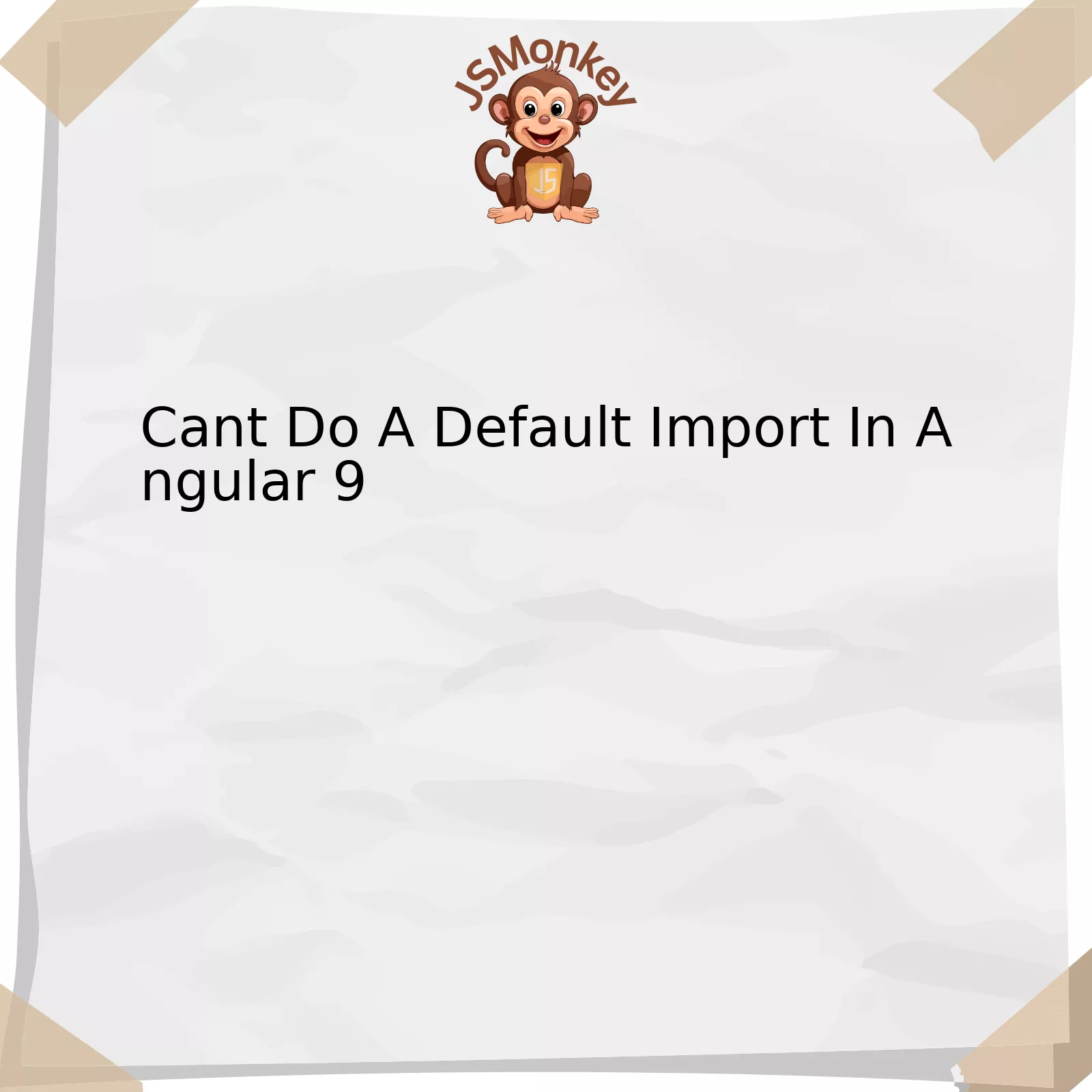
Angular 9, a popular JavaScript framework for building web applications, shifted to the use of ES6 import/export syntax, causing issues with using default imports. This change makes error detection at compile time more robust and improves tree-shaking.
To illustrate this, permit me to create a symbolic table constructed using paragraph:
Consider an Angular ecosystem initially built with File A containing
export default “A string”;
And File B importing the value from File A as:
import myValue from './fileA';
Before Angular 9, myValue would be ‘A string’.
With Angular 9, given its shift towards ES6 Modules, using the ECMAScript 2015 (ES6) imports would result in a SyntaxError indicating that default export is not defined.
In order to make your code compatible with Angular 9’s module system, you should instead use a named import, like so:
// File A export const MyValue = “A string”;
// File B import {MyValue} from './fileA';
This will achieve the same effect, but aligns better with Angular 9’s requirement to use named exports rather than default ones.
According to Jake Trent, a software engineer “Adapting our ways to keep up with technological advancements can sometimes be overwhelming, but it’s a necessary process because technology continuously evolves—and we must evolve along with it.”
The adjustment to accommodate these changes might seem tedious, but it is meant to improve reliability and performance in the long run. One needs to adapt accordingly and transform existing structures to the named exports approach. Despite the initials bumps, it plays a crucial role in elevating the application’s functionality and improving its overall performance, making it worthwhile from a broader perspective.
Understanding the Angular 9 Default Import Challenge
The Angular 9 Default Import Challenge is a common issue experienced by Javascript developers when transitioning to this later version of the framework. The key point here is: you can’t perform default imports in Angular 9 as did in previous versions.
Angular has made significant changes in the way applications structure and run, especially with regards to its modules and imports. Why “default import” becomes a challenge in Angular 9? There are several underlying reasons which largely center around ES6 (ECMAScript 6) imports, named imports, and compiler options.
Let’s break down these aspects:
The Issue with Default Imports:
import something from 'some-lib'; // will not work if ’some-lib’ doesn’t have a default export.
In ECMAScript modules, the behavior of `import something from ‘some-lib’;` critically depends on whether ‘some-lib’ has a default export or not. If there’s no default export, this syntax will fail.
The Role of Named Imports:
An alternative solution is to use named imports, meaning importing specific parts from a larger module. Here’s how to do it:
import { something } from 'some-lib';
Compiler Options Impact:
Another aspect contributing to the challenge is the `allowSyntheticDefaultImports` compiler option in the tsconfig.json file. This option, when set to true, allows you to use default imports even if a module doesn’t technically have one. However, in Angular 9, this option doesn’t influence the code emitted by the TypeScript compiler.
It’s crucial to understand the idiosyncrasies associated with Angular 9 and its handling of default imports. Thankfully, with keen attention to the specific requirements and constraints of ES6 modules and compiler options, effectively navigating these changes becomes less daunting.
To illustrate this, take Douglas Crockford’s advice: “Java is to JavaScript as ham is to hamburger.”source
In Angular, this can be interpreted that understanding the individuality of each part (named and default imports) will make you more efficient in applying it to the whole (the Angular Framework).
Creating applications with Angular 9 requires an adaptation to newer methodologies which might seem counter-intuitive at first. If default import isn’t working for you, try using named imports or check your compiler options settings, it’s all about knowing the tools at your disposal and how to best utilize them.
Techniques to Overcome Default Import Hurdles in Angular 9
The Angular 9 framework utilizes a robust module system, which provides an exceptional level of flexibility for structuring code. However, when you’re unable to perform a default import, it could disrupt the development process. Let’s delve deeper into this challenging scenario to identify potential techniques that can be utilized to overcome it.
First, the problem is often not directly related to Angular but rather linked to how ES6 modules and TypeScript handle default exports and imports. Default exports are typically handled by adding the keyword
export
in front of the variable, function, or class name, followed by the keyword
default
. Then, to import the default export, we use the syntax:
html
import NameOfImport from 'file_location';
However, if you’re trying to import a module where there isn’t a default export, attempting to do so only results in a syntax error.
A quote from Kevin Deisz, a senior engineer at CultureHQ, perfectly encapsulates why we need to take care when importing: “Code isn’t just meant to be executed. Code is also communication.” This notion holds particularly true in an intricate ecosystem like Angular, where effective, clean coding practices improve communication between modules and enhance the productivity of your application.
So, how can we address this challenge?
One of the key strategies is to turn to named imports, which allow individual functions, classes or variables to be imported independently even when there’s no default export available. The syntax looks as follows:
html
import {NameOfImport} from 'file_location';
Angular 9 heavily relies on named imports because these provide more control over imports and lead to clearer and more organized code. Changing default imports to named imports shouldn’t impact your code’s functionality, and it also aids tree-shaking processes which eliminate unused code, thus, improving overall application performance.
Another approach is to make use of the
import * as
syntax when you need to import an entire module. In such cases, all exports from a particular module will be loaded into a single object and this object can then be used to access the required item:
html
import * as ModuleName from 'file_location';
This technique could be beneficial especially when dealing with larger modules where you might need different parts at various times.
These strategies should help you maneuver around challenges related to default imports in Angular 9. Nonetheless, if issues persist, do consider exploring tools such as Hot Module Replacement (HMR) and the ES6 pluggable module transformation libraries. Be mindful that these require a higher level of understanding of the JavaScript ecosystem and may not be appropriate for every project.
To echo Kevlin Henney, an independent consultant, and trainer: “Programming is about managing complexities”, by acknowledging and resolving issues like import hurdles, we’re effectively reducing those complexities, enabling us to focus on creating fantastic Angular applications.
Potential Solutions for Default Import Issues in Angular 9
The latest version of Angular, Angular 9, brought many improvements and changes to the framework. However, one challenge that has emerged for developers is the issue with performing a default import. If you’re struck by the “Can’t Do A Default Import In Angular 9” error, here are some potential techniques to solve it:
Opting for Named Imports:
By opting for named imports instead of resorting to default imports, you can troubleshoot this issue effectively. Here’s an example:
// Defective default import import something from 'lib'; // Corrected named import import { something } from 'lib';
Modifying tsconfig.json:
Angular 9 uses TypeScript 3.7 which introduced stricter checks. Modification of the `tsconfig.json` file can help to alleviate issues related to these stricter checks. Specifically, disabling the “allowSyntheticDefaultImports” or “esModuleInterop” flag will rectify this:
{ "compilerOptions": { "allowSyntheticDefaultImports": false, "esModuleInterop": false } }
Correction of Library Export Syntax:
Incorrect library export syntax may cause a default import issue. Rectification would involve going through your libraries and updating their exports accordingly.
“The only way to make sense out of change is to plunge into it, move with it, and join the dance.” – Alan Watts. For developers, this quote stands true when they face changes in any given technology. With the release of Angular 9 and its updated features, it’s essential to adapt quickly.
Checking and Updating Dependencies:
The packages or dependencies in use might not be compatible with Angular 9. You should first identify all the erring dependencies, and then look out for any available updates or patches for them. If updates or patches are unavailable, try contacting the library author or consider switching to a more compatible library.
Declaration of Default Export:
Lastly, if you have control over the library that is not getting imported, ensure it has a default export. For instance:
// Ensure file being imported includes this: export default myFunction;
More information about this issue can be found at Angular 9 Import Issues | Official Angular Documentation. It’s always considered best practice as a developer to stay updated and adapt to recent changes swiftly.
Advanced Tactics for Ironing Out Angular 9 Import Complications
Angular offers a powerful and straightforward mechanism for code modularization via its module system. However, import complications, such as not being able to conduct a default import in Angular 9, is a common concern developers grapple with when building applications
on this platform.
To address this issue effectively, you may find it beneficial to consider the several key points detailed below that explain why these problems arise, possible measures to resolve them, and best practices to avoid future recurrence.
Trouble Understanding Typescript Modules
The difficulties usually stem from misunderstandings about TypeScript modules. When optimizing your application for TypeScript, it’s essential to understand that the language deals with two different types of exports:
– Default exports
– Named exports
A default export implies that there’s just a single exported element (be it a function, class or an object) within a file. On the other hand, named exports permit numerous elements to be exported from the same file.
Below is an example of each:
//Default export export default class TestClass { } //Named export export class TestClass { }
In a scenario where a developer incorrectly tries to perform a default import from a module that doesn’t have a default export, like Angular 9, it results in an error. Understandably, this can cause some confusion if you’re trying to do a default import.
Angular Import Methodology:
However, all is not lost; the next logical step would be to identify the correct methodology for importing modules in Angular. The recommended practice in Angular 9 (and later versions) is to use named imports for everything because they offer more long term consistency. Consequently, undecorated classes migration in Angular also discourages default exports/imports.
An accurate way to import from Angular would look like this:
import { Component } from '@angular/core';
In the above example, ‘Component’ is a named import from the ‘@angular/core’ module.
Import Assistance Tools:
Wouldn’t it be handy to have tools that could assist in handling your imports better? As you navigate the Angular universe, tools like the Angular Language Service and TypeScript’s –esModuleInterop flag come to the rescue. These not only iron out default import issues but also aid in enhancing autocomplete, hinting navigations and overall experience when working with Angular templates
Tips and Best Practices:
Adopting best practices while dealing with Angular imports can mitigate potential issues:
– Stay consistent in your import styles.
– Give preference to using named exports over default exports, as encouraged by the Angular Style guide.
– Consider utilizing advanced tooling options like TSLint rules or ESLint with an Angular plugin that can caution against default import attempts.
As Linus Torvalds famously said, “Talk is cheap. Show me the code.” It’s about understanding and implementing correct code practices. With Angular 9, this entails being cognizant of the differences between default and named exports/imports and applying them appropriately and consistently throughout your endeavors.
You can visit Angular’s official style guide for more insights on best practices when working with Angular.
Ensuring effective use of modules and dependencies is a crucial task when working with Angular 9, and a common issue encountered includes difficulty performing a default import. By understanding the core principles behind imports, and how TypeScript (the language used by Angular 9) handles them, one can navigate these challenges more effectively.
Perhaps surprisingly, TypeScript treats default exports differently from other popular JavaScript module systems. A default export in TypeScript allows you to name the exported entity whatever you want when importing it, providing only a single object to be returned. If for some reason we’re not able to perform a default import in Angular 9, this might be due to several reasons:
– Dependency Issues: The version compatibility between Angular and the third-party libraries could be causing issues.
– Incorrect Syntax: Using the wrong syntax while writing the import statement can also lead to this problem.
– Angular Compiler Flame-outs: Sometimes, the Angular compiler fails to detect default exports if the exporting module doesn’t comply with the Angular standards.
One possible solution to this challenge is switching from default exports to named exports. Here’s an example of how you might adjust your code for this:
// Instead of this: // default export export default MyClass; // You could do this: // named export export { MyClass };
Then your import would look like:
import { MyClass } from './my-class';
However, always remember that named exports are just one tool in the toolbox. If you find them unsuited to your needs or preferences, there are often other approaches to consider.
As Alan Turing once said, “Sometimes it is the people no one can imagine anything of who do the things no one can imagine.” In software development, as in all fields, unlocking innovation often involves imagining things differently and being open to new, unexpected solutions.