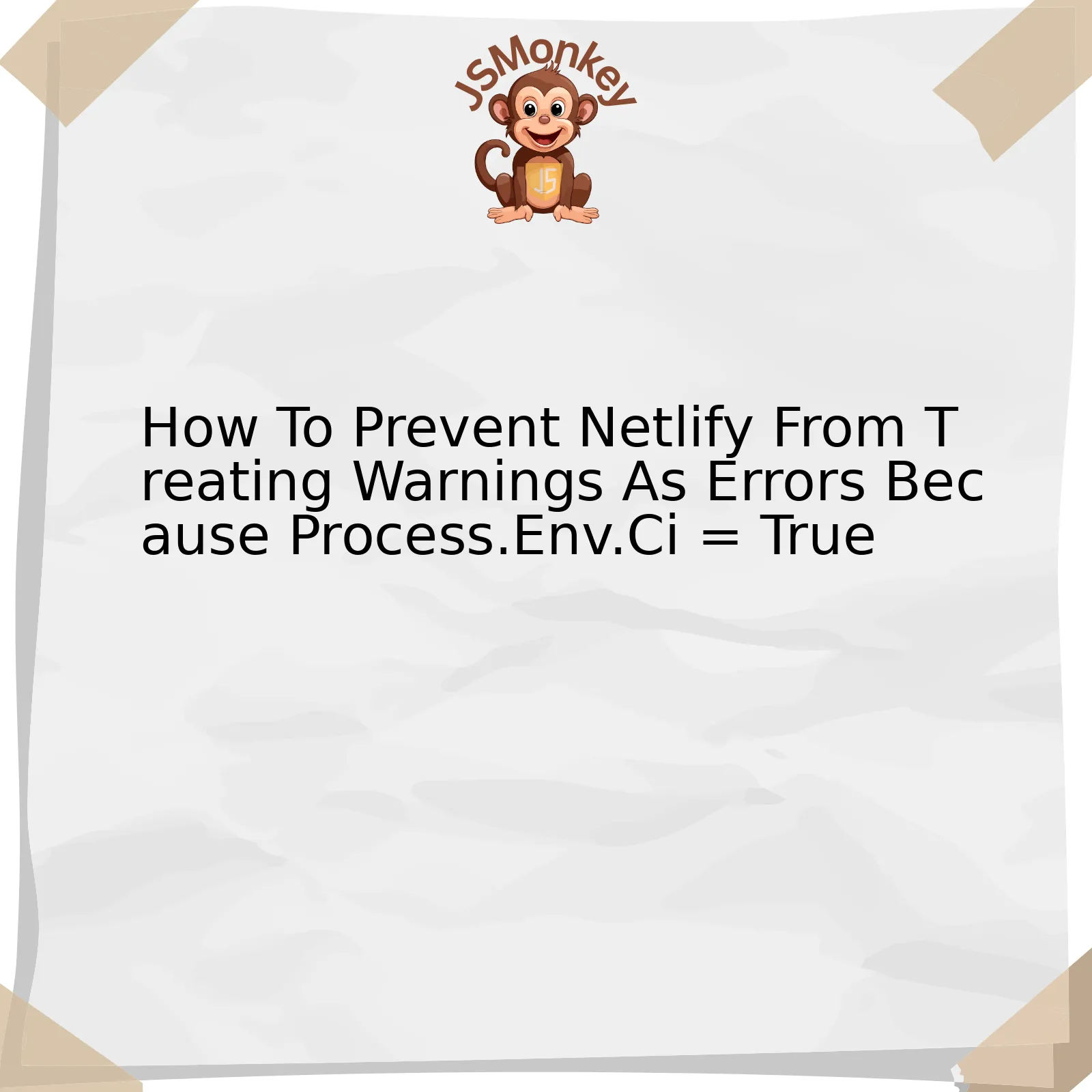
When deploying applications in Netlify, it’s essential to configure the environment such that warnings are not treated as errors. The significant factor here is
process.env.CI
. This refers to a Continuous Integration environment variable that, when set to ‘true’, causes all warnings in a project to be treated as errors.
Action | Description | Outcome |
---|---|---|
Identify issue | Warnings being treated as errors can halt the deployment process, highlighting minor issues that wouldn’t necessarily hamper functionality. | Understanding the cause of a blocked deployment. |
Modify environment variables | Setting
xxxxxxxxxx process.env.CI to ‘false’ is one way to stop treating warnings as errors within the Netlify’s build environment. It can be done by adding an Environment Variable in Netlify’s Site settings or by editing netlify.toml file. |
Allows the application to deploy while ignoring minor warning messages. |
Inspect regular deployments | Even after this setting modification, it’s crucial to ensure the usual quality check mechanisms exist so major issues don’t bypass unnoticed. | Maintains code integrity and long-term stability. |
While setting
xxxxxxxxxx
process.env.CI
to ‘false’ prevents warnings from blocking deployment, it’s important to consider this change’s implications on the software development cycle. Developers depend on warnings to identify potential issues in their code that, while not immediately problematic, may cause major headaches down the line. Thus, it’s paramount that developers carefully manage this change, ensuring they still check for and address warnings regularly during normal coding operations.
As Turing award winner Donald Knuth once said, “Beware of bugs in the above code; I have only proved it correct, not tried it”. This epic quote suggests that we shouldn’t take our code for granted, even if it seems correct. Keep debugging and optimizing until and unless you are completely satisfied with your code.
Understanding Process.env.CI in Netlify Deployment
When working on a Netlify deployment, one common issue developers encounter is the platform treating warnings as errors. This happens due to the presence of
xxxxxxxxxx
process.env.CI = true
. To better understand this and how to prevent it from interrupting your development work, let’s dive deeper.
At a Glance what is process.env.CI
In JavaScript programming, and especially Node.js environments,
xxxxxxxxxx
process.env
is a global object that provides information about the current execution environment. The property
xxxxxxxxxx
CI
within
xxxxxxxxxx
process.env
, in our case, stands for ‘Continuous Integration’. When this variable is set to true (i.e.,
xxxxxxxxxx
process.env.CI = true
), it implies that the current environment is a continuous integration server.
This mechanism is widely used by many CI servers including Netlify. By default, Netlify sets
xxxxxxxxxx
process.env.CI
to true during both build step and deploy process.
The Ramification
By setting
xxxxxxxxxx
process.env.CI = true
, any warnings encountered during the build process are treated as errors. This causes the build to fail if there’s even a single warning. While this might help in enforcing strict code, it can be overly stringent for some developers’ preferences.
Preventing Netlify from Treating Warnings as Errors
If you do not want Netlify to treat all warnings as errors, you can override the default behavior of
xxxxxxxxxx
process.env.CI
. In your netlify.toml file, or in the environmental settings of your project on the Netlify dashboard, you can provide
xxxxxxxxxx
CI=''
or
xxxxxxxxxx
CI=''
.
The process.env.CI attribute can also be overridden in several other ways. Here’s an example by adding it as a prefix to the build command in the Netlify UI:
xxxxxxxxxx
CI= npm run build
.
Remember, this strategy might make the development process smoother but it may overlook useful warnings that could prevent potential problems down the line.
Steve Jobs once said: “Sometimes when you innovate, you make mistakes. It is best to admit them quickly, and get on with improving your other innovations.” So while lowering the threshold could make things easier in the short term, it’s important to keep fine-tuning the code to ensure long-term efficiency.
Here’s an illustration of the settings:
Configuration Location | Action |
---|---|
netlify.toml file | Provide
xxxxxxxxxx CI='' or xxxxxxxxxx CI='' |
Netlify dashboard environmental settings | Add
xxxxxxxxxx CI= npm run build to the build command |
Through understanding the role of
xxxxxxxxxx
process.env.CI
in the deployment process and its default behavior on Netlify platform, developers can better manage their code deployment process, especially when dealing with code warnings and errors.
For further insights, feel free to delve into the rich resources available in the [Netlify Documentation](https://docs.netlify.com).
Solutions for Preventing Warnings Treated as Errors in Netlify
When deploying Node.js applications on Netlify, there’s a high chance that you’ll encounter an environment variable –
xxxxxxxxxx
process.env.CI
, which is set to true by default. This sets warnings as errors and halts the build causing any warnings in your code to fail the deployment. To prevent Netlify from treating warnings as errors because of
xxxxxxxxxx
process.env.CI = true
, you will need to override this behavior.
“Make it work, make it right, make it fast.” – Kent Beck, renowned software developer and the creator of extreme programming.
Primarily, consider adjusting your build command in Netlify’s build settings so that it explicitly sets
xxxxxxxxxx
process.env.CI
to false. Instead of using
xxxxxxxxxx
npm run build
or
xxxxxxxxxx
yarn build
, utilize the command
xxxxxxxxxx
CI=false npm run build
or
xxxxxxxxxx
CI=false yarn build
.
This ensures the create-react-app build script ignores any warnings and continues with the deployment process, regardless of the faults that may exist in your application’s code. Keep in mind that it doesn’t ignore the importance of resolving those warnings.
Alternatively, if you do not wish to turn off Netlify’s continuity integration or when your project’s build script is complicated – instead of altering the build command globally, one might prefer to modify specific portions of the build scripts inside the package.json file of the project. Here, you can set up commands so that they trigger with
xxxxxxxxxx
process.env.CI
set to false.
The modification could look as follows:
xxxxxxxxxx
"scripts": {
"start": "react-scripts start",
"build": "CI=false react-scripts build",
"test": "react-scripts test",
"eject": "react-scripts eject"
}
However, turning off
xxxxxxxxxx
process.env.CI
should only be a temporary solution. Ideally, warnings are there to guide us – they help us write better, more secure, and manageable code. Therefore, the best way to solve this issue in Netlify would be to actually rectify the warnings shown in your code.
An essential practice when dealing with warnings is to adhere to the principle of “linting”. Linting is a type of static code analysis used often in JavaScript to find problematic patterns or code that doesn’t adhere to certain styling guidelines. There are several tools available for linting such as ESLintsource, JSLint, and JSHint.
By taking advantage of linting tools, developers can write cleaner and maintainable code reducing the number of warnings generated during the building process.
Hence, preventing Netlify from treating warnings as errors because
xxxxxxxxxx
process.env.CI = true
involves setting the
xxxxxxxxxx
CI
variable to false in the build command or inside the package.json files and most importantly, adhering to best coding practices by rectifying the warnings.
Setting Environment Variables: A Guide to Modifying Process.Env.CI
Setting environment variables can be an intricate part of deploying your applications, particularly when using services such as Netlify. To understand how to prevent Netlify from treating warnings as errors due to
xxxxxxxxxx
process.env.CI = true
, it is essential we delve into the aspect of setting and modifying environment variables first. As a crucial point of note, the phrase ‘CI’ here stands for Continuous Integration, a development practice that requires developers to integrate code into a shared repository severally throughout the day.
Environment variables are essentially dynamic entities whose values can be changed at any given time. They have primarily been used for communication between processes. In JavaScript specifically,
xxxxxxxxxx
process.env
object is utilized in setting up these environment variables. Smilarly in the Netlify context, they are also designated locations where you can store sensitive data, like API keys or other credentials.
With this understanding, looking at the statement
xxxxxxxxxx
process.env.CI = true
in the context of Netlify deployment, it implies that continuous integration mode is activated. Here’s what happens: Any present warnings in your code will be treated as errors; therefore causing your deployments to fail should any warnings exist. This can be a desirable feature during development since it forces developers to write clean and warning-free code.
However, suppose you’d like to change this behavior for some reason, perhaps to allow deployments even if warnings are present. The solution lies within manipulating the
xxxxxxxxxx
process.envCI
value. By assigning a false value to this environment variable on Netlify, you can change their behavior towards handling warnings as errors:
xxxxxxxxxx
process.env.CI = false;
Implementing the above code snippet does not signal an end to the problem but rather provides an instantaneous fix. It’s imperative to also know how to set this variable properly within the Netlify user interface. Setting such environment variables directly in your code isn’t considered a best practice as it exposes sensitive data.
Instead, one should configure them within the build and deploy settings of their Netlify App:
- Login to the Netlify dashboard.
- From the list of your sites, select the site you want to configure.
- Navigate to “Build & deploy” settings.
- Under Environment, click on the “Edit variables” button. Here you can input your environment variable name (CI) and its value (false).
Incorporation of this strategy ensures that warnings do not halt your application deployment process. Yet,it’s advisable to explore these warnings since they often point out potential problems in the code, and might even be causing unforeseen issues.
As Joel Spolsky, Software Engineer and co-founder of Stack Overflow says, “The most important property of good code is legibility. It’s read far more than it’s written, so plan accordingly.” . Evoking his wisdom, meet warnings with a keen eye for underlying issues.
Why Does Netlify Treat Warnings As Errors and How to Change This Behaviour
A prominent question among JavaScript developers is why Netlify treats all console warnings as complete errors, causing builds to fail. This action occurs primarily because when a Continuous Integration (CI) environment runs a project’s build process, the
xxxxxxxxxx
process.env.CI
variable is automatically set to true.
This code signifies that expectedly negligible warnings should be treated with the same severity as errors. Due to this setting, minor infractions that would not affect your web app’s functionality can prevent successful deployments in Netlify.
Let’s understand the reason behind this:
– Preservation of Code Quality: An underlying principle here is maintaining superior code standards. It ensures developers solve potential issues at their earliest stages. Therefore, even warnings are taken seriously.
– Assist Debugging Process: When you treat warnings like errors, it aids early identification and fixes those issues that might spiral into bigger problems in the production phase.
To change this behaviour and prevent Netlify from treating warnings as errors, developers must modify Node.js environment variables or create a
xxxxxxxxxx
.env
file.
Here’s how to do that:
– Adjust the
xxxxxxxxxx
process.env.CI
variable by setting it to false. A practical example would be creating an environment file (.env) at the root level of your project directory, and inputting
xxxxxxxxxx
CI=false
.
– Modify the build command to manage the CI variable in package.json. So, instead of “build”: “react-scripts build”, use “build”: “CI=false react-scripts build”.
– In Netlify, go to site settings → Build & Deploy → Environment and add a new variable with the name CI and the value as false.
Each method has its pros and cons, therefore choose the one that suits best your project requirements.
Just as Leonard Euler once said, “Since the fabric of the universe is most perfect and the work of a most wise Creator, nothing at all takes place in the universe in which some rule of maximum or minimum does not appear.”, nothing happens without reason the same concept being implemented by Netlify. The approach to treat warnings as errors is a stringent measure to enhance code quality, push for early debugging, and prevent possible issues that might surface in production. But developers can modify this behavior according to their needs.
Keep in mind, making changes to these settings should be calculated and reasoned, as avoiding early warnings might lead to severe issues in the future. As with all coding decisions, consider the impacts and implications carefully before proceeding.
For more detailed sector-specific advice, consider exploring this support guide provided by Netlify community.
The strategy of mitigating the occurrence of warnings being considered as errors due to `process.env.CI = true` in Netlify involves thorough understanding and subtle handling of the CI environment. The provided roadmap illustrates key steps toward successfully preventing this warning-error transposition and maintaining a smooth deployment process in Netlify:
Step | Description |
---|---|
Navigate to your Build settings | Firstly, you need to access the Build & Deploy settings within the Netlify Dashboard. |
Edit Environment | In the Build settings, locate the Environment section. This is where all your environmental variables are managed. |
Add new variable | By clicking on the ‘Edit Variables’ option, you can add a new key-value pair. In this case, the key should be `CI` and it’s value ought to be `false`. |
Save settings and redeploy | After saving the new changes, you need to initiate a site rebuild to let the effect take place. |
Thereafter, the Continuous Integration (CI) environment in Netlify will no longer treat warnings as errors, ensuring your applications are deployed without any undue interference.
To demonstrate this more pragmatically, here’s a small piece of code that handles environmental variables:
`
xxxxxxxxxx
process.env.CI = false
`
Explicitly setting the `CI` to `FALSE` ensures that the build does not fail on warnings, thereby preventing a systematic halt over minor issues during the deployment procedure.
As Ian Sommerville, a renowned software engineer once quoted, “Software development is not a production process, but rather a creative one”. By this, we understand that some flexibility is essential in recognizing and handling non-fatal alerts without obstructing the whole deployment process. Each developer’s understanding of system variables and command-line arguments can thus be effectively applied to devise bespoke solutions tailored to their specific needs.
For additional resources, you can check out Netlify’s Official Documentation on Environment Variables. Remember, every procedure requires a systematic approach, thus following the guidelines given above will surely help you achieve your target objectives.
By maintaining a balance between secure application deployments and allowing minor warnings to coexist, the integrity of the build processes remains uncompromised. Hence, the goal lies in implementing the `process.env.CI = false` command wisely, ensuring a noise-free build process while maintaining code efficiency at the same time.