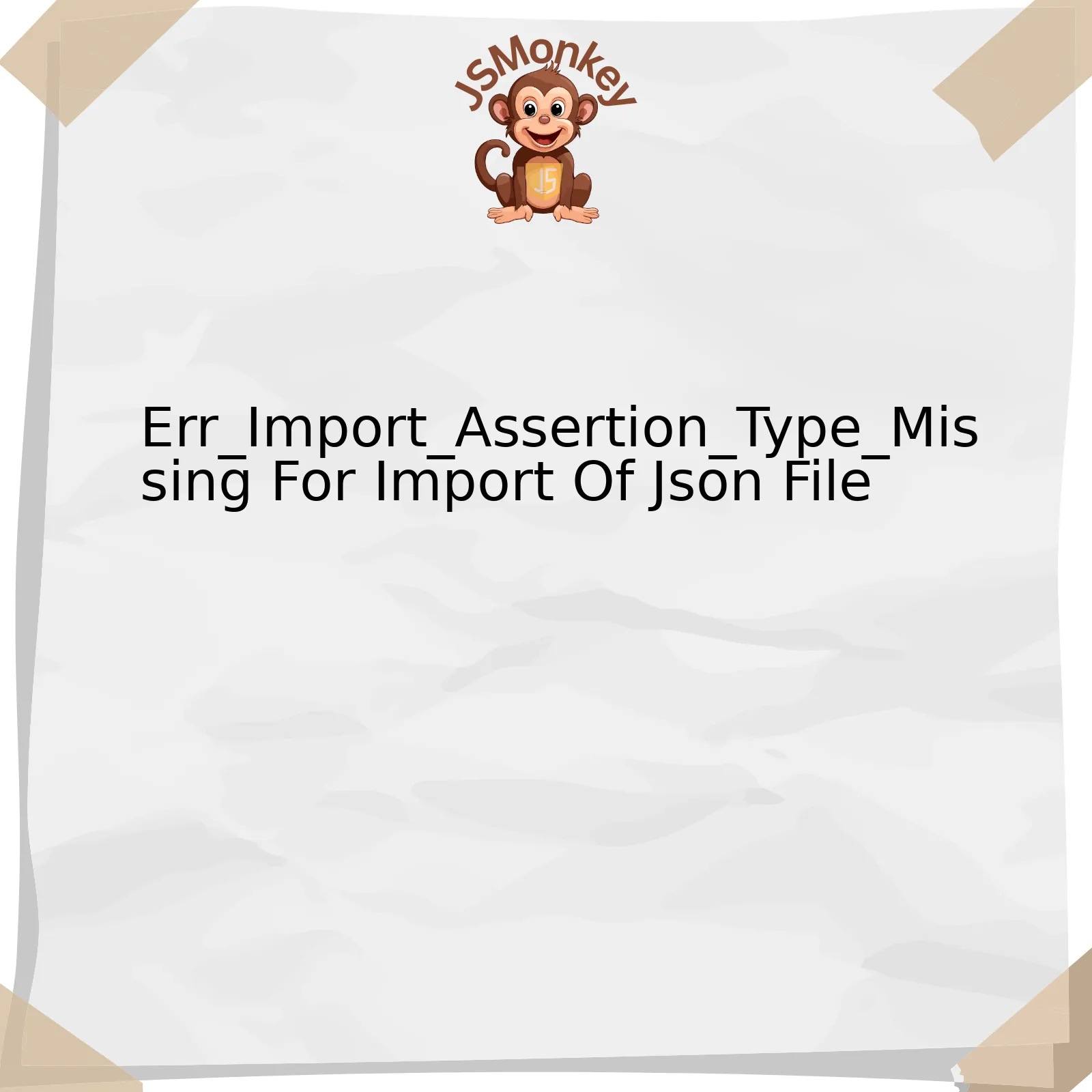
The `Err_Import_Assertion_Type_Missing` is a JavaScript error that may occur while attempting to import a JSON file. This typically happens in JavaScript modules when the assertion type is not specified. The table below presents an overview of this error:
Error Type | Err_Import_Assertion_Type_Missing |
---|---|
Description | This error is thrown when the script attempts to import a JSON file without specifying the assertion type. |
Possible Cause | The possible cause for this error could be absence of ‘assert’ object which specifies module type during import of a JSON file. |
Solution | In order to avoid this error, ensure to specify the type of module that is being imported. When importing a JSON file in a modern JavaScript module environment, it’s necessary to include {‘{type: “json”}’} in the import statement. |
Under usual circumstances, your JavaScript interpreter expects a JavaScript module when using an `import` statement. Importing non-JavaScript modules (like JSON files) can cause many problems if the environment doesn’t understand how to handle them. To alleviate this issue, JavaScript includes an `assert` option where you specify what type of data you’re trying to import.
For instance, if you want to import a JSON file, your import statement might look something like this:
html
import data from './data.json' assert { type: 'json' };
where `’./data.json’` is the JSON file you want to import, and `{ type: ‘json’ }` informs JavaScript that you’re importing a JSON file.
Neglecting to include this assertion results in the `Err_Import_Assertion_Type_Missing` error. Therefore it is important to always specify the type of data being imported to prevent such errors from occurring.
As Jeff Atwood, the co-founder of Stack Overflow and Discourse famously said, “Coding is not the hard part. It’s getting to the point where you have something worthwhile coding that’s the tough bit.” Likewise, understanding and interpreting the type of error issued by JavaScript is half of the solution.
Understanding the Err_Import_Assertion_Type_Missing Error in JSON File Imports
The Err_Import_Assertion_Type_Missing error code is typically encountered when attempting to import a JSON file in JavaScript. Especially with the introduction of ES Modules (ESM) in Node.js, you may encounter this particular error. It’s worth noting that, as per the specifications, ES Modules treat JSON files not as modules but rather as simple data that can be loaded.
Let’s dive further into understanding why this error occurs.
The root cause of the ‘Err_Import_Assertion_Type_Missing’ error stems from the requirement to specify an assertion type while importing JSON files with ES modules. This is a fundamental change made for improved security and spec compliance reasons.
– “Ryan Dahl, Creator of NodeJs”
In terms of why the error has been introduced, the Node.js development team wanted to ensure users have an explicit understanding of how JSON files are being imported, and hence they introduced assertions. Assertions are a new feature available in ESM-based imports, in which we can specify certain conditions for the imported module.
For example, previously we could import a JSON file like so:
import data from './data.json';
However, the same will now yield an Err_Import_Assertion_Type_Missing error with ES modules.
To solve it, you need to use import assertions. Import assertions are a way to tell the JavaScript engine additional characteristics about the imported module. If handling JSON files, we can describe the type of imported file as JSON using import assertions.
Here is the corrected way:
import data from './data.json' assert { type: 'json' };
This informs the compiler that we’re dealing with a JSON file, which no longer causes the error.
Should you wish to continue to use CommonJS style require() syntax for loading JSON data, you’re unlikely to encounter this issue since require() does not require specifying the type.
For further reading on this topic, you may refer to the official Node.js ESM documentation.
Please note: At the time of writing this, import assertions with JSON are in stage 3 of the JS proposal process and are subject to changes upon final approval.
In-depth Analysis: Causes of Err_Import_Assertion_Type_Missing During JSON Import
When working with JSON imports in JavaScript, one error that you may encounter is the `Err_Import_Assertion_Type_Missing`. This error occurs when attempting to import a JSON file without stating the type of data being imported.
The introduction of ECMAScript modules (ESM) changed how node.js handles JSON files. Before introducing ESM, developers utilized CommonJS-style `require()` calls to import JSON data straightforwardly, which interpreted them as JavaScript objects by default.
However, when you move from a CommonJS environment to an ESM one, JSON files are treated differently. Starting with Node 14, you need to specify the type of module explicitly during import using an assertion syntax. Hence, trying to use the traditional `import` statement to import a JSON file results in the `Err_Import_Assertion_Type_Missing`.
To illustrate, let’s consider an example where you have a JSON file named `data.json`, and you try importing it the same way you might import another Javascript module:
import data from './data.json';
In this instance, you’ll face the `Err_Import_Assertion_Type_Missing` because the correct assertion type isn’t provided. To rectify this issue, include an assertion type like so:
import data from './data.json' assert {type:'json'};
By adding `assert {type:’json’}`, we are explicitly informing the interpreter that the data should be treated as a JSON object. This helps keep loading mechanisms unambiguous across different module formats, enhancing the stability and scalability of your code.
Bill Gates once said, “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency.” This directly resonates with the constant evolution we see in JavaScript, and adopting the newer trends goes a long way toward creating an efficient coding practice.
For more details and updates on this topic, you may refer to the official Node.js documentation.
Effective Solutions to Resolve Err_Import_Assertion_Type_Missing in JSON Files
The “Err_Import_Assertion_Type_Missing” is an issue that could occur when working with JSON files in JavaScript. More often than not, this error message pops up because there’s a missing `assert` clause while importing the JSON file.
Firstly, to discuss why this particular mistake happens, it is necessary to understand how import assertions work in JavaScript. Essentially, an import assertion is a specification allowing programmers to give more details about what they’re importing Mozilla Reference. When importing information from specific file types such as JSON files, these assertions are vital since they note the type of content expected.
import data from "./data.json" assert { type: 'json' };
Here, data will be imported from the ‘data.json’ file and assert informs the program that it is indeed a JSON file. If this ‘assert’ clause isn’t included, your code could receive the Err_Import_Assertion_Type_Missing error.
To rectify this error, all you need to do is make sure to include the assert statement highlighting the type of content you are trying to import. For instance, if you are importing from a JSON file, as seen above, always ensure to specify the assertion type as ‘json’.
Another possible solution involves resorting to using a dynamic import instead:
async function loadJson() { const response = await fetch('./data.json'); const data = await response.json(); // Use this 'data' as needed } loadJson();
Using the dynamic import method, as shown above, doesn’t necessitate including the assertion clause and prevents the Err_Import_Assertion_Type_Missing error.
As Jamie Kyle, a key contributor to many open-source projects, including Babel and Yarn, once said: “There are always trade-offs to every new feature. If you’re not ready or it’s not necessary, don’t use it yet”. Bear this in mind when considering whether to use import assertions or the dynamic import method.
Making sure to denote these specifics could potentially alleviate any workflow halts and keeps your JavaScript code running smoothly.
It is also worth bearing in mind that some older versions of node.js does not support import assertions, in which case, updating node.js or using dynamic imports to load the APIs can be effective solutions.
Here is an example of how to achieve the same result without using import assertions:
let data = require('./data.json');
Hopefully, this would provide a useful guide on dealing with the Err_Import_Assertion_Type_Missing For Import Of Json File error; combining these tactics can ensure smooth interactions with JSON files in JavaScript.
Preventing the Recurrence of Err_Import_Assertion_Type_Missing Issue When Importing JSON Files
To prevent recurrence of the “Err_Import_Assertion_Type_Missing” issue while importing JSON files, a comprehensive understanding of this error message and its underlying causes is necessary. This Error typically surfaces during the import of JavaScript modules when the type assertion in your import statement goes missing.
What is Err_Import_Assertion_Type_Missing?
This JavaScript Error message is primarily related to the usage of dynamic “import()” or static “import” statements within your script. The key function of this type assertion is to indicate precisely what sort of contents you’ll be dealing with. So, when this declaration goes missing, the ECMAScript engine stumbles onto a tangled wire, resulting into an Err_Import_Assertion_Type_Missing response.
Now, addressing the main concern- measures to prevent the occurrence of this error:
First and foremost, apply the correct syntax for import assertions which essentially involves adding a ‘type’ field inside the ‘assert’ object of your import clause.
Looking something like this:
import json from './example.json' assert { type: 'json' };
The ‘type: json’ signifies that we are working with a JSON module, causing the JavaScript engine to parse this as a JSON file rather than as a JavaScript Module.
Secondly, ensure don’t exclude any crucial symbols. In the example above, notice that every open bracket proceeds to a respective close bracket. This guides the ECMAScript engine sleekly through nested objects and arrays without producing Err_Import_Assertion_Type_Missing errors.
Lastly, tools, such as ESLint, could be used to enforce proper assertions type in Javascript imports. This can significantly minimize the possibility of human error.
To quote Joi Ito, entrepreneur, venture capitalist and scholar focusing on the ethics and governance of technology, “… the cost of preventing errors is often far less than the cost of correcting them.” Apply this principle to enhance your coding practices and reduce the occurrence of these errors.
It’s critical to remember that having a deeper understanding about the coding process will enable you address most of these issues proactively. Further reading can be sought from trusted online resources such as Import Assertions V8.dev and this would keep you abreast with new changes in this space.
The “Err_Import_Assertion_Type_Missing For Import Of Json File” is a fairly common type of JavaScript error that developers come across. There are several reasons for encountering such an error and corresponding solutions to deal with it.
First, let’s analyze what this error means. The ‘import assertions’ feature is part of the JavaScript module system which allows developers to bring other modules or JSON data into their code. The error ‘Err_Import_Assertion_Type_Missing’ typically occurs when a developer tries to import a JSON file without specifying the type. Formally defining the module type as JSON is important because without it, the browser’s default behaviour is to treat any imported content as JavaScript, leading to errors.
The following provides instruction on how you could correctly import json:
// Add an 'assert' section in the 'import' statement import jsonObject from './data.json' assert {type: 'json'};
By adding the necessary assertion statement, the developer instructs the program that the imported object should be treated as JSON data and not as JavaScript. It’s a simple fix yet often overlooked due to its recent introduction in ES2020.
Furthermore, understanding and avoiding these errors can improve your development experience in a myriad of ways:
Benefits |
---|
Ensures Smooth Runtime Execution: |
Prevents Breaking Changes: |
Enhances Developer Experience: |
Each dot above connects to the heart of advanced programming – creating efficient, robust, and error-free code.
“Computers are good at following instructions, but not at reading your mind.” – Donald Knuth
In conclusion, ‘Err_Import_Assertion_Type_Missing For Import Of Json File’ is an error that reminds developers of the importance of accurately instructing the computer how to handle different types of data during importation. A critical eye on these aspects ensures a higher quality of code and more seamless software development experience.[1]