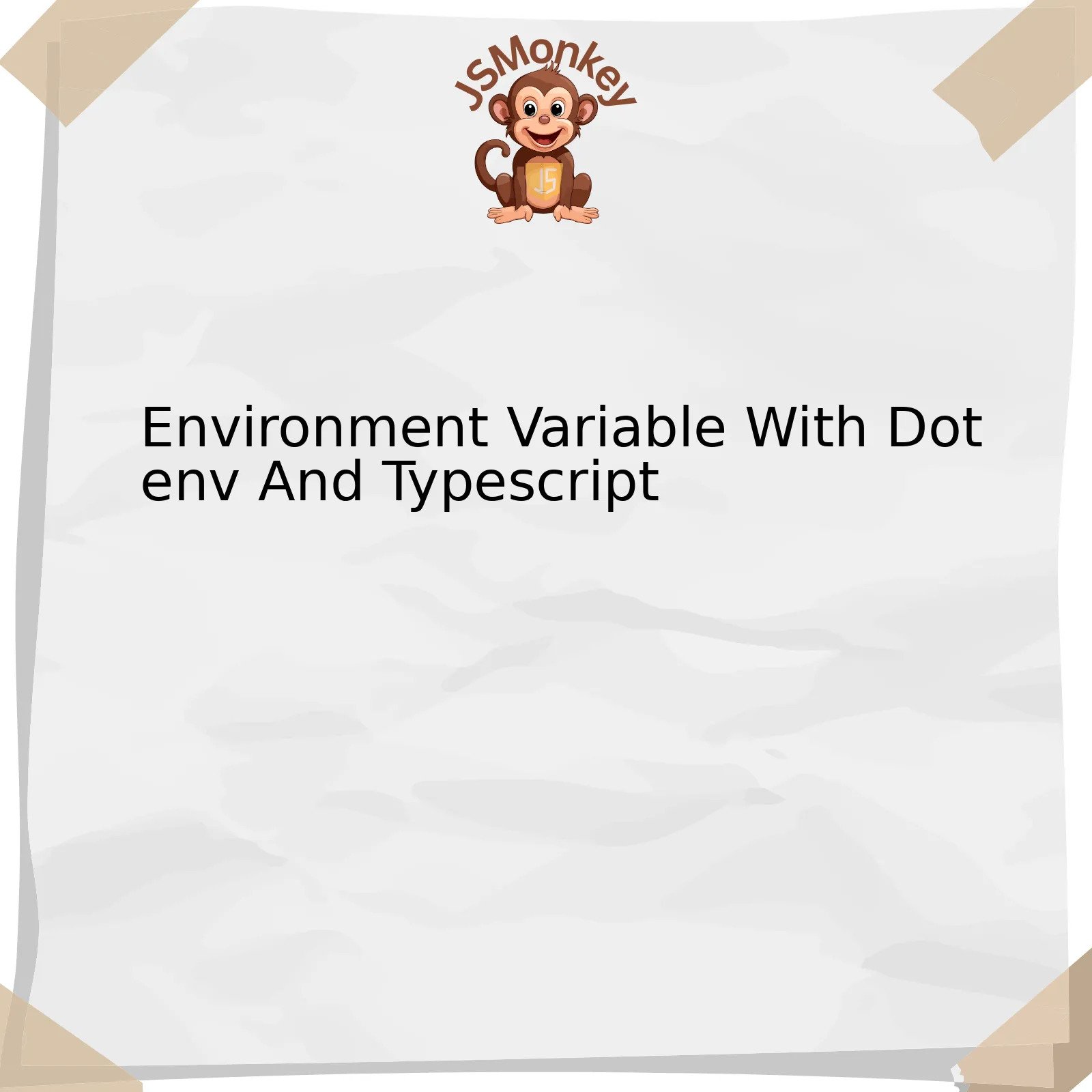
Utilizing environment variables is a vital aspect in the creation of scalable applications, and when dealing with Node.js projects, this can be achieved optimally using dotenv along with TypeScript. Let’s bring into focus their interaction and usage:
Components | Description |
---|---|
Environment Variables |
These are external factors that define behavior within an application. They allow for flexibility, giving developers the ability to tailor the application’s functions based on various conditions. |
dotenv |
A zero-dependency module in node.js used for loading environment variables from a .env file into process.env. It is extremely advantageous in creating distinct environments for development, testing, and production. |
TypeScript |
A statically compiled language to write clear and simple JavaScript code. It enables Type checking which ensures that the values we assign to our variables or pass as arguments in our program behave exactly as expected. |
The conjunction of these three elements creates a highly flexible and productive environment for JavaScript developing.
Let’s delve into the way these elements work together. After installing the dotenv package (`npm install dotenv –save`) and importing it into your TypeScript file, you may then call `dotenv.config()` at the start of your application. In effect, this method reads a `.env` file from your project root, parsing the contents into `process.env`. Therefore, named exports could be created directly from `process.env`.
For instance:
import 'dotenv/config'; const POSTGRES_USER: string = process.env.POSTGRES_USER; const POSTGRES_PASSWORD: string = process.env.POSTGRES_PASSWORD;
In the above snippet, POSTGRES_USER and POSTGRES_PASSWORD are environment variables stored in a .env file. This approach cultivates better security measures within your application and strengthens the systematic management of data.
To quote David Walsh, renowned web developer and creator of the popular blog davidwalsh.name, “Configuring project environments is one of the first tasks to tackle when creating new software applications.”
Importantly, while working with TypeScript and environment variables, it can be beneficial to create a type declaration for `process.env`. It helps in autocompletion and safeguards against mistakes related to type.
declare global { namespace NodeJS { interface ProcessEnv { POSTGRES_USER: string; POSTGRES_PASSWORD: string; } } }
With this integrated pattern of environment management using dotenv and TypeScript, there’s an enhancement in productivity; your code becomes more structured, readable, easier to reason about, and ultimately, more maintainable.
Understanding the Role of Environment Variables in TypeScript
Environment variables are an essential part of modern application development. They give us the ability to configure and modify the behavior of our software at runtime without changing the source code itself. In TypeScript, a superset of JavaScript, we manage these variables similarly.
An environment variable contains configuration information for command-line interfaces and applications running within them. By convention, they are usually uppercase with words separated by underscores – for instance,
ENV_VARIABLE_NAME
. For diverse reasons such as enhancing the security, regulating the configuration management, ensuring scalability, we employ environment variables in TypeScript.
However, managing these variables efficiently can be complex to handle manually, especially when dealing with different servers or stages like production, staging, and development which potentially requires distinctive configuration settings. This is where dotenv comes into play.
This open-source module, i.e., [dotenv](https://www.npmjs.com/package/dotenv), makes handling environment variables in a Node.js project proficiently. To use dotenv in your Typescript project, it has to be installed along with its types package as development dependency via npm:
npm install dotenv @types/dotenv --save-dev
Dotenv streamlines the process of integrating environment variables by allowing developers to specify them inside a file (generally named `.env`), hence protecting sensitive data like API keys or database credentials.
Once installed, all you need to do is load the dotenv config into your script. Typically, this would be in your main server file:
import dotenv from 'dotenv'; dotenv.config();
Afterward, the environment variables declared in the .env file should be accessible through
process.env.YOUR_VARIABLE_NAME
.
Before deploying the application, ensure that the .env file is added to the .gitignore file because we don’t want our configs holding sensitive info exposed in VCS ([version control system](https://www.atlassian.com/git/tutorials/what-is-version-control)).
We must not ignore the importance of types in TypeScript. To get benefits from typings with dotenv, declare a type for your environment variables to avoid errors. Create an `environment.d.ts` file and place your variables inside it:
declare global { namespace NodeJS { interface ProcessEnv { NODE_ENV: 'development' | 'production'; API_KEY: string; } } }
Now, you have full intellisense support when accessing these environment variables.
As [Robert C. Martin](https://twitter.com/unclebobmartin) puts it “It’s not at all important to get it right the first time. It’s vitally important to get it right the last time.” The .env feature aids in getting configurations right for diverse environments without changing codebase frequently thereby reducing chances of errors.
Leveraging Dotenv for Efficient Configuration Management
Leveraging dotenv for Efficient Configuration Management in a TypeScript Environment
Growing ever popular in JavaScript development, dotenv is an excellent package offering developers a streamlined method for managing application configuration. Combining the power of dotenv with TypeScript allows one to harness strong typing benefits in tandem with environment variable management.
When developing scalable applications which need to work across different environments, using environment variables is paramount. These environment-specific configurations allow your application to adapt its behavior accordingly. Factors such as database credentials or API keys will often differ substantially between environments, and dotenv provides a medium to manage this efficiently without harming your code’s directness.
The primary focus here is to make use of dotenv in a TypeScript setting, which involves a few simple steps that are easy to follow:
1. Begin by installing the dotenv package from npm.
2. Create a “.env” file in your root directory containing your environment variables. Remember not to include sensitive information in the file; your .gitignore should always exclude it.
3. Utilize the dotenv package in your application to parse these variables and make them available.
Each step can be executed as follows:
// Step 1: Install dotenv npm install dotenv // Step 2: Add some environment variables in your .env file DB_HOST=localhost DB_USER=root DB_PASS=s1mpl3 // Step 3: In your application: import dotenv from 'dotenv'; dotenv.config(); console.log(process.env.DB_HOST); // Should print "localhost"
To craft an efficient and fluid system, it may be beneficial to create separate environment files like `.env.development` or `.env.production`. During runtime, the correct file can then be loaded based on the NODE_ENV value.
Although dotenv + Typescript operates seamlessly, leveraging type safety can provide the extra layer of reliability. A simple method would be to create a configuration object that accesses environment variables and associates them with TypeScript types:
// config.ts import dotenv from 'dotenv'; dotenv.config(); export const config = { dbHost: process.env.DB_HOST as string, dbUser: process.env.DB_USER as string, dbPass: process.env.DB_PASS as string, };
In this way, advantages from both dotenv and TypeScript are concurrently exploited, giving you an effective and robust configuration management system.
As Robert C. Martin, author of “Clean Code,” said:
>”A system with good architecture is easier to modify, easier to understand, easier to ensure, more robust and more cost-effective.”
One could say that utilizing dotenv in a TypeScript context for managing configurations is certainly a step towards building that good architecture.
Effective Techniques to Link Dotenv and TypeScript
Effective techniques to link Dotenv and TypeScript mainly revolves around the process of managing environment variables. Environment variables are essentially ‘global’ variables specific to your operating system. They occasionally contain sensitive information, such as API keys, passwords, etc. When using Dotenv with TypeScript, these environment variables can be effectively managed and applied as needed.
1. Installation of Dotenv
First and foremost, you should ensure that Dotenv is installed in your application.
npm install dotenv
2. Initializing Dotenv
To initialize Dotenv, you’d import the module into your TypeScript file just like any other npm package:
import * as dotenv from "dotenv"; dotenv.config();
With this block of code in place, Dotenv’s `config()` function will read your `.env` file, parse the contents, assign it to `process.env`, and make them available throughout your TypeScript application.
3. Creation and Usage of .env File
Inside the .env file, you’d typically define your environment variables in the form of `NAME=VALUE`. Each variable should be on a separate line. For instance:
DB_HOST=localhost DB_USER=root DB_PASS=password123!
After initializing Dotenv, these variables defined in the .env file can be accessed through `process.env` object within your TypeScript application. For instance,
const host = process.env.DB_HOST; const user = process.env.DB_USER;
4. Type Definitions for Environment Variables
In TypeScript, we often have to explicitly define the types of variables. To leverage TypeScript’s static type checking, a common practice is to create a custom type definition for the process.env object. This can be achieved by creating a `@types` directory at the root of your project.
Create an `env.d.ts` file in the `@types` directory and express the expected variables like so:
declare namespace NodeJS { interface ProcessEnv { NODE_ENV: 'development' | 'production'; DB_HOST: string; DB_USER: string; DB_PASS: string; } }
Quote: “First, solve the problem, then write the code.” – John Johnson
By following these aforementioned techniques, you can effectively manage environment variables using Dotenv in your TypeScript application. However, remember to always exclude your .env files from version control systems to prevent exposing sensitive data.
Best Practices in Managing Environment Variables with Dotenv & TypeScript
When it comes to managing environment variables effectively in a TypeScript project, a best practice approach involves the use of the Dotenv library. This library is designed and built to load variables from an `.env` file into `process.env`.
The Dotenv library can prove notably beneficial in several ways:
- It isolates the configuration, providing consistency across all stages of development.
- It promotes clean code principles by separating configuration from application logic.
- It helps keep sensitive data such as database passwords or API keys secure by preventing them from being hard-coded into the program.
Here’s an illustration of an effective way to utilize Dotenv with TypeScript:
// Import the env config import dotenv from 'dotenv'; // Init the dotenv dotenv.config();
Following this initialization, you can access values via `process.env.YOUR_VARIABLE`.
However, there are a few key things to note when using Dotenv and TypeScript together:
– Types need to be explicitly set for environmental variables to work with TypeScript.
– The process can be streamlined by creating type definitions for your environment variables.
For instance, one could define types like so:
interface ProcessEnv { [key: string]: string | undefined } declare namespace NodeJS { export interface Process { env: ProcessEnv } }
In the spirit of renowned technology consultant Martin Fowler’s assertion that “Any fool can write code that a computer can understand. Good programmers write code that humans can understand,” clear organization and consistent methodology in handling environment variables will undoubtedly facilitate easier understanding and management by developers on the project.
It’s worth mentioning that maintaining .env files securely is crucial. These files should never be pushed to repositories, particularly public ones. To avoid accidental pushes, it’s a common practice to add `.env` files to `.gitignore`.
The utilization of Dotenv with TypeScript in the aforementioned way contributes to a clean, secure, and efficient approach to managing environment variables. These practices can greatly aid developers in effectively organizing their projects while ensuring code confidentiality.
Reference:
– Dotenv on NPM
– How to read env variable in Javascript – Stack Overflow
– Development Environment with Docker and TypeScript
Utilizing environment variables in TypeScript with the help of dotenv offers a secure and efficient approach towards managing configurations within your application. The dotenv module is a sterling tool designed particularly for this role. Its primary function enriches your project by loading variables from a .env file into `process.env`.
Placed at the root of a project, the .env file provides a reliable storage space for sensitive data such as API keys, passwords, or any other piece of information you would not wish to expose publically on platforms like GitHub.
To leverage the advantageous features of dotenv in Typescript, adaptation via definition files (like @types/dotenv) becomes necessary because dotenv natively does not support Typescript. You install this type definition alongside dotenv which bolsters it with Typecripts static type-checking ability, allowing developers to access environment variables seamlessly in their codebase.
Here’s an example:
// Importing the necessary modules import * as dotenv from "dotenv"; // Invoking the dotenv configuration dotenv.config(); console.log(process.env.APP_PORT); // This will print the value of APP_PORT from .env file.
In significant projects, you’d likely have separate .env files for different environments (development, production, etc.). This setup ensures each environment runs smoothly with its unique set of environment variables.
As Orinami Olatunji put it “Communicating with the environment in a Node.js application helps the app to be smarter and dynamic.”. This interaction proves instrumental in creating scalable applications characterized by strong performance metrics.
In the grand scheme of things, the ideal combination of dotenv and TypeScript serves as a valuable toolkit for both administrators and developers alike, positioning them to achieve better security, code maintainability, and overall scalability within their application development needs.