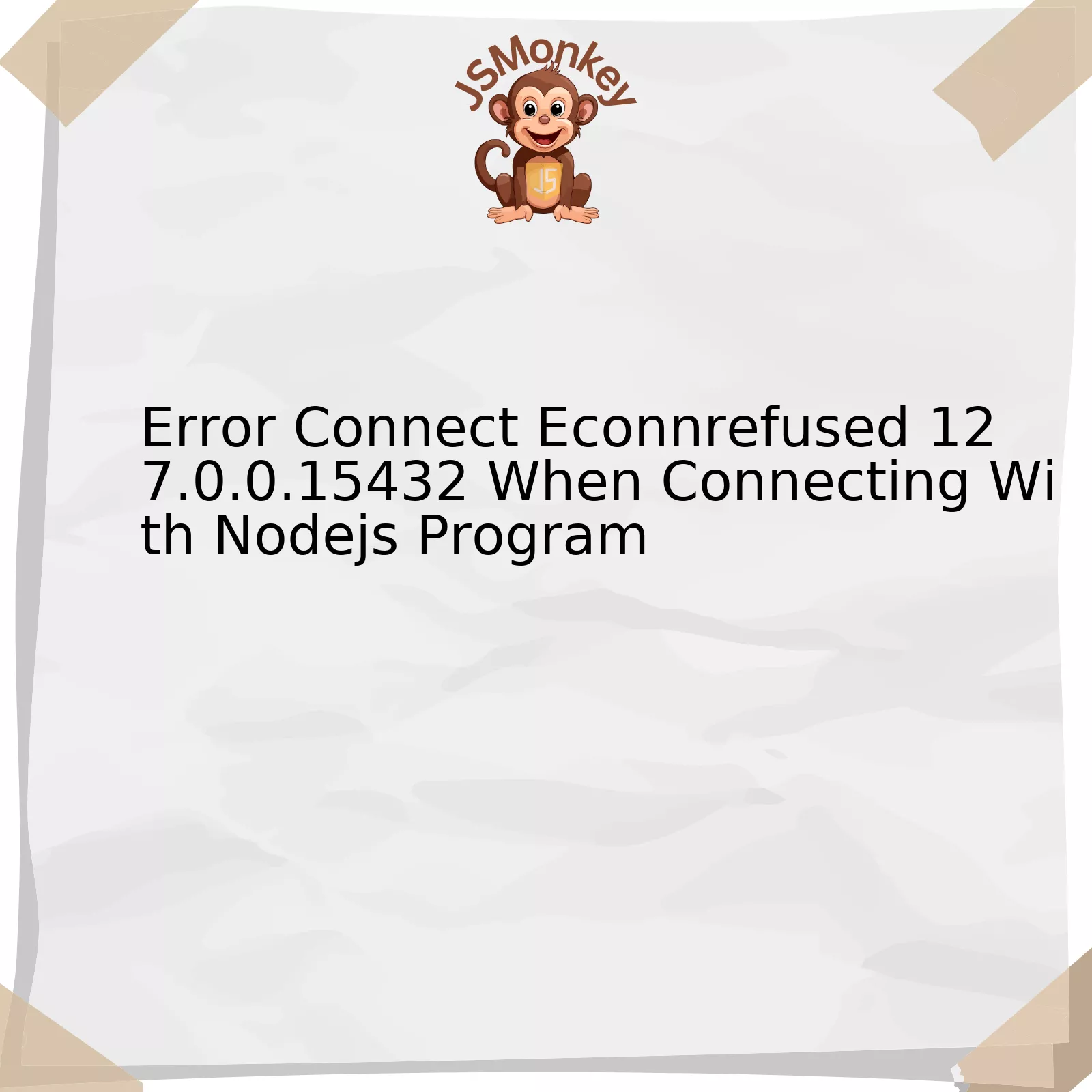
The “ECONNREFUSED” error that you’re encountering is quite common and it’s related to Node.js trying to connect to a specific address— in this case, 127.0.0.1:5432— but there isn’t anything set up there to accept the connection. The term ECONNREFUSED means that the connection was explicitly rejected by the machine you are trying to connect to. In order to better explain this, let’s consider the following table which summarizes key details of this error:
Error Message | Meaning | Possible Causes | Resolution Steps |
---|---|---|---|
Error Connect ECONNREFUSED 127.0.0.1:5432 |
The attempt to connect to the server at 127.0.0.1 on port 5432 was refused. | – Target server is not running. – Firewall blocking access. – Address or port specified incorrectly. |
– Verify if the server is running and accessible. – Check if a firewall is obstructing access. – Cross-verify the IP address and port. |
While dealing with this kind of error, it must be acknowledged that various factors could lead to its manifestation. Here are some potential culprits:
1. **Server Not Running**: Ensure that the server that Node.js is trying to connect to is running. You can often check this through a control panel or command line tool.
2. **Incorrect Port/Address**: The error could result from Node.js attempting to link to the wrong port or address. Confirm the correct inputs are being used.
3. **Firewall Restrictions**:Firewalls may block certain ports for security reasons. Ensure your connection request isn’t being blunted by firewall rules.
To fix these Node.js “ECONNREFUSED” errors, you might take steps such as verifying server operations, confirming the address and port, or adjusting firewall settings. Keep in mind that all networking issues involve careful troubleshooting and multiple potential points of failure.
As Wendell Odom once said, “When it comes to networking, data will inevitably need to be transmitted through various routes and means. Understanding this interconnectedness makes for better networking…and better troubleshooting.”
Hence, it’s important not only to know how to handle such errors but also understand what might cause them to ensure enhancements in your Node.js programming journey.
Understanding the “Error Connect Econnrefused 127.0.0.15432” Issue in Nodejs
The “Error Connect Econnrefused 127.0.0.15432” issue in Node.JS signifies a failed attempt to establish a communication link between the client and the server. The underlying reason lies within the fact that the program is trying to connect to a server at IP 127.0.0.1 on port 5432, but was unable to find any active process listening at this particular network address.
A couple of situations may lead to the manifestation of this problem:
– Server not Active: If your server isn’t running or has been shut down abruptly, this error can occur.
– Firewall Restrictions: Sometimes, the system firewalls or network security protocols might prevent a successful connection opening.
– Incorrect Port Number: It’s also possible that the application tries to connect with an incorrect port number.
– Network Address Bindings: Bindings associated with the networking interfaces could be misallocated.
Resolving the “Error Connect Econnrefused 127.0.0.15432” involves circumventing these underlying issues:
Action | Explanation |
---|---|
Ensure Server is Running | Before establishing a connection, check whether the server is active and is ready to accept new connections. |
Check Firewall Settings | A review of firewall settings could help ensure no policies are obstructing the communication flow. Work with your firewall settings to exclude your port from being blocked or alternatively whitelist your application process for the firewall. |
Verify Port Allocations | Make certain that you connect to the correct port. Cross-verify port number details in your connection string. |
Review Network Interface Bindings | Ensure that correct IP addresses are set in the ‘listen_addresses’ of your PostgreSQL.conf file and you’re connecting to an IP address that PostgreSQL is actually bound to. |
An example of a connection string can be demonstrated by using Node.js `pg` library:
const { Pool } = require('pg') const pool = new Pool({ host: '127.0.0.1', port: 5432, user: 'yourUsername', password: 'yourPassword', database: 'yourDatabase' })
As the renowned software developer, Linus Torvalds once relayed, “Talk all you want about ‘advanced’ features; what most users care about in the end is reliability.” Thus the importance of addressing such connectivity issues for ensuring seamless interaction between client-server applications.
Remember, fundamental to addressing error-related problems in the realm of programming is understanding the nature of the error itself. An effective approach would be to diagnose each potential cause systematically, eventually leading you to the root problem.
Reference:
Node PostgreSQL Docs
Troubleshooting Steps for Econnrefused Errors in Nodejs Program
The error connect `ECONNREFUSED 127.0.0.1:5432` in a Node.js program appertains to a common issue – the connection is being rejected by the server for one of several potential reasons. The specific error message indicates that your Node.js client is attempting to establish a network communication at localhost (`127.0.0.1`) on port `5432`. This might be typically associated with a PostgreSQL database, given this port is often utilized for its services.
Troubleshooting Steps
A systematic approach should be employed to detect and resolve this type of issue.
Step 1: Verification of Server Status
Ensure, primarily, that your PostgreSQL server is up and running. Use the command `
psql -U postgres
` in your terminal for checking the server status. If the server isn’t live, it won’t accept connections, and restart is prerequisite.
Step 2: Examine Connectivity
Inspect whether other applications are successfully connecting to the same server, possibly eliminating machine-wide network issues. Experiment with simple code snippets, or use a PostgreSQL client software such as pgAdmin.
Step 3: Port Configuration
As the problem might stem from incorrect port configuration, verify that your application is using port `5432` for PostgreSQL connection. You’d typically configure the port data in a settings file or an environmental variable.
Step 4: Validate Code Snippets For Connection
The issue could emanate from connection settings in your Node.js program.
Consider a typical setup using `pg-promise`:
const pgp = require('pg-promise')(/*options*/); const database = pgp({ user: 'postgres', password: 'password', host: 'localhost', port: 5432, database: 'database' });
Double-check that there are no typographical mistakes in the crucial elements such as ‘user’, ‘password’, ‘host’, ‘port’, and ‘database’.
Step 5: Firewall Rules
Firewalls interfere with connectivity by preventing unwarranted access. Review your firewall settings to ensure they permit connections on the specified port.
Bill Gates once highlighted, “It’s really clear that the most precious resource we all have is time.” In professional programming endeavors, efficient error-resolution practices indeed save us much of this invaluable resource.
Importance of Correct Server Configuration to Avoid ‘Econnrefused’ Errors
The ‘ECONNREFUSED’ error, specifically ‘Error Connect ECONNREFUSED 127.0.0.1:5432’, is a common issue encountered by JavaScript developers working with Node.js programs. This error essentially means that the connection was explicitly denied by the server due to incorrect or inadequate configurations on the server end while connecting with a Node.js application.
There are several potential reasons for experiencing this error:
– The server refuses the connection made by the client.
– There might be insufficient permissions given to the Node.js application to connect with the server.
– The server might be unreachable because it’s down, rebooting, or overly busy handling other requests.
Additionally, solving the ‘Error Connect ECONNREFUSED 127.0.0.1:5432’ requires understanding and implementing correct server configuration.
Server Configuration Factor | Description |
---|---|
Valid IP Address and Port | The IP address (localhost or 127.0.0.1 in most cases) and port number (5432 in the context of this question) need to be correctly configured in both the server and Node.js application. |
Correct Database Credentials | If your Node.js application is trying to connect to a database server (Postgres in our case, given we’re speaking about port 5432), make sure that the database user credentials (username and password) are accurate and have valid permissions. |
Firewall Rules | The firewall shouldn’t block incoming connections from the Node.js application to the server. Configuring appropriate inbound rules in the firewall settings plays a critical role. |
Server Availability and Performance | The server needs to be up and running while the Node.js application tries to connect. Proper server monitoring helps detect issues beforehand and increases the server’s availability and performance. |
Here is a simple illustration on how one might establish connection from Node.js to PostgreSQL:
javascript
const { Pool } = require(‘pg’);
const pool = new Pool({
user: ‘my_username’,
host: ‘127.0.0.1’,
database: ‘my_database’,
password: ‘my_password’,
port: 5432,
});
In this instance, if ‘Error Connect ECONNREFUSED 127.0.0.1:5432’ error occurs, check each of the factors enumerated in the table above to resolve the issue.
As Todd Motto, a Google Developer Expert, puts it succinctly, “Treat your code as prose.” This idea extends to server configurations as well. A correctly configured server not only ensures a seamless connection with your Node.js application but also significantly reduces the occurrence of errors like ‘Econnrefused’. Ensuring that the server configurations are always in their most optimal state will make the tasks of debugging and problem-solving much simpler.
Practical Solutions to Fix ‘Econnrefused 127.0.0.15432’ Error Using Node.js
The ‘ECONNREFUSED’ error typically appears when a connection attempt has been rejected by the server. In this context, ECONNREFUSED 127.0.0.15432 signifies that your Node.js program is attempting to connect to the IP address 127.0.0.1 (localhost) at port 5432 (default PostgreSQL port), but it cannot establish this connection.
Given below are practical solutions and steps to troubleshoot ‘Error Connect Econnrefused 127.0.0.15432’ issue in Node.js:
A. Examine the Server Status:
Start by checking if the server (in this case PostgreSQL running at port 5432 on localhost) is active and accepting connections. Use appropriate command line tools or GUI based administration tools to check the server status.
B. Verify Correct Connection Parameters:
Ensure that your JavaScript code uses the accurate network information for connecting to the server. Common mistakes may include typos in the host name or incorrect port number. Here’s an example for a typical connection setup:
var pg = require('pg'); var conString = "postgres://username:password@localhost:5432/databaseName"; pg.connect(conString, function(err, client, done) { ... });
C. Firewall or Network Issues:
A local or network firewall might be blocking connections to the target port. On Unix-based systems, you can use the `netstat` command to see if anything else is already listening on the desired port.
D. Incorrect Database Configuration:
PostgreSQL is configured to deny remote connections by default. However, if your node.js application is running on the same machine, ensure that your PostgreSQL database is configured properly to accept connections from localhost.
“As Bill Gates once said, ‘We all need people who will give us feedback. That’s how we improve.’ The same is true for software – feedback in the form of error messages is an important tool for finding and fixing issues.”
Most importantly, debugging network errors requires patience, a methodical approach, and a clear understanding of network principles. It’s not uncommon for minor details to be the cause, therefore it is recommended that every configuration detail be reviewed closely.
In high-level terms, we must ensure that our Node.js application can reach the server and that the server is correctly configured to accept connections from our application.
For further information on this, check out [Common System Errors in Node] where ‘ECONNREFUSED’ is listed as well as various other common errors you might come across when running your Node.js applications.
“Undoubtedly, the Error Connect Econnrefused 127.0.0.15432 when connecting with Node.js program presents a major hurdle for developers. It’s reminiscent of a scenario where one is trying to open a locked door, but the key isn’t fitting correctly. This error typically arises due to network constrictions and usually implies that your Node.js program is trying to establish a connection to a server, but the server isn’t accepting the connection.
Addressing this issue involves identifying potential causes such as:
* Incorrectly configured connection parameters
* Network restrictions on the server end
* The specified port might not be in active use
Let’s consider an example in which these causes manifest. Meanwhile, the solution revolves around rectifying these potential stumbling blocks.
var mysql = require('mysql'); var con = mysql.createConnection({ host: "localhost", user: "yourusername", password: "yourpassword" }); con.connect(function(err) { if (err) throw err; console.log("Connected!"); });
In the above code snippet, ensure that the hostname, username and password are correct and that the MySQL server is running properly.
Aligning your approach towards resolving this error can drastically streamline your overall Node.js development experience. As Jamie Zawinski, a renowned software engineer once said, ‘Software is like entropy. It is difficult to grasp, weighs nothing, and obeys the Second Law of Thermodynamics; i.e., it always increases.’
A strategic resolution to technical glitches like the Error Connect Econnrefused 1270.0.0.15432 when connecting with Node.js program includes diligent analysis, understanding the underlying platform, and learning from community forums or online resources.”