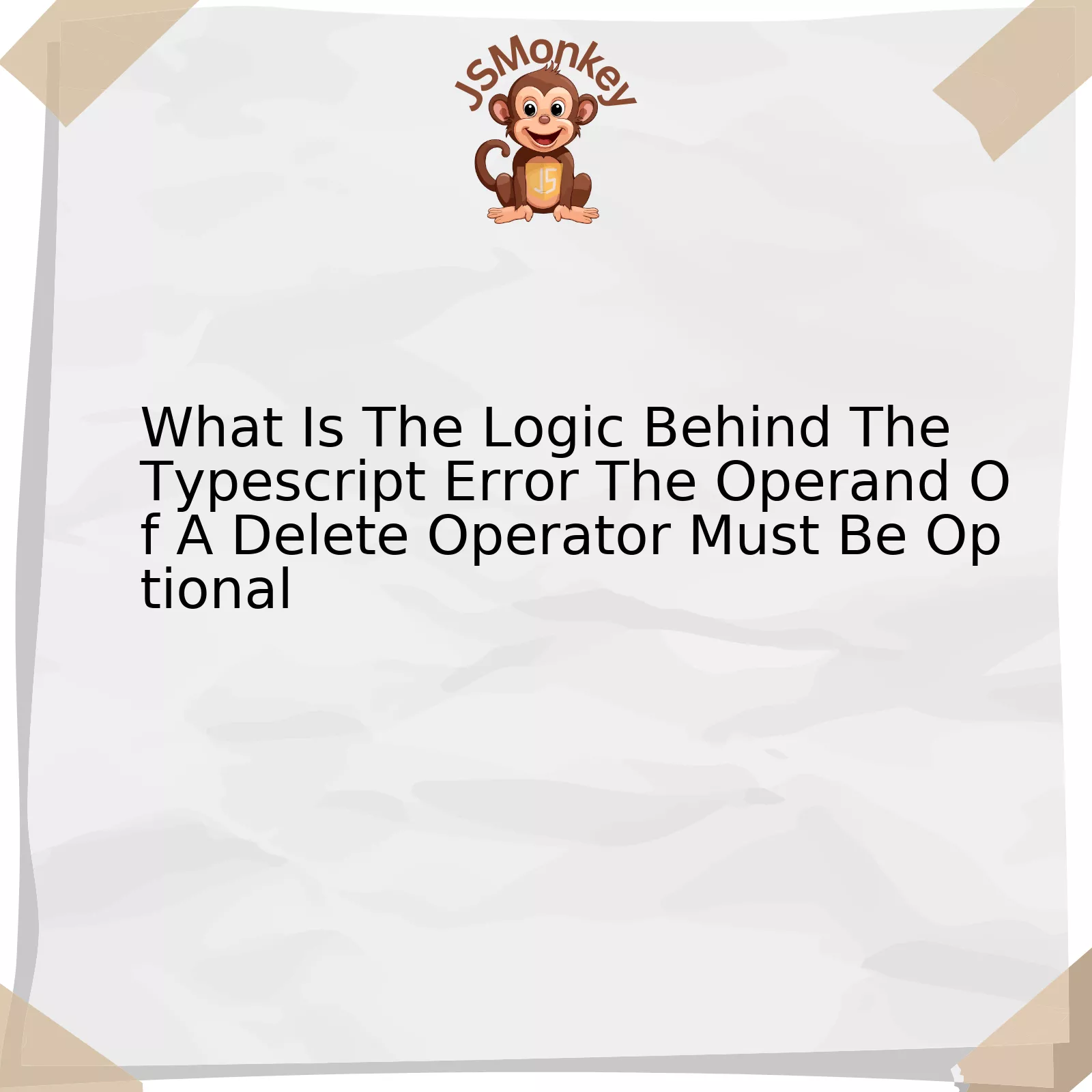
The TypeScript error “The operand of a delete operator must be optional” can be a bit disconcerting to those unfamiliar with it. To better understand the logic behind this error, we need to delve into TypeScript’s underlying principles and how they interact with JavaScript’s `delete` operator.
Let’s start by illustrating this concept through a table.
Concept | Description |
---|---|
TypeScript Static Type Checking | TypeScript is equipped with static type checking, which allows early detection of potential errors in the code during development phase. |
Object Properties | In JavaScript, properties can be added or removed from objects dynamically. In TypeScript, the types are used to infer object shape, making properties essentially non-optional to maintain. |
‘Delete’ Operator | The ‘delete’ operator in JavaScript is used to remove properties from an object. |
Error: Operand of a Delete Operator Must Be Optional | This TypeScript error is triggered when you try to use ‘delete’ on a property that hasn’t been defined as optional in TypeScript. |
Now, let’s dive deeper into these aspects and distill the logic behind this TypeScript error.
TypeScript is a typed superset of JavaScript, meaning it adds certain features on top of JS for enhancing developer productivity and code quality. One such feature is static type-checking, which provides developer feedback about potential issues in the code at compile-time, rather than at runtime (as is the case with JavaScript).
JavaScript is dynamic by nature and allows adding or removing properties from an object. But TypeScript, due to its type inference, has a clear shape (or structure) to objects defined by types and interfaces. Deleting a property that is part of this defined shape does not match the static nature anticipated by TypeScript.
This is where the `delete` operator comes into play. The `delete` operator in JavaScript allows developers to remove properties from an object. When used on a property that is part of the statically typed shape in TypeScript, it poses an issue since TypeScript expects that property to exist based on object’s definition.
If you attempt to delete such a property, TypeScript will throw the error “The operand of a delete operator must be optional.” Essentially, the runtime behavior (deleting the property) is trying to contradict the compile-time contract (the property should exist), hence the error.
An applicable coding example:
typescript
interface MyObject {
nonOptionalProp: string;
}
const myObj: MyObject = { nonOptionalProp: ‘Hello World’ };
delete myObj.nonOptionalProp; // Error: The operand of a delete operator must be optional
In the context of TypeScript definitions, if a property should indeed be removed at some point in the code, it should be defined as optional with the use of ‘?’ symbol:
typescript
interface MyObject {
nonOptionalProp?: string;
}
const myObj: MyObject = { nonOptionalProp: ‘Hello World’ };
delete myObj.nonOptionalProp; // No Error
Remembering the words of Addy Osmani, a Google engineer and author specialized in JavaScript, “_Being aware of JavaScript’s failings can help you create more robust designs_”, this TypeScript feature contributes majorly to the robustness and reliability of your application by guarding the sanity of your object’s shapes and minimizing destructive operations. This is seen particularly via the adherence to defining properties, which might be susceptible to removal, as explicitly optional, fostering better code organization and maintainability.
Understanding Typescript Errors: The Operand of a Delete Operator Must Be Optional
If you’ve ever encountered the TypeScript error message stating, “The operand of a delete operator must be optional,” then you would understand that it could be both perplexing and complex to navigate. Nevertheless, it is essential to comprehend the reasoning behind this TypeScript characteristic for robust code execution and effective debugging.
This error is fundamentally based on JavaScript’s delicate relational bond with TypeScript. Fundamentally, TypeScript is merely JavaScript at its core but embodied with types. This functionality enhances JavaScript with static types which augments the readability, predictability and reliability during runtime.
The statement
delete object.property
in JavaScript smoothly deletes a property from an object. However, the same does not hold without reservations in TypeScript which extols additional type safety. TypeScript aims to offer a compilation environment where the behaviour of your code mirrors as closely as possible to its runtime behavior. Ideally, once a property has been defined as part of an object’s type, it should always exist on that object, and deleting the property violates this principle.
Here’s why: imagine that we have an interface defined like this:
interface User { name: string; age: number; }
And a user object like this one:
let user: User = { name: "John", age: 25 };
If we were to execute
delete user.age
, we’d invalidate our `User` interface, because there would no longer be an `age` property on our `user` object. That would defy the purpose of TypeScript’s type-checking feature.
To work around this issue, we’ll need to make any properties that may be deleted at runtime `optional` in our TypeScript interfaces. Optional properties are denoted using the question mark (`?`) syntax:
interface User { name: string; age?: number; // age is now optional }
Our `user` object is still a valid `User` even if we delete the `age` property:
delete user.age
– since TypeScript views this property as optional.
Understanding these core concepts and adaptively applying them to your JavaScript codes will not only equip you with robust coding abilities but also give you an in-depth understanding of the efficient TypeScript environment.
“The most effective debugging tool is still careful thought, coupled with judiciously placed print statements.” Remarkably, this quote by Brian Kernighan, a renowned Canadian computer scientist, resonates very well in this context about understanding TypeScript errors. Being mindful of potential pitfalls, and planning for them in our code, can save us a lot of debugging time in the long run.
Exploring the Fundamentals: Why must the Operand in Delete Operators be Optional?
The `delete` operator in JavaScript is a powerful tool that allows developers to remove a property from an object. While this utility can be invaluable when managing dynamic data structures, its usage warrants caution; inadvertent removal of a crucial property could lead to significant errors and system instability. Therefore, a question emerges: why must the operand of a ‘delete’ operator be marked as optional within TypeScript?
In answering the question, we’ll dissect the fundamental reasoning behind TypeScript’s design decision for this error ‘TS2790: The operand of a ‘delete’ operator must be optional’.
-
Implications of Non-Optional Properties:
If a developer indicates a certain property as non-optional during declaration in TypeScript, it implies that the property has been considered necessary, or essential for the functioning of the application. When an attempt is made to delete this indispensable property using the `delete` operator, TypeScript raises an alarm in the form of the error message `TS2790`. This is TypeScript’s way of safeguarding potential system crashes due to unintentional erasure of vital properties.
-
TypeScript's Strictness :
The structure of TypeScript is essentially stricter than JavaScript. TypeScript discourages practices which trigger high possibilities of runtime errors. Using the `delete` operator on a non-optional property creates uncertainty about whether the object will maintain its type correctness after the operation. A significant feature of TypeScript is its ability to ensure type safety, thus a flag will be raised in advance before any potential issues occur.
-
Encouragement to Use Optional Properties:
With the requirement that the operand of a `delete` operator must be optional, TypeScript encourages prudent programming. Making a property optional is a clear sign that it might not always be present, and could be deleted at some point within the application lifecycle. Such a coding style ensures developers think through their code design before implementation, leading to predictable and consistent results.
Here’s a small demonstration:
type User = { id: number; } let user: User = {id: 123}; delete user.id; //Will throw the error `TS2790`
And if we alter the code passage just slightly by marking the property as optional:
type User = { id?: number; //`id` is now an optional property } let user: User = {id: 123}; delete user.id; //Will not throw any error
As Edsger W. Dijkstra, one of the major influences in computing science, once said, “Programming is one of the most difficult branches of applied mathematics; the poorer mathematicians had better remain pure mathematicians.” TypeScript, with its strict rules like the requirement for optional operands on deletion, serves as a mathematical enforcement aid. It helps developers deliver robust, reliable, and less buggy applications, despite the initial increase in complexity.
References:
- ECMAScript® Language Specification – Optional properties
- TypeScript Handbook – Property Modifiers
Decoding the Logic Behind Typescript Error and Usage of ‘Optional’ in Delete Operator
The TypeScript error “The operand of a delete operator must be optional” arises when the property that is to be deleted from an object has not been declared as optional. The underlying logic behind this error is deeply founded in the TypeScript language’s strict type-checking feature.
In TypeScript, properties defined in an interface or object type are expected to always exist. This expectation makes it possible for TypeScript to provide reliable static type checking and autocompletion features. However, this standard behavior can become problematic when you want to delete a property from an object.
For instance, consider the following code:
interface MyObject { prop1: string; prop2: number; } let obj: MyObject = {prop1: 'Hello', prop2: 42}; delete obj.prop1;
This causes a TypeScript error because `prop1` is a required property, and TypeScript treats any attempt to remove it as an error.
To fix this issue, one should declare that the property is optional using the ‘?’ operator. Once a property is declared as optional, TypeScript recognizes that its presence on an object is not guaranteed, and thus allows the use of the `delete` operator. Here’s how we adjust the above example:
interface MyObject { prop1?: string; prop2: number; } let obj: MyObject = {prop1: 'Hello', prop2: 42}; delete obj.prop1; // This now works
So importantly,
* TypeScript’s strict type-checking enforces all object properties to be present unless they’re explicitly marked as optional.
* Optional properties, denoted by appending a ‘?’ to the property name in the object declaration, are properties that could either hold a value or be undefined.
* Try to only use the `delete` operator on optional properties to avoid generating a TypeScript error.
These bullet points demystify ‘Optional’ in the delete operator and the logic behind the TypeScript error stating “The operand of a delete operator must be optional.”
In the spirit of tying programming to real life, Jeff Atwood, the co-founder of Stack Overflow, once said1, “Coding is not just ‘how’, but also ‘why’. It’s useful to capture the reasoning while it’s fresh.” Engaging with these TypeScript errors and understanding the logic behind them not only helps us code better, but also appreciate the ‘why’ of how the language has been architectured.
Practical Guide on Navigating Through ‘The operand of a delete operator must be optional’ error in TypeScript
The TypeScript error “the operand of a delete operator must be optional” often appears when attempting to remove properties from an object. This error can seem puzzling at first, but by understanding the principles behind the TypeScript language you would gain insight into why this error occurs and how to successfully navigate through it.
Firstly, one of the key characteristics of TypeScript is its ability to enforce a strict set of rules during compile time. This is largely beneficial as it helps avoid potential pitfalls even before the code is run. One of these strict type checking rules applies to deleting properties from objects.
For instance, in JavaScript, properties could be effortlessly deleted from objects using the `delete` operator:
javascript
let obj = { x: 10 }
delete obj.x
Despite being valid JavaScript code, TypeScript presents with the error “the operand of a delete operator must be optional”, once this operation is attempted.
The reason for this is tied to TypeScript’s design philosophy. The main purpose of TypeScript is to provide types to JavaScript, creating a more robust codebase which makes catching bugs easier. For TypeScript, once a property has been defined on an object, it assumes that exists throughout your code’s execution.
So, when we attempt to eliminate a property with the `delete` operator, TypeScript acknowledges this action as attempting to change the structure of the typed object – hence raising an error. In essence, this error message is just TypeScript’s way of maintaining structural integrity throughout our application.
Before delete | After delete |
---|---|
{x: number} | {} |
However, there are specific cases where TypeScript allows the deletion of properties. If the property marked as optional using the question mark symbol (`?`) then we can safely delete the property without TypeScript throwing an error.
let obj = {x?: 10} delete obj.x
So, to circumvent the “the operand of a delete operator must be optional” error, it might sometimes require restructuring your code or object types to include optional properties if such a deletion operation is necessary.
A fundamental understanding of TypeScript’s type system and why this error occurs aids in helping developers avoid this issue and reinforces sound coding habits. As Robert C. Martin has said: “Indeed, the ratio of time spent reading versus writing code is well over 10 to 1. We are constantly reading old code as part of the effort to write new code. …[Therefore,] making it easy to read makes it easier to write.” [Source](https://www.oreilly.com/library/view/clean-code-a/9780136083238/) So, understanding this TypeScript error “the operand of a delete operator must be optional” is part and parcel of creating clean, readable, and maintainable code.
A key aspect to consider surrounding the TypeScript error “The operand of a delete operator must be optional” lies intrinsically within the core principles and rules that govern TypeScript. Fundamentally, TypeScript shrouds itself with a protective layer of type safety unparalleled by its counterpart – JavaScript.
Let’s travel deeper into this concept.
In TypeScript, any property that is ever assigned the ‘delete’ operator should be declared as optional. This rule is in place due to TypeScript’s static type checking mechanism. Essentially, when we try to delete a non-optional property in TypeScript, it incurs an error because doing so violates the very fundamental contract we’ve established for our object. This contract guarantees that certain properties will always be available.
Consider the following TypeScript code:
interface Foo { bar: string; } function doSomething(foo: Foo) { delete foo.bar; // Error: The operand of a delete operator must be optional. }
In this example, ‘bar’ is a non-optional property in our interface ‘Foo’. So, deleting ‘bar’ from any ‘Foo’ object would result in the violation of the established contract, causing TypeScript to emit an error.
The logic behind this error message is rooted deeply in guaranteeing type safety and predictability. It ensures developers can confidently access properties knowing they exist, thereby reducing the potential occurrence of unexpected errors at runtime. Let’s refer to Anders Hejlsberg quote, the father of Typescript:
“The overriding design goal for TypeScript is that it makes you more productive by catching errors in the development rather than in the field.”[source]
However, if the need arises to delete some property from our objects, making them optional is a viable option:
interface Foo { bar?: string; } function doSomething(foo: Foo) { delete foo.bar; // OK }
Here, by making ‘bar’ an optional field in the ‘Foo’ interface, its deletion no longer conflicts with TypeScript’s rules. This still embraces type safety and provides developers with flexibility for property deletion operations.
Understanding this key intersection between types and code execution ensures a comprehensive command over TypeScript. It underlines how TypeScript navigates a balance between enforcing stringent type safety while providing enough room to facilitate complex programming needs. The realization of this insightful TypeScript rule acts as a step towards informed coding practices and robust software development.