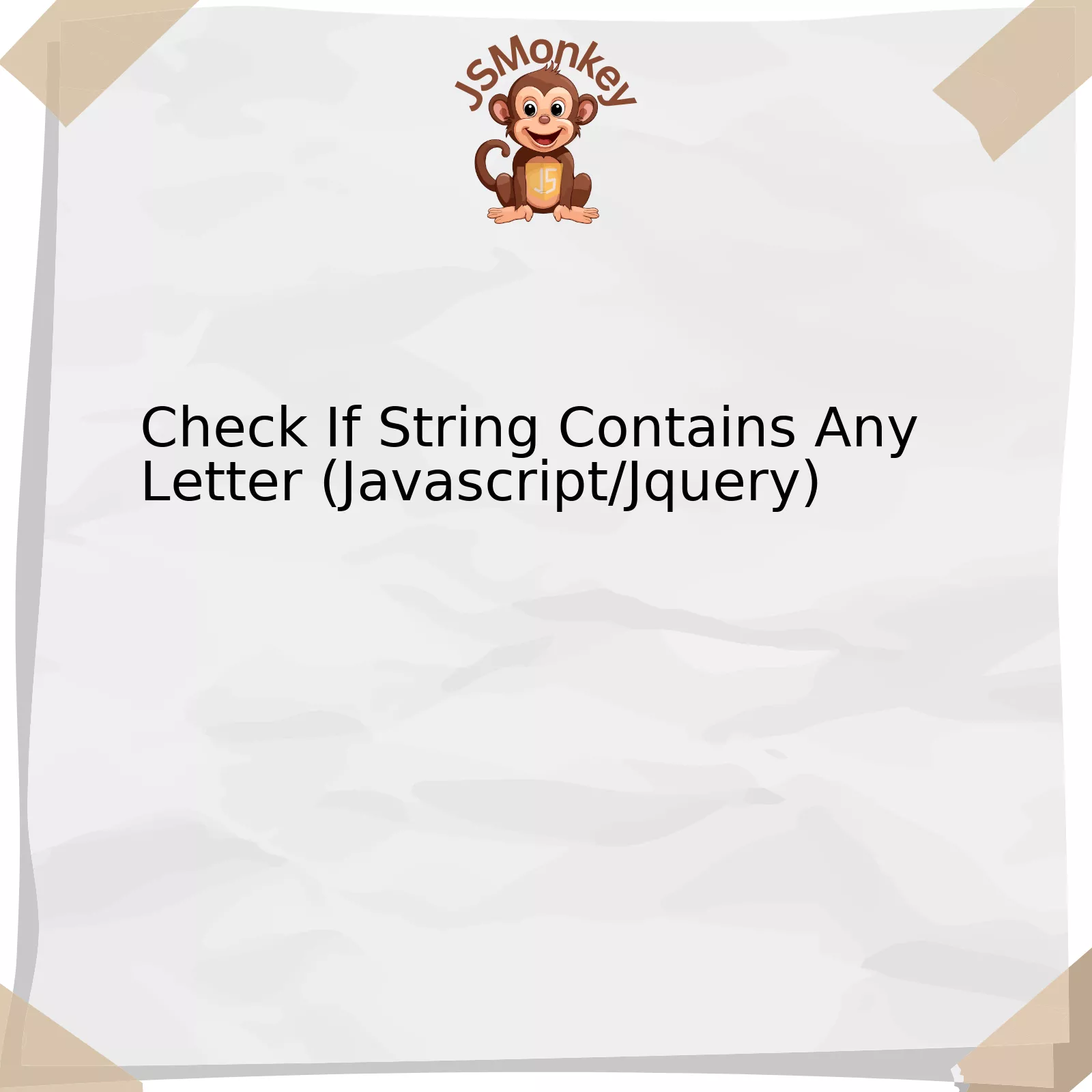
Method | Description |
---|---|
test() |
This method executes a search for a match between a regular expression and a specified string. Returns true or false. |
match() |
This method searches a string for a match against a regular expression, and returns the matches as an Array object. |
Checking if a string contains any letter in JavaScript or jQuery is ideally achieved by utilizing specific methods embedded within these programming languages. These two functionalities under discussion are `test()` and `match()` methods.
The `test()` method in JavaScript engages in a search process for a relation between a regular expression and a specific string. This produces a Boolean value after executing the operation, indicating whether the sought-after match was found- it reflects ‘true’ for a positive finding, and ‘false’ for a negative outcome.
Here’s an example of how you would use it:
let regex = /[a-zA-Z]/; console.log(regex.test("1234")); // false console.log(regex.test("1234a")); // true
On the other hand, the `match()` method probes a string in search of a match that identifies with a regular expression. It outputs the matches in the form of an Array object. If there is no match, it will return `null`.
For instance, for using `match()`, this could be seen:
let str = "Hello World!"; let result = str.match(/[a-z]/gi); console.log(result); // ["H", "e", "l", "l", "o", ..., "l", "d"]
To further enlighten on the subject, *[a-zA-Z]* in a regular expression indicates a range from ‘a’ to ‘z’ and ‘A’ to ‘Z’, hence encompassing all alphabets. The `i` flag renders the search case-insensitive.
As the famous computer scientist Grace Hopper once said, “To me programming is more than an important practical art. It is also a gigantic undertaking in the foundations of knowledge.”
In summary, both methods serve effectively when seeking if a string contains any letter in JavaScript or jQuery. They employ regular expressions—a powerful tool in pattern matching and parsing strings, making them extremely essential and handy for programmers and developers specializing in these languages.
Understanding String Operations in JavaScript
To understand how to check if a string contains any letter in JavaScript, we need first to delve into the fundamental concepts about string operations. By string operations, we are referring to the various actions that you can perform on a text (strings), such as finding, replacing, or comparing values.
JavaScript provides us with several built-in methods which facilitate a plethora of String operations. Notably, among these functions, the ‘match’ and ‘test’ functions serve prominently when it comes to checking letters in a string.
Check If a String Contains Any Letter Using match() Method
The match() method is used to search strings using regular expressions. Here’s an example:
const str = "1234"; const regex = /[a-zA-Z]/g; // specifies any letter from a - z (case insensitive) const found = str.match(regex); if(found) { // code for when a letter is found } else { // code for when no letter is found }
In this snippet, match() uses a regular expression to look for any occurrence of a letter (upper or lower case) in the string. If it finds a match, it returns an array containing the matches. Otherwise, it will return null.
Check If a String Contains Any Letter Using test() Method?
The RegExp test() method is an alternative approach. This method checks for matches within a string and returns a boolean value: true if it finds a match, false otherwise.
const str = "1234"; const regex = /[a-zA-Z]/; // specifies any letter from a-z (case insensitive) const hasLetter = regex.test(str); // gives you true if a letter is found, or false if no letter is found in 'str'
Using these two techniques—match() and test()—you can not only detect the existence of any letter with JavaScript, but also ascertain their position or frequency within your strings.
As former Google Developer Expert Addy Osmani said, “First do it, then do it right, then do it better.” Grasping these fundamental string operations can lead to more efficient and cleaner codes which are easier to maintain in the long-run.
For additional details on JavaScript String Manipulations you can visit Mozilla Developer Network guide on Regular Expressions.
Utilizing jQuery to Validate Strings for Letters
For any application’s data validation process, mastering how to perform letter checks on strings is a crucial requirement. In JavaScript, multiple methods exist for validating if a string contains any letter(s), either by utilizing pure JavaScript or with the help of libraries such as jQuery.
Using Regular Expressions in JavaScript
You can use regular expressions (Regex) for this purpose. They allow you to match patterns within a string and can be quite formidable for checking if a string contains letters. Here’s an example:
var str = "123abc456"; var regex = /[a-zA-Z]/g; console.log(regex.test(str)); // returns true
The regular expression
/[a-zA-Z]/g
here checks whether there are any uppercase or lowercase letters in the given JavaScript string and returns a boolean value – true if the string has a letter and false if it doesn’t.
String.prototype.search() Method
Another native way to check if a string holds any letters is using the
String.prototype.search()
function available in JavaScript:
var str = "123abc456"; var result = str.search(/[a-zA-Z]/); console.log(result > -1); // returns true
In this method, the
search()
method will return the index of the first letter match it encounters. If no matches are found, it returns -1.
Warning:
Both methods mentioned above work perfectly, but they may fail on Unicode characters. For example, non-ASCII letters such as “å” would not be matched by these expressions. To include such letters, modify the regular expression to
/\p{L}/
.
Utilizing jQuery and Regular Expressions
Though jQuery itself does not provide any inbuilt functions for this specific task, you can combine it with Regular Expressions through event handlers and validators to enhance your validation processes on user inputs:
$("#inputId").on('input', function() { var input = $(this).val(); var regex = /[a-zA-Z]/g; if(regex.test(input)) { alert("String contains letters"); } });
This would raise an alert as soon as the user enters a letter into the identified input field.
“h5>Takeaways:
– Opting for a regular expression test or
String.prototype.search()
can be a matter of personal preference or driven by code efficiency.
– jQuery does not inherently include string validation utilities for checking presence of letters. Nevertheless, its interactivity can be interfaced with Regex for both real-time and after-input validations.
“One of the best programming skills you can have is knowing when to walk away for awhile.” – Oscar Godson.
Exploring Methods for Identifying Letters in JavaScript Strings
Exploring Methods for Identifying Letters in JavaScript Strings Relevant to Checking If String Contains Any Letter
When you’re working with strings in JavaScript or jQuery, one of the common tasks might be checking if a string contains any letter. There are various ways in which developers can perform this check.
Typically, this involves utilising methods like
RegExp.test()
,
String.indexOf()
,
String.includes()
or using Regular Expression directly. Each of these strategies depends on different approaches:
- Regular Expressions and RegExp.test() method:
The
RegExp.test()
method is quite efficient as it ends its search as soon as it finds a match. This saves computational resources specially for very large strings. The following code snippet shows how you can use
RegExp.test()
to find whether a string contains any letters:
let str = “Hello123”;
let regex = /[a-zA-Z]/g;
if(regex.test(str)){
console.log(‘Letter Found’);
} else {
console.log(‘No letter Found’);
};
- String.indexOf() method:
Another approach to check if a string contains any alphabet character involves using the powerful
indexOf()
method built into JavaScript’s String object. This method will return the first index at which a specified character or string can be found, or -1 if it can’t be found. Here’s an application of this approach:
function hasLetter(s) {
for (let i = 0; i < s.length; i++) {
const code = s[i].toUpperCase().charCodeAt(0);
if (code >= 65 && code <= 90) {
return true;
}
}
return false;
}
- String.includes() method:
Although it’s not as efficient as the RegExp.test() method due to its linear search algorithm, the String.includes() method is straightforward and easy to read. This method determines whether one string may be found within another string, returning true or false as appropriate.
Below is a relevant quote from Jamie Zawinski which emphasizes the power of regular expressions when used appropriately in such situations:
“Regular expressions are incredibly powerful, but they can also be fairly complex. By combining different regex ingredients together like an alchemist, you can concoct some impressive coding solutions—even with just a basic understanding of what everything does.” [source](https://www.devtopics.com/20-great-programming-quotes/)
Remember, each solution has its own strengths and weaknesses, and the right approach usually falls upon the specific requirements of your project. Also, these techniques hold up regardless of whether you’re using plain JavaScript or jQuery since jQuery is essentially a library built on top of JavaScript.
In-depth Study of Character Verification in Javascript and jQuery
In JavaScript and jQuery, character verification involves the systematic process of determining the presence, or lack thereof, of specific characters within a string. These operations lie at the heart of input validation, data manipulation, and even search functionality.
String Verification in JavaScript
JavaScript provides multiple ways to check if a string contains any letter:
- Handy String methods like
indexOf()
,
includes()
, and
search()
- The crucially important Regular Expression (regex) method, known as
test()
To validate whether a string contains any letter with the
indexOf()
or
includes()
methods, you’d need to iterate through each letter of the alphabet, checking the string against each letter. However, the smarter approach is to utilize the power of regular expressions via the
test()
method.
An implementation of this would be:
const str = "123456"; const regex = /[a-zA-Z]/; console.log(regex.test(str)); //Returns false as there are no letters
This code snippet utilises a nifty regular expression that searches for any occurrence of the English alphabet’s letters, whether lowercase or uppercase, within a given string.
Character Verification in jQuery
As for character validation using jQuery, you should know that jQuery doesn’t provide direct methods for string verification unlike JavaScript. Instead, it’s common to use jQuery alongside built-in JavaScript methods for this purpose. Here’s how you do it in a jQuery environment:
let str = $('#inputField').val(); let regex = /[a-zA-Z]/; if(regex.test(str)){ console.log("The string contains a letter"); }else{ console.log("The string doesn't contain any letters"); }
In this code, ‘#inputField’ is the id of a hypothetical input field. We retrieve its value and store it in
str
. A Regular Expression is created utilizing JavaScript’s built-in capabilities, allowing us to scan for both lowercase and uppercase letters in the English alphabet. The
test()
method comes into play again, telling you whether the string contains any letter.
As Bill Gates once said, “The computer was born to solve problems that did not exist before.” Both jQuery and JavaScript are tools to handle complex tasks that sprout with the evolving technological environment. Managing string inputs and checking for specific characters within them, whether it’s to validate user inputs or to search for specific keywords, is one such task that can be handled efficiently using these languages.
For further reading, please check the MDN web docs on Regular Expressions, it provides an excellent starting point for learning about character validation in JavaScript.
Throughout this exploration of string analysis with Javascript and jQuery, we’ve investigated how to ascertain if a string contains any alphabetical characters. It’s apparent that these methodologies cater well to a range of programming needs from parsing user input to analyzing vast volumes of text data.
Let’s highlight the three main techniques for checking if a string contains any letters in Javascript:
// Using Regular Expression const hasLetter = /([a-z]|[A-Z])/.test(string); // Using Array.some() function const hasLetterArr = [...string].some(char => /^[A-Z]$/i.test(char)); // Using String.prototype.search method const hasLetterSearch = string.search(/[a-zA-Z]/) !== -1;
When deciding which solution is best for a given task, several factors are noteworthy:
Performance: While regular expressions offer a clear, concise way to inspect the content of a string, they might not perform as efficiently on larger strings or datasets.
Readability: The array-based
some()
method can be more readily understood by less experienced devs or those unfamiliar with regular expressions.
Familiarity: The
search()
method uses a similar pattern to regular expressions, but may feel more intuitive to developers familiar with other languages that have a similar built-in function.
In the realm of jQuery, implementing a simple plugin to check for letter presence could use a similar regex strategy in a function:
$.fn.hasLetter = function() { return /([a-z]|[A-Z])/.test(this.val()); };
As succinctly put by tech author Marijn Haverbeke, “Programs that modify themselves can be powerful, but they’re hard to reason about,” emphasizing the constant balance programmers must employ between functionality and understandability.
Truly, using JavaScript and jQuery to analyze strings is a delicate fusion of art and science, with diverse methods offering different areas of performance strength or ease-of-use. Each method has its own merits and could be deemed the best fit depending on context and specific requirements.source