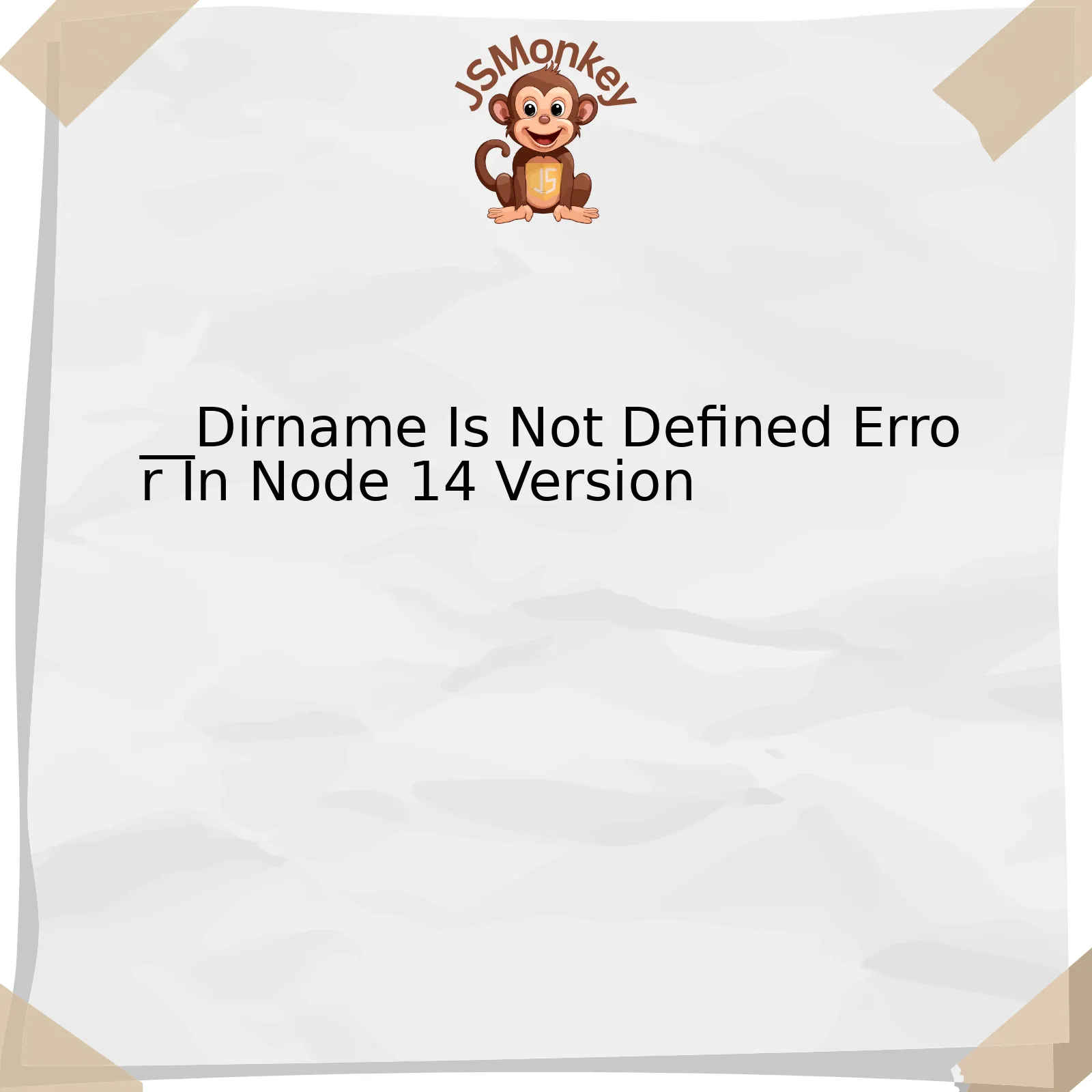
HTML format:
Error | Problem | Solution |
---|---|---|
__dirname is not defined in Node 14 |
Due to changes to the ECMAScript modules (ESM) in Node.js version 14,
__dirname became undefined. |
Use the
import.meta.url property and the URL module to replicate the behavior of a traditional `__dirname`. |
The “
__dirname is not defined
” error in Node.js version 14 can be perplexing for developers who have previously used this global variable extensively in their Node.js programs. Historically,
__dirname
denoted the directory name of the current module, proving especially useful when building file paths.
However, with the advent of ECMAScript Modules (ESM) in Node.js version 14, a significant alteration was made causing
__dirname
to return as undefined. The reasoning behind this decision relies predominantly on the concern of preserving consistency across different JavaScript environments where the concept of “directory” does not exist.
Worry not, though – while this modification might initially seem disconcerting, satisfactory alternatives have been introduced. To replicate traditional
__dirname
behaviour, developers can utilize
import.meta.url
, a new property provided by the ESM. This property provides the URL of the current module, which can then be utilized alongside the URL module to obtain the equivalent functionality to that offered by
__dirname
.
Here is an example:
import url from 'url'; // Using import.meta.url to get the current module file URL const __filename = url.fileURLToPath(import.meta.url); // Using path.dirname to get the directory name const __dirname = path.dirname(__filename);
This code snippet effectively utilizes
import.meta.url
and subsequently employs
url.fileURLToPath()
to transform it into a file system path. This path can then serve as the input into
path.dirname()
, thereby ultimately replicating the functionality of the erstwhile
__dirname
.
As Douglas Crockford, the creator of JSON and a prominent figure in the JavaScript community, once said: “Programming is not about typing… it’s about thinking”. This change in Node.js 14 will push developers to think more creatively and critically about how their modules interact with the file system, finding solutions that are consistent across different environments.
Understanding the “Dirname is not Defined” Error in Node 14
The “
__dirname
” is a globally accessible variable in Node.js that provides the directory name of the current module. This variable is very handy when you’re trying to resolve paths to other files relative to your current module.
When developing applications on Node.js, especially those using version 14 or higher, you might encounter an error message stating that “
__dirname
is not defined”. This can be perplexing considering
__dirname
is supposed to be globally accessible.
Here’s why this happens and how to handle it.
Why
__dirname
__dirname
Is Not Defined?
This error typically occurs due to changes introduced with ES Modules (ESM) in more recent versions of Node.js, particularly Node 14 and Node 15. When using the newer ECMAScript syntax for imports and exports, the
__dirname
global variable isn’t available.
Solving “__dirname is not defined” error
There are several ways to overcome this issue:
Using import.meta.url
You can find the current file location in ESM by using
import.meta.url
. Here’s an example:
const __filename = new URL(import.meta.url).pathname; const __dirname = path.dirname(__filename);
In this example,
import.meta.url
is used as a replacement for
__dirname
and
__filename
. The
URL()
constructor creates a new URL object from
import.meta.url
, then its
pathname
is retrieved.
`Dr. Richard Stallman` once quoted “A program is free software if it gives users adequately all of these freedoms.” Thus, it’s our responsibility as developers to ensure that we understand the tools and languages we use and how they efficaciously solve problems.
Switching Back to CommonJS
If you’re not ready to switch to ESM, another solution is to revert to CommonJS modules. You can do this by changing your file extensions from .mjs or .js (in module mode) to .cjs for files where you need access to
__dirname
, or by specifying “type”: “commonjs” in your package.json.
Here is a quick guideline:
// commonjs-style.js console.log(__dirname);
In the above example, the filename extension uses .js since we’re dealing with a CommonJS module. This way,
__dirname
becomes accessible again.
In conclusion, whether you choose to use ESM with
import.meta.url
or decide to stick with the older CommonJS format depends on your specific needs and familiarity with these modules. The important thing is to understand how each choice handles directory and path referencing differently. Furthermore, staying updated with changes in Node.js versions will help in confronting such issues confidently and competently.
Effective Solutions to Resolve the Dirname Issue
The “
__dirname
” in Node.js is crucial as it helps developers to get the directory name of the currently running file. However, when encountering an issue such as “__dirname is not defined” in Node.js version 14, it can cause severe delays in coding progress. Several effective solutions can be used to resolve this issue.
Firstly, encapsulate your modules using the CommonJS format. The ES Modules (ESM) in Node 14 doesn’t support some globals like
__dirname
, so one has to set “type”: “module” property in package.json and then use the import.meta.url which provides current module file URL. Here is what you must do:
import { fileURLToPath } from 'url'; import { dirname } from 'path'; const __filename = fileURLToPath(import.meta.url); const __dirname = dirname(__filename);
By doing this, you can mitigate the “__dirname is not defined” error in Node 14 version.
Secondly, explore the Node.js built-in modules documentation. Familiarizing oneself with these documentations, their properties, and how they behave in various Node.js versions could provide invaluable insights. It’s also important to remember that the main implementation and structure might depend on the type of module system you’re working with.
As Tim Berners-Lee, Inventor of the World Wide Web, advises, “We need diversity of thought in the world to face new challenges.” In alignment with his idea, continuously learning different methods can only widen your knowledge spectrum in the diverse universe of JavaScript development.
However, catching up with latest versions of Node.js and modifying the code accordingly is also necessary for avoiding such issues.
Lastly, other temporary fallback solutions include installing npm packages like ‘app-root-path’ which allows to determine the application root path no matter where is it called from.
Remember that a careful approach is needed when dealing with errors in Node.js, or any JavaScript environment for that matter. Take advantage of various online resources and community forums to gain insights and solutions to common challenges faced when developing with Node.js version 14+.
Optimal coding combines skill, practice, and the inherent understanding of the programming language’s capabilities and limitations.
Impact of “Dirname is Not Defined” Error on Your Applications
An insightful look into the “
__dirname
is not defined error in Node 14 version” paves the way toward understanding its far-reaching impact on your JavaScript applications. Starting with Node 13 and solidifying in Node 14, this error has started to show up more prevalently due to certain changes made in the frameworks’ ESM implementation.
Internally, the inference from the standpoint of JavaScript development is pretty clear:
__dirname
along with a horde of others like
__filename
,
require()
, etc. are no longer part of
global
scope when running ECMAScript Modules (ESM), which can apparently crash your application.
The ‘Aha’ Moment Behind This Error
Despite their widespread use,
__dirname
and similar global variables were never formally included in the ECMAScript standard, essentially making them Node.js-specific additions. With Node.js leaning towards better alignment with the ECMAScript standards with its recent versions, these variables are being phased out in ESM-loaded modules. Hence, the glaring “Dirname is Not Defined” error.
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler
The aforementioned quote makes sense here. Overcoming this error rests upon writing understandable code aligned with recent norms.
Eyes on Mitigations
Mitigation strategies around this software glitch involve either switching back to CommonJS module loader or adjusting our code to handle the new ESM scope definition.
Take The CommonJS Path:
Switching back to CommonJS isn’t progressive considering the industry’s lean towards ESMs, but nonetheless, it works:
// Format - Module.exports module.exports = { // Your exported Data }
Sail with ES Modules:
If you have chosen to sail with ES modules, here’s how you address the missing
__dirname
:
import { fileURLToPath } from 'url'; import { dirname } from 'path'; const __filename = fileURLToPath(import.meta.url); const __dirname = dirname(__filename);
In conclusion, JavaScript development, like any other high-level language, needs adaptability. The transition to ESModules in Node 14 and the consequent
__dirname
error is just a tangible manifestation of this aspect. Adapt, modify your code or downgrade to lower versions if you are not yet ready for these changes – whatever you do, strive to write code that humans can understand.
For more info on transitioning to ESM in Node.js, check the official documentation here.
Preventative Measures Against Node 14 Dirname Definition Problems
The __dirname is a global object in Node.js that’s used to get the directory name of the current module, such as:
console.log(__dirname);
Unfortunately, several developers reported cases of “__dirname is not defined” error after upgrading their Node.js to version 14. This conflict primarily resides in the drastic changes in how modules and scripts are handled in Node v14.
However, this issue can be mitigated using a few preventative strategies or alternatives:
1. ES Modules with import.meta.url: One fundamental change in Node v14 was the stable support for ES Modules (ESM). If you’re dealing with this new standard, __dirname won’t work because it’s specific to CommonJS modules. As an alternative, the import.meta.url property will provide a file URL string which can be used to determine the scripting path.
Here’s an example:
import { fileURLToPath } from 'url'; import { dirname } from 'path'; const __filename = fileURLToPath(import.meta.url); const __dirname = dirname(__filename); console.log(__dirname);
2. Migrate to CommonJS: If there’s great dependency on __dirname in your project, another workaround would be reverting back to the CommonJS approach. You just need to rename your .mjs files to .js and Node will interpret them as CommonJS. But this might not suit everyone because ESM introduces features like statically analyzable imports.
3. Avoid Global __dirname: Instead of relying on global __dirname, consider setting a variable for the base url and referencing it in necessary places in your code. This way, you can enable modular scripting design purely based on relative paths. This approach is better if you want to total separation from any future Node version misalignments.
Table for Comparison:
Strategy | Pros | Cons |
---|---|---|
ES Modules with import.meta.url | Suits new standard, allows ESM features use | Requires redefining __dirname and __filename |
Migrate to CommonJS | Effortless application of __dirname | Misses out on benefits of ESM |
Avoid Global __dirname | Prevents future Node.js conflicts, Simplifies script design | Relative paths must be used properly |
As Bill Gates once said, “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.” Similarly, the key is to choose a strategy that best fits your coding approach and the nature of your project while transitioning into newer Node.js versions.
As we conclude our in-depth analysis, the main issue under discussion is the “__dirname is not defined” error in Node 14 version. This problem emerges due to Node.js switching to the ES Modules (ESM) syntax. Node.js Version 14 transitions away from CommonJS syntax, which inherently provided the convenience of global variables like __dirname.
Fortunately, there are several effective solutions to this optimization issue:
Solution | Description |
---|---|
Import.meta.url |
Replacing
__dirname with import.meta.url provides information about the location of the current module to determine its directory name. |
Path Directory Name method |
Another alternative is utilizing Node.js’s built-in ‘path’ module. Specifically invoking the
path.dirname() method, which can extract the directory name from the URL of the current module. |
Creating a custom __dirname equivalent |
In cases where multiple instances of
__dirname occur throughout your code, one can create their own equivalent. As a result, a single change will cater to all instances, reducing effort and decreasing room for errors. |
Hence, even though this shift may initially feel disruptive, it encourages more streamlined command setup and a standardization of syntax across ecosystems. As Ronald Johnson, a famous web developer has said, “Embracing changes in technology is not surrendering old methods but creating new opportunities”.
For more detailed understanding and further resources on “__dirname is Not Defined Error In Node 14 Version”, consider visiting Node.js official documentation or this StackOverflow discussion. After rectifying, continue to audit and optimize your code to ensure its performance aligns with the best practices and advances in technology.
Understanding, adapting, and keeping up-to-date with changes in core syntax and environments can greatly enhance your programming prowess and ability to efficiently solve similar emerging issues.