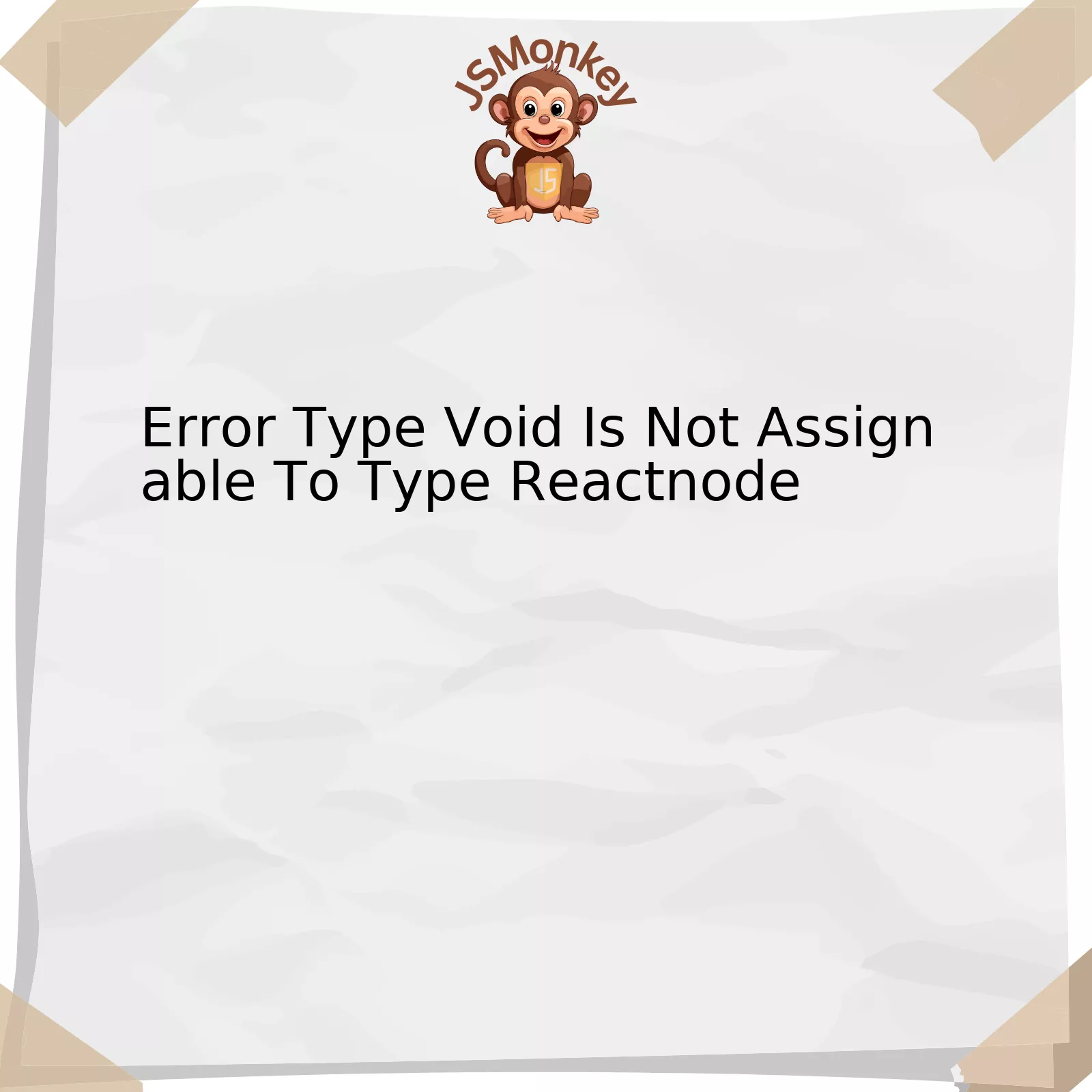
The “Error: Type ‘void’ is not assignable to type ‘ReactNode'” is one of the common type-related errors encountered by JavaScript developers when utilizing React. The root cause of this error generally hinges on the fact that a JavaScript function encapsulating some JSX code does not return anything, hence the ‘void’.
Let’s explore the subject in a thematic table format:
Term / Concept | Explanation |
---|---|
Type ‘void’ | In JavaScript, ‘void’ indicates that a function does not return anything. This could be an intentional programming style or an oversight. A function declared as void cannot return any values. |
Type ‘ReactNode’ | ‘ReactNode’ refers to anything that can be rendered by React. It includes but is not limited to null, boolean, number, string, and objects like elements or array of nodes. |
The Error | This error expresses that instead of a ReactNode, the function is producing ‘void’ which cannot be rendered by React. |
Now let’s dig deeper into the issue at hand.
Correctly identifying the root cause of this error requires understanding the context in which it occurs and a thorough knowledge of the nature of both ‘void’ and ‘ReactNode’. As explained in this [post](https://stackoverflow.com/questions/54905376/type-void-is-not-assignable-to-type-reactnode), a function intended to render a React component should always return a valid ‘ReactNode’, not ‘void’.
For instance, consider the following piece of erroneous code:
const DisplayName = (name: string) => { console.log(name); };
This function logs the inputted name instead of returning it. The ‘void’ type is thus unassignable to ‘ReactNode’, which results in this specific error being generated.
A potential solution would involve modifying the function so that it returns an acceptable value for ‘ReactNode’; such as:
const DisplayName = (name: string): JSX.Element => { return <p>{name}</p>; };
In this enhanced code snippet, a paragraph element housing the name is explicitly returned.
Professor Richard Jones encapsulates our conversation hitherto succinctly:
“Debugging is like doing a jigsaw puzzle with millions of pieces and no picture on the lid.” Finding the cause of any kind of bug, including type mismatching issues requires thorough knowledge, patience, and precision.
Understanding Void Error in React Development
The error message “Type ‘void’ is not assignable to type ‘ReactNode'” typically arises in React development when a function or component returns `void` rather than a valid `ReactNode`. React demands that each component must return a `ReactNode` – an understandable concept, taking into account React’s component-based architecture. A `ReactNode` can be any legitimate JSX or null.
To cherish the importance of this requirement, consider viewing your application as a tree structure where components form nodes. Each node on the tree must contain either another component/node (JSX) or be empty (null). Returning `void` disruptively interrupts this structural pattern, bestowing upon you, the developer, the aforementioned error message.
So what does ‘void’ mean in JavaScript? In essence, it evaluates the given expression and then returns undefined.
void
is frequently used for functions which perform an action but do not return a value (like console.log).
Consider the following erroneous example:
jsx
const ExampleComponent: React.FC = () => {
console.log(“This component does nothing!”);
}
In this case, `ExampleComponent` essentially returns `void` because no value is returned from it. Console.log merely performs a task, results hold no substantial value, hence it ends up returning `void`. This activity contrasts with React’s architectural prerequisites, generating the “Type ‘void’ is not assignable to type ‘Reactnode'” error message.
Conversely, a correct approach would look something like this:
jsx
const ExampleComponent: React.FC = () => {
console.log(“This component does nothing!”);
return null;
}
With this corrected code snippet, instead of returning `void`, the `ExampleComponent` now properly provides back `null`, aligning itself convincingly with being a legitimate `ReactNode`.
As per Facebook’s software engineer Dan Abramov’s insights on code writing best practices, “Code is read far more times than it’s written”. Our primary goal should always be to make our programs as understandable as they can possibly be. By adopting React’s requirement of returning a `ReactNode` from every component, we thereby solidify the uniformity and readability of our applications’ code structure.
However, remember this error message isn’t just about proper code writing practices. It also emphasizes on understanding the nature of JavaScript and how different types (like `void`) can interact with libraries such as React. So, the next time you encounter the “Type ‘void’ is not assignable to type ‘Reactnode'” error, rather than merely correcting it, take a moment to appreciate the architectural decisions underpinning the React framework that helps us build structured, readable code.
Resolving “Void is Not Assignable to Type Reactnode” Issue
Delving into the problem “Void is Not Assignable to Type Reactnode”, it’s essential to understand what this error indicates before we venture into finding solutions. The error message derives from TypeScript, a superset of JavaScript that introduces types. It typically suggests an incompatibility between the type of a component’s expected return value and what your function or component is currently trying to return.
In this case, React requires a visual element such as JSX or null (ReactNode), while the function or component is programmed to give nothing back (void).
To have a grasp on why this could occur, consider the below sample code:
html
import React from ‘react’;
function MyComponent(): void {
}
Notice anything strange? Yes, our `MyComponent` doesn’t return anything – this implicates void, which runs contrary to what a React component is expected to provide. Promisingly though, this issue can be resolved by having the function return the necessary JSX:
html
import React from ‘react’;
function MyComponent(): JSX.Element {
return
}
Or alternatively, simply by omitting the return type:
html
import React from ‘react’;
function MyComponent() {
return
}
John Resig, creator of jQuery famously stated, “Make it work first before you start optimizing”. So, remember to properly define each component’s functionality before diving into advanced topics like type annotations.
The correction addressed here alters the void type introduction to become another type (JSX.Element) or completely erases it. Thus, the return type harmonizes with what’s expected from a React Component i.e., ReactNode, thereby escaping the error condition.
Understanding the ins and outs of TypeScript type-safety in Javascript and React’s ecosystem is crucial, especially when creating reusable components or scaling your application. But, as we’ve seen here, the resolution doesn’t always need to be complicated.
Remember to make full use of the features TypeScript offers for a more bug-free and robust code.
As Alan Kay would say: “Most software today is very much like an Egyptian pyramid with millions of bricks piled on top of each other, with no structural integrity, but just done by brute force and thousands of slaves.”
So, avoid turning your codebase into a burdensome pyramid of bugs by understanding and fixing your type errors promptly. Don’t hesitate to take advantage of the clear-cut error messages that TypeScript provides.
It’s also suggested you dig deeper into TypeScript’s [official website](https://www.typescriptlang.org/docs/) to enlighten yourself on its powerful features and conventions when it comes to typing in JavaScript, especially focusing on ReactNode and void types.
You can check out this [article](https://fettblog.eu/typescript-react/components/) on using TypeScript effectively with React. It expands on best practices about describing component interfaces, handling props, using type safety with higher-order components, and many more topics pertinent to React and TypeScript collaboration.
The journey to becoming an established developer is an iterative process of learning, making mistakes, correcting them, and most importantly, growing with each experience. Make use of every opportunity to learn more, especially when dealing with the type-checking obscurities presented by TypeScript in React Apps. This will set a strong foundation for writing cleaner, safer, and more efficient code moving forward.
Best Practices for Avoiding the React Node Type Void Error
React Node Type Void Error: Best Practices for Avoidance
An error commonly encountered in React development environment is the “Error Type Void Is Not Assignable To Type Reactnode”. This occurs when a JavaScript function that returns nothing (void) is attempted to be used as a React component which should return a component tree otherwise referred to as
ReactNode
. In this context, let’s delve into some best practices for avoiding this error:
Create Functional Components Correctly
Ensure to structure your functional components appropriately. In JavaScript, a function that doesn’t have a return statement implicitly returns undefined. Therefore, always ensure that your React components render something or null.
Consider this simple example:
function HelloWorldComponent() { console.log('Hello world'); }
The component above won’t work as it returns void. To resolve the issue, you can make it return something valid like below:
function HelloWorldComponent() { console.log('Hello world'); return null; }
Take Advantage of TypeScript Type Annotations
By explicitly annotating function return types with TypeScript, it ensures the right type is returned from functions to stay in line with React’s expectations. For instance, use
ReactElement | null
instead of
void
.
Example:
const HelloWorldComponent: React.FC = (): React.ReactElement|null => { console.log('Hello World'); return null; }
Safeguard Against Possible Undefined Return Values
Understanding that some built-in JavaScript methods like Array.prototype.map() might return undefined also helps prevent unintentional void return values.
As Bill Gates once said, “Measuring programming progress by lines of code is like measuring aircraft building progress by weight.” Avoiding these errors isn’t just about code style or aesthetics, but a serious matter of guaranteeing the reliability and operability of software applications.source This understanding is key to developing error-free React components.
Insights into Common Causes of Void and ReactNode Errors
The error message “Type ‘void’ is not assignable to type ‘ReactNode’” is one of the common issues encountered by developers who use React, a leading JavaScript library for building user interfaces. This issue relates to the way JavaScript handles void and React handles Node.
Understanding The Cause Of The Error
React language uses its own data type: `ReactNode`, whereas, void is a fundamental JavaScript primitive that represents the absence of any return value from a function.
In JavaScript, `void` expresses an absence or non-existence of returns. A function declared as `void` can’t return anything, not even null or undefined. Here’s an example:
function helloWorld(): void { console.log("Hello, world!"); }
On the other hand, `ReactNode` is a commonly used data type in React. It’s meant to encapsulate “everything that can be rendered.” In essence, a `ReactNode` could be a `ReactElement`, `ReactFragment`, `string`, `number`, or another instance of `ReactNode`.
So, when you encounter this error, it means a JavaScript function returning a void type is trying to return a value which React -with its stricter typing- doesn’t recognize.
Looking at these descriptions, we can deduce that this error typically occurs when defining custom components or handling event handlers in React and incorrectly stating the void function type where a `ReactNode` should be.
Possible Solution To The Error
One way to resolve the problem is to ensure that all components and event handlers correctly return a `ReactNode` object. For functions not intended to produce a renderable output, their type declaration should reflect this.
For instance, if you’re creating a click event handler in React that logs the clicked element’s id to the console but isn’t supposed to return a value (i.e., it should be void), the function may look something like this:
function handleClick(event: React.MouseEvent): void { console.log(event.currentTarget.id); }
In this example, the event handler `handleClick()` correctly specifies its return type as `void`, as it is not supposed to return any renderable output.
As Donald Knuth, a computer scientist, once said, “Programmers are always surrounded by complexity; we can’t avoid it…Our task is to solve the puzzle.”
Consider carefully matching your functions’ return types with their intended outputs, and do not remain stuck with ‘void’ when you absolutely need to offer a ‘ReactNode’. This way, you’ll likely avoid the error message “Type ‘void’ is not assignable to type ‘ReactNode’.”
Key On-topic References:
Please note that multiple solutions may exist depending on the context of the issue within the code structure.
Breaking down the issue of “Error Type Void Is Not Assignable To Type Reactnode”, it essentially arises when there is an attempt to assign a value that does not have a renderable output to an object expected by React. The error implies that void or an unrestrained empty output cannot be set as a valid type in ReactNode.
To comprehend this further, let’s bring into vision ReactNode itself. It is essentially a type which fits any sort of React child, meaning anything that could be included inside tags while rendering components in a React component tree. This recourses string, number, JSX Element, arrays, fragments, and so on.
Type | Is Applicable? |
---|---|
String | Yes |
Number | Yes |
JSX Element | Yes |
Fragments | Yes |
Void | No |
Imagine under the hood in React’s world, where data validations are executed, whenever encountering a void type, it throws the error. The void descriptor struggles to draw a direct correlation with what needs to be displayed on user interface as part of
ReactNode
.
Refactoring is required to ensure the appropriate output format. If function used returns void, an exemption needs to be placed. Work around may include incorporating conditions to assign default values or display substitute material covering the void value rather than making the function return void directly.
Code variant would look like this helpful modification:
<div> {myFunction() || 'Default value or content'} </div>
This approach follows perceptive advice from eminent code maven, Robert C. Martin: “Leave the code cleaner than you found it.” With such a disciplined habit, we won’t just address this predicament but improve the overall quality and maintainability.
For a detailed millieu on dealing void in ReactNodes, head to stackoverflow. If not addressed, rendering issues may reflect on UI impacting user experience by intermittent display problems, making proactive intervention paramount.