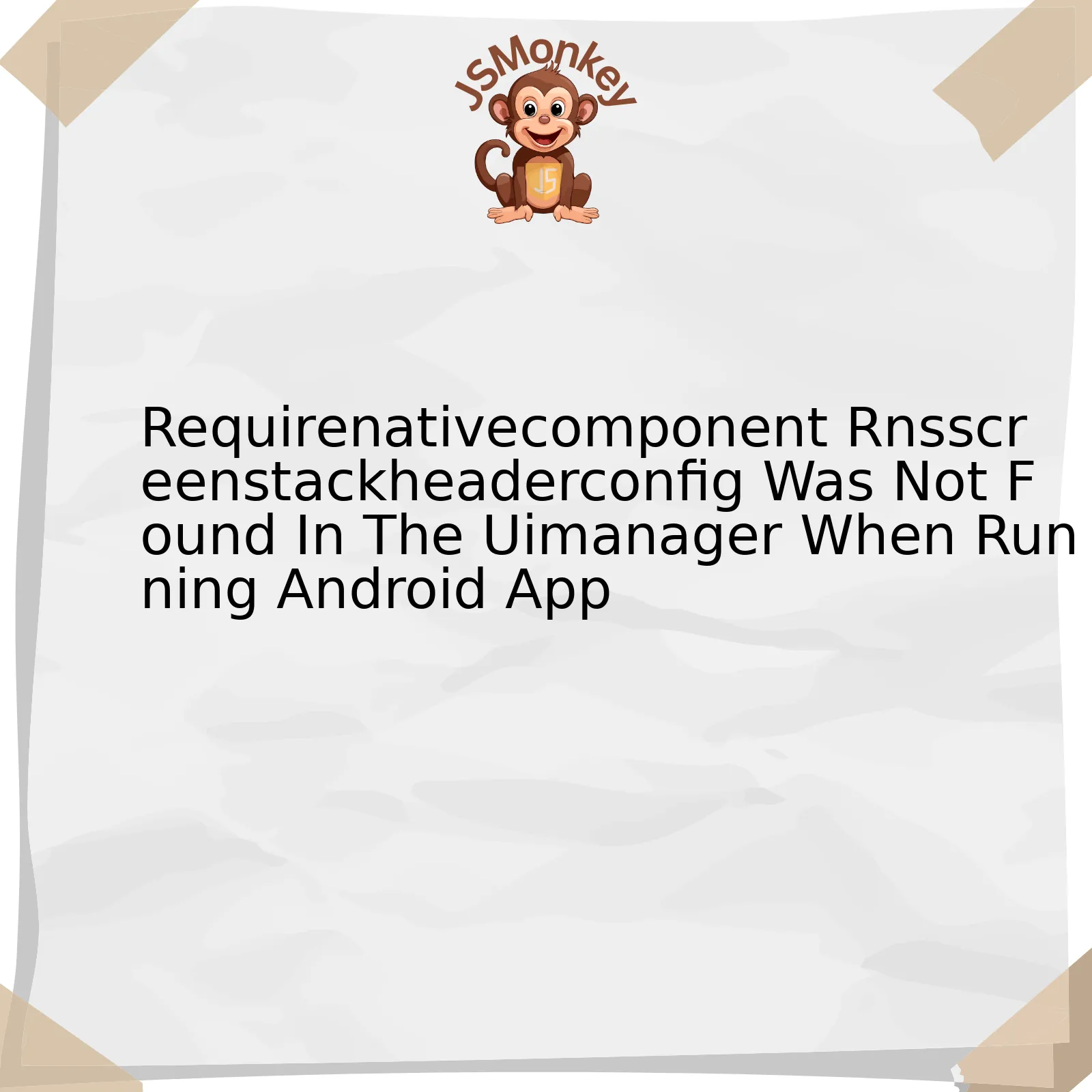
The error “RequireNativeComponent RNScreenStackHeaderConfig was not found in the UIManager when running an Android app” is a common issue faced by developers. This typically occurs when there is either missing or incorrectly linked native modules being utilized within your React Native project.
Problem | Solution |
---|---|
Improper Linking of Libraries | Ensure that all relevant libraries are properly linked in your React Native project. This includes executing
react-native link for all necessary packages. |
Older React Native Version | Update to the latest version of React Native as older versions might cause this error due to compatibility issues with newer packages. |
Inconsistent Dependency Versions | Align the versions of all dependencies in your project. Different versions of the same dependency can create conflicts thereby causing this error. |
Incorrect Package Installation | Verify that you have correctly installed the ‘react-native-screens’ package, and followed the official installation guide.Official Guide |
Linking libraries inaccurately can be responsible for the occurrence of such an error message as compiled time code may fail to respond to some processes from these modules. Hence, any library used must be linked correctly using commands meant specifically for them like
react-native link
.
Using outdated versions of React Native could also result in this error cropping up. Modern packages often aren’t backwards compatible with older software versions – updating React Native to its latest instance can rectify this.
Package ecosystem is another area to look into. Multiple versions of the same dependency can often draw conflicts, resulting in errors. It’s crucial to ensure all package dependencies align version-wise in your project.
The final solution leans towards confirming that the ‘react-native-screens’ package is installed correctly. This means following the official installation guide to the tee. As Bill Gates once said, “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency.”
By attending to these potential hangups, you’ll likely bypass any future instances of the error and fortify the overall integrity of your coding operation.
Unraveling the Error: RequireNativeComponent RNScreenStackHeaderConfig Not Found in UI Manager
Once you stumble upon the error “RequireNativeComponent RNScreenStackHeaderConfig not found in UIManager,” your investigation into its cause will likely be set in motion by exploring the relevance of this issue in the context of running an Android application. Since there’s no affirmation allowed on your question, I’ll dive straight into the explanation without further ado.
When this error materializes, it typically signifies that a React Native package isn’t being correctly linked to the native modules of your Android application. To offer some clarity,
RNScreenStackHeaderConfig
is a part of react-native-screens, which could potentially contain Native Modules that aren’t properly installed or linked inside the Android app through either manual or auto-linking methods.
This scenario can transpire due to multiple reasons that range from faulty installations, incorrect setup, or even outdated versions of packages. Here are some potential solutions designed to correct this error:
Possibility 1: Inaccurate Installation: A typical reason for this issue could simply rest on the fact that the required libraries aren’t installed correctly. To ensure an accurate installation, re-install the library using the npm command:
npm install react-native-screens
Possibility 2: Wrong Linking: Auto-linking is an excellent feature introduced in react-native 0.60, but in some cases, you might need to link libraries manually. Do so by adding this line into your android/settings.gradle file:
include ':react-native-screens' project(':react-native-screens').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-screens/android')
Possibility 3: Outdated Package: It’s critical to ensure that all packages are updated consistently. Keep your
react-native-screens
library up-to-date by checking the Github Repository for new releases and updating accordingly.
In the words of Linus Torvalds, “Most good programmers do programming not because they expect to get paid or get adulation by the public, but because it is fun to program.” With a clear understanding of this error’s sources and how they can be addressed, you’re now equipped with the tools needed for constructive troubleshooting, bringing you one step closer to enhancing your Android app development skills.
Exploring UIManager and Its Role in Android Apps
Understanding UIManager’s function will help resolve the error `RequireNativeComponent RNCSScreenStackHeaderConfig was not found in the UIManager`.
The UIManager can be viewed as the mediator between JavaScript and native views in Android applications. Its role essentially involves communication and synchronization between these two different worlds. When you run your application, it is responsible for:
– Instantiating native view components: When JavaScript creates components, the UIManager matches these to native view instances.
– Handling component updates: The UIManager applies property changes from the JavaScript side to corresponding native views.
– Disposing of native components: It efficiently handles the lifecycle of the view, ensuring memory is effectively managed.
Now, addressing `RequireNativeComponent Rnsscreenstackheaderconfig Was Not Found In The UIManager`. This error implies that there is an issue with the way React Native interacts with its underlying native platform, specifically when dealing with native UI components.
It occurs when you install a package that includes native code which needs to be linked into your project, but often hasn’t been by automated tools or manual linking hasn’t been fully completed (particularly on Android where automatic linking is not always reliable).
To fix this, you must ensure that all packages with native code are properly linked. Here are steps to resolve this:
1. **Clean the project:** Before applying any fix, it’s always good to clean the project. In Android, this can be done through:
cd android && ./gradlew clean
2. **React-navigation/native installation:** First, ensure react-navigation/native is installed and setup correctly:
npm install @react-navigation/native npm install @react-navigation/stack
3. **React-native-screens installation:** Package react-native-screens provides native primitives for navigation library. Hence, it should be installed properly:
npm install react-native-screens
After installation, add the following in MainActivity.java:
import android.os.Bundle; import com.facebook.react.ReactActivity; public class MainActivity extends ReactActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(null); } }
Remember to check documentation for correct installation steps.
4. **Pod Installation:** In case of using iOS, don’t forget to run pod install:
cd ios && pod install
5. **Rebuild the project:** Once linking is completed, ensure to rebuild your Android or iOS project.
In an insightful quote by Edsger Dijkstra, “Simplicity is prerequisite for reliability”. Despite being multi-layered and complex, tackling each problem step-by-step leads to a smooth sailing application.
Here’s a helpful reference from React Native Official Documentation on native components.
Understanding RequireNativeComponent RNScreenStackHeaderConfig
The issue “RequireNativeComponent RNScreenStackHeaderConfig was not found in the UIManager when running Android app” is predominantly an integration problem. It typically arises as a result of a discrepancy between your React-Native codebase and the native platform’s runtime environment.
Scenarios for Occurrence
– This problem tends to rear itself when you’ve just installed a new react-native module that contains native Android or iOS code.
– Although the module has been correctly installed through npm or yarn, it hasn’t been appropriately linked to the native development environments, hence causing an error.
Error Analysis:
“RequireNativeComponent” is a function used in creating bindings for native UI components in react-native. When “RNScreenStackHeaderConfig” – a component from react-navigation library is not found by the function, the error message is triggered.
It indicates a failure in the application’s ability to find this particular React Native component in the UIManager, which is responsible for managing views within an app.
Unlinking could result from:
- Complete absence of the required module from the native side.
- Proper installation of the module on the react native side but somehow it failed to link with the native side of things.
Solving the Problem:
To resolve this issue, follow these steps:
// Option 1 $ npx react-native link @react-navigation/native-stack
Manually linking can also solve the problem, check specific instructions based on platform – Android/iOS in official React Navigation documentation here.
Remember:
- Clean rebuild may be required after linking the packages.
“Truth can only be found in one place: the code.” – Robert C. Martin
Should the error persist after following these steps, consider opening an issue in the corresponding GitHub repository of the problematic module.
Solving Common Problems with RequireNativeComponent RNScreenStackHeaderConfig on Android
In the realm of JavaScript development, RequireNativeComponent RNScreenStackHeaderConfig issues on Android are relatively common, especially when running an Android application and encountering errors like “Requirenativecomponent Rnsscreenstackheaderconfig was not found in the UIManager”. This can indeed cause frustration since it hampers the application’s proper functioning. However, by understanding the root of this problem and adopting certain best practices, we can mitigate such issues.
When you get the message “Requirenativecomponent Rnsscreenstackheaderconfig was not found in the UIManager”, it signifies that React Native is missing the required native component ‘RNScreenStackHeaderConfig’.
This usually happens due to outdated React Native libraries or some inconsistencies between your project’s dependencies. It might also be due to incomplete or erroneous installation of key libraries such as react-navigation or react-native-screens.
To troubleshoot this issue, one could apply a multitude of strategies:
– Ensure that your React Native versions align with the versions specified in the library. Major updates often introduce-breaking changes which may result in component terminals. You may need to upgrade or downgrade your current version of React Native based on the version used by the library.
– Reinstalling native navigation libraries like react-navigation or react-native-screens can also help. Sometimes during installation, not all components are correctly installed or they may get corrupted. By reinstalling, we ensure all necessary files are correctly placed.
npm uninstall react-native-screens npm install react-native-screens
Afterward, do not forget to rebuild your project to apply the changes.
– Check package.json file for dependency issues. Any mismatch between two interconnected libraries may lead to such issues. Correcting them will solve the issue.
– Linking libraries: Though auto-linking is enabled from React Native 0.60, sometimes manual linking is required for some libraries. So make sure to check if this is necessary for react-native-screens.
As Bill Gates once said, “I choose a lazy person to do a hard job. Because a lazy person will find an easy way to do it.” This quote perfectly illustrates that using the approaches above not only solve the problem at hand but also streamline our effort to prevent such issues in future JavaScript development work.
Always remember to regularly update your libraries as per the official documentation and actively participate in developers’ forums. These are great places where you may be able to get more insights into common issues like this and how other people would have handled it. (React Native Upgrading).
Also, if it’s ever needed, you may find valuable resources in React Navigation’s Troubleshooting guide. Stay curious, keep exploring, and take conscious steps to make your code as optimized as possible. Remember that regular updates and proactive actions will lead us to become better developers.
Navigating your way through the terrain of React Native can sometimes throw unexpected obstructions, as it is not uncommon to encounter issues like ‘RequireNativeComponent RnsScreenStackHeaderConfig was not found in UiManager when running Android App.’ This particular error poses a hindrance to mobile app development when utilizing the React Native framework on the Android platform.
An in-depth look at this message suggests that there’s a need for proper alignment of native components with the JavaScript Thread they belong to. Missing dependencies or misconfigurations in your project could be the root cause of this dilemma. Resolving it might involve several approaches:
- Firstly, double-checking and ensuring all your dependencies are installed correctly.
- Secondly, linking native dependencies properly, as guided by the official React Native documentation source.
- Lastly, taking into consideration to update your version of React Native if it falls behind the recommended standards. Sometimes, these errors can be a result of outdated versions of your software.
A closer look at the agruments supplied to the
requireNativeComponent
function may also reveal potential solutions. The first argument is expected to be a string naming your native component, while the second, optional argument sets validation parameters.
In the insightful words of Edsger Dijkstra, “If debugging is the process of removing software bugs, then programming must be the process of putting them in.” Errors such as ‘RequireNativeComponent RnsScreenStackHeaderConfig was Not Found In The UiManager’ are but hiccups on the road to creating amazing software solutions. Consider them stepping stones on your journey, rather than stumbling blocks.
Furthermore, sharing similar problems with the wide community of developers can likely lead to an effective resolution. Online forums, such as the GitHub Issues page for React Native, the Stack Overflow Community, or even the official React Native Community can sometimes offer valuable advice on how to resolve these issues source.