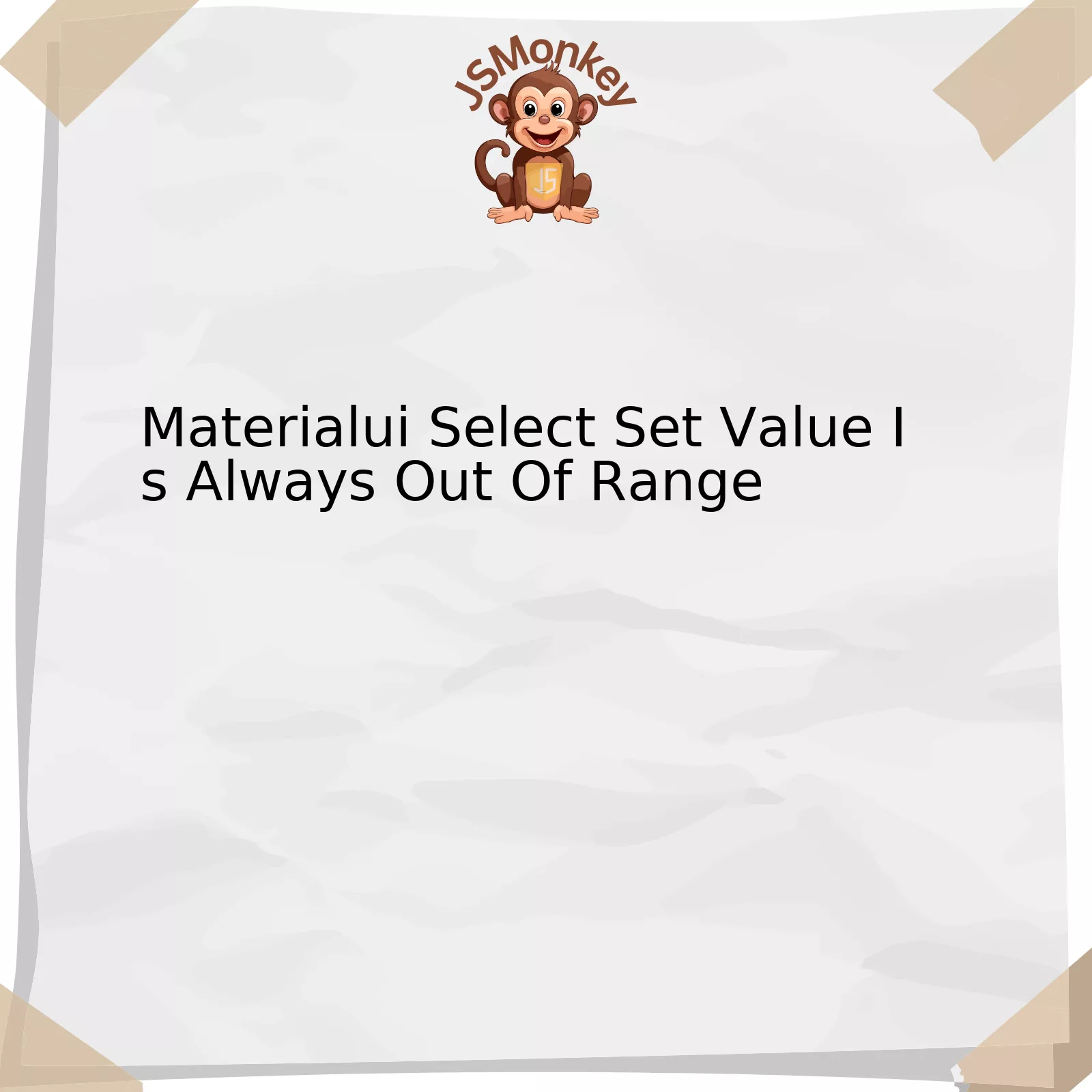
Error Type | Possible Causes | Solution Approach |
---|---|---|
Material-UI Select Set Value Out Of Range |
|
|
In a scenario where Material-UI Select set value is constantly reported as being out of range, it’s common that one of three issues could be causing this mishap: improper use of state management, a failed prop type — which happens when the value’s data type doesn’t match the expected data type; or the manifestation of conflicting values being passed into the Select component.
The state management strategy within your coding needs to be attentively evaluated. JavaScript’s
setState()
method helps maintain the state of an object. Deviating from its proper implementation might cause the set value to fall out of range. It’s pivotal to ensure all value changes are conveyed through the state for the component’s correct operation.
A mismatch in the data type of the assigned value can trigger a ‘failed prop type’ warning. Aligning the value’s data type with what’s expected by the Select Component – usually a string or a number – will resolve this issue. Data manual conversion is possible: numbers can be converted to strings using the
.toString()
method, and vice versa using the Number() function in JavaScript.
Conflicting values passed into the Select component, oftentimes as a result of being dynamically generated or fetched, can lead to out-of-range errors. Allocating unique value sets to each property parameter is central towards steering your path clear from discrepancies.
As Bill Gates once said, “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency. The second is that automation applied to an inefficient operation will magnify the inefficiency.” This speaks volumes for building clean, efficient code when using frameworks like Material-UI.
Understanding the Material-UI Select and Its Values
Material-UI is one of the most used React UI libraries due to its flexibility and wide array of components. One such component is the Select component, which presents the user with a list of options in a dropdown menu.
The ‘Select’ component from Material-UI works similarly to a regular select field, albeit with additional styles and features for enhanced user interaction. Its value represents the current selection and can be manipulated via React’s state management.
<Select value={this.state.myValue} onChange={this.handleChange} > <MenuItem value={10}>Ten</MenuItem> <MenuItem value={20}>Twenty</MenuItem> <MenuItem value={30}>Thirty</MenuItem> </Select>
In the above code snippet,
this.state.myValue
holds the current value of the select component. An error often encountered is “Material-UI Select set value is always out of range.” When you try to set a value that isn’t part of the MenuItem element, this exception will surface.
Interactively incorporating the use of an array when assigning values on each MenuItem can lower the chances of encountering such an error.
Let’s contemplate a situation where you have an array of objects dictating your MenuItems:
const myOptions = [ {name: "Option 1", id: 1}, {name: "Option 2", id: 2}, {name: "Option 3", id: 3} ]
You might utilize this array to auto-generate your MenuItem elements:
<Select value={this.state.myValue} onChange={this.handleChange} > {myOptions.map((option) => <MenuItem value={option.id}> {option.name} </MenuItem> )} </Select>
This way, you can better manage the range of your Select component, minimizing the chance for “out of range” errors.
Remember, when utilizing Select in Material-UI, always make sure that:
– The value attribute on the Select component corresponds to a legitimate value from within the MenuItem components
– When dynamically generating MenuItems from an array, ensure the array is correctly set up and the values assigned are consistent.
As Andy Hunt, one of the authors of ‘The Pragmatic Programmer’ once shared – “It’s all about finding the right abstractions”. This conceivably captures the goal of understanding Material-UI’s Select component while avoiding common pitfalls. Further reading on this topic could provide more practical understanding.
Range Issues in Material-UI Select: A Closer Look
Material-UI’s Select component is a powerful form control that provides an intuitive interface for users to select an item from a dropdown list. However, developers often encounter range issues when attempting to set the value of a Material-UI Select programmatically; specifically, end users might find the error message: “Material-ui select set value is always out of range”.
To understand the root cause of this problem, it’s important to first grasp how setting values in Material-UI Select components work. The `value` prop in the Select component is used to set a pre-selected value coming from a controlled input. This value should match one of the `value` props of the MenuItem components nested within the Select component.
Progressing from that premise, if you encounter a “out of range” error, it might likely be due to the following reasons:
- Input Value Not Matching MenuItem’s: If the assigned value to the Select component doesn’t match any of the MenuItems’ values, the compiler would return an out of range error because it can’t map the Select’s value to a valid MenuItem.
- Mismatched Data Types: The values assigned to the Select and MenuItems components must have the same data type (string, number, etc.). A mismatch could result in subsequent range issues.
- Asynchronous Data Loading: If your MenuItems’ data are fetched asynchronously – say, from an API call – there might be a delay in loading the options. Consequently, the system may attempt to assign a value to the Select component before its correspondents on the MenuItems are available, thereby causing the range error.
One way to fix this issue is by ensuring the Select value always matches one of the MenuItem’s values.
Here’s an example setup:
<Select value={this.state.value} onChange={this.handleChange}> <MenuItem value={10}>Ten</MenuItem> <MenuItem value={20}>Twenty</MenuItem> <MenuItem value={30}>Thirty</MenuItem> </Select>
In this code snippet, the `value` prop is set to a state variable, and only valid values from MenuItem (10, 20, 30) can be assigned.
Furthermore, if your data is being fetched asynchronously, ensure the value assignment occurs after the MenuItems are fully loaded.
Finally, be sure all involved value types are consistent. If they’re supposed to be strings, for instance, adhere strictly to that data type. As Ada Lovelace once said, “The Analytical Engine does not make any mistakes about its arithmetical calculations.”. This concept is true with JavaScript as well; the input must match precisely in terms of data type and value for the operation to work correctly.
For more intensive information/documentation about Material-UI Select component handling, you might want to visit their official documentation.
Solving Common Problems with Setting Value Range in Material-UI
Understanding the challenges associated with setting value ranges in Material-UI and specifically, dealing with the issue where the Material-UI select set value appears to always be out of range can indeed seem daunting. However, there are workable solutions for these problems which we will discuss in detail.
First, let’s examine the problem with a concrete example: Consider a situation where you are trying to create a Material-UI Select component with options from 1 to 5, when you try to set a value for the Select component that goes beyond this range say 6 or 0, the application renders nothing because the value is considered as outside the defined range.
The error might look something like this when rendered in the console:
Warning: Failed prop type: The value provided to Select is invalid.
This happens because the `value` property is expected to correlate with one of the `MenuItem` values. If it does not, Material-UI considers the value uncontrolled and gives you this warning.
There are several ways we can tackle this problem:
* Set Initial Values – Always ensure that the initial value that you provide falls within the specified range. For instance, if your array elements run from 0-5, any initial value given should be within this scope.
>
* Handle Undefined/null values – Another common issue is null or undefined values. If the selected value is `null` or `undefined`, ensure you handle these issues by either flagging an error message or simply providing a default value that lies within the established range.
* Data Validation – Think about implementing data validation to prevent users or developers from inputting values outside the allowed range. Validations should ideally check both the lower and upper boundaries of your value range.
To quote Linus Torvalds, “Most good programmers do programming not because they expect to get paid or get adulation by the public, but because it is fun to program” source. Hence, these issues and errors can be considered a part of the journey instead of a stop sign. It gives an opportunity to delve deeper into the intricacies of Material-UI and JavaScript, allowing better understanding of not just its capabilities but also its limitations.
The overlying principle to tackling this problem lies in making sure that the value provided is within the established range of selectable values. By judicious use of error handling and validation, you can ensure that your Select component performs as expected.
Practical Solutions to Out-of-range Errors in Material-UI Select
Material-UI Select is a popular drop-down list component in React powered by Material-UI. Having “Out of Range” errors can be frustrating when working with this tool. It typically occurs when the value assigned to the Material-UI Select component doesn’t exist among the given options, causing it to display an “Out-of-range” error.
Possible causes:
Using keys/values that do not exist within the options provided to the Select could yield the “Out of Range” error. Another cause may be related to using incorrect or mismatched data types for the options and selected values.
Practical solutions:
- Ensure Correct Matching Of Option Values And Assigned Value
- Use Default Option Placeholder When No Value Matches
- Convert Option Values To The Same Datatype
Here, ensure that `selectedValue` matches one of the option values being mapped. Also, ensure both option value and selectedValue are of the same type.
By assigning a default value (like empty string “”), you’ll have a placeholder setting preventing out-of-range errors if `selectedValue` doesn’t match any option’s value.
If your values from an API or backend server are number types, while Material-UI Select uses string values as underlying structure, it may result in mismatch. Transforming those values data type is another way of avoiding the error, by using Number() or toString() method as required.
Frankly speaking, “Out-of-range” errors in Material-UI Select generally indicate a mismatch between the stated value and available options. Solutions then center around ensuring that these two parameters align correctly.
As Donald Knuth once said, “Science is knowledge which we understand so well that we can teach it to a computer.”. So understanding our tools and components is crucial in leveraging them for effective solutions.
Remember, consistency is key when working with data types and structures in JavaScript. Always ensure your values align with what is expected by the targeted components.
For more insights check out, Material-UI documentation on Selects.
Delving into the subject of “Materialui Select Set Value Always Out Of Range”, the intricacy arises when trying to set or change the value via external factors, leaving developers feeling like they’re contending with an insurmountable range issue. The paradox appears as though the value you’re endeavoring to implement is perpetually out of bounds.
This issue can be primarily traced back to asynchronous behaviors linked with event handling in JavaScript and states within React. However, unraveling this puzzle doesn’t need troubleshooting line by line of your code; it might just require a perceptual shift.
Thorough comprehension instills the wisdom that assignment of value to the Material-UI Select component doesn’t sit solely on hard coding but leans exceptionally on syncing state variables correctly.
Consider the illustration below:
const [myValue, setMyValue] = React.useState(''); ...
In the above code, the initial state of
myValue
is set to an empty string. The
value
prop connected to Material-UI’s Select component is now tied to
myValue
. When there’s a change in select input, the function provided to
onChange
is executed. This is where we call
setMyValue
and pass the updated value, thus maintaining the desired synchronization between our state variable and the select input’s value.
“A good programmer looks for ways to create ‘layers of abstraction’ that hide the complexity of the low-level machinery” – Andrew Hunt and David Thomas, authors of The Pragmatic Programmer.
Yet, do bear in mind that with great flexibility comes greater responsibility: This solution proceeds on the caveat of assured synchronous and orderly operation of the set state function. Remember, it’s pivotal to have a sound understanding of the fundamental technologies at work here: Javascript, React, and Material-UI which altogether prop up this structure. (Reference) Keeping these key objectives in mind, getting Material-UI Select set value abiding within range doesn’t seem that elusive anymore.