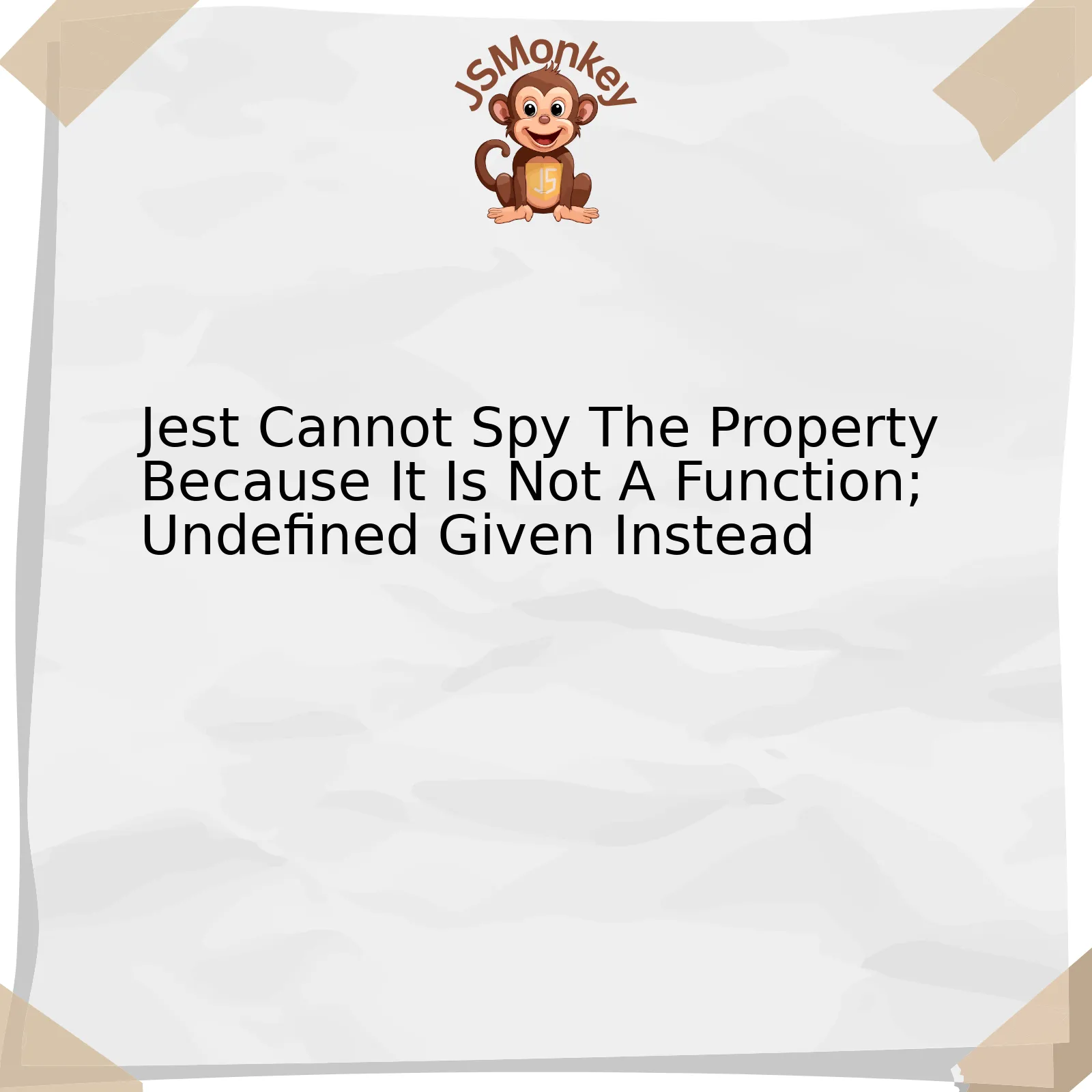
Problem | Causes | Solutions |
---|---|---|
Jest cannot spy the property because it is not a function; undefined given instead |
|
|
As illustrated in the above table, the issue, “Jest cannot spy the property because it is not a function; undefined given instead,” arises chiefly due to three main causes.
Firstly, this error may pop-up when the specified property doesn’t exist on the object being tested. For instance, when attempting to spy on a method that hasn’t been defined within the object. In such a case, validating the existence of the property is paramount.
Secondly, the problem can occur when the aforementioned property is present but isn’t a function. To rectify this, users should confirm whether the attribute they’re trying to spy on is essentially a method or an ordinary property.
Lastly, incorrect configuration or improper setting of Jest spies can lead to this error. Jest spies allow JavaScript developers to keep track of function calls and replace functions with mock implementations. If these setups are misdefined, it could lead to the software mistakenly identifying functions as undefined.
Some solutions include:
* Ensuring that the property you aim to spy on indeed exists within the object.
* Identifying the nature of the property in question. If it’s there, determine if it’s a function or an ordinary property.
* Properly setting up Jest spies, sabotage the real behavior and have control over results. To accomplish this, developers can refer to proper documentation [examples](https://jestjs.io/docs/jest-object#jestspyonobject-methodname).
As Jeff Atwood, co-founder of Stack Overflow, said,” Coding is not just about code, it’s predominantly a problem-solving task.” Therefore, understanding specific problems by breaking them down into their root causes plays a vital role in finding efficient solutions, just like dealing with this Jest spying error.
Understanding the “Jest Cannot Spy The Property” Error
The “Jest Cannot Spy the Property” error is a common issue encountered in Jest, a testing framework for JavaScript. This error typically occurs when you’re attempting to spy on a property with Jest’s `spyOn` method that isn’t a function, or it could be triggered if the property you’re trying to spy on doesn’t even exist.
To comprehend this error better, let’s break down the given topic: “Jest Cannot Spy The Property Because It Is Not A Function; Undefined Given Instead”.
Here are the key takeaways:
– First and foremost, Jest’s `spyOn` method is used to track calls on methods and functions by creating a mock function similar to the original function. You can use it to assert that a certain function was called with specific arguments or to count how many times it was executed.
const object = { method: () => { console.log("Original function") } } jest.spyOn(object, 'method')
In the example above, we are spying on the method ‘method’ of the defined ‘object’. Note that ‘method’ is indeed a function.
– Secondly, when receiving the error “Jest cannot spy the property because it is not a function; undefined given instead”, it means that the property you’re trying to spy on either doesn’t have a defined function or the property altogether doesn’t exist on the object.
For example, attempting to spy on a non-existing property ‘nonExistingMethod’ of our previous object would throw the discussed error:
jest.spyOn(object, 'nonExistingMethod')
Or attempting to spy on a property that is not a function, e.g., a numerical value or string:
const incorrectObject = { property: "Not a function" } jest.spyOn(incorrectObject, 'property')
Such actions would result in the “Jest Cannot Spy The Property” error.
In order to avoid this error and successfully implement testing with Jest, one should ensure:
– The property exists on the object.
– The property you’re spying on using `jest.spyOn()` is indeed a function.
– The object and its properties are properly imported if they originated from another module.
As wisely expressed by Robert C. Martin (also known as Uncle Bob), a notable software engineer and author, “The act of describing what we want the system to do helps us understand what we want the system to do.” Therefore, getting to grips with errors such as “Jest Cannot Spy The Property” is crucial not only for functional coding practices but also for a wholesome understanding of the code tested with Jest.
For detailed guidance on testing with Jest, consider referring to [Jest’s official Documentation](https://jestjs.io/docs/en/getting-started).
The Impact of Undefined Function on Jest’s Spy
Errors like “Cannot spy the property because it is not a function; undefined given instead” are undoubtedly negative impacts when trying to use Jest’s spying functionalities. They mainly occur if you’ve attempted to spy on a method or property which doesn’t exist, or isn’t available in the scope. It’s safe to indicate that this issue is directly related to an ‘undefined’ error.
Understanding the following can help address this:
1. Mocking and Spying
-
Jest.spyOn()
: This function in Jest is a powerful feature used for creating mock functions that allows us to spy on the function call, and simulate return values or exceptions.
- The error message – “Cannot spy the property ___ because it is not a function; undefined given instead.” reveals that
jest.spyOn()
cannot recognize what it has been directed at as a function.
2. Undefined Errors in JavaScript
- In JavaScript, ‘undefined’ is a built-in global variable that is automatically assigned by the system for uninitialized variables, missing parameters, and unknown property references. It signifies the absence of an established value.
- If assertion methods in Jest encounter objects or functions they’re asked to act upon but remain undefined, errors would be thrown accordingly.
3. Treating The Problem Within Jest
- Most of these issues resolve by doing reality-checks – ensure that you have imported/required modules correctly, reference functions accurately, and place calls within the appropriate scope.
- Null checks or checking for existence before the spying process can prevent bugs from creeping in unwittingly.
Remember Jean-Baptiste Queru’s quote, a well-renowned software engineer: “Coding is understanding.” Ensuring that your references exist and have defined values is a fundamental practice in programming. When you don’t use assertions on undefined properties or methods, Jest and your test suite will function smoothly.
For instance, suppose we have an object
calculator
that is supposed to have a method named
process
. Here’s an example of how to correctly set up a Jest spy:
// Assuming the calculator and its method exist const calculator = { process: () => "Done" }; it('should call process', () => { const spy = jest.spyOn(calculator, 'process'); calculator.process(); expect(spy).toHaveBeenCalled(); });
Essentially, this scenario underpins the consequence of calling an undefined function on Jest’s Spy – invalid operation and debugging the provoking error message.
Troubleshooting Steps: Bypassing Not a Function Errors in Jest
Addressing the error “Jest Cannot Spy The Property Because It Is Not A Function; Undefined Given Instead” requires a systematic approach. This specific issue is related to spying on methods or properties in Jest—a widely used testing framework in JavaScript—which surfaces when the item you’re spying on does not exist, or is not recognized as a function by Jest.
Effective Troubleshooting Measures:
To bypass and resolve this issue, several steps could be adopted:
Avoid Mocking Imported Modules Directly
When testing components that import other external modules, it’s advised to refrain from directly mocking these imported modules. Here’s why:
“It’s generally considered best practice in unit testing not to mock modules that you don’t own because doing so can lead to brittle tests.” – Nyman Vong, Technical Director at Medium One.
Introducing
jest.mock()
for spying can lead to the “Not a function” error if the spied module doesn’t exist or have the specified function.
Ensure Correct Syntax When Spying Methods
It’s important to make sure we are specifying the correct syntax while using spy methods. Incorrect syntax could lead to Jest not recognizing the method as a function.
The correct syntax for spying on a method is:
const spy = jest.spyOn(object, 'methodName');
.
Proper Initialization of Components or Functions
Before you spy on them, ensure that your components and their associated methods are properly initialized and available in your test environment.
For instance,
let component; beforeEach(() => { component = new MyClass(); });
If the methods are not correctly initialized, jest.spyOn might not recognize the property as a function which leads to the error.
Use Fixtures for Your Test Data
Carefully structure your inputs for units under test. Sometimes, using incorrect or improperly formatted test data may lead to the inability of Jest’s spying methodology in identifying a function.
Surround Your Code with Error Handlers
It’s always good practice to surround your tests with error handlers like .catch() or .finally(), just to ensure that any potential exceptions are caught early, and can be addressed appropriately.
test() { ... } .catch(err => console.error(err));
By following these steps, you should avoid stumbling upon “Not a Function” errors while using Jest. For more insights and guide on JavaScript testing with Jest, explore Official Jest Documentation.
Implementing Solutions for “Undefined Given Instead” Issues in Jest
Jest, an open-source JavaScript testing framework developed by Facebook, offers a diverse suite of features that help developers test all JavaScript code, including React applications. While Jest is favored for its simplicity, it does bring about certain challenges in real project implementations. Amongst these, the caveat of “Cannot spy on property because it is not a function; undefined given instead” and “undefined given instead” errors can befall developers quite frequently. In this context, various remedial strategies can be considered to address these issues.
Firstly, let’s understand suffering the error “Cannot spy on a property because it is not a function; undefined given instead”. This occurs while spying on a function or method that does not exist or is not defined correctly. Essentially, the case arises because Jest selectively binds setRoles depending on whether you’re in production or development mode. Jest.spyOn() calls fail since the properties they’re trying to spy on do not exist in the execution environment.
A start for the solution would often involve rectifying the references:
// Wrong jest.spyOn(MyObject.prototype, 'myMethod'); // Correct jest.spyOn(instance, 'myMethod');
The `prototype` has been changed to a particular `instance` of your object. The Jest API has no compatibility with static methods, thus this workaround provides rescue.
Moving onto the “Undefined Given Instead” issue, the probable reason can be that the function you want to test has not yet been defined or imported correctly. To successfully run Jest spies, pay high attention to:
– How the function is exported,
– How it is imported into test file,
– Real-time changes to checked files,
Incorporation of these practices individually will likely mend the common Jest challenges experienced in JavaScript development lifecycle.
To quote Miriam Suzanne —a web developer, “It’s not just about writing code. It’s about sharing the knowledge”. While solving problems comes with experience and dedication, sharing what we know can help other developers avoid the common errors or dilemmas that we have faced.
Speaking of third-party useState hooks, these are also a commonly encountered situation. Here, instead of accessing the property you aim for, access the Hook Instance directly by using `jest.spyOn(React, ‘useState’)`.
As with any development tool, Jest should be mastered through both understanding its application, and overcoming common pitfalls to test and debug JavaScript code effectively.
Remember Billy Gregory’s words: “When UX doesn’t consider all users, shouldn’t it minimize harm for those it doesn’t serve?” Just like in inclusive design, in testing too, we must attempt to foresee as many edge cases as possible for comprehensive validation.
Despite all efforts, remember that errors and exceptions are normal aspects of programming. They contribute significantly towards improving the robustness of your code by questioning assumptions and minimizing potential flaws, thereby making the project increasingly resistant to future issues.
Jest, a globally acclaimed JavaScript Testing Framework, provides us with the necessary tools to sync our testing strategies with current development methodologies. One of its notable features is function spying – allowing one to track calls to a particular function. But it’s not always sunshine and rainbows in the world of coding, as we encounter issues such as: ‘Jest cannot spy the property because it is not a function; undefined given instead’. This error typically arises when we try to ‘spy’ on a function or method that doesn’t exist.
Before venturing into the resolution, it is important to understand why it happens:
• The occurrence of this error can be attributed to multiple causes but is predominantly due to an attempt to spy on an object property that is not definable as a function.
• It might also occur if the object property hasn’t been initialized before Jest’s spying mechanism steps in.
So, what are the possible steps to rectify the issue?
Resolution Steps |
---|
Make sure that the property being spied on is a function. |
Validate if the said property has been instantiated before the spy gets invoked. |
Recheck your code for typographical errors where the function name could possibly be misspelt. |
In case of using ES6 classes, ensure that you deploy instance methods instead of arrow functions. |
Our code ecosystem can experience hiccups such as ‘Jest cannot spy the property because it is not a function; undefined given instead,’ which all software developers must tackle effectively. Thanks to the robust community online ready to help and well-documented articles, finding solutions to these small roadblocks has become more accessible.
To draw a quote from Adrian Crockford, the man who brought us JSON format – “Programming is the most complex artefact that humans have ever created. It involves a significant amount of creative engineering and problem-solving skills.” Thus, such hurdles are more opportunities to learn new things about this wonderfully complex artefact in doing so, we evolve as better developers.
For a wider context on JavaScript Testing Library Jest, you can refer to Jest documentation.
Remember, in our path to developing impeccable software, it is not the syntax or the method, but thorough testing and handling these minor obstacles that shape us into excellent coders.