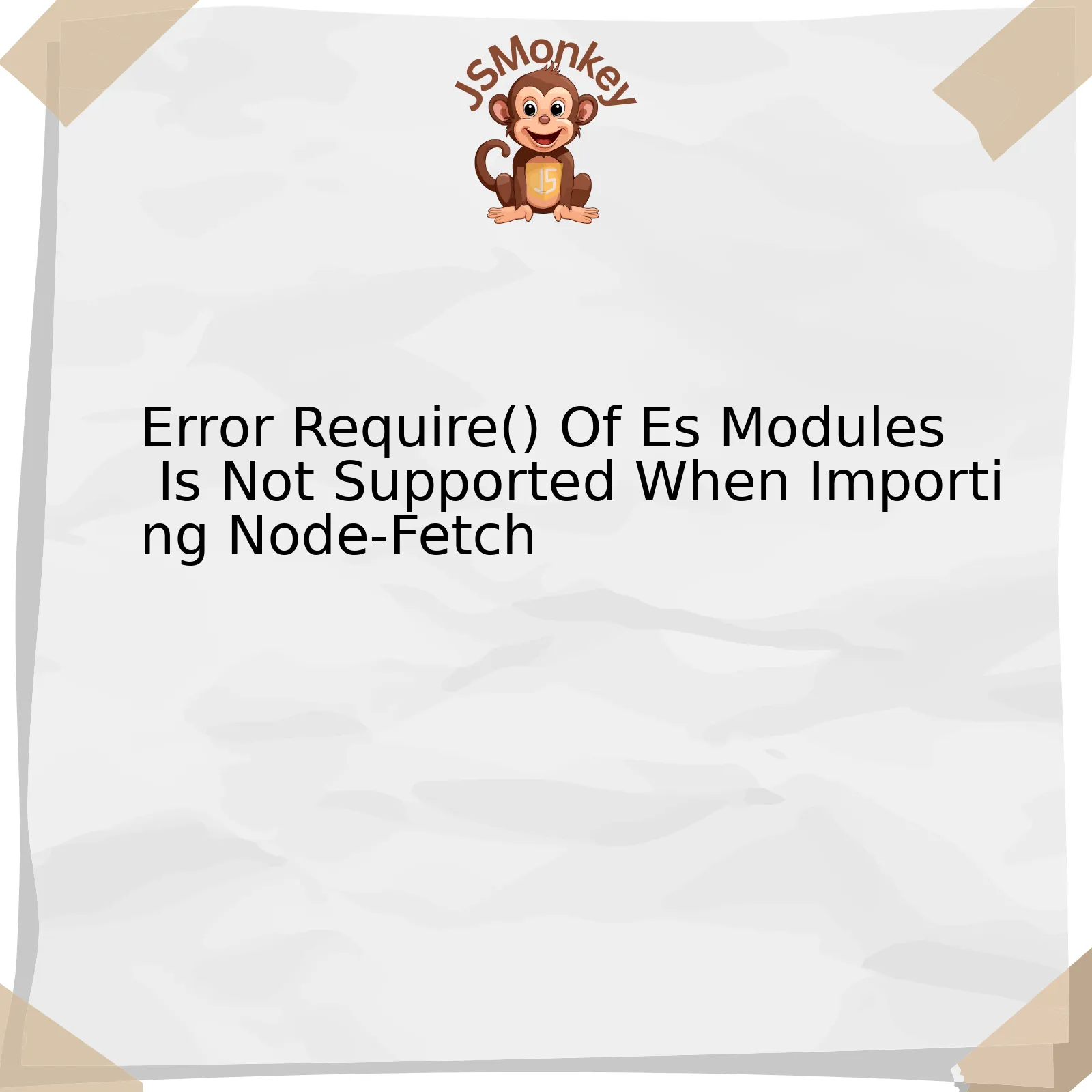
The ES (ECMAScript) Modules syntax is a standard for modular JavaScript which was introduced in ECMAScript 2015 also known as ES6. However, it’s important to acknowledge that the Node.js built-in `require()` method with ES6 Modules poses challenges due to the distinction between import/export and require/module.exports systems.
To better understand this, let us group relevant information about `require()`, ES modules, and how they interact in Node.js environment using the Node-fetch library.
Category | Details |
---|---|
require() function |
This is how Node.js imports modules before ES6. It synchronously loads module content. |
ES6 Modules Import | This feature provides an integrated, compact syntax to load modules asynchronously, but it’s not natively compatible with
require() . |
Error generation scenario | The error “Require() of ES modules is not supported” arises when you try to utilize
require() to import an ES Module structure such as Node-fetch module, designed as an ES module. |
Solution | To resolve the issue, use the ES6
import keyword instead of require() . Alternatively, use the ‘.default’ property when requiring an ES module. |
Let’s elaborate on the error trigger scenario. Essentially, Node-Fetch module targets ES6 specifically. Thus, it is engineered using ES6 export default format.
This creates an incompatibility when utilizing
require()
, as pre-ES6 modules do not recognize ES6 export syntax.
For instance, when you write:
const fetch = require('node-fetch');
Node.js, not recognizing ES6 import/export syntax, throws an error “Require() of ES modules is not supported”.
To bypass this, adopt the standard ES6
import keyword to import the Node-Fetch module. Here's an example:import fetch from 'node-fetch';This code aligns with ES6 standards and will import the Node-Fetch function appropriately without throwing errors.
However, if you prefer to stick with
require(), attaching '.default' to the end of
require()would solve the issue:
const fetch = require('node-fetch').default;In this case, .default is the exported object from a module in ES6 module system that we can conveniently access using commonjs.
Therefore, comprehending these distinctive aspects of JavaScript's modularity allows us to effectively mitigate errors such as "Require() of ES modules is not supported". As Jamie Kyle, a notable JavaScript developer observed - "Understanding your tools means better productivity, less frustration and more quality output."
To simplify things further, developers can consider using tools like Babel or TypeScript which handle module compatibility issues providing consistent syntax norms between client and server-side rendering.
It's important to keep abreast of evolving coding standards to ensure old and new techniques coexist harmoniously, to minimize interruptions during application development.
Understanding Why Require() of ES Modules Isn't Supported while Importing Node-Fetch
The error "require() of ES modules is not supported" usually comes up when you're trying to import an ES Module using the require() function in Node.js. This scenario applies to thenode-fetchpackage, which can give rise to such an issue.
Diving deeper into ES Modules and CommonJS in Node.js:
Primarily, JavaScript modules come in two main types:
require()
function for module loading.
import/export
syntax.
Node.js primarily functions on CommonJS, but it also provides support for ES modules. However, importing an ESM package via the
require()
function is not endorsed. Whereas
node-fetch
is an ES module, errors arise if we try importing it using
require()
.
Solving "require() of ES modules is not supported" error in Node-fetch:
To fix this, you can use dynamic
import()
statements or convert your application to use ESM syntax.
Using Dynamic
import()
:
const fetch = (...args) => import('node-fetch').then(({default: fetch}) => fetch(...args));
Converting to ESM Syntax:
Note: You will need to set proper file extensions (.mjs) or add "type": "module" in your package.json for it to work correctly.
import fetch from 'node-fetch';
As Douglas Crockford, a JavaScript developer well known for his work on JSON data format, once said, "Programming is not about typing, it's about thinking". These solutions provided are aimed to inject more understanding rather than being quick fixes. Deep comprehension of the underlying JavaScript module systems will substantially benefit when encountering related scenarios in your coding journey.
More information on ES modules in Node.js can be found in this official Node.js documentation.
Investigating Common Issues and Solutions for Node-Fetch Imports Failures
When delving into the commonly faced issues surrounding the importation of Node-fetch in JavaScript, it is vital to emphasize that one of the recurrent challenges is associated with the "Error Require() of ES Modules is not supported".
Why does this error occur? JavaScript has hitherto been impeded by its lack of a standard module system which, when conjoined with its independent peripheral environment compatibility requirements, engenders a complicated ecosystems of script loading solutions. Over time, the community consolidated around CommonJS modules (as used by `Node.js`) and ECMAScript 2015 (ES6) modules. The key discrepancy between these two pertains to when they execute their imports - ES6 executes at compile-time while CommonJS modules execute at runtime.
The error under scrutiny here arises when developers iterate from the traditional `require` syntax intrinsic to CommonJS towards ES6 modules, via utilisation of the `import` syntax. If you're keen to leverage ES6 modules whilst using Node.js (or any derivative technologies), it's crucial to comprehend that ES6 and CommonJS manage modules differently. Easy slip-ups or misunderstanding can lead to errors like `Error: require() of ES modules is not supported`.
Overcoming the Error
An immediate solution that might emerge would be to resort to CommonJS style requiring Node-fetch as opposed to importing.
const fetch = require('node-fetch');
This circumvents the entire issue about ES Modules altogether but could be an inconvenience for those who are determined or required to adopt ES6 syntax and features. It also flies in face of the industry-wide adoption of ES6 syntax for modern web development.
Making Use of .mjs Extension
A workaround brought along by Node.js involves changing your `.js` files, housing import statements, into `.mjs` files. This informs Node.js that it should parse the contained code as ES6 module syntax. In this respect, your previous `node-fetch` import will appear akin to:
import fetch from 'node-fetch';
Embedded within a `.mjs` file and will achieve flawless execution.
Flagging Node.js for ES Modules
In instances where changing each Javascript file's extension feels inconvenient or is interpreted as too large of an undertaking, you also possess the option of conveying to Node.js that it should treat all `.js` files as ES modules—under the asset of a given project.
To accomplish this you essentially craft a package.json file within your project’s root directory (in the event one does not already exist) and incorporate a `"type": "module"` property.
While these resolutions might prove handy, developers must also consider that adopting ES Modules syntax entails a broader shift in how your entire codebase interacts with imports and exports, not limited to `node-fetch`.
As Douglas Crockford, a keen observer and architect of JavaScript once said:
"The good thing about reinventing the wheel is that you can get a round one."
Often, overcoming these roadblocks is part and parcel of the progress we make in JavaScript development. [source]
Exploring Alternatives to Improve ES Modules in Your Node.Fetch Functionality
When it comes to enhancing ES Modules in your Node.Fetch functionality, there's inevitably a discussion about the pervasive error - "Require() of ES Modules is not supported", that crops up when using the popular library Node-Fetch.
The common reason why developers encounter this issue pivots on the interplay between CommonJS and ES Modules. Here's an analysis:
- Node.js originally supported CommonJS modules. Accordingly, 'require' is utilized to import modules.
- ES Modules - a relatively new introduction in JavaScript, uses 'import' to bring in modules. This is where the conflict arises with older libraries, like Node-Fetch which were designed keeping in mind, CommonJS support.
This then, further accentuates the need for improving ES Module handling within the Node.Fetch framework.
Worth considering here, some valid alternatives or solutions to rectify this error:
1. Unifying Import Syntax:
Instead of using
const fetch = require('node-fetch')
, utilize
import fetch from 'node-fetch'
. Although, be mindful that Node.js may necessitate the use of the .mjs file extension or setting "type": "module" in your package.json to permit ES module syntax.
2. Using createRequire:
Utilize the 'createRequire' function from 'module' class. Scott Hanselman's quote, "The only way to make the deadline—the only way to go fast—is to keep the code as clean as possible at all times," holds apt for the use-case. Clean, straightforward code often offers the best solutions. For instance:
import { createRequire } from 'module'; const require = createRequire(import.meta.url); const fetch = require('node-fetch');
This allows you to use 'require' inside an ES module, incrementally bridging the gap between the two formats.
3. Transpilation: Transpile your code using Babel or Typescript. In this way, at runtime, you're always using CommonJS, thereby, circumventing the issue.
4. Switching Libraries: Consider switching to a modern library with better ES Modules support such as Axios.
For further reading, I would recommend exploring Node.js ES Modules documentation or Node's documentation on
require.
Precisely, It is essential to understand the significance of different module systems, their strengths, and weaknesses, to make a well-informed choice when building Node.js applications. Delving deeper into these tactics should lead to an enrichment in your usage of the ES Modules within the Node.Fetch functionality.
Enhancing Code Quality: Best Practices Avoiding Error with Require() of ES Modules When Using Node-Fetch
Ensuring code quality when working with JavaScript, particularly in the dynamically changing environment of ES modules and Node.js is paramount. To mitigate errors related to `require()` of ES Modules when importing Node-Fetch, certain practices come highly recommended.
Firstly, understanding the cause of “Error Require() Of Es Modules Is Not Supported” is crucial. This error typically comes about because the CommonJS modules use `require` method which is not inherently compatible with ES6 syntax that depend on ‘import’ for module loading. Node-Fetch, being an HTTP client for Node.js making use of promises, leans more towards the modern ES6 module syntax.
import fetch from 'node-fetch';
Therefore, to circumvent the issue, a change in the manner of importing modules becomes necessary. Using dynamic imports instead of static imports could resolve this hiccup. Dynamic imports offer a workaround till Node.js supports ES6 imports natively. The node-fetch module can be imported with async functions, presented in the following format:
(async () => { const fetch = (...args) => import('node-fetch').then(({default: fetch}) => fetch(...args)); const response = await fetch('https://google.com'); // ... })();
We could also make use of Babel, a transpiler that converts the ES6 import/export syntax into CommonJS require/module.exportssource. For example:
const fetch = require('node-fetch');
Lastly, experiment with ECMAScript module packages in Node.js using the `–experimental-modules` flag.
Considering the cautionary remark by Kevlin Henneysource, an independent consultant, trainer, reviewer and writer, “the difference between a tolerable programmer and a great programmer is not how many programming languages they know, and it’s not whether they prefer Python or Java. It’s whether they can communicate their ideas.” It’s essential for developers to share such best-practices & solutions among the community. On maintaining code quality and enhancing it, the robustness of our applications depends.
When working with the Node.js environment, you may encounter an issue where the require() of ES modules is not supported when importing `node-fetch`. The visible result of this concern presents itself as an error message that states: **”require() of ES Modules is not supported”**. This predicament occurs because, unlike CommonJS modules where you can use the require() function, ES6 modules advocate for the use of import/export syntax.
var fetch = require('node-fetch'); |
import fetch from 'node-fetch'; |
Incorrect way in an ES module | Correct usage with ES Module syntax |
To elucidate, this transition to using ES Modules came about as a way to standardize JavaScript and allow for more advanced features, such as static analysis and improved optimization on bigger codebases. As part of this standardization process, ‘import’ and ‘export’ were introduced – these modern replacements for ‘require()’ bring with them several benefits like compile-time (static) module resolution and an overall syntactic ease.
Unfortunately, legacy codes or those who are shifting their base might still depend on the `require()` function, thus causing difficulties. Notably, this discrepancy rarely arises because of the node-fetch module but typically happens due to the method utilized to require it.
Clarifying and combatting this error necessitates an understanding of both coding environments as well as effective ways to bridge the proverbial gap between them. When interoperability is required, built-in Node.js functions like `import()` and `createRequire()` can be quite helpful. Equally viable, though requiring a little more effort, is the preprocessing of your source code using a tool like Babel.
Lastly, to borrow a line from Graydon Hoare, the creator of Rust programming language: “I think everyone who codes has a naive belief they can do it better, until shown how large the problem is.” With this in mind, being patient and systematic about facing such issues in JavaScript can continue to be the most effective strategy. For more context and solution to this error, visiting sites like Stack Overflow could offer interactive help from myriad developers encountering similar challenges.