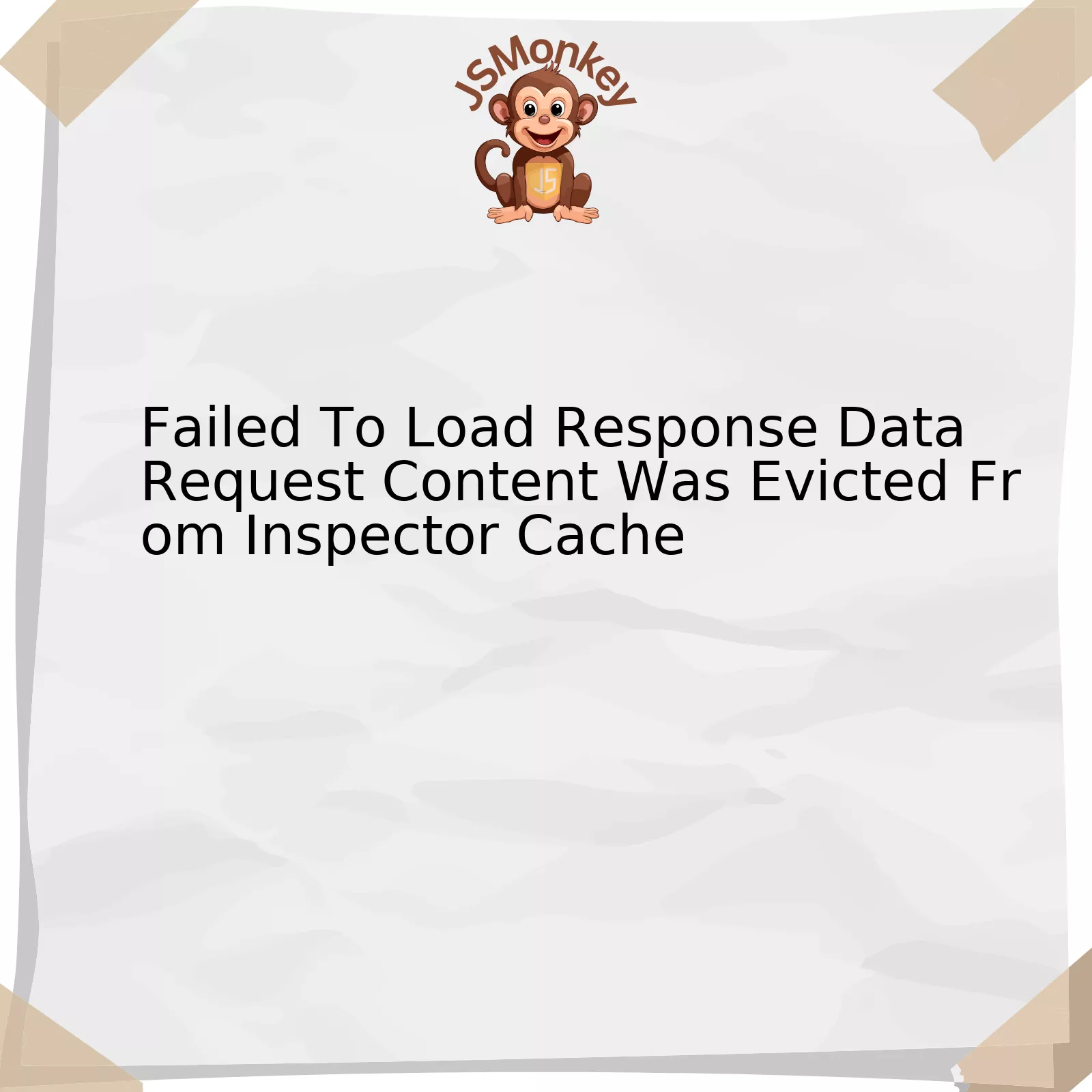
When you encounter the error message “Failed to Load Response Data – Request Content Was Evicted From Inspector Cache,” it implies an issue that obstructs the web inspector from storing and displaying the response data from a particular request. The request content is evicted or excluded because the cache storage limit has been reached.
Issue | Explanation |
---|---|
Eviction of Request Content | The browser’s DevTools console caps the total amount of data it can store. This includes all network requests and responses. When this limit is reached, older and non-essential information is “evicted,” or removed from cache storage to retain newer data. |
“Failed to Load Response Data” error | This error signifies that the requested content was among the data which has been evicted from the cache. Hence, you’re unable to view the requested data in the Developer Tools Network Tab as it no longer exists in the cache storage. |
The Google Chrome DevTools console, for instance, limits the size of logged data (requests/responses) to 20MB (as of Chrome 84). As such, if you have lengthy sessions with several network calls, the capacity of the cache might be exceeded, resulting in eviction. It’s not an application bug or a browser defect.
There’s limited action you can take to avoid seeing this error. However, there are few steps you can take to ensure vital data isn’t lost:
- Use ‘Preserve log’ checkbox: This option allows you to save all network requests between page loads.
- Frequent Refresh: Regular refreshing prevents the console from reaching its maximum storage limit.
- Filter Responses: This helps you reduce the retrieved data by focusing on responses you’re interested in, thus reducing the amount of network data saved.
If your primary intention is to monitor a specific type of request and response, consider deploying automated testing tools. Tools like Selenium WebDriver or Puppeteer can help execute this task more efficiently without worries about manual errors or information loss.
As Steve Jobs once said, “Simple can be harder than complex: You have to work hard to get your thinking clean to make it simple.”. This quote sufficiently illustrates that simplicity may be the key in managing response data efficiently within your web inspector.
Understanding the Impact of Cache Eviction on SEO
Cache eviction plays a crucial role in web development, especially from an SEO perspective. Whenever a cache eviction takes place, it implies that data initially stored temporarily for quick retrieval is being deleted from the system’s memory cache. This event typically occurs due to overflow issues or to accommodate newly fetched data.
When discussing the specific issue of “Failed To Load Response Data: Request Content Was Evicted From Inspector Cache”, we are looking at a situation where some data intended to be displayed on a web page was evicted from a browser’s inspector cache. This could occur due to:
– An accumulation of too much data forcing the browser to free up some space.
– Manual cache cleaning by a developer or user.
– Inadvertent clearing by an automatic cleaning tool or process.
The Implications
Cache eviction can inadvertently impact the website’s function and experience, subsequently influencing its SEO (Search Engine Optimization) standing. Here’s how:
1. Slower Website Performance: When the cached data is evicted, pulling information directly from servers affects the speed and responsiveness of a site. As speed is a ranking factor for search engines like Google, slower load times may lower the website’s SEO rankings. Google’s research indicates that as page load time goes from one second to ten seconds, the probability of a mobile site visitor bouncing increases by 123%.
2. Increased Server Load: Fetching data afresh each time places an additional demand on your server, thus increasing its load. This might result in even slower loading times if many users access the content simultaneously.
3. Spoiled User Experience: If the cache eviction results in failed requests and the delivery of partial content to the browser, which subsequently leads to errors such as “Failed To Load Response Data” being displayed on the page, the user experience will be negatively affected. Google has made it clear that a positive user experience is vital for good ranking in its search algorithm.
Here’s an example of how you might handle caching and eviction for better SEO:
// Fetch API request fetch(myURL, { cache: "no-store", // this directive ensures the request will not be fulfilled from cache method: 'GET', headers: { 'Content-Type': 'application/json' } })
The simple quote from Mitchell Hashimoto, the co-founder of HashiCorp, perfectly encapsulates this principle: “The most important thing with software is making the complex simple”. So, strive to deliver content efficiently, with speed and simplicity, for a great user experience which positively impacts your SEO.
Carefully handling cache eviction and ensuring optimal website performance are critical aspects to consider. The recent shift towards Core Web Vitals optimization underscores the need for more focus on user experience optimization not just for SEO, but for the overall success of web-based platforms.
Remember to consistently monitor and scrutinize your site’s performance. As scenario demands, make use of cache policies to control eviction and experiment when necessary. By staying vigilant, you can help ensure smoother, faster loading times, better user experiences, and improved SEO outcomes.
Analyzing How to Prevent Content Eviction from Inspector Cache
Let us get started on the topic of “Failed To Load Response Data Request Content Was Evicted From Inspector Cache”, specifically in the context of preventing content eviction from Inspector cache.
The inspector cache is a crucial component of developer tools in various web browsers, including Google Chrome and Mozilla Firefox. Its primary function is to store information about network requests, such as HTTP headers, request parameters, and response data. This aids developers in debugging network-related issues.
However, in certain scenarios, you may receive an error message indicating that the request content was evicted from the inspector cache. The content eviction typically happens when there’s a limitation on resources (like memory), and the browser decides to free up some space to make room for more recent or relevant data.
Inspector Cache | Role | Reason for Eviction |
---|---|---|
Browser Developer Tools | Stores network requests information | Memory constraint/Limited resources |
To avoid this situation, consider the following strategies:
* Maintain Freshness: Keep refreshing the page. Many browsers, like Chrome, can delete cached data each time you refresh.
* Set Persistence: Explicitly set the network log to preserve log info in your console settings. In Chrome, this option can be toggled under the Network tab.
* Reduce Payloads: If feasible, minimize the size of the assets you’re working with. Smaller responses will consume less memory.
It’s worth noting, however, that these strategies might not always work as some browsers may still choose to perform eviction even if these measures are in place.
I will leave you with a quote from Grace Hopper, one of the pioneers of computer programming: “To me programming is more than an important practical art. It is also a gigantic undertaking in the foundations of knowledge.”
Do not mistake evictions for an error or malfunction; it is part of the browser’s mechanism used to manage resources effectively. While we can work around it to some extent, it is a built-in functionality that exists for a critical reason.
If developers are frequently encountering content eviction issues, they may need to reevaluate their coding practices or consider switching to a developer tool with more robust caching mechanisms. Examining the size and frequency of network requests could provide insights into how data management might be improved.
More information about Inspector Cache can be found on Chrome Developers official site.
It’s crucial to remember these strategies when dealing with an event where the requested content was evicted from the inspector cache. Being proactive and understanding why content eviction happens can prevent this issue from becoming a recurrent problem.
Methods to Optimize Website Performance Post-Cache Eviction
Website performance optimization is a core aspect of front-end development in terms of ensuring user experience and satisfaction. Post-cache eviction may involve the deletion or removal of certain data items from a cache memory to allow for new entries. However, this process can lead to an error known as “Failed To Load Response Data Request Content Was Evicted From Inspector Cache”. This refers specifically to a situation where the browser’s DevTools couldn’t retrieve any required HTTP response data because it was erased from the inspector cache.
Coming at this issue from the angle of JavaScript development, here’s how we could go about tackling this:
Optimizing Server Responses
The very first step towards improving your website’s efficiency post-cache eviction would be through optimizing server responses. A developer may leverage various HTTP headers keys and values to manage caching directives on the client-side. For instance, setting proper
Cache-Control
,
Pragma
, and
Expires
header fields.
“Latency will dwarf bandwidth at some point.” – Steve Souders
This quote illustrates the importance of effective server responses. Reducing latency by implementing effective server responses ultimately bolsters website performance.
Avoid Oversized Cookies
Cookies are often used to personalize user experiences, but overuse can result in excessive data being sent with every HTTP request causing delays. If you need to save substantial client state data, consider using sessions or local storage instead.
localStorage.setItem('key', 'value'); let data = localStorage.getItem('key');
Data Minification
Minifying CSS, HTML, and JavaScript files can effectively save bandwidth and speed up loading times. This process involves removing all unnecessary characters without changing its functionality.
Lazy Loading
Implement lazy loading to delay the initialization or loading of assets until they’re needed. This could be particularly useful for images, large datasets, or any heavy UI elements that don’t need to appear right away when a page loads.
Remember, being aware of cache eviction and managing it efficiently will lay the foundation for an optimized web experience. Each method mentioned holds its unique prowess in improving website performance post-cache eviction, especially after a “Failed To Load Response Data Request Content Was Evicted From Inspector Cache” error. It’s about working out which strategies are most relevant and effective for each individual site or application.
Strategies for Recovering From a Failed Response Data Load
Facing the challenge of “Failed To Load Response Data” or “Request Content Was Evicted From Inspector Cache” can be frustrating for any JavaScript developer. This is a situation that generally arises when the inspected data is no longer present in memory allocated to Chrome DevTools. Essentially, these messages indicate that the response payload of a network request has been evicted from the browser to save memory.
Though this phenomenon may complicate debugging efforts in certain scenarios, there are several approaches one can adopt to mitigate its effect and ensure a smoother data recovery:
Prevention of Automatic Cache Clearance
The straightforward solution lies in preventing automatic cache clearance. Chrome’s DevTools settings allow you to bypass the cache while DevTools is open. By using shift+reload, you can forcefully reload the page and capture the full data load WITHOUT the auto-clear feature. This way, it helps in capturing that elusive information.
// You can find this setting in: // DevTools > Settings > Preferences > Network > Disable cache (while DevTools is open)
Persistent Fetch Results
Another strategy involves leveraging the Fetch API which is built into modern browsers. This API keeps the results persistently as compared to other regular network requests.
Using the fetch API, the responses can be kept without being cleared instantly. Here is an example of how you can use the Fetch API on your own:
// A typical fetch request fetch('https://example.com/data') .then(response => response.json()) .then(data => console.log(data));
Downloading & Offline Analysis
In some situations, the responses might be too large for the browser to handle effectively. In such cases, you can download the response directly from the network tab, allowing for offline analysis at a later stage. This is especially useful if you’re dealing with larger json responses that can cause the browser to slow down or crash.
“Testing leads to failure, and failure leads to understanding.” – Burt Rutan (Aerospace Designer)
Server Logs
If all else fails, there’s always the more traditional method of resorting to server-side logging. By keeping an accurate log of data sent and received on the server side, one may cross-check the expected response whenever a ‘request content evicted’ error occurs.
MDN Web Docs – Fetch API offers more insight into using the Fetch API correctly for debugging and other purposes.
Additionally, consider running your tests in controlled environments to regularly capture and review all network traffic. This can greatly assist in understanding the sequence of events leading up to an unexpected eviction event, thus providing important cues for response recovery strategies.
The challenge presented by the phrase “Failed To Load Response Data, Request Content Was Evicted From Inspector Cache” represents a commonly encountered issue in the world of Javascript development. When coming across this issue, developers are often left bewildered and seeking in-depth insights and solutions to tackle it.
Fundamentally, this problem surfaces when response data fails to load. The reason? Request content being evicted from the inspector cache. In simpler terms, when an inspector such as DevTools is open, it caches network responses. However, owing to memory management and efficiency, it may occasionally discard older entries to accommodate new ones, resulting in the specified error message.
Resolving this occurrence necessitates a few key steps:
– Close and reopen DevTools: This can trigger the freshly collected and stored response data.
– Increase allotted cache storage: Manipulating settings to allow storage of larger amounts of network request data may prove beneficial.
Probable cause | Solution |
---|---|
DevTools discards cached data to save memory | Reopen DevTools or tweak settings for larger data storage |
Consider what Brian Kernighan, one of the pioneers of early programming languages once said: “Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.”
With that in mind, understanding how the inspector cache works, anticipating potential issues like Request Content Evictions and taking proactive steps to manage cache storage can prevent stumbling upon the ‘Failed to Load Response Data’ notice. By heeding these tips, one can experience a smoother, more productive coding environment in their JavaScript development journey.
To further enrich your understanding, the depth of resources available online such as MDN Web Docs and various developer forums can be valuable tools to leverage. They not only provide step-by-step solutions but also an abundance of insights and best practices to better navigate the Javascript landscape.