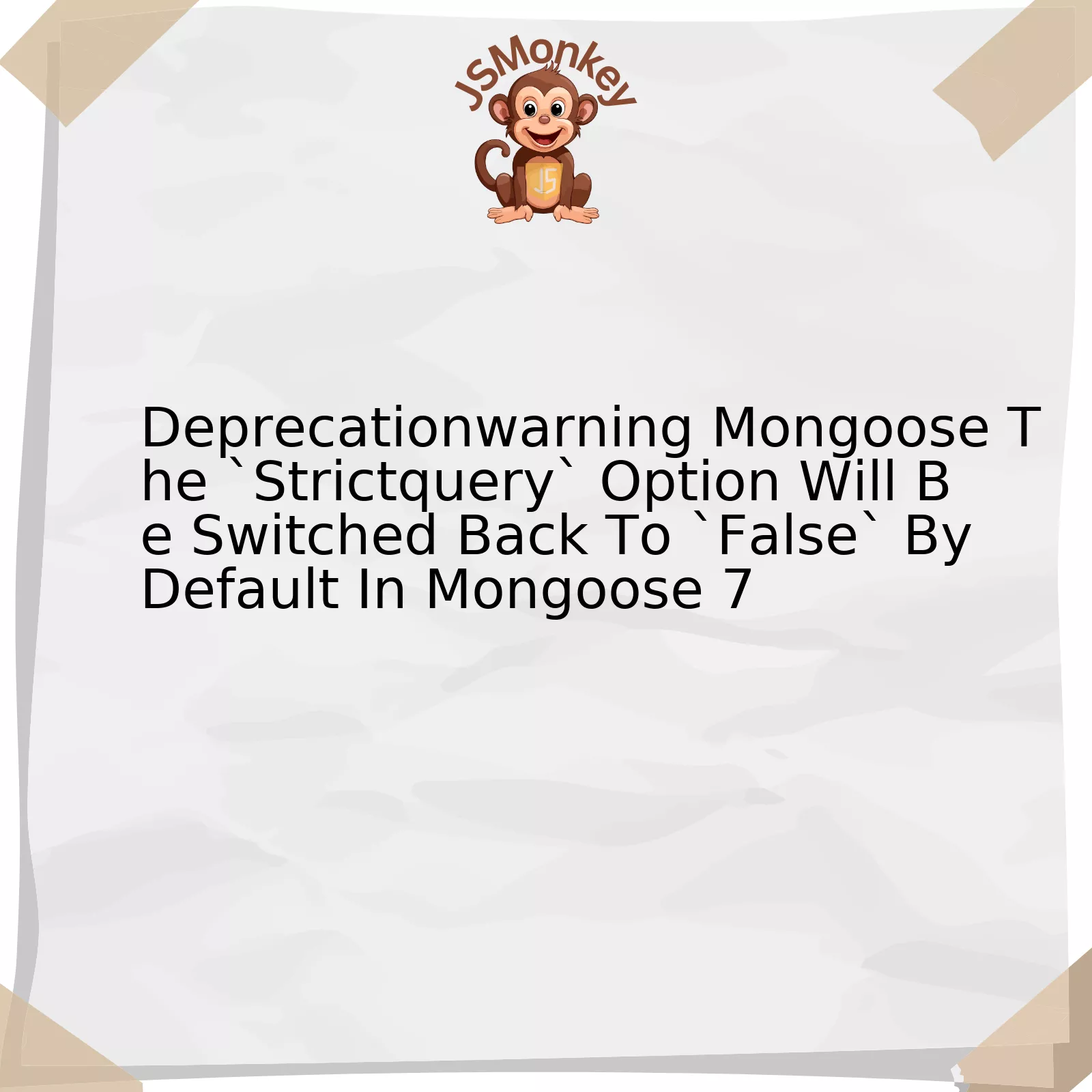
Term | Description |
---|---|
Deprecation Warning | A notice given by a software developer showing that certain features or technologies are no longer recommended for use and might become obsolete in future updates. |
Mongoose | An Object Data Modeling (ODM) library that provides a rigorous modeling environment for your data. It works on the top of Node.js and supports out of the box, MongoDB built-in features thus, providing everything needed to work with MongoDB. |
`strictQuery` option | A feature in Mongoose which when set to `true`, filters out properties that aren’t in the schema while making queries. Conversely, if set to `false`, it doesn’t filter these properties. |
Mongoose 7 | The anticipated version of Mongoose where the `strictQuery` option will be switched back to `false` as default. |
The above-mentioned organizing structure presents an overview of the terminology related to “Deprecation warning: Mongoose The `Strictquery` Option Will Be Switched Back To `False` By Default In Mongoose 7”. Now, let’s delve into these concepts.
A “Deprecation Warning” is a form of communication from the software developers to its users that a particular feature or practice is no longer recommended. This might happen due to several different reasons such as better alternatives becoming available, the deprecated feature having unresolvable issues, or it simply being outdated.
Speaking specifically about Mongoose, it’s an elegant object modelling for node.js and MongoDB that provides a schema-based solution to model your application data. Featuring built-in type casting, validation, query building, and business logic hooks, it’s a comprehensive tool for handling database operations.
One of these features or options in Mongoose is the `strictQuery` option. The purpose behind this option is to filter out queries on properties that do not exist in our database schema when set as ‘true’. But if it’s set to ‘false’, mongoose does not suppress the properties not defined in the schema and executes the query incorporating the entire request sent by the user. This could potentially lead to inefficient queries, or worse, to security vulnerabilities in your application.
Lastly, addressing the part “will be switched back to `false` by default in Mongoose 7”, implies that the developers of the Mongoose library have expressed their intent to change the default setting of the `strictQuery` option from ‘true’ to ‘false’ in the upcoming version 7 of Mongoose. This exhibit exemplifies the dynamism in software development practices where previous conventions are reassessed and reevaluated frequently.
According to Jeff Atwood, Co-founder of Stack Overflow –
“The best code is no code at all.”
The decision to alter the default setting may have been directed towards reducing the unnecessary complexity of filtering out non-schema properties for every query, thereby endorsing Jeff’s wise words.
Understanding the Impact of DeprecationWarning on Mongoose
The `DeprecationWarning` is essential to understand as it impacts how you write and update your Mongoose code. This particular warning pertains to the `strictQuery` option being switched back to `false` by default in Mongoose 7.
So firstly, let’s understand what `strictQuery` means. Within Mongoose, `strictQuery` option, when set to true, prevents undefined schema path filters from being used in a query (like findOne(), find(), countDocuments()).
When this value is switched to `false`, the developer may utilize non-defined schema path filters in their queries which Mongoose will not remove. It gives greater flexibility but at the expense of potentially compromising data integrity, with increased risk for bugs and inconsistencies.
const mongoose = require('mongoose'); const Schema = mongoose.Schema; const blogSchema = new Schema({ title: String, author: String, body: String, },{ strictQuery: false }); // Allows non-defined schema paths const Blog = mongoose.model('Blog', blogSchema);
Over time, though, the use of `strictQuery` has revealed some contradictions within Mongoose API behavior. To resolve these issues, a decision was made to switch the default status of the `strictQuery` option back to `false`. It’s important to note that the choice isn’t being removed, just its default setting is being changed in the upcoming Mongoose version.
This impacts developers using Mongoose as now they need to selectively opt for using `strictQuery: true` where necessary, otherwise, their codebase may have unexpected behavior changes or potential data integrity issues after upgrading to Mongoose 7.
However, always remember how developer Eric Elliott puts it, “Programming is largely about trade-offs” so keep this change in mind before updating to Mongoose 7. Adapt accordingly and safeguard your codes to prevent any potential issues due to this change.
For more information about Mongoose, you may always refer to the Mongoose documentation.
Navigating Changes: The Reversion to StrictQuery as Default in version 7
In the context of JavaScript development, Mongoose is an Object Data Modeling (ODM) library that provides a simplified way to interact with MongoDB. Like any active software library, updates and changes are constantly made to improve performance, add features and fix bugs. However, understanding these changes may sometimes be challenging particularly when it comes to the shift away from the `strictQuery` default in version 7.
The ‘DeprecationWarning: Mongoose strict’ update informs developers about the planned reversion of the `StrictQuery` option back to `False` by default in the upcoming Mongoose 7 release. Here’s what this means:
The `strict` setting in Mongoose has been an essential feature for safeguarding your schema definitions. When turned `true`, which has been the default setting, Mongoose will ensure the data you send for creating or updating documents adheres to your defined Mongo schemas —discarding everything else that doesn’t match.
In essence, the `strictQuery` option once set to `false` loosens this strict adherence by allowing the use of properties in queries that aren’t defined in the schema. This influences only query projections but does not change any other behavior. It essentially offers more coding flexibility.
Why is this important?
- The impending change could redefine how we approach our schema designs and data handling strategies.
- With no automatic discarding procedure, businesses dealing with vast datasets would need to ensure their data integrity manually.
- It might significantly crash applications based on the `strictQuery` true concept especially if there’s a misunderstanding of its new application.
To prepare for this, it would be advisable to start test-running your operations with `strictQuery` set to `false`. Developers can initialize it in the Mongoose connection as follows:
mongoose.connect('mongodb://localhost/test', { strictQuery: false });
Notably, as Steve Jobs once said, “Innovation distinguishes between a leader and a follower.” Therefore in technology, it is wise to adjust and adapt to these changes promptly. Updating library versions promises enhanced performance, thus staying up-to-date can significantly boost the reliability of your applications — Making them more efficient and abreast with the latest tech standards.
You can find out more about this change and its potential impact on Mongoose’s official website.
A Deep Dive into Functionality with ‘False’ StrictQuery Option
As a robust tool for interacting with MongoDB databases, Mongoose has been extensively employed by Javascript developers. One specific function that has been under the radar is the `strictQuery` option. As noticed in the deprecation warning, this function will be switched back to ‘false’ by default in the upcoming version of Mongoose 7.
Let’s dive a little deeper into its functionality and unpack the implications it holds for those using Mongoose:
- Essentially, the `strictQuery` option functions as a strict mode for queries in Mongoose.
- When enabled (set to ‘true’), it adds an additional layer of security by preventing queries on fields that aren’t explicitly defined in the schema — thereby blocking any unrecognized or unintended query parameters from passing through.
A typical use case could look like this:
// Define the schema const UserSchema = new mongoose.Schema({ name: String, email: String }, { strictQuery: true }); // Unexpected field ‘age’ will be ignored let data = await User.findById(userId).select('age');
This setting was designed with the aim to enforce better data integrity and prevent potential security issues. However, it also comes with performance implications due to the added overhead of checking against the schema definition for every query. This, coupled with potential unforeseen limitations on complex querying, led to a debate on its usefulness.
Hence, the decision was made to set its default value back to ‘false’ in the forthcoming Mongoose 7. This allows users the freedom to opt in only when required, keeping in mind the trade-off between performance and strictness.
Notably, this is both a breaking change and a subtle one – unless developers are making explicit use of this feature, they’re unlikely to even notice this shift in default functionality.
This is reminiscent of a quote from software engineer and author, Martin Fowler: “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” The decision by Mongoose to switch the default `strictQuery` option back to ‘false’ marries this wisdom with practicality and usability.
Linking to the Mongoose documentation provides an in-depth understanding of how to adjust and use the `strictQuery` function according to individual needs. Understanding its nuances will increase code efficiency and potentially prevent unwanted surprises during your data modeling journey.
It’s important to fully understand your tools in order to harness their full potential, Glenford Myers, an American software engineer, highlighted this by saying *”A good programmer does not merely debug his programs; he understands why they work.”*
While strictness might be pivotal for some, others may appreciate the flexibility granted by not enforcing it. Figuring out where you stand on this matter depends solely on your unique business logic and circumstances.
Implications and Workaround Strategies for The Switch Back in Mongoose
The implications of the `StrictQuery` option in Mongoose defaulting back to `False` primarily revolve around the latitude with which developers can institute database queries.
Firstly, it becomes important to note that with `strictQuery: false`, Mongoose will not filter out undefined field paths in query selection. In effect, this allows your application to interact with your MongoDB database even if you don’t explicitly outline the schema for certain fields.
Implications
- Increased Risk of Query Errors: With the newfound ability to interact with undefined schema fields, the risk of undocumented modifications increases. If a developer isn’t careful, they might end up querying fields they didn’t intend to, potentially leading to erroneous results and data inconsistencies.source
- Inaccurate Reporting and Misinterpretation: The lack of query validation opens up the possibility of inaccurate reporting due to unvalidated or unmatched query parameters.
- Security Concerns: Not applying strict schemas can expose the database to potential threats owing to broader query parameters that go unchecked. This makes deliberate or accidental misuse easier.
Workaround Strategies
- Explicitly Set Option to `True`: One workaround strategy is to explicitly set the `strictQuery` option to `true`. This forces Mongoose to ignore any query parameters that don’t match up with defined schemas, and provides an extra layer against inadvertent querying errors.
- Defined Schema: Make sure all desired fields are included in your schema definition to alleviate dependency on the `strictQuery` setting. This ensures full control over what fields can be queried and reduces the risks associated with an open schema.source
- Runtime Warnings: Implement a functionality that raises warnings during development runtime whenever an undefined field is being queried, encouraging developers to keep their schemas updated and maintained.
The quote by Rob Pike, “A little copying is better than a little dependency.” resonates well with this situation. Instead of depending on the `strictQuery` option setting and dealing with potential issues it might bring forth, the ideal solution encourages us to depend more on our explicit schema definitions. This reduces errors, enhances security, and makes our databases more predictable and easier to manage. It requires more effort in the beginning but pays off in long-term application stability.
// Explicitly set strictQuery to true var connection = mongoose.createConnection("mongodb://localhost/test", { "config": {"autoIndex": true }, "userNewUrlParser": true, "useUnifiedTopology": true, "useCreateIndex": true, "useFindAndModify": false, "serverSelectionTimeoutMS": 30000, // Keep trying to send operations for 15 seconds "socketTimeoutMS": 45000, // Close sockets after 30 seconds of inactivity "family": 4, // Use IPv4, skip trying IPv6 "strictQuery": true });
Highlighting the technical dynamics surrounding the
DeprecationWarning
from Mongoose, it’s valuable to glean some pivotal insights. The warning message pertains to the behavior of the `strictQuery` option that will automatically revert to `false` by default in the forthcoming Mongoose 7.
In its formative iterations, Mongoose adopted a `strict` schema configuration philosophy. This provided an added layer of data integrity and minimized the likelihood of unwittingly writing errant data to your MongoDB database. Under this regime, any fields not defined explicitly in the schema model would be disregarded outright when saving a document.
However, one must heed the harbinger presented by the `DeprecationWarning`. Come Mongoose version 7, this `strictQuery` option will be automatically set to `false` unless decreed otherwise by developers. In essence, spurring more leniency on query filters which don’t correspond with schema paths. Accordingly, if you were to assign an object with extraneous properties as a search parameter in `findOne` or `find`, Mongoose will no longer dismiss those properties, but instead, include them in the ensuing MongoDB query.
Considerable caution should be exercise while managing this paradigm shift. Shifting towards laxness could fundamentally impact application behaviors and potentially introduce unforeseen bugs. Therefore, it’s advised for developers to purposefully specify the `strictQuery` setting as per the needs of their application rather than relying on defaults.
As Brandon Sanderson well said: “The mark of a great man is one who knows when to set aside the important things in order to accomplish the vital ones.”
Mongoose Version | `strictQuery` default |
---|---|
Pre-7 | true |
7+ | false |
The involution of Mongoose’s `strictQuery` doctrine illustrates a change in software’s life – a metamorphosis grounded on insightful feedback, experiences learned, and strides in the greater JavaScript ecosystem.
For further reading, visit the Mongoose`s official documentation on ‘Strict Mode’ here.
Remember, “The only thing that is constant is change” (Heraclitus of Ephesus). As it stands true for the evolution of programming libraries, keep pace with these changes to thrive in your development journey.