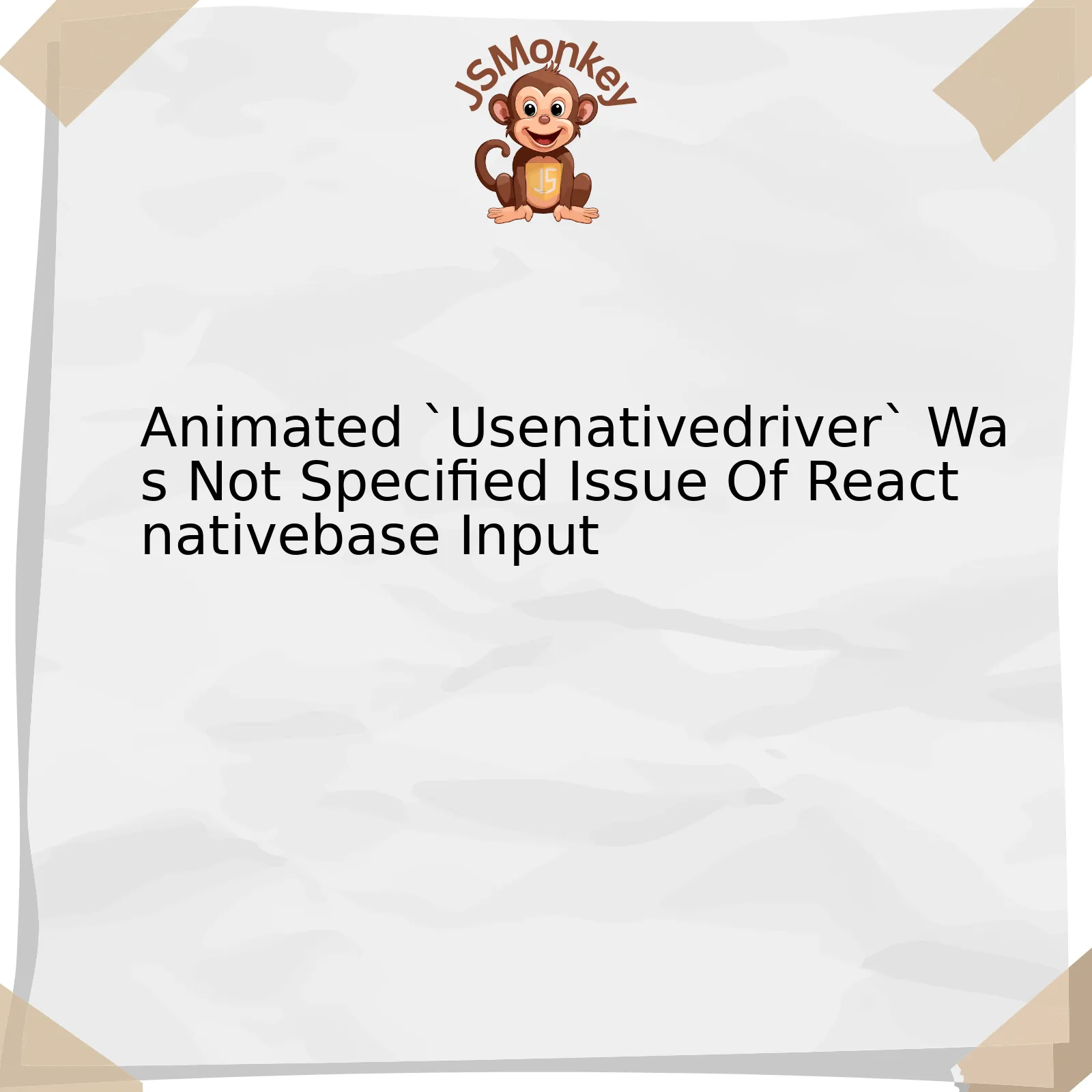
Property | Description |
---|---|
useNativeDriver |
This is a property associated with animation systems in React Native. It permits animations to be sent directly to the native module, bypassing JavaScript thread. This leads to smoother and more efficient execution of animations. |
ReactNativeBase Input |
It’s a key component provided by the React Native Base library. It serves as an enhanced version of typical input fields, offering added flexibility and customization options for developers. |
‘Not Specified’ Issue | The ‘not specified’ problem transpires when this
useNativeDriver property isn’t set during configuration. Since it is integral to the implementation of animations, its absence can lead to unexpected behaviors or errors. |
Expanding on the table, let me dive deeper into each point.
The
useNativeDriver
is a boon to developers working on enhancing the aesthetic appeal of their apps using various animations. By allowing the animations to be driven directly on the native side, it provides a significant boost in performance compared to running everything on the JavaScript thread.
On the other hand, we have
ReactNativeBase Input
. Leveraging the power of this component can give your app’s input fields a level of aesthetics and fluidity that is rivaled by few. However, its deep association with underlying animations means any missed or misconfigured properties can lead to hiccups in the UI/UX.
This brings us to the ‘Not Specified’ issue. If the
useNativeDriver
property is not declared in your animation configurations, react-native doesn’t know if you prefer running animations on the JavaScript thread or natively. It ensues in inconsistencies, sending you into debugging frenzy.
To avoid this issue, you should explicitly define whether you want to execute animations natively or on the JavaScript thread. For example:
Animated.timing(this.state.animatedValue, { toValue: 1, duration: 500, useNativeDriver: true, // Specify here }).start();
Remember what Bill Gates once quoted about technology and problem-solving: “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency. The second is that automation applied to an inefficient operation will magnify the inefficiency.”. This emphasizes the importance of correctly implementing and leveraging technological features provided by libraries like React Native Base.
Understanding the ‘Usenativedriver’ in React Native Base Input
The `useNativeDriver` is a configuration option for animations in React Native. It’s utilized to specify whether the animation should be performed on the native side or not, directly affecting the smoothness and performance of the animations. As for the input component from React Native Base, the said specification impacts how the keyboard behavior is managed upon user interaction.
When you encounter an issue stating – “Animated useNativeDriver was not specified”, it means that the `useNativeDriver` value has not been provided explicitly. This case is rampant in the inputs of the ReactNativeBase library. The error screams for one necessity – the system must know whose responsibility of accomplishing the animations lies where: JavaScript, or the Native UI thread?
Let’s elaborate on those two:
– JavaScript Thread: By default, the JavaScript thread handles your animations. It implies that every time you animate a component, JavaScript creates each frame for the animation which can cause offbeat scenarios if your JavaScript thread is busy.
– Native UI Thread: Alternatively, offloading work to native speeds up the animation process thanks to the inherent prowess of native code over JavaScript regarding raw computation power. That frees up the JS thread and also results in smoother animations with a higher frames-per-second (fps) count.
Given these circumstances, it’s advisable to specify the `useNativeDriver` parameter when you are creating animations. Here’s a general example of how you’d specify this in your code:
Animated.timing(this.state.animatedValue, { toValue: 1, duration: 500, useNativeDriver: true, // Explicitly mention this }).start();
You’ll notice the addition of `useNativeDriver: true` in the animation config. This form explicitly tells the system to run the animation on the Native (UI) thread.
However, remember that using `useNativeDriver: true`, might not always be beneficial depending on the type of animations or transformations you are applying.
As an additional note, there’s a word of wisdom by Jim Barksdale, former CEO of Netscape – “The main thing that has caused companies to fail, in my view, is that they missed the future.” That’s why it’s pivotal for developers to stay ahead and updated about such nuances in frameworks like React Native.
Look at React Native’s official documentation to get more insights into this topic.
Resolving the Animated ‘Usenativedriver’ Not Specified Issue
The
useNativeDriver
warning often occurs when you’re using animations in React Native. This notification implies that the
useNativeDriver
property has not been specified for the particular animation.
To dispel the issue at hand, particularly for ReactNativeBase Input animations, you’ll need to set the
useNativeDriver
property. Considering the inefficiency of JavaScript-based animations in terms of performance, Facebook’s React Native team implemented a native driver that sends animations across the bridge only once and then performs them on the native side. This dramatically increases the fluidity and responsiveness of animations, hence its indispensable usage.
Here is a fundamental example of how you can specify it:
Code Before | Code After |
---|---|
Animated.timing(this.state.animatedValue, { toValue: 1, duration: 500 }).start(); |
Animated.timing(this.state.animatedValue, { toValue: 1, duration: 500, useNativeDriver: false //add this line }).start(); |
In this specific case, proper use of the
useNativeDriver
trait is mandatory. The value can be either true or false, reflecting whether you want to use native driver for the animation or not. Note, however, that not all styles and types of animations can leverage the native driver. Transformations like scale, translate, rotate, and opacity would thrive with the native driver due to their direct mapping on Android and iOS platforms.
As Michael Jackson, co-creator of React Router said, “Getting good at programming is like getting good at anything else. It takes a lot of practice.” Consequently, as you tackle the issue of ReactNativeBase Input in your animations, I encourage you to lunge into this practice and consequently unearth the versatility of the
useNativeDriver
property. You’ll need all the resolve to debug and optimize your animations, but eventually, your app performance’s noticeable improvement will justify the effort.
You can read more about the native driver in the official documentation [link].
Advanced Approaches to Tackle React Native Base Input Bugs
In the context of React Native base input bugs, developers frequently encounter an issue related to the Animated `useNativeDriver` not being specified. Understanding this problem first necessitates understanding how React Native and its animations work. React Native is renowned for being flexible and allowing developers to code in JavaScript while delivering a near-native app experience. However, like any technology, it also has its share of quirks. One such quirk is the notorious Animated `useNativeDriver` bug.
The `useNativeDriver` property is crucial to the execution of animations in React Native. When set to true, it directs the animation to be sent directly to the native side as opposed to JavaScript thread. This can dramatically increase performance, especially on lower-end devices or complex views with many layers of depth.
]]> Animated.timing(this.state.animatedValue, { toValue: 1, duration: 500, useNativeDriver: true, // Add this line }).start(); ]]> |
However, omitting the `useNativeDriver` property or leaving it undefined can trigger an error simply because - as of React Native 0.62 - you're required to specify whether or not you want your animations to use the Native Driver.
Fixing this issue revolves around two main strategies:
- Always define the `useNativeDriver` property within your animation configuration.
- Use a defensive programming approach; ensure all animations have fallback values that prevent a cascading failure of your entire application if an error arises.
You can also make use of some available toolkits and guidelines. For instance, the official React Native documentation mentions the Animated library that provides a host of utility functions that aid in the handling of animations, including dealing with the `useNativeDriver` property.
At times, patches often arrive via updates to the libraries you're using in your project. Regularly updating them ensures not just improved functionality, but also critical bug fixes.
By considering these methodologies, you should be well-equipped to tackle quirks like the Animated `useNativeDriver` issue as you advance in your React Native development journey.
Check out this link to learn more about Animation in React Native base.
Make sure to check any changes into your version control system to back up your progress. Following these strategies will not only improve your application's performance but also elevate your proficiency within the React ecosystem. Using these strategies further enhances your ability to anticipate and rectify such issues in future developments, fostering greater efficiency and precision in crafting seamless user experiences.
Tips and Best Practices for Using Usenativedriver in Reactnativebase Input
The `useNativeDriver` property is an important aspect to consider when working with animations in React Native. Sometimes, you may encounter an issue where the `Animated useNativeDriver` was not specified, leading to warnings in the console. This tip focuses on understanding what this property does and how to use it effectively to fix this common problem in react-native-base input.
Here's a deep dive into the concept:
- What `useNativeDriver` Does
The `useNativeDriver` attribute enhances the performance of your animation sequences. Unlike the typical JavaScript-driven animations in React Native that run on the JavaScript thread, setting `useNativeDriver` to true allows the animations to run directly on the UI thread. This has substantial repercussions for dealing with animations at scale as it significantly boosts speed and smoothened transitions, reducing the likelihood of dropped frames.
- Best Practice for Using Usenativedriver
Consider whether or not to use `useNativeDriver`. Although it is a powerful tool, it’s not universally applicable to all types of animations. Only specific properties such as opacity and transform are compatible with the native driver. Attempting to animate non-compatible properties will lead to runtime errors. So, it’s crucial to only set `useNativeDriver` to `true` when appropriate.
Here’s an example:
<Animated.View style={{opacity: this.state.fadeAnim}}> <Text>Fading in</Text> </Animated.View>
In this code snippet, an opacity animation perfectly warrants the use of `useNativeDriver` because opacity is a supported property.
- Fixing "Animated `useNativeDriver` Was Not Specified" Issue
This warning mostly occurs due to a missing specification for the `useNativeDriver`. In recent versions of React-Native, its specification became mandatory for improved execution control and better debugging. To fix this, you can set the `useNativeDriver` value explicitly in your animation setup:
this.state.fadeAnim = new Animated.Value(0); Animated.timing( this.state.fadeAnim, {toValue: 1, duration: 2000, useNativeDriver: false}, ).start();
In this code, `useNativeDriver` is explicitly set to `false`. The warning should no longer occur after including it.
To wrap it up: understanding when and how to use the `useNativeDriver` property in React Native animations is key to efficient programming. While aiming for superior performance, always ensure you’re abiding by the compatibility constraints of this property to prevent unnecessary runtime errors.
Happy coding!
As Bill Gates once said,
"The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency. The second is that automation applied to an inefficient operation will magnify the inefficiency."
The same holds true with the use of native drivers in animations. Efficient usage significantly boosts your application's overall performance, while improper utilization only leads to greater problems.
For more in-depth details, please check out these articles on React Native's official documentation and on React Native Best Practices.
React Native Base components have enjoyed extensive usage in the development of cross-platform applications. In particular, their user input management features have been well-reputed for their performance and ease-of-use. Nonetheless, there are certain issues developers frequently encounter, particularly the `Animated 'useNativeDriver' was not specified` issue.
In order to fully grasp the implications of this issue, it's necessary to comprehend the role that 'useNativeDriver' plays within the React Native animation architecture. Typically, animations are handled on the JavaScript thread, but this can lead to performance problems when the thread is congested with other tasks. The 'useNativeDriver' property allows animations to be offloaded to the native (iOS/Android) side, freeing up the JavaScript thread for other tasks.
To resolve the `Animated 'useNativeDriver' was not specified` issue, a developer should explicitly declare whether the useNativeDriver should be used. A specified Boolean value (true or false) should be incorporated into the animation configurations, such as
{useNativeDriver: true}
or
{useNativeDriver: false}
.
The choice between 'true' and 'false' ought to be informed by your specific app requirements. If you're utilizing an animation that can be managed natively (e.g., opacity or transforms), setting 'useNativeDriver' to 'true' could yield significant performance improvements. However, for complex animations or those manipulating non-natively-animatable styles, 'useNativeDriver' must be set to 'false'.
Remember, specifying 'useNativeDriver' isn't just about averting warnings—it offers a chance to improve your application's performance. Your pursuit for a smoother cross-platform application experience starts with an understanding of how animations work within the React Native context and ends with effective management of these critical resources.
As the technology sage Steve Jobs once stated, “Details matter, it’s worth waiting to get it right”.
References (check the hyperlink for more information):
1. [React Native Animation](https://reactnative.dev/docs/animations)
2. [React Native Base Input Components](https://docs.nativebase.io/Components.html#input-default-headref)