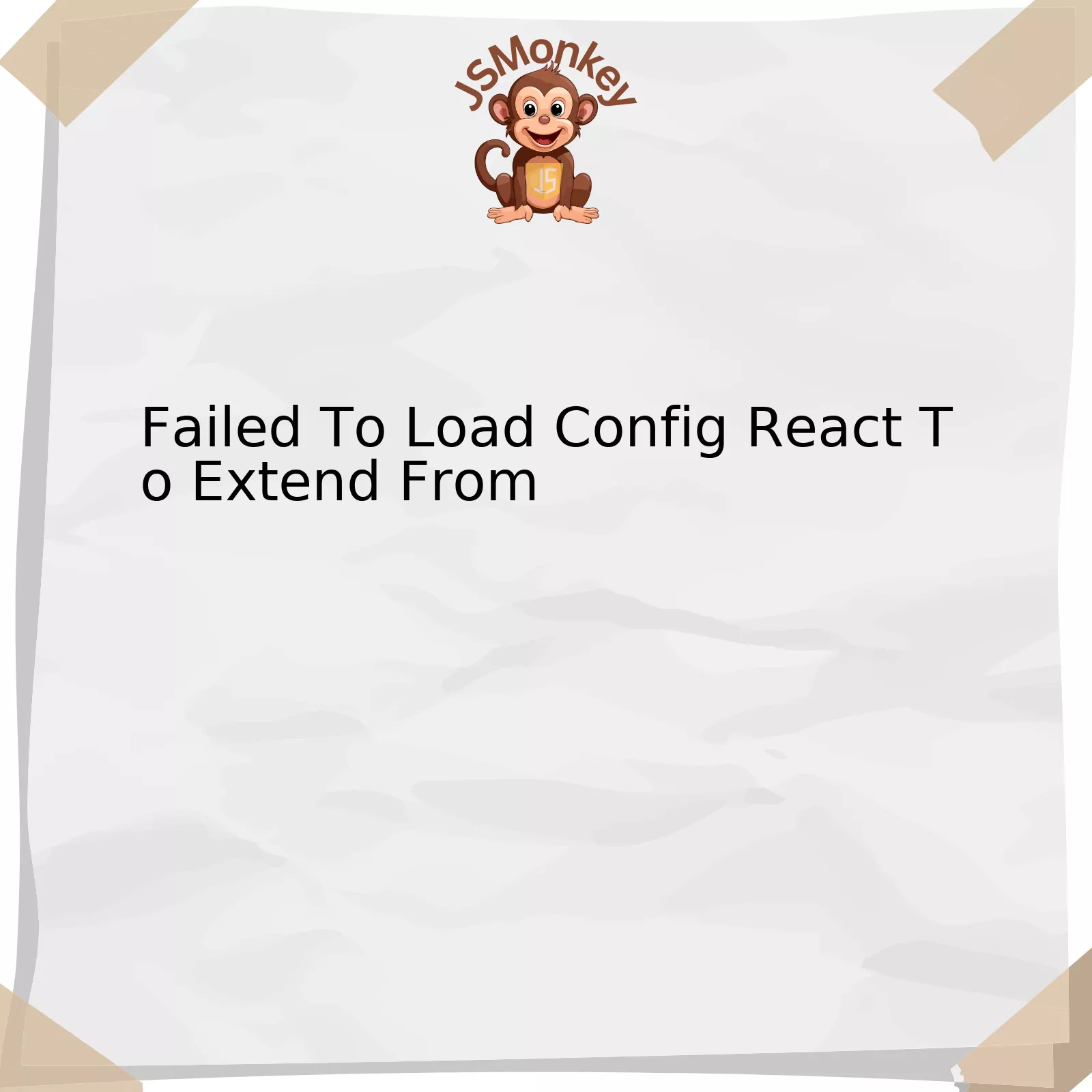
The “Failed To Load Config React To Extend From” error is often encountered during the setup or configuration phase of a React application. The primary reason behind this error message is specific to the improper configuration or incomplete installation of the ESLint plugin or the incorrect extension of ESLint rules.
Reasons | Solutions |
Absence of an ESLint plugin | Install the necessary ESLint plugins for your project. For example, you can use the npm command –
npm install --save-dev eslint-plugin-react |
Inaccurate configuration of ESLint rules | Ensure that the .eslintrc.js file includes accurate presets and plugins configuration. |
Dependency conflict | Check package.json for any discrepancies in versions or conflicts between dependencies. Use
npm install --update to update packages. |
Node modules not installed correctly | Delete node_modules folder and package-lock.json file. Then run
npm install . |
React is a very versatile library which allows the integration of multiple plugins such as ESLint to help maintain coding best practices. The ‘extends’ keyword in ESLint helps to provide a set of base rules which are then modified or overridden based on personal style requirements or team conventions.
If the error message mentioned above occurs, it indicates that the React configuration cannot be loaded or extended from – this could be due to the ESLint configuration error. This situation may arise in cases where there’s an absence of certain ESLint plugins or a conflict of versions between dependencies.
To rectify this situation, it is crucial to ensure that you have correctly installed all necessary ESLint plugins for your project. Incorrect extension of ESLint rules might also cause such errors; hence, making sure .eslintrc.js (ESLint configuration file) includes accurate presets and plugins configuration is essential.
Sometimes, it’s possible that your node modules are not installed correctly or there might be some discrepancies in your `package.json` causing dependency conflicts. In such cases, deleting the node_modules folder and the `package-lock.json` file and rerunning npm install often fixes the problem.
Quoting the father of Javascript Brendan Eich, “Always bet on JS”. Regardless of any temporary hurdles during setup or configuration, Preact’s flexibility and wide community support offer easy solutions and workarounds.Reference
Understanding the “Failed to Load Config React” Error
The “Failed to Load Config React to Extend From” error is among the common challenges that developers come across when working with a JavaScript library known as React. This error usually arises due to the configuration settings made for the project that might be either incorrect or incomplete. However, it’s crucial to understand the root cause of this issue and find an appropriate solution.
While setting up a project with React, developers extend ESLint configurations by using different plugins or shareable configurations. You might encounter the error message if the setup process was not executed correctly or if the needed dependencies were not installed.
Example of the error message:
Failed to load config "react" to extend from
Overall, three main reasons explain why you could be experiencing the “Failed to Load Config React to Extend From” error:
Firstly, lack of installation or incorrect installation of necessary packages such as eslint-plugin-react or eslint-config-react-app could trigger this error. These two packages provide essential rules that enforce React best practices.
Secondly, improper referencing of package names in your project’s ESLint configuration file (.eslintrc file) might cause the error. To rectify this, ensure the correct name of the package is used that matches precisely with the one listed in your package.json file.
Lastly, the presence of multiple versions of ESLint in your project might also lead to the “Failed to Load Config React to Extend From” error. Having multiple versions of ESLint can cause confusion during the interpretation process, hence triggering the error.
To troubleshoot and resolve this error, follow these steps:
– Check whether all the required packages are installed in your project.
npm install eslint-plugin-react eslint-config-react-app --save-dev
– Verify the names of the extended packages in your .eslintrc file. They should match the ones in your package.json file.
– Uninstall duplicate versions of ESLint from your project to maintain one version.
npm uninstall eslint
npm install eslint --save-dev
The challenge of solving coding errors is universal, and it reminds me of a quote from Thomas Edison: “I have not failed. I’ve just found 10,000 ways that won’t work.” So keep trying until you find your solution!
For more in-depth knowledge on this topic, feel free to check the official ESLint Configuration Files guide.
Troubleshooting Steps for the “Failed to Load Config React” Issue
The “Failed to Load Config React” error is a common problem encountered by Javascript developers when configurations do not load as expected within the context of React.js projects. This issue often arises when attempting to extend configurations.
Troubleshooting Steps
The causes of this issue may vary, but here are a few potential reasons and corresponding steps to resolve them:
1. Inadequate or Incorrect ESLint Configuration:
ESLint, an open-source JavaScript linting utility, aids in maintaining code quality. If it’s not configured correctly, it could result in the “Failed to Load Config React” error message (ESLint User Guide). To solve the problem:
- Ensure that ESLint is installed and working correctly in your development environment.
- Verify the paths provided to the key ‘extends’ in the ESLint configuration file (e.g., .eslintrc).
“Talk is cheap. Show me the code.” Linus Torvalds
Consider this example:
{ "extends": ["react-app", "react-app/jest"] }
If the paths mentioned above are correct, then ‘react-app’ & ‘react-app/jest’ should be available within your node_modules folder.
2. Misconfigured Node Modules:
Node modules play a pivotal role in building JavaScript applications. One possible cause of this error might be due to misconfigured or missing required modules. Here is how to handle this aspect:
- Delete ‘node_modules’ folder and ‘package-lock.json’ files from your project directory. Then run
npm install
to reinstall all modules again.
- If the issue persists, inspect your ‘package.json’ for any deprecated or absent react scripts, modules & configs and install/update as needed.
Example:
npm uninstall eslint-config-react-app
And then
npm install eslint-config-react-app
3. Versioning Problems:
In some cases, the error in question could be due to version compatibility issues among React, ESLint, Babel ESLint, etc. Downgrading or upgrading specific modules according to the software requirements may resolve this issue. Please refer to official documentation and community forums to find the recommended versions (React Official Documentation).
4. Global versus Local Package Conflicts:
Another reason for such an error might be due to conflicts between globally installed and locally installed packages. Give precedence to local installation and ensure that ‘eslint’ and ‘eslint-config-react-app’ are stated in your devDependencies.
The approaches discussed should primarily help you tackle the “Failed to Load Config React” error. As with all problem-solving strategies in coding, it’s a step-by-step process, and perseverance is key to success.
Exploring Root Causes: Why Does ‘Config Fail To Load’ in React?
There could be several reasons why the configuration fails to load in a React application. Sometimes, it happens when React attempts to extend from a non-existing or incorrect configuration. The configuration that React is trying to inherit from (via “extends” in your .eslintrc file) may not exist, may be spelled incorrectly, or may be unreachable because of the folder structure.
Cause | Remedy |
---|---|
"extends" refers to a non-existant configuration |
Make sure the configuration you’re referring to in
"extends" exists and is spelled correctly. |
"extends" entry is mis-spelled. |
Double-check spelling and case-sensitivity in your configuration files. |
Configuration is unreachable due to folder structure. | Revisit your project’s folder structure. Ensure that, relative to your .eslintrc file, the path to the extended configuration is accurate. |
Moreover, issues like this can also occur due to version mismatches between modules. If one module updates and others do not, it can lead to unexpected problems including the ‘Config Failed To Load’ error. Always check if all your packages are up-to-date.
The famous tech influencer Martin Fowler once said:
“If you can’t write it down in English, you can’t code it.”
This quote applies to .eslintrc configs as well. Allowing the system to read invalid configurations will certainly trigger exceptions like these.
Depending on the issue, you can try reinstalling your node modules, update your dependencies, reconfigure your settings, or even look into other suggestions made by the online React community.
A good practice is to check first the official React documentation which provides troubleshooting tips for a variety of common mistakes and errors that occur during development. You can also explore online forums, such as Stack Overflow or GitHub issues, where you may find solutions from other developers who experienced the same problem.
Understanding the root cause of why ‘Config Failed To Load’ in a React application might demand a fair amount of patience and debugging but it’s an integral part of programming life. In such situations, always remember the words of inventor Thomas Edison who said:
“I have not failed. I’ve just found 10,000 ways that won’t work.”
So don’t be frustrated with these sorts of problems, instead take them as opportunities to learn and grow your tech expertise further.
Effective Solutions for Resolving ‘React Failed to Load Configuration’ Problems
To address issues such as “Fail to Load Config ‘react’ to Extend From,” it’s essential to understand what this error means and how to implement effective solutions. This error typically emerges when the ESLint configuration for React is not correctly set up in your project. ESLint enhances JavaScript code quality by identifying and fixing problematic patterns, bugs, or non-adherent coding standards. As such, a missing or incorrect ESLint configuration frequently triggers the error message.
Diagnosing the Problem:
The problem generally arises due to either of the following circumstances:
– Non-installation or improper installation of ‘eslint-plugin-react’
– Incorrect reference to the React configuration
Solution Measures:
Here are two efficient steps that could potentially resolve the issue:
1. Installation or Reinstallation of eslint-plugin-react. Missing ‘eslint-plugin-react’ can be the causes of the problem. Therefore, you can get started by installing or reinstalling the ‘eslint-plugin-react’ package. It can be added via npm or yarn depending on which package manager you’re utilizing. Here’s the npm install command:
npm install eslint-plugin-react --save-dev
And for yarn:
yarn add eslint-plugin-react --dev
After executing one of these commands, the plugin should be added to your devDependencies in your package.json file.
2. Proper Configuration of the Config File. In cases where the error continues to persist despite the ‘eslint-plugin-react’ being in place, it’s worth considering checking the ESLint configuration. Ensure the extension of eslint-config-react-app is correctly referenced.
For instance, let’s consider modifying the .eslintrc.js file:
module.exports = { "extends": ["react-app", "plugin:react/recommended"], "plugins": ["react"] }
Note that the “plugin:react/recommended” configuration is added to the “extends” array and the “react” plugin is included in the plugins array – this demonstrates a correct reference to eslint-config-react-app.
It is worth citing Kent C. Dodds, an experienced JavaScript developer who once said, “code quality tooling should assist developers and not get in their way.” Thus by correctly installing ESLint plugins and setting up configurations, these tools can help maintain code quality without hindering the development process.
The underlying theme here is that troubleshooting any coding challenge involves understanding the problem first, exploring possible reasons behind it, and adopting analytical approaches for resolution. I hope this explanation will help many developers resolve the ‘Failed to Load Config React To Extend From’ error efficiently.ESLint: Configuring Plugins.
To speak on the technical issue of “Failed To Load Config React To Extend From,” one must get to the root of the problem which typically originates from a variety of sources. Such error messages are commonly encountered when trying to set up or work with a React project, emanating from mismatches existing between installed dependencies.
npm install eslint-config-react-app babel-eslint@9.x eslint@5.x eslint-plugin-flowtype@2.x eslint-plugin-import@2.x eslint-plugin-jsx-a11y@6.x eslint-plugin-react@7.x
This command can help to resolve such issues by ensuring that your installation includes the correct versions of requisite dependencies for React.
However, while rectifying these mismatches is essential, equally as crucial is understanding the underlying mechanisms that cause this error. React applications depend heavily on an array of packages and dependencies which need to intercommunicate seamlessly. Whenever there’s a mismatch in the configurations as they load, it results in errors like “Failed To Load Config React To Extend From.”
Therefore, an efficient way to combat this issue is maintaining updated packages and using suitable version control strategies. This ensures that incompatible versions do not get into your dependency tree. Maintaining strict control over packages and dependencies might seem daunting initially, but in the long run, it aids in preempting potential issues such as “Failed To Load Config React To Extend From.”
Renowned software engineer Martin Fowler once proclaimed, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand”. Hence, part of writing good code requires acknowledging and efficiently dealing with errors like “Failed To Load Config React To Extend From” rather than sweeping them under the rug. Handling them properly leads to more robust, reliable, and less buggy code.
For further information on similar topics, consider referencing official React documentation via this link: React Documentation.