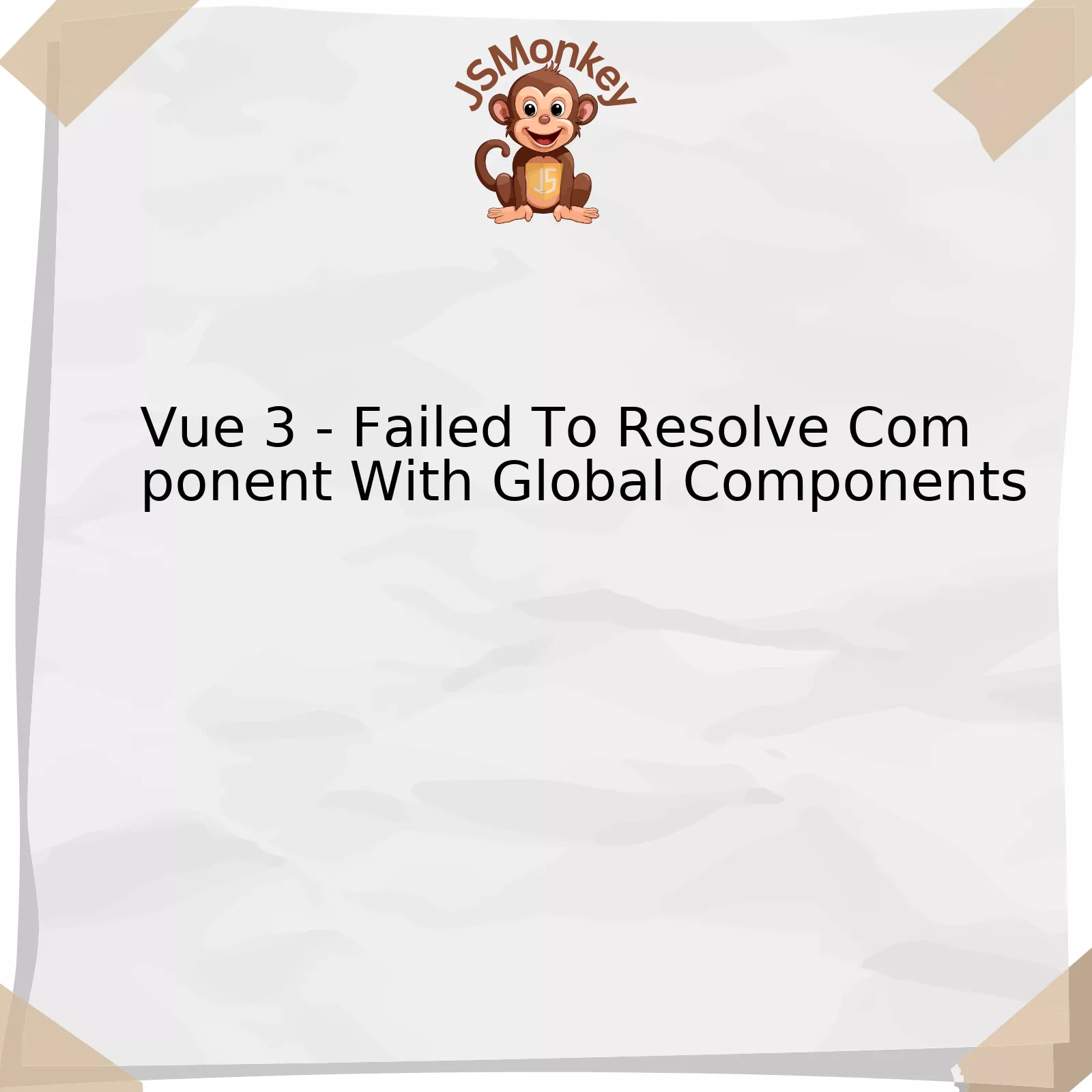
Vue 3 has introduced a change that could potentially lead to the error “Failed To Resolve Component” with globally registered components. In Vue 2, all globally registered components were available in every component’s scope which made it easy but also cumbersome managing larger Vue.js applications. Because of this, Vue 3 has changed the way how global components are handled. They are no longer automatically available in every component’s scope.
Let’s envision the relevant comparison between Vue 2 and Vue 3 in terms of global components:
Aspect | Vue 2 | Vue 3 |
---|---|---|
Global Components Availability | Automatically accessible in every component’s scope. | Not automatically accessible in every component’s scope. |
Registration Method | Registered using Vue.component() | Must be declared in the setup() method using app.component() |
Usability | Highly beneficial for smaller projects. | More flexible and scalable for larger projects. |
To define a component as global in Vue 3, you can use the following
code snippet
:
import { createApp } from 'vue'; import MyComponent from './MyComponent.vue'; const app = createApp({}); app.component('my-component', MyComponent); app.mount('#app');
In this snippet, the component `MyComponent` is defined globally by using `app.component()` method. This means that it is now available for usage throughout the whole application. If this procedure is skipped and you attempt to use a global component as it was possible in Vue 2, you will see the error: “Failed To Resolve Component” because Vue 3 is not aware of your global component.
It’s important to stress how reconstructed component registration helps to increase maintainability and performance in large scale applications. As Linus Torvalds once said, “Talk is cheap. Show me the code.” Vue 3’s approach walks us forward into a disciplined realm of component organization, offering room for complex projects to breathe and grow efficiently.
For more information about migrating from Vue 2 to Vue 3, you can check Vue’s official documentation site [here](https://v3.vuejs.org/guide/migration/global-api.html#globals-api).
Understanding the Root Cause of Component Resolution Failure in Vue 3
Understanding the basis for component resolution failure in Vue.js Version 3, especially as it relates to problems associated with the global components not being resolved, is a critical step towards more effective troubleshooting and resolution of JavaScript code errors. Such comprehension hinges on a deep awareness of Vue.js’s internal workings, particularly how Vue resolves components.
The
app.component
method is used to globally register or resolve components in Vue 3 systematically. While in Vue 2, global registration of components was achieved via
Vue.component
, Vue 3 adopts a slightly different approach with the new
createApp
method introduced by the Vue team. This change helps in ensuring that no global mutable state exists in applications developed using Vue 3.
VUE 3- Failed To Resolve Component With Global Components:
This issue could be due to multiple potential causes which include:
– Incorrect Component Registration: A leading root cause is often tied to incorrect global component registration. If correct syntax and methodology are not observed when registering components, Vue cannot resolve them, leading to errors.
For instance, consider a scenario where you attempt to register a component named ‘MyComponent’ globally within the main.js file as follows:
import { createApp } from 'vue'; import MyComponent from './components/MyComponent.vue'; const app = createApp({}); app.component('my-component', MyComponent);
When trying to use ‘MyComponent’ in a template later, if it is not called correctly (i.e., using its kebab-case name ‘my-component’), Vue will fail to resolve the component and throw an error.
– Use of Anonymous Functions: If anonymous functions are used to define Vue components, particularly those installed globally, the framework might have difficulties resolving them.
The solution to the ‘Failed To Resolve Component’ error largely depends on determining the exact cause of the problem. Simply ensuring proper naming conventions when declaring and using components in templates can potentially rectify the issue. As a best practice, use the kebab-case name (like ‘my-component’) in templates even if the component was registered with a camelCase or PascalCase name.
Bad | Good |
---|---|
javascript import MyComponent from ‘./MyComponent.vue’; export default { \\ template |
javascript import MyComponent from ‘./MyComponent.vue’; export default { \\ template |
As the renowned programmer Ada Lovelace once said, “The science of operations, as derived from mathematics more especially, is a science of itself, and has its own abstract truth and value.” Understanding the causal basis of errors is an inherent part of programming, and it rings especially true when dealing with Vue.js components.
Exploring Vue 3’s Global Components: A New Approach that May Lead to Conflicts
The new approach to global components in Vue 3, while potentially enhancing the flexibility and reusability of the framework, may also lead to scenarios where component resolution fails. This is an issue that can manifest itself particularly when switching from Vue 2 to Vue 3. Hence, understanding the intricacies of this system will be crucial for developers looking to use Vue at scale.
Understanding Vue 3’s Global Component Resolution
It is worth noting that Vue 3 introduces a sea change in how global components are handled compared to its predecessor, Vue 2.
In Vue 2:
- All globally registered components were accessible anywhere within the application, including child components.
In Vue 3:
- Global registration does not make them available everywhere anymore. They are only automatically available in template literals; they remain unavailable in single-file components unless manually imported.
Though this represents a more modular approach with fewer implicit dependencies, it poses a conflict with usages established in Vue 2, and has potential to throw up errors relative to failed resolution.
Globally Registered Components May Still Fail to Resolve
Hence, even if you have correctly registered your components globally in Vue 3, you may still find yourself facing a “Failed to Resolve Component” error. The subject of this discussion is this error that can occur when using global components in Vue 3.
VUE_WARN: Failed to resolve component: componentName
Here are some steps to troubleshoot:
- If the error persists despite verifying correct global registration of the components involved, cross-check the usage site. Remember, Vue 3 changed the rules: even globally-registered components need explicit importation when used outside of template literals.
- Keep track of naming conventions. Vue 3 is case-sensitive and treats component IDs differently from Vue 2.
However, this is not to dismiss the advantages that Vue 3 comes with. With its design approach, it aims to increase performance and maintainability by reducing implicit dependencies through the global registration system.
“Understand well as I may, my comprehension can only be an infinitesimal fraction of all I want to understand.” – Ada Lovelace, programmer and mathematician
For a more in-depth understanding, please refer to Vue’s official documentation here .
Therefore, while the new approach to handling global components in Vue 3 carries a learning curve and potentially troubleshooting some unforeseen errors, embracing it provides promising benefits in terms of components management and overall performance. Hence, moving forward, developers need to tackle these intricacies responsibly to make their journey with Vue 3 rewarding.
Troubleshooting Guide for “Failed To Resolve Component” Error in Vue 3
The “Failed To Resolve Component” error in Vue 3 commonly occurs when the Vue instance hasn’t correctly recognized a component. When working with global components, it can be particularly perplexing. Below is an in-depth guide to troubleshooting this issue.
One possible cause of the error message may be that you’re attempting to use a component that hasn’t been globally registered yet. When a particular component isn’t defined in the Vue instance, Vue will treat it like a native HTML element and produce the said error.
Error |
Vue.js cannot find the component because it hasn’t been declared. |
Solution |
Define your component in Vue by using the component registration scripts. |
For example:
// Import the necessary resources import { createApp } from 'vue'; import MyComponent from './MyComponent.vue'; // Create a new vue instance const app = createApp({}); // Register your component globally app.component('my-component', MyComponent) // Mount your application app.mount('#app');
Following the above code snippet ensures your component (`MyComponent` in this case) becomes universally recognizable across all parts of your Vue application.
Another root of the problem might involve incorrect component naming. It’s important to remember that Vue follows kebab-case when dealing with template components.
Error |
Vue is unable to work with component names formatted differently from its default naming scheme. |
Solution |
Ensure your component’s name matches Vue’s kebab-case convention in templates. |
So if your component’s name is `MyComponent`, you need to use `my-component` in your template.
As Bill Gates states: “I choose a lazy person to do a hard job. Because a lazy person will find an easy way to do it.” Following the guidelines, error messages, and conventions employed by Vue can simplify the resolution of this issue, saving you time for more complex aspects of development.
Ensuring components are globally declared before usage and proper naming convention adherence, which all goes hand-in-hand with effective problem solving and application debugging, can resolve the common issue of “Failed To Resolve Component” Error in Vue 3 within global components context. (Vue.js).
Implementing Solutions: How to Correctly Register Global Components in Vue 3
If you’re experiencing issues while trying to register global components in Vue 3, it may be due to how your global components are being registered. A common error that results during this process is “Failed to resolve component”. This error happens when there’s an attempt to use a component before it has been properly registered within the framework.
Let’s shed more light on different mechanisms available and their correct utilization for registering globally-required components in Vue 3.
Registering Global Components with App
In Vue 3, registration of global components shifted from using `Vue.component()` to leveraging APIs provided by the `app` instance that is returned by `createApp()` function.
Here’s a basic example code snippet on how this looks:
const app = Vue.createApp(App) app.component('MyComponent', MyComponent) app.mount('#app')
In this code snippet, ‘MyComponent’ is the name of the globally available component.
Using an Automatic Global Registration Method
If you have many components that you would like to register globally, an automatic global registration method might be beneficial. In this method, all components contained within a certain directory could be automatically registered as global components:
const app = Vue.createApp(App) const files = require.context('./GlobalComponents', true, /\.vue$/i) files.keys().map(key => { const name = key.match(/\w+/)[0] app.component(name, files(key).default) }) app.mount('#app')
This way, every vue component within the ‘./GlobalComponents’ directory will be registered as a global component.
The “Failed to Resolve Component” error basically means Vue doesn’t know about your component because you haven’t registered it yet. If you find yourself encountering this error message, it’s recommended to reconsider & verify the earlier steps performed to register the global component using one of the above methods.
Without forgetting, remember the wise words of Addy Osmani who said, “First do it, then do it right, then do it better.” When dealing with Global Components with Vue, following this iterative process can greatly help to cope with the learning curve and rapidly changing technology.”+”
The occurrence of “Vue 3 – Failed To Resolve Component With Global Components” is a frequent dilemma experienced when applying Vue.js 3 in development projects. This issue predominantly arises from a contrast in how local and global components are handled between Vue.js 2 and the Vue.js 3 version.
The error usually manifests when attempting to globally register components. The underpinning reason for this is the transition in Vue.js 3 to using an application instance for certain tasks where earlier versions used the Vue constructor, including registering global components.
The resolution to this circumstance consists of adjusting your component registration technique to align with the changes introduced in Vue.js 3.
Upon diagnosing the error as “Failed to resolve component”, consider checking:
- The import path and filename if the import statement is failing to locate the component.
- The component’s naming convention, ensuring it adheres to PascalCase or kebab-case rules.
- For proper use of application instances during component’s global registration.
When tinkering with existing systems for migrating to Vue.js 3, understand that:
- Rather than `Vue.component(‘MyComponent’, MyComponent);` you’ll instead be using the new application instances which look like this: `app.component(‘MyComponent’, MyComponent);`
- It’s essential to note, ‘app’ is not universally predefined. It is obtained by creating an instance of Vue: `const app = Vue.createApp({})`
Regarding these factors in the decision-making process can aid rectify the issue and enhance the smooth functioning with Vue.js 3.
It’s through a deep understanding of these elements and a commitment to solid design principles that software engineers can fully exploit the considerable power that Vue.js offers. As Facebook co-founder Mark Zuckerberg once said, “The biggest risk is not taking any risk. In a world that is changing really quickly, the only strategy that is guaranteed to fail is not taking risks.”
Take this moment to learn, adapt and grow with the improvements brought on by Vue 3 – For without it, you might miss out on an immense opportunity to bring your dev skillset one step closer to the future.