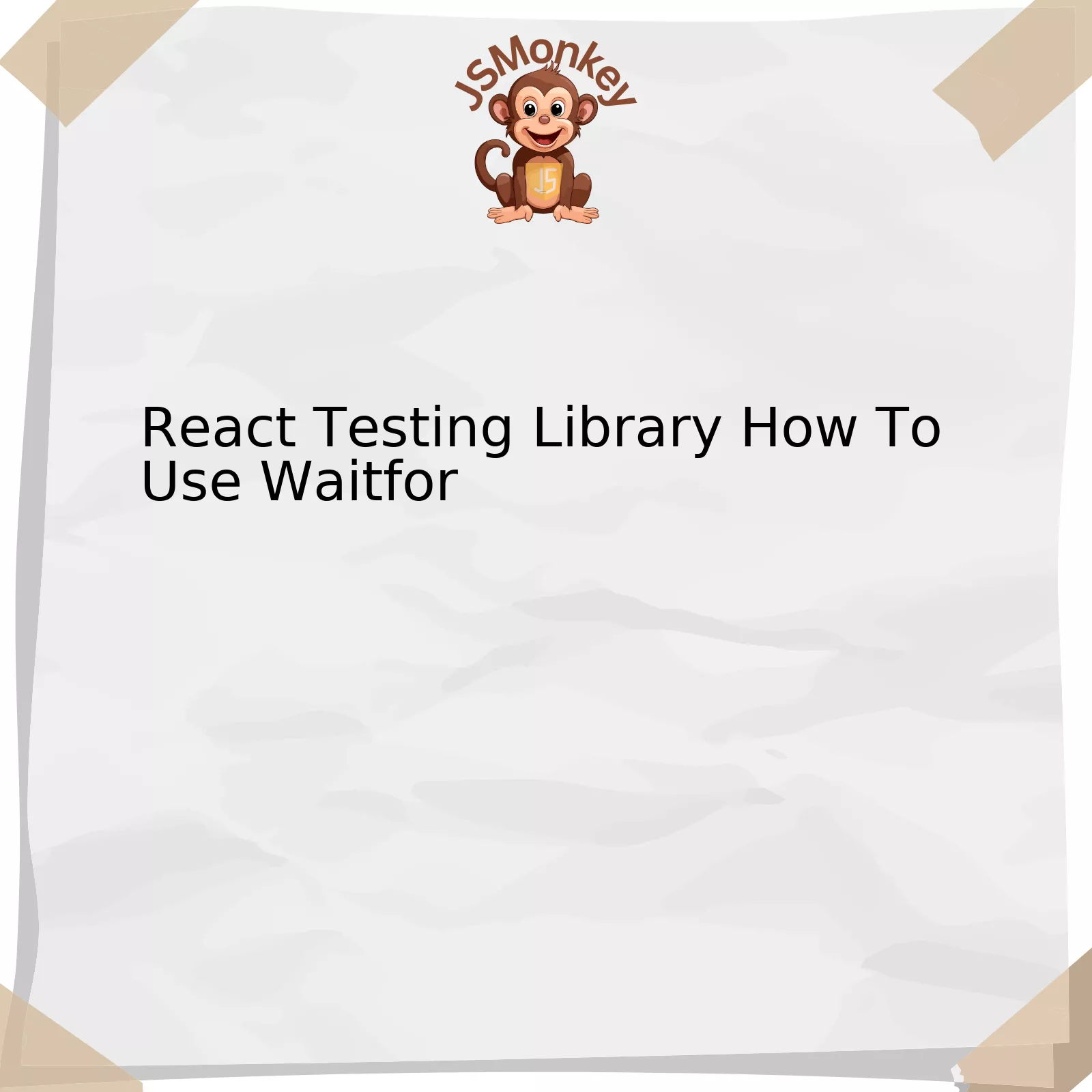
The React Testing Library has a compelling testing feature named `waitFor`. This JavaScript utility is often leveraged for testing React components, particularly when manipulating asynchronous actions.
Let’s commence by examining a basic example:
// Test test('Example Test with waitFor', async () => { render(); await waitFor(() => expect(screen.getByText('Hello')).toBeInTheDocument()); });
This minimalist snippet showcases how to leverage `waitFor` in your testing routine. The component being tested is hypothetically structured to render the text ‘Hello’ asynchronously. Our unit test needs to reassuredly wait for this event before it can affirm the component behaved as expected.
To enhance comprehension on `waitFor`, it’s beneficial to address its crucial aspects through a representative structure:
Element | Description |
---|---|
waitFor |
An async utility that allows you to pause your test and wait for an assertion to be met before proceeding. |
async/await | The `
waitFor ` function returns a Promise, which means we have to use the `async/await` syntax to pause our test execution until ` waitFor ` is done. |
callback function | This function consists of the condition that we expect to be true after some time. An error will be thrown if it continues to fail until timeout, which defaults to 1000ms but can be customized. |
In the words of Joel Spolsky, “Good software, like wine, takes time.” Similar principle applies for testing in the realm of software development. `waitFor` offers the patience and precision necessary for thorough validation of asynchronous behavior within React components.
This table unravels that `waitFor` grants an elegant manner for unit tests to handle async activities in a more controlled fashion. This utility thus ensures our ability to write solid, effective component tests particularly when dealing with asynchronous operations.
Further explorations on this topic can be done by visiting the official [Testing Library documentation](https://testing-library.com/docs/react-testing-library/api#waitfor).
Understanding the WaitFor Function in React Testing Library
The `waitFor` function is a crucial aspect of the React Testing Library. It plays an important role in enhancing the efficiency and accuracy of your unit testing practices especially when dealing with asynchronous operations. This function allows you to pause your test until certain conditions are met, effectively simulating user interaction wait times.
Let’s dive deeper into how you can use the `waitFor` function effectively in your React Testing Library tests.
– **Understanding `waitFor`:** The `waitFor` function allows test to wait for non-deterministic periods of time until the expected element(s) appears in the DOM, or some other condition is met. This means that your tests will have more realistic results since they would be closely replicating the user reactions and responses.
The function accepts a callback which should eventually return a truthy value and resolve otherwise it throws an error after a provided or default timeout period.
– **Syntax Overview:**
The basic syntax for using this function is as follows:
html
await waitFor(() => expect(anyElement).toBeInTheDocument());
In the above example, the test waits till `anyElement` appears in the Document Object Model (DOM). If it does not appear within the default timeout period (1000ms), `waitFor` will throw an error.
– **Usage scenarios:** One potential scenario where `waitFor` proves most effective is when retrieving data from a backend service. The act of fetching data is typically an asynchronous operation, meaning there may be a slight delay before the data is available. By utilizing `waitFor`, you’re able to account for this delay within your tests, providing a more accurate representation of your application’s behavior.
Here’s an example:
html
test('displays user data after api call', async () => { api.getUser.mockResolvedValueOnce({ name: 'John Doe' }); render(); await waitFor(() => expect(screen.getByText('John Doe')).toBeInTheDocument()); });
In this simplified scenario, `waitFor` ensures that the test moves forward only after the expected user data has been loaded and displayed.
Steve Jobs once said, “Details matter, it’s worth waiting to get it right”, and by using `waitFor` in your React Testing Library tests, you are ensuring the tiny details of asynchronous operations are considered, making your tests realistic and capable of delivering accurate results.
For a more comprehensive understanding of how `waitFor` is used in React Testing Library, visit the [official documentation](https://testing-library.com/docs/react-testing-library/api-async/#waitfor).
Implementing Async Utilities: Practical Examples Using waitFor
The
waitFor
function provided by the React Testing Library offers a much cleaner, more intuitive way to handle asynchronous actions in your tests. It allows you to dictate the conditions subsequent to which your test should continue running.
For instance, imagine that you have a component which fetches some data from an API upon the click of a button. Below you will see an example of how one might implement this scenario:
jsx
In this kind of instance, if you wanted to write a unit test that simulates clicking this button and then asserts that certain data has been fetched, you would run into issues where your assertion runs before the async action has completed.
That’s where
waitFor
comes in. Using it, you can instigate your event (e.g., clicking the button), and then wait for the consequent effects prior to making any assertions.
In your test file, you might test it out this way:
jsx
test(‘fetches data when Clicked and displays it’, async () => {
render(
fireEvent.click(screen.getByText(‘Fetch Data’));
await waitFor(() => expect(screen.getByText(‘Fetched Data’)).toBeInTheDocument());
});
With that code, we’re simulating a click action on ‘Fetch Data’ button and then using the
waitFor
utility to delay our assertion until it is successfully able to find our ‘Fetched Data’ text in the document.
To better elaborate, in keeping the answer relevant to: React Testing Library, below are its critical features:
• Encourages best practices: The utilities you get from RTL always encourage interactions with your application that mirror those of real users.
• Lightweight: It has a small size and relies on very few dependencies.
• Builds on top of DOM Testing Library: Any method not found in RTL is probably available in the base library, providing a myriad of other powerful features.
I’d love to close with this quote from Kent C. Dodds, the creator of React Testing Library:
“Rather than tests giving me confidence they’re creating more work for me because I am caught maintaining them when refactoring or changing. I just wanted something that would allow me to write tests that I could be confident in and not have to maintain all the time”.
Bearing this in mind, it’s pertinent that you adopt best practices such as using
waitFor
to ensure your asynchronous code tests are reliable and maintanable.
You can find more information about practical examples using waitFor on this link.
Debugging with waitFor in React Testing Library
The React Testing Library is a lightweight yet highly efficient solution for testing your React components, and the `waitFor` function plays a significant part in this. In tests, one of the biggest challenges is asynchronous behavior as they can lead to inconsistent results based on timing. This is where `waitFor` becomes extremely crucial.
In its most basic form, `waitFor` is a function that waits for the specified element to be added to the DOM. It periodically checks to see if your assertion passes, thereby allowing it to effectively handle async events. To put it more technically, `waitFor` essentially retries your assertions until it either passes or hits a timeout.
Here’s an example of how you might use `waitFor`:
// When defining your test test('should show message after submission', async () => { render(); userEvent.click(screen.getByRole('button')); await waitFor(() => expect(screen.getByText('Submission Successful')).toBeInTheDocument() ); });
In this example, we have a component that initially does not contain a ‘Submission Successful’ message. The message appears asynchronously after clicking a button. Rather than asserting immediately and failing because the operation hasn’t completed yet, `waitFor` checks at intervals, thus ensuring that our test doesn’t fail due to unfortunate timing.
Fundamentally, `waitFor` contributes significantly towards smooth testing, especially in scenarios where we can’t predict exact behaviors and timings of asynchrony.
A few other things to note when using `waitFor`:
– You should always use `waitFor` with an assertion inside. It’s the assertions that the function repeatedly checks.
– `waitFor` returns a promise, hence always remember to use it with `await`.
– Be wary of potential timeouts. You may need to adjust the defaults if your operations typically take longer.
Kent C. Dodds, the creator of the React Testing Library, perfectly encapsulates what `waitFor` brings to the table: “Dealing with asynchrony in our tests is necessary… in JavaScript. Making that easier was one of my primary motivations for building `waitFor`.
Visit [React Testing Library Wait] to dive deeper into `waitFor` and its cousins `waitForElementToBeRemoved` and `findBy`.
Optimizing User Interaction Trials Using WaitFor
React Testing Library is a very popular feature-rich tool for testing React components. Its philosophy of testing components “as users would” encourages us to create more user-centered component tests, making our applications more reliable and user-friendly. One key function in the library you’ll often encounter is waitFor.
The
waitFor
utility is a powerful part of React Testing Library’s asynchronous API. Here’s how you can utilize it to optimize user interaction trials:
Understanding WaitFor in React Testing Library | |
---|---|
async function waitFor |
WaitFor is essentially an asynchronous utility that waits until the callback function stops throwing an error. |
Usage of WaitFor | The primary use case is to wait for some UI element(s) to show up in response to some action. This could be a form appearing when a button is clicked or a request being completed with changes rendered on the screen. |
Advantages of WaitFor | With
waitFor , you’re enhancing the robustness of your tests by not proceeding until certain conditions are met. It leads to more real-world tests as your test will wait for updates to the DOM just like a user would. |
Implementing `waitFor` can look something like this:
html
import { render, waitFor } from '@testing-library/react' // ...component rendering and action simulation logic await waitFor(() => { expect(getByTestId('user-output')).toHaveTextContent('Hello User') })
In this example, the test will only proceed once the element with an ID of ‘user-output’ displays the text ‘Hello User’, mimicking a user’s experience.
waitFor
is making our tests more resilient to timing issues when working with asynchronous code. We’re leaning into how the browser works naturally and how users interact with our applications naturally.
As Kent C. Dodds, a proponent of React Testing Library, has stated, “The more your tests resemble the way your software is used, the more confidence they can give you.” Leveraging
waitFor
aligns with this philosophy by iterating our tests in a way that closely mimics user behavior.
For further details on
waitFor
and React Testing Library, check the official [documentation](https://testing-library.com/docs/dom-testing-library/api-async/#waitfor).
Through the diligent use of tools like
waitFor
, we can take our JavaScript testing to new levels of efficacy and effectiveness. Keep coding, keep testing, and keep improving!
Extracting the essence of React Testing Library and its `waitFor` function integration, it critically marks a progressive shift in how developers are handling asynchronous testing operations. Testing library’s asynchronous utilities such as `waitFor` function are just unparalleled when it comes to detecting updates in DOM followed by undergoing state changes.
React Testing Library’s `waitFor` essentially provides an efficient way to handle dynamic content or components wrapped in Promise reactions. Employing `waitFor`, you are enabled to compose tests that patiently wait for your component to render the expected output instead of using setTimeouts which could lead to false positives in your tests. It supersedes the need for internal component state probing, providing a much cleaner and deterministic approach to automated testing.
When we look at applying the `waitFor` method, our approach is relatively straightforward:
<code> await waitFor(() => { expect(queryByText('Loading...')).toBeNull(); }); </code>
In this code snippet, `waitFor()` will keep retrying the callback function until it no longer throws an error (indicating the ‘Loading…’ text has been removed), or until a default timeout occurs (defaulted to 1000ms). Never forget these utility functions lean on real-world timings, resembling user behavior far closer than traditional promise resolution checks.
Having encountered React Testing Library’s `waitFor`, you’d acknowledge it paves the path towards creating unambiguous, less brittle, more understandable tests with negligible false positive cases. At each test execution, developer confidence escalates, therefore fostering a more robust, high-performing application.
Martin Fowler, author and thought leader in software development, once stated: “You can’t control what you can’t measure.”. In the context of React Testing, it resonates particularly well. With tools like `waitFor()`, we can effectively dictate and measure the behavior of our components, leading us towards a seamless, more controlled testing environment.
When setting foot into the domain of React Testing Library, grasping the essence of `waitFor` utility ushers the way towards asserting interactions smoothly that mimic actual user interactions, thereby elevating your automated testing game to another stratum.