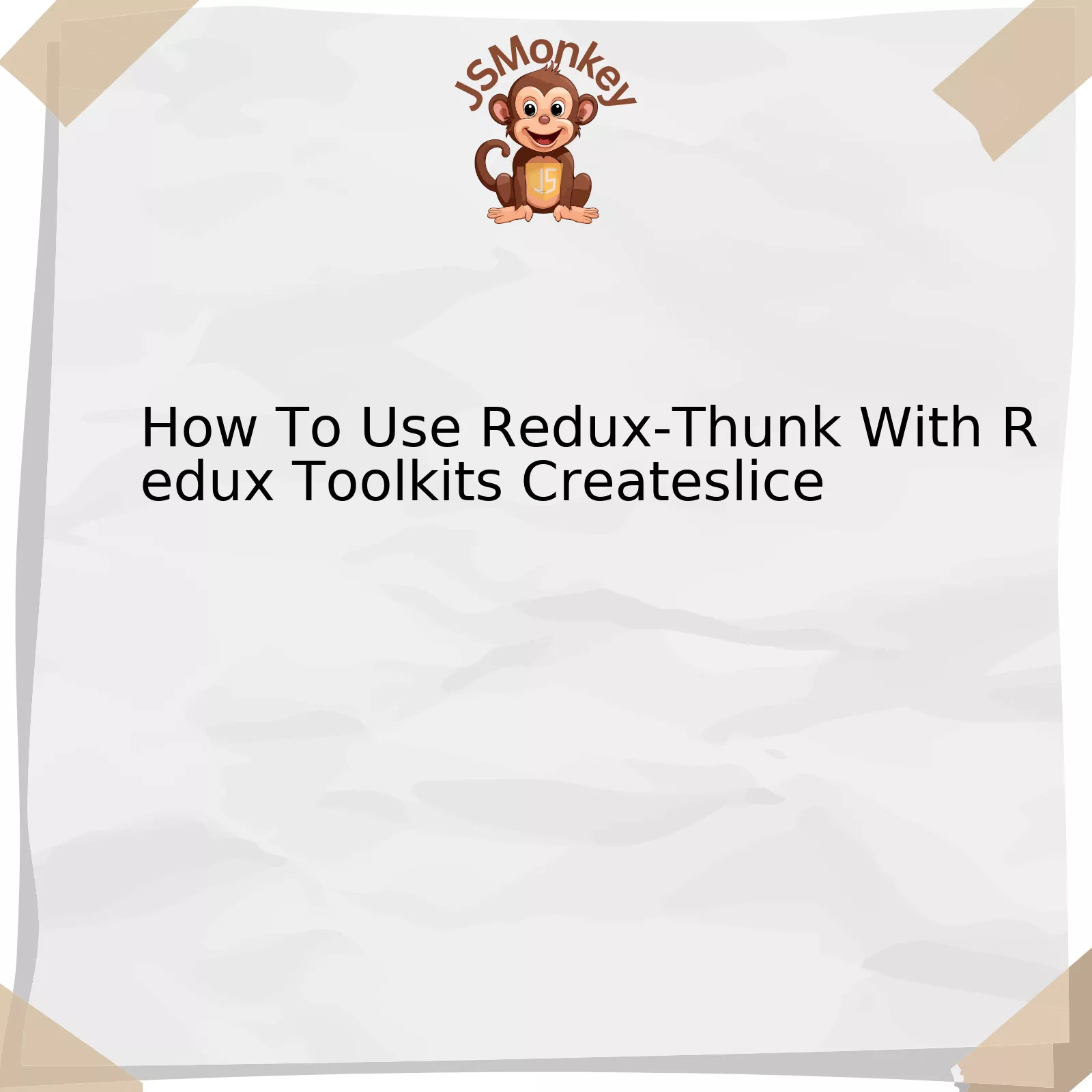
Using Redux Toolkit’s createSlice with Redux-Thunk provides a clean, organized solution to manage state in a JavaScript application. Why? Because createSlice helps auto-generate action creators and action types, reducing boilerplate, while Redux-Thunk permits handling asynchronous logic inside action creators.
To understand the process, let us start from the basis:
Step | Action | Description |
---|---|---|
Set Up Store |
configureStore() |
This is where all reducers are brought together. By default, this function sets up the Redux DevTools Extension and Redux Thunk. |
Create Slice |
createSlice() |
It generates slices of the Redux store, creating a slice reducer with corresponding action creators and action types. |
Create Async Action | Redux Thunk | You would implement asynchronous functions that return an action to be dispatched when the promise is resolved, typically related to API calls. |
Dispatch Actions |
dispatch() |
Within components, use this function to send actions which are either handled synchronously by reducers or as async functions in Redux Thunk. |
Now, let’s deep dive into what these steps entail.
Set Up Store: Using configureStore() from Redux Toolkit, we can set up our application store. This method bakes in the common practices of setting up middleware with a handful of customizable options. Importantly, it encompasses Redux Thunk and the Redux DevTools Extension.
Create Slice: Redux Toolkit’s createSlice() API is a function that receives an object describing reducer logic and state, and auto-generates action creators and action types. This results in less boilerplate and leaner code. It takes the name of the slice, initial state, and an object with reducer functions, then churns out a slice object with corresponding actions and the reducer.
Create Async Action: Redux Thunk shines while dealing with complex or asynchronous operations. It allows us to return a function instead of an action object, providing control over dispatch. For instance, you can make API calls in your action creator, wait for it to conclude, and subsequently dispatch actions according to result (fulfill, reject).
const fetchUserById = userId => async dispatch => { const response = await userAPI.fetchById(userId); dispatch(userReceived(response.data)); };
Dispatch Actions: Lastly, you dispatch these actions from your components. Remember, both sync actions from createSlice and thunk functions are dispatched in the same manner. Redux Thunk steps in when it detects an action creator returning a function and provides dispatch as an argument.
To quote Dan Abramov, creator of Redux: “Dispatching async actions is no different from dispatching sync actions.”
This encapsulates the process of using Redux-Thunk paired with Redux Toolkit’s createSlice(). The synergy of the two simplifies state management within your application.
Understanding Redux-Thunk and Redux Toolkit’s Createslice
Redux-Thunk and Redux Toolkit’s createSlice are two powerful aspects within the Redux ecosystem of front-end development with JavaScript. The aim here is not just to understand these concepts individually, but also how to effectively use Redux-Thunk within Redux Toolkit’s createSlice.
I. Redux-Thunk
“Programming is a skill best acquired by practice and example…” – Brian Kernighan, developer
Redux-Thunk fills a critical niche in Redux: handling asynchronous code and side-effects. As middleware that allows dispatching functions rather than plain objects, Redux-Thunk enables the writing of logic-heavy actions without cluttering the reducer or component.
Consider this snippet:
const myAction = () => async (dispatch, getState) => { const response = await someAPI.getData(); dispatch({ type: 'MY_ACTION', payload: response }); };
This Thunk action creator postpones the dispatch until an API call returns the data. It helps ensure the application’s state is modified only once we receive new information.
II. Redux Toolkit’s createSlice
Redux Toolkit’s createSlice addresses the infamous boilerplate redundancy in Redux code. By auto-generating action creators based on the reducer methods you define, it keeps the process DRY and code readably brief.
A sample syntax using createSlice looks like:
import { createSlice } from '@reduxjs/toolkit' const counterSlice = createSlice({ name: 'counter', initialState: 0, reducers: { increment: state => state + 1, decrement: state => state - 1 } })
Here, ‘increment’ and ‘decrement’ are action creators seamlessly converted into strings like ‘counter/increment’ and ‘counter/decrement’.
III. Dispatching Thunks from Slices
It is vital to note that ‘createSlice’ only automates actions linked with state updates. Asynchronous operations or side-effects are still handled separately, but can be organized in the same slice file.
Returning to the concept of Redux-Thunk, we can leverage asynchronous code within our slices:
import { createSlice, createAsyncThunk } from "@reduxjs/toolkit"; import { someApi } from "./api"; export const fetchUserData = createAsyncThunk( "user/fetchData", async () => { const response = await someApi.fetchData(); return response.data; } ); const userSlice = createSlice({ name: "user", initialState: { data: [] }, extraReducers: (builder) => { builder.addCase(fetchUserData.fulfilled, (state, action) => { state.data = action.payload; }); }, }); export const { actions, reducer } = userSlice;
This integrates Thunk-returned promises into a slice by using ‘extraReducers’. Here, the ‘fetchUserData’ action creator does not directly change the state. The ‘user/fetchedData’ action dispatched upon fulfilled promise does modify the state.
In essence, successfully integrating Redux-Thunk with Redux Toolkit’s createSlice combines the ability to handle logic-intense, asynchronous actions and creating concise and effective reducers without redundant code. Redux Toolkit Documentation provides comprehensive guidance for additional learning. Harnessing these two tools amplifies JavaScript developers’ power in crafting sophisticated and maintainable front-end applications.
Implementation of Async actions using Redux-Thunk with CreateSlice
Implementing async actions using Redux-Thunk with CreateSlice involves several steps. First, it’s crucial to have a grasp of Redux Thunk itself – an essential middleware that allows you to dispatch actions that return a function rather than an object. This functionality proves particularly useful when dealing with asynchronous operations like API calls or timeouts, as it enables the action to pause, await the outcome, then resume and dispatch new actions in response to the received data.
Moving on to the implementation process, we begin by creating a slice using the `createSlice` function provided by Redux Toolkit. A slice resembles the traditional root reducer object – but encapsulated inside an object wrapper.
import { createSlice, createAsyncThunk } from '@reduxjs/toolkit' export const fetchPosts = createAsyncThunk('posts/fetchPosts', async () => { const response = await fetch('https://jsonplaceholder.typicode.com/posts') return (await response.json()) }) const postsSlice = createSlice({ name: 'posts', initialState: [], reducers: {}, extraReducers: builder => { builder.addCase(fetchPosts.fulfilled, (state, action) => { return action.payload }) } }); export default postsSlice.reducer
In the example above:
– `createAsyncThunk` is used to handle the API call, which will dispatch actions before and after the API request.
– Inside the `extraReducers` field, code listens to the dispatched actions and adjusts the state accordingly.
Noteworthy is Redux Thunk’s instrumental role in managing state that’s dependent on asynchronous activities. It reschedules actions to occur when results are ready, circumventing latency issues from delayed resolve times of promises.
As Ian Sommerville said, “Software systems are increasingly ubiquitous in all aspects of our lives. If they don’t work correctly, they can result in serious harm or fatalities.” This quote is a reminder of how vital it is to use competent tools like Redux Thunk when writing JS code.
Furthermore, `@reduxjs/toolkit` embraces an opinionated pattern that simplifies the Redux setup. Previously tedious steps have been streamlined – with less boilerplate and more optimization out-of-box. The toolkit fosters efficiency and boosts performance through automated process improvements and best practices recommendations.
To learn more about implementing async actions using Redux Thunk within Redux Toolkit’s CreateSlice, visit one of these [exhaustive posts](https://www.reddit.com/r/reactjs/comments/ciag1p/whats_the_best_way_to_handle_api_calls/) on the subject hosted on r/reactjs or [the official Redux Toolkit documentation](https://redux-toolkit.js.org/usage/usage-guide#async-requests).
Error Handling in Redux Thunk implemented within CreateSlice
The implementation of error handling with Redux-Thunk within CreateSlice from Redux Toolkit presents a compelling topic.
Redux-Thunk is middleware that allows you to write action creators returning functions rather than the formulaic plain object. It proves vital when managing asynchronous actions where a promise needs resolution before dispatching an outcome.
const myAction = () => async (dispatch) => { // ...asynchronous code... dispatch(...) }
For instance, applied in a CreateSlice context, it might look like this:
const mySlice = createSlice({ name: 'myFeature', initialState, reducers: { startAction(state) { ... }, finishAction(state, action) { ... } } }) export const myAsyncThunk = () => (dispatch) => { dispatch(mySlice.actions.startAction()); doSomethingAsync().then( result => dispatch(mySlize.actions.finishAction(result)), error => console.log('An error occurred!', error) ); };
In this example, we’ve produced an asynchronous action `myAsyncThunk` using Redux-Thunk that makes use of actions defined through Redux Toolkit’s `createSlice`.
Discussing error management, chances are high that during the system execution, network errors or internal application discrepancies may constitute hurdles to how the application processes actions. Effective error handling methods become then essential.
Instead of logging errors simply in the console as shown above, incorporate proper error handling via additional action types such as `actionFailure`. Add this into your slice, set some state like `hasError` or `errorMessage`, and dispatch this action in where there’s an error. The adjusted example could be like:
const mySlice = createSlice({ name: 'myFeature', initialState, reducers: { startAction(state) { state.loading = true; }, finishAction(state, action) { state.loading = false; state.data = action.payload; }, actionFailure(state, action) { state.loading = false; state.error = action.payload; } } }) export const myAsyncThunk = () => (dispatch) => { dispatch(mySlice.actions.startAction()); doSomethingAsync().then( result => dispatch(mySlice.actions.finishAction(result)), error => dispatch(mySlice.actions.actionFailure(error)) ); };
The data processing flow in your UI will now be able to cope with these errors by inspecting the `error` field of the respective slice and display meaningful user feedback.
In hindsight to Jeff Atwood’s quote stating that “Coding is not ‘fun’, it’s technically and ethically complex”, detecting and handling errors when using Redux-Thunk within CreateSlice builds on the continuous strive for code stability and user experience improvement. To further deepen your knowledge in this area refer to the official [Redux Toolkit documentation](https://redux-toolkit.js.org/).
Best practices for Maintaining State with CreateSlice & Redux Thunk
Maintaining state with CreateSlice and Redux Thunk involves strategic incorporation of both these tools to implement asynchronous actions and reduce boilerplate within your Redux-based application effectively. While CreateSlice, part of the Redux Toolkit eliminates the need to write action types and actions manually, Redux Thunk provides middleware that allows you to write action creators that return a function instead of an action. As such they become an essential part of any modern JavaScript application state management.
When using Redux-Thunk with Redux Toolkit’s createSlice, consider these best practices:
• Initialize State Appropriately:
Define the initial state in the slice. This could be an object, an array or any value that best describes the initial state of the slice data. For instance,
const userSlice = createSlice({ name: 'user', initialState: { name: '', age: null }, reducers: {...} });
• Define Async Actions Inside Slice:
Redux Thunk works best when async actions are located inside the created slice. This helps keep the related logic encapsulated into one area of the codebase, contributing to clean and maintainable code.
• Avoid Mutating State Directly:
It might be tempting to mutate the state directly, but this can lead to errors and inconsistencies. Use the immer-powered produce function automatically provided by the createSlice reducer to make as if you’re modifying the state directly.
• Making Use of Loading States:
Manage loading states for async requests to provide users with feedback while actions are being processed. You could use flags such as `isLoading`, `isSuccess`, and `isError` set to true or false depending on the state of the request.
• Error Handling:
Always handle possible errors from API calls to prevent your application from breaking and enhance the user experience. You could create error actions in the event that an API call fails.
Consider this example,
export const fetchUserById = createAsyncThunk( 'users/fetchByIdStatus', async (userId, thunkAPI) => { const response = await userAPI.fetchById(userId); if (response.error) { let error = response.error.message || 'API Error!'; return thunkAPI.rejectWithValue({ error }); } return response.data; } );
The renowned software engineer Linus Torvalds once said: “Good programmers know what to write. Great ones know what to rewrite (and reuse).” So remember to make use of the benefits offered by Redux Toolkit and Redux Thunk to write better, more maintainable JavaScript code.
• Use of Selectors:
Selectors can be utilized to access state slices directly from components without inundating the component with data it doesn’t need. By doing so, you’ll prevent unnecessary renders and improve the performance of your application.
For extensive documentation on how to harness these best practices, refer to the comprehensive guide on the official Redux Toolkit Docs here: [Redux Toolkit Docs](https://redux-toolkit.js.org/).
After delving deep into the complexities and intricacies of leveraging Redux-Thunk with Redux Toolkit’s CreateSlice, a couple of pivotal aspects emerge.
Firstly, Redux-Toolkit’s createSlice is instrumental in generating reducer functions and actions, thus simplifying your coding process and enhancing readability. We’ve understood that you can initialize it with a name, an initial state, and an object full of reducer functions.
const todoSlice = createSlice({ name: 'todos', initialState: [], reducers: { addTodo: (state, action) => { state.push(action.payload); }, }, });
Importantly, you’ll recall that Redux-Thunk adds another dimension to Redux – enabling us to dispatch functions, also known as thunks. Thunks encapsulate complex synchronous logic, housing a plethora of actions that don’t necessarily return immediately.
So, how do these two powerful features interact within your redux store?
The flexibility of Redux Toolkit allows it to be customized with middleware during its configuration. Including Redux-Thunk as a middleware through configureStore, enables thunk actions to be dispatched from within your application.
import { configureStore } from '@reduxjs/toolkit'; import todoReducer from './todoSlice'; export default configureStore({ reducer: { todos: todoReducer, }, middleware: getDefaultMiddleware => getDefaultMiddleware().concat(thunk), });
These dispatched thunks can then update the state of a slice by dispatching the actions generated by createSlice.
In this way, Redux-Thunk’s promise-based asynchronous control flow coupled with Redux Toolkit’s simplified state management paradigm makes for a potent combination in managing complex state scenarios in your application.
To echo a sentiment by Eric Elliott, a notable programming writer, “The best code is no code at all”. Indeed, the aim here is to reduce the amount of boilerplate code you have to write and increase the readability and maintainability of your codebase through logical structuring and compartmentalization.
Having said that, implementing Redux Thunk with Redux Toolkit’s createSlice not only helps streamline asynchronous actions in a Redux setup but also significantly condenses and enhances code scalability. Mastering these powerful tools truly carves an avenue to efficient and effective state management.
For further reading and illustration of using Redux-Thunk with Redux Toolkit’s CreateSlice refer to “Redux Toolkit usage with TypeScript“.