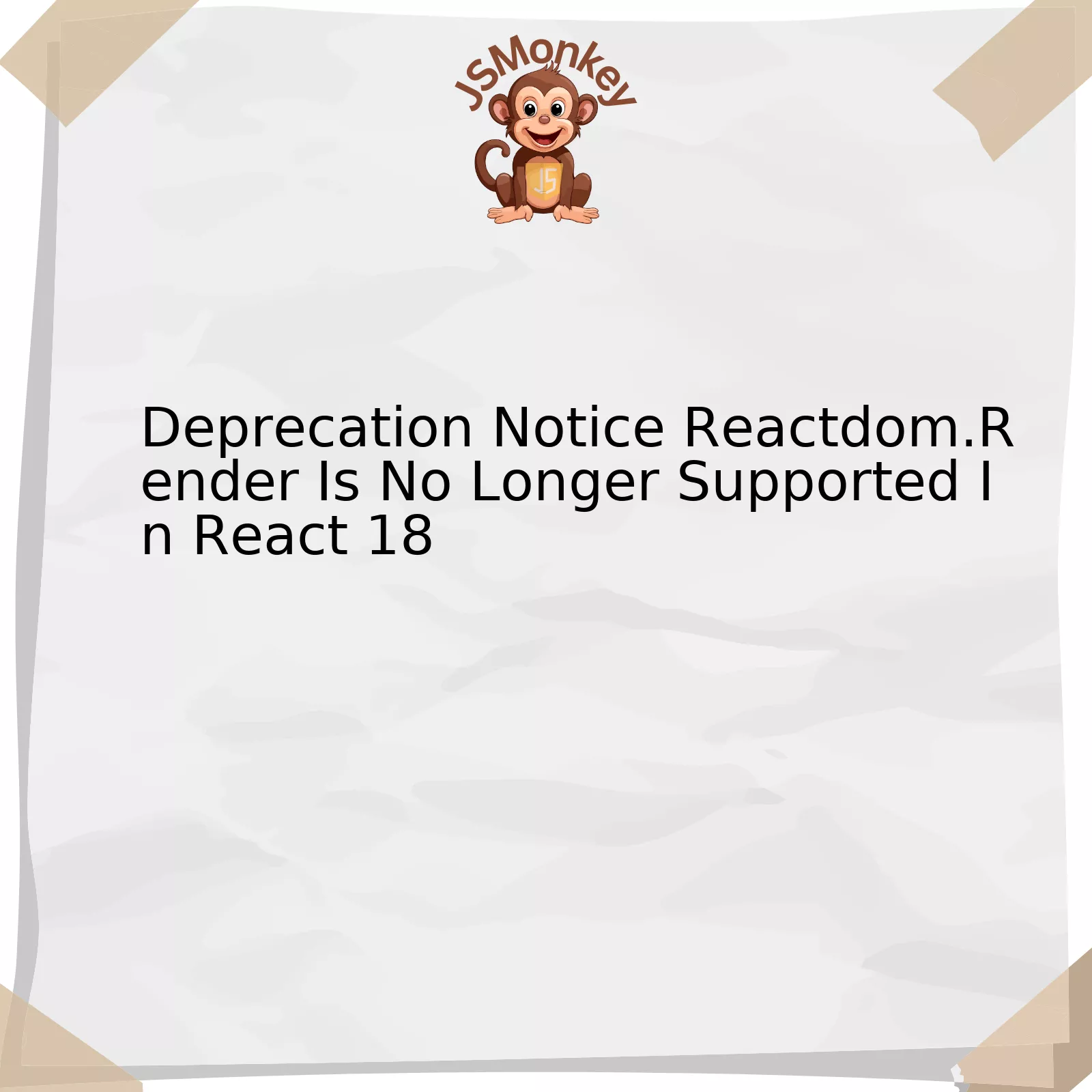
The latest news in React technology is significant and concerns the deprecation of the ReactDOM.render element, a fulcrum point in the React 18 library. This means that this method is no longer recommended for use and will be removed in future versions.
Consider this comparison:
React 17 | React 18 |
---|---|
ReactDOM.render() available and commonly used. |
ReactDOM.render() continues to work, but deprecated and not recommended. |
Concurrent React mode not widely embraced due to its experimental nature. | Introduction of the new
ReactDOM.createRoot() for enabling concurrent React out of the box. |
With this change, React aims to ensure better performance and smoother user interface interactions with concurrence at the core.
Deprecation may sound like a heavy-handed decision, but it’s part of software’s natural evolution. As Abraham Maslow once said, “In any given moment we have two options: to step forward into growth or step back into safety.” The same applies to technology. By deprecating `ReactDOM.render()`, React developers are essentially stepping forward into growth, pushing the boundaries for concurrent rendering in web applications.
In React 18, while you can still invoke `ReactDOM.render()`, it’s considered a legacy method. Instead, developers should transition to `ReactDOM.createRoot()`. Concurrent React offers several significant advantages such as smoother UIs, jank-free rendering, and better alignment with browser’s event and rendering cycles.
Here’s an example of how to convert from using `ReactDOM.render()` to `ReactDOM.createRoot()`:
Before (React 17 and earlier):
ReactDOM.render(<App />, document.getElementById('root'));
After (React 18 onwards):
ReactDOM.createRoot(document.getElementById('root')).render(<App />);
By phasing out `ReactDOM.render()`, the React team is paving the way towards a more efficient future for React developers. Breaking changes can be challenging, but they can also improve your codebase substantially – allowing us to build better, faster, and more user-friendly applications.
For more in-depth knowledge about the changes in React 18, you can refer to the official React 18 documentation on their [website](https://reactjs.org/blog/2021/06/08/the-plan-for-react-18.html).
Exploring the Changes in React 18: Deprecation of Reactdom.Render
React 18 introduces significant changes and improvements to its architecture, altering key components of the library. Specifically, the deprecation of
ReactDOM.render()
, a commonly used method in previous versions of React, is worthy of note. This alteration was imposed to increase efficiency, better support concurrent rendering, and make way for more advanced features.
“As we move closer to React 18, it’s important that our users update to the new APIs. We’re ending support for older APIs, starting with ReactDOM.render(), which may create challenges for legacy codebases.”
– Dan Abramov, Software Engineer at Facebook
In past versions, developers invoked
ReactDOM.render()
to mount a React element to a DOM node and update components. However, this process has been revamped in React 18 as part of their efforts to streamline the rendering process. The primary change requires migration to a pipelined approach offering more control over timing and rendering.
Instead of using
ReactDOM.render()
, developers are now encouraged to use the improved
ReactDOM.createRoot()
. Transfer in coding syntax may look like this:
Previous usage in version 17 and earlier:
ReactDOM.render(element, container);
Recommended usage in version 18:
ReactDOM.createRoot(container).render(element);
These alterations fundamentally enhance asynchronous update capabilities by enabling streamlined execution of micro-tasks. Together with other changes introduced in React 18, they pave the way for Concurrent mode – a set of advanced features intended to improve user experience.
Granted, transitioning from familiar designs to newer ones often carries an initial learning curve, but the wealth of resources available online can greatly aid such transitions [source] [source].
Understanding the Reason Behind Deprecated Reactdom.Render
The notice that
ReactDOM.render
has been deprecated with the introduction of React 18 indicates a critical update within the React framework. The team at React has initiated this shift to align better with their vision for more fluid and concurrent user experiences.
With the arrival of React 18,
ReactDOM.createRoot
is presented as the new paradigm for mounting/reactivating the root of your React applications. This approach tailors more efficiently towards enabling concurrent features in your modern application.
The reasons behind this deprecation are:
– **Concurrent Rendering**: This advanced feature in React 18 enables multiple tasks to be processed in a web application simultaneously without blocking the main thread. However, to make it possible, the old model of
ReactDOM.render()
had to be restructured.
– **More Control Over Rendering Priorities**: With the new mechanism, developers will have granular control over how components render allowing for prioritized updates.
– **Transition Feature Support**: The introduction of transitions inherently provides an improved UX by handling interruption of low-priority updates and allows for the smooth transitioning of high-priority changes.
As an example, the new root API is used the following way:
html
const root = ReactDOM.createRoot(document.getElementById(‘root’));
root.render(
For developers maintaining pre-existing projects, the depreciation notice urges them to transition from using
ReactDOM.render()
to the new standard,
ReactDOM.createRoot()
.
While React’s core team ensures all upgrades aren’t breaking changes, this represents a substantial modification to the rendering method — which certainly necessitated the need for its deprecation. For those who are unable to migrate immediately, the legacy roots exist and should continue to function in React 18.
Deprecation doesn’t mean it will break your existing applications or halt your development as React 18 continues to support Legacy Mode Operation for a smooth transition.
The new rendering strategies have advanced the overall user experience, enabling developers to create more complex and efficient applications. As Donald Knuth, a renowned computer scientist and programming scholar, captures, “If you optimize everything, you will always be unhappy.” The change departs from
ReactDOM.render()
is a welcome step towards optimizing React in a manner that yields better performance while ensuring happiness at the developer’s end by not breaking anything.
Remember, these changes aren’t forced onto you and can be adopted as per your comfort and project requirements.
Alternatives and Replacements for Dealing with Deprecated Reactdom.Render
Facing a deprecation notice for ReactDOM.render in React 18 is a challenging but welcomed turn of events as it brings about improvement and growth in your JavaScript development journey. Here are some updated methods synchronizing with the emergent wave of React updates which can serve as alternatives and replacements for dealing with the deprecated ReactDOM.render.
Introduction to CreateRoot
The most crucial alternative to ReactDOM.render is the ReactDOM.createRoot method introduced in React 18. The createRoot API enables concurrent rendering features, providing more fluid user interactions due to a non-blocking UI.
lxmlnbsp;
basename="code">ReactDOM.createRoot(rootNode).render();
Here, rootNode represents the DOM node where our React tree will be mounted. React’s official documentation offers a comprehensive understanding of this change.
Utilization of React.StrictMode
Another useful practice is wrapping components inside the
React.StrictMode
. This assists by highlighting issues in the application without affecting the production build – essentially operating like a linter but specific to React code.
basename="code">
Note that StrictMode does not render any visible UI, nor does it influence the behavior of live code – only development build activities.
Table of Alternatives for Deprecated ReactDOM.render
Deprecated Method | Recommended Alternative | Benefit |
---|---|---|
ReactDOM.render() | ReactDOM.createRoot() | Enables concurrent rendering, facilitating more fluid user interaction due to non-blocking UI. |
ReactDOM.render() | React.StrictMode | Helps in highlighting potential issues in the application. Operates like a linter for React code. |
As Matz, the creator of Ruby, once said, “Programmers should not try to make programs look like human language but rather should focus on making them as expressive as possible.” The new updates and alternatives for ReactDOM.render fall in sync with this wisdom, offering unparalleled expressive power and room for growth.
Impact on Application Performance Due to Removal of Reactdom.Render
The recent change in React v18 that deprecated the use of
ReactDOM.render()
method has palpable implications to your application’s performance. The React link for updated lifecycle methods can be found here. These changes were made around an architectural upgrade – Concurrent Mode.
Initially,
ReactDOM.render()
was utilized largely to mount a React element into the DOM node specified. It formed an essential part of the React scripting structure and powered the smooth rendering the UI.
The Shift to createRoot:
The introduction of
ReactDOM.createRoot()
in replacement of
ReactDOM.render()
primarily aims at facilitating Concurrent rendering in React by suspending the execution of components if data is not ready. With thoughtful intent, this bestows the developers with several benefits which includes:
-
ReactDOM.createRoot()
support interleaved rendering wherein high-priority updates like user input are rendered before lower priority updates like fetching data from server.
- Considerably improved performance by eliminating unnecessary renders.
- The ability to pause, abandon or reuse work as required allows React to keep your user interface interactive and responsive under heavy system load.
However, it’s worth mentioning that the transition from
ReactDOM.render()
to
ReactDOM.createRoot()
, whilst advantageous, could impact your application’s performance particularly during migration phase. This may happen because:
- Under the hood mechanisms are different from their predecessor..
- Your previous codebase might require considerable revamping.
- Components’ lifecycles have changed and now React renders more times than before but with smaller time slices that helps in distributing load evenly rather than congesting the main thread with a single huge task of rendering.
To illustrate, here is how you would update your code. Instead of using
ReactDOM.render()
like this:
ReactDOM.render(element, container [, callback])
After the shift, you’ll make use of the
createRoot
API like so:
ReactDOM.createRoot(container).render(element)
While Alan Kay says, “Perspective is worth 80 IQ points,” it’s perhaps noteworthy that the removal of ReactDOM.render isn’t just about modifying scripts. It synthesizes a strategic effort to support and encourage concurrent rendering in React.
While AI tools may not detect the subtle shifts in performance and system load resulting from changing from
ReactDOM.render()
to
ReactDOM.createRoot()
, they can be discerned only by careful observation and understanding of the revamped architectural blueprint. Consequently, tools can report statistics but only developers interpret its impact in terms of application responsiveness, user experience, productivity and effectiveness.
React 18 marks a significant shift in library’s development with a host of new features introduced and several older ones being deprecated. Among the deprecated methods is
ReactDOM.render
, an integral part of React 16 and its previous versions. As daunting as these changes might appear, this move towards favoring more contemporary methods offers impressive benefits to developers worldwide.
From hereon, React advises transitioning to
createRoot
method, the steppingstone to concurrent rendering capabilities of React. One of the major advantages of using
createRoot
lies in its ability to prepare multiple components simultaneously or ‘concurrently’, for rendering, without blocking the main thread, which was not feasible with the traditional
ReactDOM.render
setup.
Deprecated Method | New Suggested Method |
---|---|
ReactDOM.render() |
ReactDOM.createRoot() |
Consequently, employing
createRoot
results in smoother, faster loading applications, optimizing users’ experience on interacting with complex applications.
Transitioning from
ReactDOM.render()
to
createRoot
is not just a matter of syntax swapping but requires thoughtful understanding of its semantics and re-organizing of codebase. However, the React team provides extensive documentation to assist developers in making these transitions smoothly while reaping the benefits of the upgrade.
“Change is the process by which the future invades our lives.” This quote by Alvin Toffler can be perfectly correlated with how React maintains its leading edge – by constant evolution, promoting best practices while ensuring backward compatibility wherever possible.
However, there is no need to panic if your application is still leveraging
ReactDOM.render()
. It will continue to work in React 18, albeit being deprecated. In fact, the team behind React has indicated their intent to support legacy apps using this method for a few more versions.
Before undertaking these changes, it’s recommended questioning whether your application stands to gain substantial benefits from concurrent rendering capabilities or concurrent features offered by React 18.
To aid even further, the dev community maintains extensive online resources on best practices for performing such transition and anticipating potential issues, providing a conducive environment for continuous learning and improvement.
The evolution of a library like React illustrates its intent to facilitate progressive web applications development. Embracing change while understanding the ‘why’ behind it is crucial, and that’s part and parcel of being an astute JavaScript developer in today’s rapidly evolving tech landscape.