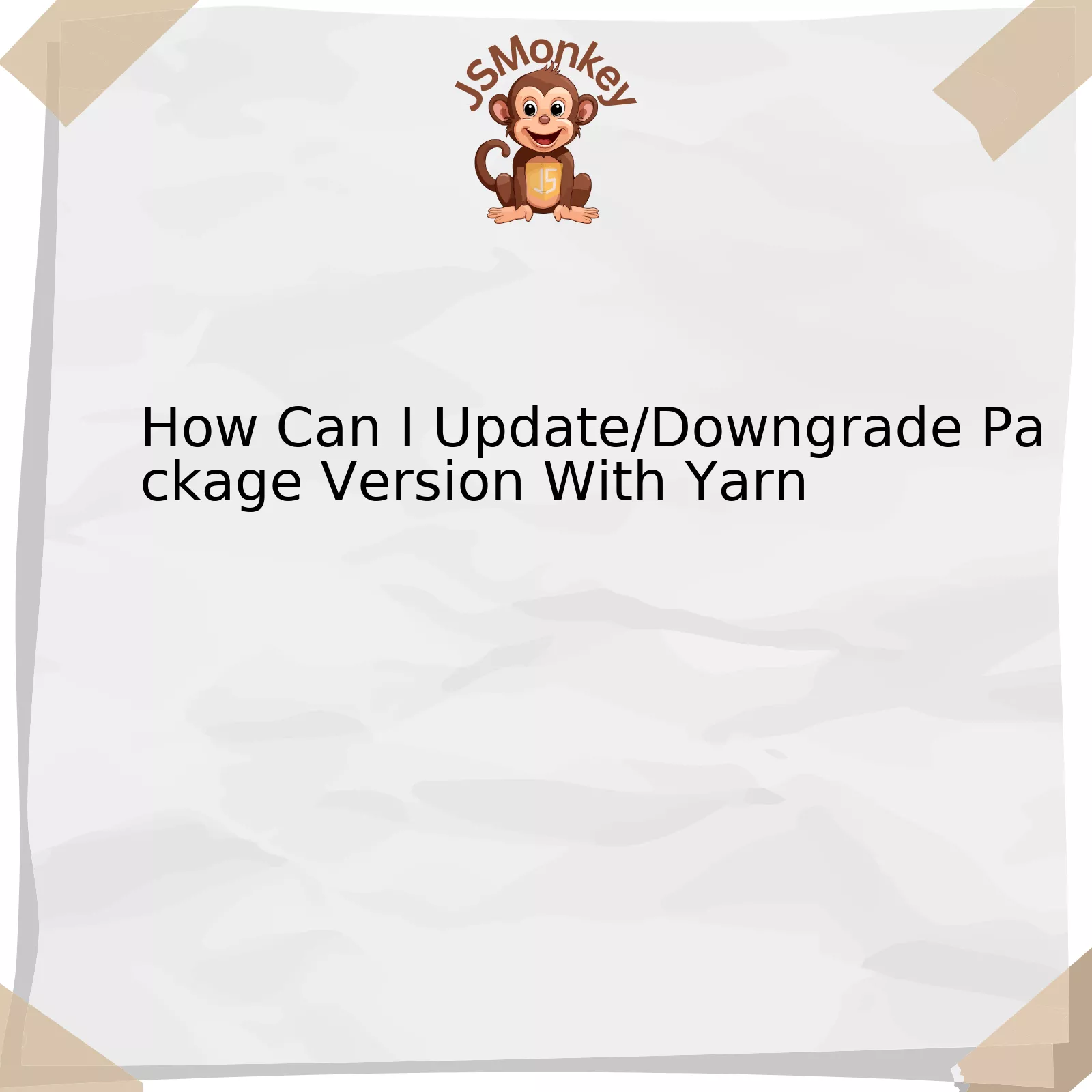
Command | Description |
---|---|
yarn upgrade [package] |
This command upgrades a package to its latest version. |
yarn upgrade [package]@[version] |
This command upgrades or downgrades a package to a specific version. |
yarn upgrade --latest [package] |
This command forcefully upgrades a package to its latest version even if it’s considered a major update. |
The table above provides commands that’ll assist in updating or downgrading the package version with Yarn.
Yarn is a highly reliable, fast, and secure dependency management system used by JavaScript developers. Upgrading and downgrading packages with Yarn can often be seen as a standard part of any development process. The reason behind package updates lies in the addition of new functionalities, improving performance, as well as fixing bugs or security vulnerabilities identified in previous versions.
On the other hand, you might need to downgrade a package version due to incompatibilities, problematic updates, or because the latest iteration doesn’t cater to your needs. In all cases, it’s essential to understand how to manipulate package versions using Yarn correctly.
The ‘
yarn upgrade [package]
‘ command updates the specified package to its latest version. This process works within the semver rules, abiding by the versioning declared in your “package.json” file’s dependencies. If you aim for a more targeted approach and require a specific version only, then make use of the ‘
yarn upgrade [package]@[version]
‘ command. This option will update or downgrade your package to the selected version, granting you better control over your project’s components.
In some situations, you might require an update to the latest iteration regardless of its impact on other aspects of your application. The demand for such a function brings about the ‘
yarn upgrade --latest [package]
‘ command, most useful in facilitating major updates. However, it’s prudent to consider the implications and volatile nature of this action carefully.
“Remember that simplicity, readability and explicitness should be the landmarks of your code, not just adding the latest packages because they are trendy”, Tomislav Bacinger, JavaScript Developer and Author.
This highlights the fact that while managing package versions is crucial, the primary aim should always be to write simple, readable and explicit code. Upgrading or downgrading packages should only serve to preserve or enhance these qualities.Reference
Understanding Yarn Package Management
Yarn is a package manager for your JavaScript programming endeavors, that wraps up your JavaScript code into manageable packages, establishes dependencies (the various tools, servers and system tools required), and creates easy-to-follow versions of your code. Essentially, it provides what JavaScript developers need in order to maintain control over their project.
Why Yarn?
“Package management is one of the important aspects of an application’s infrastructure. It makes a developer’s life easier.”
– Bilgin Ibryam, Red Hat Developer
Yarn has been lauded for its efficient cache mechanism, selective version resolution feature, and flat mode installation capabilities among other benefits.
Managing Package Updates with Yarn
Understanding how to update or downgrade packages using Yarn is crucial:
• To upgrade any package in your project with Yarn:
Navigate to your project’s root directory and use the following command:
yarn upgrade [package_name]@[version_number]
Should your aim be to keep track of the latest version, integrate
--latest
flag in your command:
yarn upgrade [package_name] --latest
This command updates a single package in the listed dependencies and modifies ‘yarn.lock’ file along with ‘package.json’ files in the current working directory.
• If you want to downgrade any package in your project:
The procedure to go about this isn’t very different from an upgrade:
yarn add [package_name]@[version_number]
By specifying the version number, you can both upgrade or downgrade a package.
Remember, these operations can affect shared assets and projects, especially when there are multiple programmers collaborating on a shared project. So, always communicate with your team whenever you’re planning to make major modifications to a package.
Documentation details for updating or downgrading packages can also be found on Yarn’s official documentation
Moving Forward with Yarn
Pragmatic package management will assist you in managing complex projects, making them more controllable and maintainable. In conclusion, a firm understanding of how to use Yarn will enhance your software development process by allowing you to efficiently manage dependencies and packages.
Leveraging Yarn for Efficient Package Versioning
Leveraging Yarn (Yet Another Resource Negotiator) for efficient package versioning is a practical implementation for JavaScript developers. It’s designed to aid in the management of NPM dependencies by offering features makeup for certain drawbacks of npm. A specific area where Yarn shines is in updating or downgrading package versions.
When looking at how you can update or downgrade package versions with Yarn, there are essentially two primary methods:
1. Immediate Updates through
yarn upgrade package-name
Command: For minor updates, the immediate mode gives a swift option. You can run the command ‘
yarn upgrade package-name
‘, replacing ‘package-name’ with your specific package name. This automatically upgrades the named package to the latest version. While handy for incremental updates that don’t alter core functionality significantly, one has to consider that this method can potentially introduce breaking changes since it doesn’t discriminate against types of upgrades.
2. Manual Changes via
package.json
followed by ‘
yarn install
‘: If you need to rollback a package version, this approach affords more control over the process. Alter the version number directly in your
package.json
file, then run ‘
yarn install
‘ to sync up your local environment. This manual change method has the added benefit of allowing for specific version number updates or downgrades, rather than simply jumping to the latest one.
Yarn Commands | Uses |
yarn upgrade package-name |
Update to the latest version |
yarn add package-name@version-num |
Add specified version of a package |
yarn remove package-name |
Remove installed package |
On the topic of version control and leveraging tools such as Yarn, software engineer, Nils Frahm once stated: “Programming is not about typing, it’s about thinking”. It emphasizes the need to give serious thought when making updates or downgrades- considering the implications for the entire codebase.
For an extensive look at yarn commands, see the official Yarn Classic CLI documentation.
. Using Yarn efficiently facilitates seamless transitions between different versions of packages, while reducing potential complications that could arise during these processes.
Note: These strategies only alter your local development environment. If you’re part of a team, ensure to communicate regarding changes as everyone should be on the same page with respective to package versions.
Steps to Update Package Versions using Yarn
Updating or downgrading your package version with Yarn can be achieved through the following steps. Do note that accurate and clear documentation is considered the backbone of effective coding, making it possible to understand the entire process and mitigate any potential hitches.
Step 1: Identify outdated packages
Choosing to use the Yarn outdated command helps developers identify modules that are currently out-of-date. The said command also provides comprehensive details about the installed versions, latest versions, along with the respective package type.
yarn outdated
Running the command above displays a user-friendly table showing:
– Package name
– Current version
– Wanted version
– Latest version
Step 2: Upgrading the packages
The yarn upgrade command is often utilized when developers wish to update all dependencies to their latest version.
yarn upgrade
Though useful, this command does not update the packages listed in the ‘devDependencies’ of your package.json file. To do so, you’ll have to run another command:
yarn upgrade --latest
Step 3: Update a specific package
Individual package upgrade or downgrade demands you specify the package’s name. Neither npm nor yarn deconstructs the semantic version for these packages. Therefore, an explicit version number must be included as shown below:
yarn upgrade@
This command will change the version number in your package.json file.
Despite its simplicity in terms of application, these steps enable JavaScript developers to update and manage their packages effectively, creating a more efficient and responsive process. As Edsger W. Dijkstra famously stated, “If debugging is the process of removing software bugs, then programming must be the process of putting them in.” With Yarn, the possibility of introducing bugs is significantly reduced due to its rich toolkit for package management. (Yarn documentation).
When considering best practices in modern web development, it’s vital to frequently contrast your application dependencies for updates. Keeping packages updated ensures your applications are secure and stable by patching possible security vulnerabilities and bugs, and exposes the latest features that come with newer versions of the library or framework.
Guidelines for Downgrading Package Versions with Yarn
The Yarn package manager, excellent for managing JavaScript dependencies, affords developers wide latitude when it comes to updating or downgrading package versions. This versatility is of necessity in scenarios where newer or older versions of libraries and frameworks may deliver better performance or compatibility with existing software ecosystems.
When seeking to downgrade a package version with Yarn, heed the directives delineated below:
• Procure the version information of available packages: Get acquainted with the range of available versions by running
yarn info [package]
. Available package versions will be enumerated under the “versions” property
• Downgrade to the desired version: With the knowledge of available versions, proceed to the actual downgrade by executing
yarn upgrade [package]@[version]
, replacing [package] with the name of the package needing a downgrade, and [version] with the specific target version.
Markedly, this is thrillingly reminiscent of Lincoln Baxter’s profound sentiment regarding adaptation in the technological realm: “Don’t reinvent the wheel, just realign it.” This notion rings particularly true when deploying older package versions may engender improved alignment with existing code.
On instances demanding an upgrade, follow these steps:
• Upgrade to the latest version: Command Yarn to effectuate an upgrade by entering
yarn upgrade [package]
; the package manager would then orchestrate an upgrade to the latest stable release. Alternatively, deploy
yarn upgrade [package]@latest
to achieve similar results.
• Upgrade to a specified version: When there’s need to advance to a specific package version, entrar
yarn upgrade [package]@[version]
into your terminal. Replace [package] and [version] with the package name and the preferred version respectively.
A keen observation would reveal that both upgradation and downgradation processes utilize the same command syntax—
yarn upgrade [package]@[version]
—, with alterations in the specifics of the package and version. This instance is a testament to the efficacy and adaptability of Yarn as a package manager, aligning seamlessly with Baxter’s philosophy on tech adaptation.
For more information regarding Yarn commands and usage, please refer to the Yarn documentation.
To upgrade or downgrade any package version using Yarn, it’s as simple as knowing the specific command lines to punch into your interface. Be guided by these following steps:
First off, you require an understanding of the package.json file in your application. This is an exceptional tool that keeps track of your project dependencies. When you add a new package with Yarn, the changes are reflected here.
yarn add [package]
This syntax allows you to install a new package while simultaneously adding the details to your package.json file. It’s important to not overlook this attribute.
But there are those times when you might need to change the version of your package. To achieve that, you’ll first need to understand yarn’s versioning rules.
Yarn would normally abide by the rules set for semantic versioning — a set of guidelines that define how version numbers are assigned. Here, each version has three parts: MAJOR.MINOR.PATCH. These are incremented based on whether there were any breaking changes, minor changes, or patches (bug fixes), respectively.
Semantic Versioning is a crucial concept that prevents your project from breaking because of changes in dependency versions.
Updating your package can be done simply and straightforwardly. You will need to use:
yarn upgrade [package]
This line of code will update your package to its latest version.
There are moments, though, when you might require downgrading your package version. That can be achieved by the install command while giving the specific version
yarn add [package]@[version]
Here’s a live example – say you want to downgrade your express package from v5.0.1 to v4.0.1. Here’s what you should do:
yarn add express@4.0.1
In a nutshell, updating or downgrading your package version with Yarn revolves around the semantic versioning rules. By mastering this rule-set, you’re ascertaining fewer issues in your project due to dependencies.
As Brian Kernighan famously said, “Controlling complexity is the essence of computer programming”. This rings particularly true when dealing with package management and version controls – your task as a JavaScript developer is not just solving problems, but managing complexities. Therefore mastering tools such as Yarn is essential for successful coding practices.
Lastly, it is fundamental to appreciate that the ‘upgrade’ or ‘add’ commands don’t affect all packages in your project by default, rather only those specified. To update/downgrade all packages at once, using ‘*’ wildcard character would be required.
yarn upgrade *
This command prompts Yarn to automatically update every single package in your project.