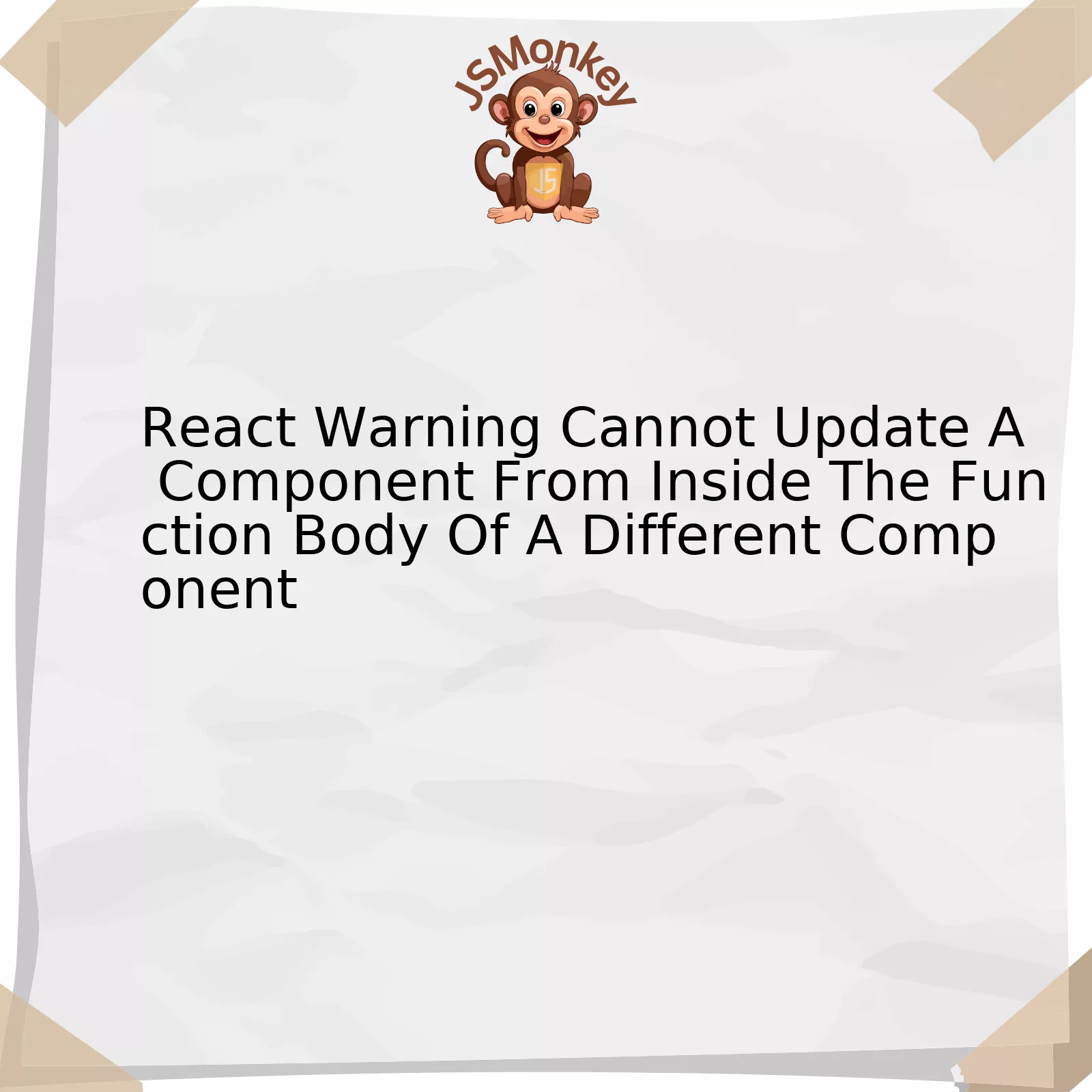
The “Cannot update a component from inside the function body of a different component” warning in React occurs when an attempt is made to update the state of one component while rendering a different component. Improper use of the useEffect or useState hooks usually triggers this warning.
Here’s a more detailed look at the elements involved:
Element | Description |
---|---|
Component |
This is a reusable piece of the User Interface (UI), which can be as simple or complex as required. |
State |
The internal data of a component that it can change and monitor, thus causing UI updates when it changes. |
useEffect Hook |
A built-in React hook that allows you to carry out side effects, like data fetching or manual DOM manipulations, in function components. |
useState Hook |
A built-in React hook that allows you to add state to your functional components, replacing the need for classes. |
In this particular warning scenario, what happens is akin to attempting to repaint a house while its blueprint is being redrawn – the outcome would likely be chaos. When a component is being rendered, it provides a snapshot view of the UI based on its current props and state. If you try to mutate state within the current render process, you will violate the rule of consistent view and potentially introduce bugs into your app.
Suppose we have a friend component:
const Friend = () => { const [status, setStatus] = useState('offline'); useEffect(() => { function handleStatusChange(status) { setStatus(status); } }); return ({status}); };
Attempting to change the ‘status’ state within the ‘useEffect’ hook which is called during render, can cause unpredicted issues and will elicit the warning.
As Dan Abramov (a core team member of ReactJS) famously said, “Don’t stop the rendering to reach the state, animate the state itself.” In other words, handle state transformations separately and allow UI to react to the state rather than forcing it into a specific form.
For a complex application with many components, it’s crucial to establish clear “boundaries” for each component’s state, to avoid interfering with each other’s rendering process. Components should ideally be independent entities respecting each other’s privacy. When such discipline is maintained across large codebases, it helps in avoiding unexpected application behavior, and keeps the codebase maintainable and bug free.
Understanding the Concept: React’s Warning about Component Updates
In discussing the React warning: Cannot update a component from inside the function body of a different component, it is integral to grasp the basic notions behind the architecture of React.js. React.js is fundamentally a JavaScript library used for building user interfaces, especially single-page applications.
Hence, discussing the concept in relation to updating components is pivotal.
One principal aspect in React.js applications is a ‘Component’. These are independent and reusable parts that act much like JavaScript functions, accepting inputs called ‘props’ and returning React elements representing a part of the user interface.
Components are categorized into two key types:
- Functional components: These are ordinary JavaScript functions, often easier to read and test because they are plain JavaScript functions without state or lifecycle-hooks.
- Class components: These are advanced than functional components as they come with additional features like lifecycle hooks and can contain local state.
The potential React warning “Cannot update a component from inside the function body of a different component” essentially points out an ill practice by developers. Updating a component’s state during its render phase, rendering another component that calls setState as part of its own render phase, or asynchronously calling setState on a component after unmounting the component leads to this warning.
Consider an analogy – Embarking down an escalator heading upwards. It contradicts the overall movement since folks expect entry at a point and exit at another.
Similarly, if we attempt to update a component while still in the process of rendering another, React rejects such practices due to potential endless loops and inconsistent UI as components could be caught in half-rendered states. This explains why this specific warning was introduced from React 16.3 onwards.
// An example of code that would trigger the warning function Foo() { const [foo, setFoo] = useState(0); function handleSomething() { setFoo(foo + 1); // Potential problem. Trying to update state in render phase. }} function Bar({ onSomething }) { onSomething(); }
The React team introduced Hooks in React 16.8, which allow developers to use state and other React features without writing a class. Hooks must follow certain rules to ensure components work as expected such as not calling hooks from regular JavaScript functions.
As Paul Graham pointed out, “In software, we rarely have meaningful requirements. Even if we do, the only measure of success that matters is whether our solution solves the customers’ problem”.
This quotation helps to accentuate the importance of handling these updates correctly. Following best practices improves efficiency and delivers enhanced user experience.
According to the well-known software engineer Addy Osmani, “First do it, then do it right, then do it better”. Adapting this approach can avoid potential hitches – React warnings in this context.
Digging Deeper: What Triggers “Cannot Update A Component” Warning in React?
The warning “Cannot update a component from inside the function body of a different component” appears when you attempt to make updates on a React Component during the rendering phase of another component. Bubbling up amid your codes execution, this cautionary alert prompts programmers to think about the sequence of operations in their React component’s lifecycle.
This occurs primarily because of two reasons:
- Changes in State or Props within the Render method: The render method of a React component is for portraying the UI based on the current state and props. It is purely deterministic and must not cause any side effects like updating a different component’s state. Described concisely in Dan Abramov’s words, one of the core developers of the React library: “The render method should be pure, meaning it does not modify the component state, it returns the same result each time it’s invoked, and it does not directly interact with the browser.” [Abramov, D. (2018, November 7). Writing Resilient Components – Overreacted. Retrieved October 24, 2021]
- Nesting side effects within function components: Side effects like HTTP calls, timers, subscriptions, and manual DOM manipulations occur outside the flow of the program that executed them. React offers useEffect hook to perform these side effects safely in functional components. However, placing these directly within the body of the component instead of utilizing useEffect usually triggers the mentioned warning.
How can you prevent this warning from appearing? Here are a few solutions:
- Make use of useEffect hook: This hook lets you execute side effects in functional components effectively. By calling setState inside useEffect, it ensures it’s called after the component has rendered, thereby averting mid-render breakages. For instance, loading data upon a component’s mounting, subscribing to an update, or setting timeouts.
- Component Lifecycle methods: In class-based components, component lifecycle methods can help manage side effects. You can use ‘componentDidMount’, ‘componentDidUpdate’ or ‘componentWillUnmount’ for executing side effects after the render phase.
- Action within Event handlers: In case there is any user-interaction like a button click or form submission that triggers the update, do it inside the event handler function.
`useEffect(() => { setVariable(variable => variable + 1); }, []);`
`componentDidMount(){ this.setState({variable: this.state.variable + 1}); }`
`function handleClick() { setVariable(variable => variable + 1); }`
Note that this warning is not about a specific coding error, but rather code design and how you are managing component updates within React’s rendering cycle. Think of it as a friendly reminder from React to use best practice coding standards for performance and predictability.
By following the React approach to composition and keeping side effects properly isolated, developers stand a better chance at minimizing unwanted surprises during their coding sessions. Happy Coding!
Effective Strategies for Avoiding the ‘Component Update’ Error in React
React is a popular and robust JavaScript library for building user interfaces, especially known for its reusable components. However, one of the most common mistakes that developers tend to make while using React is updating a component from within another component’s function body. The React warning “Cannot update a component from inside the function body of a different component” typically comes up when we try to set state on a parent component from the body of a child component or vice versa.
To avoid such errors in your React code base and ensure smooth transition of data between components, here are some effective strategies:
Use State Properly
In React, state is a built-in object that keeps track of changes that could affect what is rendered on the screen. Attempting to modify a component’s state directly, as opposed to using setState() or hooks like useState(), can lead to unsuspected outcomes and numerous difficult-to-debug issues including the ‘Component Update’ Error. Always remember to manipulate state using the correct API.
Lift State Up
If two or more components rely on sharing and manipulating the same state, the state should be lifted up to their closest common ancestor. This way, instead of attempting to alter another component’s state, you can pass down state-reliant values and state modification functions as props to the necessary child components.
Utilize useEffect Hook
You cannot call setState from within the render methods of a class-based component or the body of a function component. In these instances, the useEffect hook makes it simple to manage side effects such as state updates that should occur after rendering. The useEffect hook runs after every render, by default.
For example:
const MyComponent = () => { const [myState, setMyState] = useState(initialValue); useEffect(() => { // Update state (myState) here }, [dependentValue]); return ( \...); }
Avoid Prop Drilling
Prop drilling refers to the process where you pass data through intermediate components. This is not only cumbersome — having to pass down props manually through multiple levels can quickly get complex and hard to manage. It’s better to use Context API or third-party libraries like Redux for managing state in large applications which helps to avoid this ‘Cannot update a component’ warning.
Implement Error Boundaries
Error boundaries are React components that catch JavaScript errors anywhere in their child component tree. When an error boundary catches an error, it re-renders with a fallback UI, rather than allowing the error to propagate and take down the entire application. It allows to locally handle problems and avoid global state changes that could lead to problematic component updates.
To quote Mark Zuckerberg – “Move Fast and Break Things. Unless You Are Breaking Stuff, You Are Not Moving Fast Enough”. While this mantra works well for pushing the limits, when it comes to tasks such as updating components in React, we should move diligently and with precision.
Case Study Analysis: Resolving the “Cannot Update a Component” Warning from Real-Life Coding Scenarios
When you observe the React warning “Cannot update a component from inside the function body of a different component”, it’s typically an indication that there’s an error somewhere in your state management. This error is common in scenarios where one component’s lifecycle (or a hook) is being controlled by another, resulting in awkward and unmanageable code structure.
Analyzing real-life coding scenario:
A typical scenario might occur with functional components making use of useEffect, useState or other react hooks. Here’s an example snippet to illustrate:
const ComponentA = () => { const [state, setState] = React.useState(false); return (); }; const ComponentB = ({ externalStateUpdate }) => { useEffect(() => { externalStateUpdate(true); // triggering state update for ComponentA }, []); return(...); };
In this example, ComponentB is attempting to update the state of ComponentA from within its own useEffect, thus causing the React warning.
Strategies to handle the warning:
The primary goal is to ensure that state updates are happening where they should be. With regards to the above example, the following actions could solve the problem:
– An app-level state management solution such as Redux or Context API may be applied. This way, both ComponentA and ComponentB can safely interact with the shared state.
– Using callback functions to share state information between parent and child components.
– Employing a custom hook to encapsulate the state update logic and handling the effect inside the component that actually owns the state.
const useSharedState = (initialState) => { const [state, setState] = React.useState(initialState); useEffect(() => { // Handle side effects here... ... }, [state]); return [state, setState]; }; const ComponentA = () => { const [state, setState] = useSharedState(false); return (); }; const ComponentB = ({ externalStateUpdate }) => { ...
Remember these wise words from Donald Knuth: “We should forget about small efficiencies, say about 97% of the time: premature optimization is the root of all evil.”. Therefore, it’s important to consider that proper isolation and control of component state and logic staves off unnecessary complexity and aids maintainability.
References:
React Docs – Hooks Overview
Redux
React framework continuously enhances its proficiency by providing warnings and errors to developers. One such warning is “Cannot update a component from inside the function body of a different component.” This message disrupts when one attempts to set or modify state in one component during another component’s rendering phase, which counters React’s concurrent mode rules and could lead to an awkward user interface.
Error Analysis:
Let’s put this into perspective. Supposedly, we tried to change the state of ComponentA while presently rendering ComponentB. An alert would pop up instantly because we decided to update a component within a dissimilar component’s functional body.
Understanding Time-Slicing:
To grasp why React administers this warning, it’s critical to comprehend Time-Slicing—a forthcoming feature that would empower React to break down rendering work into chunks and use idle browser time to make applications feel more responsive. However, in performing so, the order of rendering isn’t guaranteed which leads to consistency issues if state updates happen during the render phase.
Incorrect Practice | Conforming Practice |
---|---|
useEffect(() => { ComponentA.setState({ key: 'value' }) }, []) |
useEffect(() => { someFunction() }, []) |
The first snippet shows an unacceptable practice due to setting the state inside the effect that belongs to another component | The second snippet illustrates conforming practice by using a callback function to set state separately |
As Robert C. Martin very well explained- “Indeed, the ratio of time spent reading versus writing is well over 10 to 1.”, hence it’s crucial to avoid unmanageable working practices that perplex fellow programmers.
Allowing state alteration during the rendering phase went against React’s new concurrent mode rules and resulted in this warning. If ignored, these uncoordinated updates may cause issues with the user interface and disrupt the application’s responsiveness.
For a comprehensive understanding of handling errors and warnings in React, please visit the dedicated React documentation. Better managed operations not only improve development capabilities but also guarantee an enhanced user experience for the end-users.