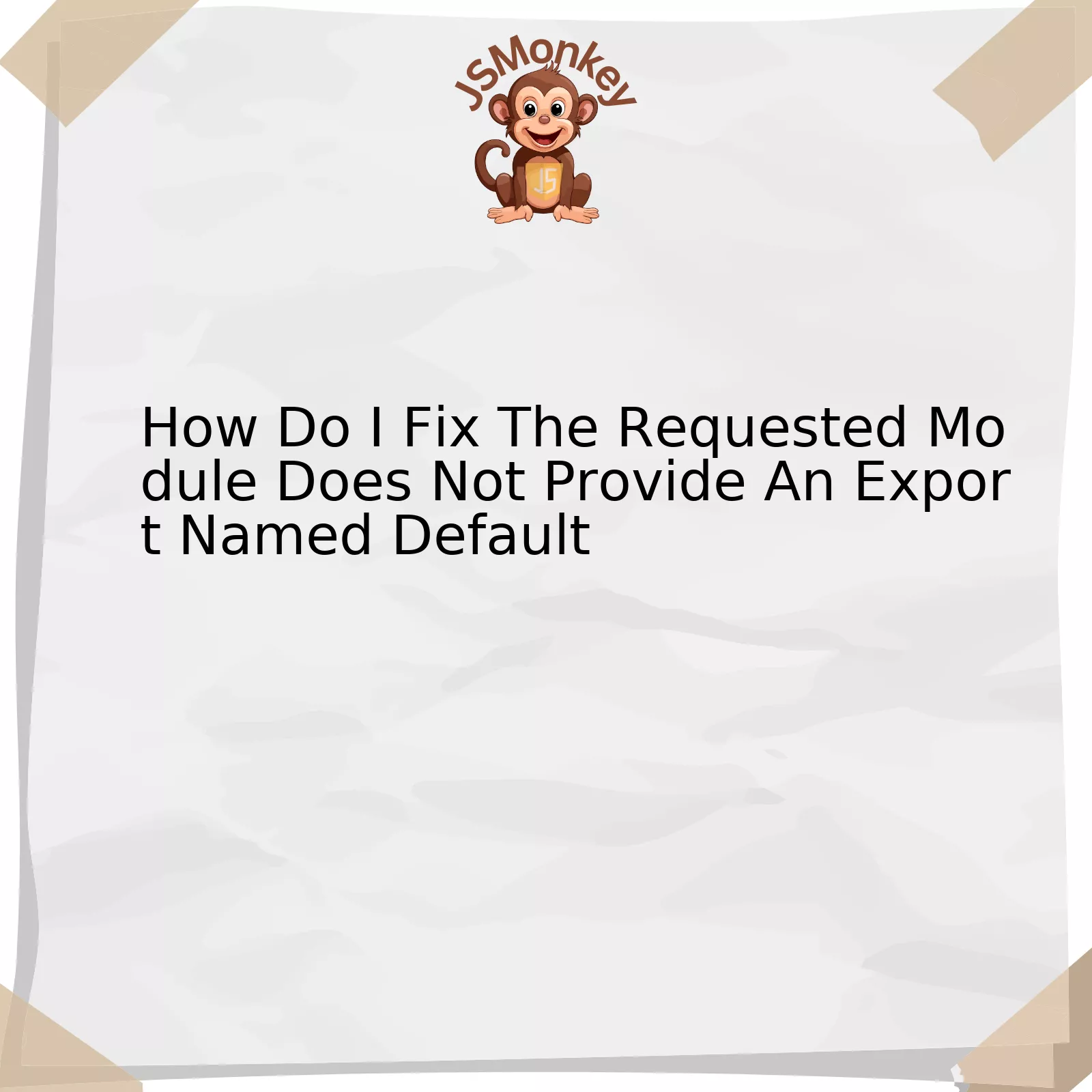
Having trouble with the ‘Requested Module Does Not Provide An Export Named Default’ error is common amongst JavaScript developers. When you face this message, it essentially means that your code is trying to import a default export from a module that doesn’t have one.
There are several reasons why this issue may come up:
Reason | Solution |
---|---|
The imported module does not have a default export in its code | Review the module file and ensure there’s a default export. If not, change the import statement in your code or request the owner of the module to consider exporting. |
The file containing the main entry point for the module is incorrect | Ensure the main field in the package.json file is pointing to the correct file which exports the required functionality. |
The module uses a feature incompatible with your project setup, e.g., CommonJS together with ES6 modules. | Consider converting all imports/exports into one type, either ES6 modules (import/export) or CommonJS (require/module.exports). |
Now let’s delve a little deeper into these issues:
– A primary cause stems from an absence of default exports inside the imported module code itself. Without such exports, the error will rise when other files attempt to import them. The solution is to add a default export to the module. For example, a default export in JavaScript looks like this:
export default myFunction;
If for some reason adding a default function to the specific module is not ideal or possible, changing how you import that module would be another approach. Instead of using the default syntax, use a named import:
import { functionName } from './moduleName';
– Sometimes, the main field in the package.json file points to an incorrect or nonexistent module file. This could lead to issues when your project attempts importing functionality. The fix is to adjust the ‘main’ field by pointing it towards the correct entry point for the module.
– Issues also arise if you are trying to consume ES6 modules in a CommonJS context, or vice versa. Mixing module type isn’t recommended – consistency will help avoid headaches with compatibility and syntax. Look through your codebase to ascertain which style is prevalent, then convert your import/export statements according to this style.
As Bill Gates once said, “I choose a lazy person to do a hard job. Because a lazy person will find an easy way to do it.” Correctly structuring and organizing your JavaScript code can help you catch any export/import errors before they occur, hence saving you troubleshooting time down the road.
Understanding “The Requested Module Does Not Provide an Export Named Default” Error
The message: “The requested module does not provide an export named ‘default'”, fundamentally arises while importing a JavaScript module when there is no default export found within the referenced module. The JavaScript ES6 implements two forms of export statements, specified as:
1. Named Exports: Where more than one component can be exported and has to be imported using the exact names encapsulated inside curly braces `{ }`. It would look like this:
`
export let variable1 = value1;
`
2. Default Exports: Only one component per module can use default export and it could be imported without specifying its original name. Sample representation would be:
`
export default functionOrVariableName;
`
Thereby, if a module doesn’t contain any `export default`, any attempts to default import it will lead to the error in question.
In order to decipher the issue and mitigate the “requested module does not provide an export named ‘default'” error, you should consider the following steps:
– **Cross-check Your Import Statement**: Make sure you are referencing the correct file path in your import statement. Rectify any possible mistakes pertinent to case-sensitive issues or wrong filename.
– **Validate Export Syntax**: Ensure the respective module where the problem is being flagged contains the necessary `export default` declaration. If overlooked, simply update the code to add this key directive.
When correcting the error, for instance, upon the scenario that DetailModel.js exports only named exports such as:
// DetailModel.js
const details = {...};
export { details }
Then we must make corrections to the import statement in our main file, possibly titled DetailsPage.js:
// DetailsPage.js
import DetailsModel from './models/DetailModel'
This needs to be modified to import DetailsModel as a named import:
// DetailsPage.js
import { DetailsModel } from './models/DetailModel';
Another way is to simply transform the named export to a default one, should `DetailsModel` be the main point of concern in our module:
// DetailsModel.js
export default details;
Using JavaScript to harness modules and maintaining clean code can be notably daunting. To echo Steve Jobs: “Simple can be harder than complex: You have to work hard to get your thinking clean to make it simple. But it’s worth it in the end because once you get there, you can move mountains”. To have effective usage along with simple, lean maintenance and debugging, sticking to the rules of named and default exporting/importing becomes crucial. We must adhere to principles of detailed importing/exporting nomenclature to keep our JavaScript code effective, reliable, and sustainable. One might need to cross-verify each step and make sure all coded elements are synchronized and in precise correlation to their respective calling pieces.
Troubleshooting Steps to Fix the Module Export Issue
There are several key measures that you can take to resolve the “Requested Module Does Not Provide an Export Named Default” issue. This error typically occurs in JavaScript development when there’s an attempt to import a default export from a module, but the module doesn’t have a default export defined.
Here are actionable steps to help you troubleshoot this problem:
Step 1: Verify The Module
Ensuring the existence of the module you’re trying to import from is essential. If it does not exist or has been incorrectly named, the default export error is likely to occur. Additionally, check and confirm that your path to the module is correct.
“As developers, we must treat debugging problems as a detective would an investigation. Tease apart the clues, piece together the puzzle.” – Anonymous
Step 2: Check The Syntax
JavaScript ES6 introduced modules and with them, a new syntax for importing and exporting functions, objects, or values. Therefore, make sure you’re using the correct syntax for named exports and default exports.
// INCORRECT - trying to import a default export that doesn’t exist import Something from './modules/yourModule.js'; //CORRECT - importing named export import { Something } from './modules/yourModule.js';
Step 3: Confirm The Default Export
To import something as a default export, it should have been exported as default from the module initially. But if a value hasn’t been defined as a default export in a module, it cannot be imported as one. You can fix this by going to the module and either adding a default export or changing your import statement to reflect a named export, which does exist.
Step 4: Compatibility Issue
Ensure that there aren’t compatibility issues between the JavaScript versions in the project. Some ES6 features, such as modules, may not be supported in older engines. It’s prudent to identify if this is a potential compatibility issue and to use Babel or another transpiler to resolve it.
Step 5: Update Dependencies
Sometimes, having outdated dependencies causes this error. An update could help, as newer versions of dependencies typically fix bugs and compatibility issues that might have been causing the “Requested Module Does Not Provide an Export Named Default” error. Use npm or yarn to update your dependencies.
These steps should provide a comprehensive method for resolving the error. It might require a little bit of patience and critical investigation but ultimately will improve the stability and effectiveness of your JavaScript development experience.
Remember to always understand how your code works, specifically regarding how modules export and import values in the context of JavaScript.
Exploring Common Causes for the ‘Module Does Not Provide an Export Named Default’ Problem
Navigating the issue of ‘Module Does Not Provide an Export Named Default’ essentially revolves around understanding how ES6 modules function, and correctly employing the import and export syntax. This problem typically surfaces when there’s a mismatch between exporting an item as a default from a module and then importing that default elsewhere.
Understanding ES Modules
ECMAScript 6 (ES6), also known as ECMAScript 2015, introduced a standardized module format for JavaScript, enabling developers to dynamically load modules at runtime without the need for libraries like RequireJS or CommonJS. These ES6 modules feature two primary methods for exporting contents: named exports and default exports.
• Named Export: With this method, multiple exports within a module can be accomplished. Here’s an example:
export const name1 = "..."; export const name2 = "...";
And these exported variables can be imported like so:
import {name1, name2} from "./moduleName";
• Default Export: Every module can have one, and only one, default export. For example:
export default "...";
The default export can be imported using a simple syntax:
import moduleName from "./moduleName";
How To Fix ‘Module Does Not Provide an Export Named Default’
This error generally arises from trying to use the default import syntax with a module that hasn’t designated anything as a default export. Essentially, it’s a miscommunication related to exporting and importing rules in your code base. Here are some solutions:
First, verify the module you’re trying to import. Does it contain a valid ‘default’ export? If not, this could be the root cause of the error message.
If the module does indeed contain a ‘default’ export but the issue persists, it may be due to using a different version of ECMAScript in your environment than the one used when the module was written. ECMAScript 2015 has strong support in modern environments but can run into compatibility issues with older environments.
It’s also beneficial to consider if there are any required Babel presets or plugins needed for your code to transpile correctly. These tools can help rectify the mismatch between import and default export syntax.
Throughout your delve into resolving this issue, remember these wise words from Bill Gates: “The computer was born to solve problems that did not exist before”.
Your path to fixing ‘Module Does Not Provide an Export Named Default’ lies in cementing a deeper understanding of import/export syntax in ES6 modules, ensuring you’ve correctly incorporated this syntax in your project, and verifying your codebase uses a JavaScript version compatible with ECMAScript 2015.
Implementing Solutions and Preventive Strategies for Module-Export Related Problems
As a JavaScript developer, dealing with module-export related problems can sometimes become challenging. Often you will encounter an issue stating: “The requested module does not provide an export named ‘default'”. Such obstacles can disrupt the workflow and be a source of frustration. But fear not, as reflecting upon solid solutions and preventive strategies can lead us towards smooth execution.
Understanding the Problem
This error suggests that we are trying to import a module using a default import syntax, but the module does not have a default export. The JS module system essentially includes two kinds of exports –
named exports
and
default exports
. While a module can contain multiple named exports, it can only have one default export.
“Make things as simple as possible, but not simpler” – Albert Einstein.
Resolving the Issue
Primarily, your solution lies in understanding how the required module is being exported. Let’s begin with these simple steps:
• Start by reviewing the module you are importing from. If the module doesn’t utilize a default export, then you need to adjust your import syntax accordingly.
// Incorrect syntax import MyModule from './moduleName.js'; // Correct syntax if no default export exists import * as MyModule from './moduleName.js';
• If the module explicitly uses `export default`, you can use the default import syntax. Alternatively, for modules exporting functions or variables, use the syntax `{ myNamedExport }`.
Preventive Strategies
Errors like these act as catalysts to enhance our programming competency. By adopting some preventive strategies, we can fortify our coding approach.
• Familiarize yourself with JavaScript Export Statements. This knowledge can go a long way in helping you comprehend what you are importing and how to do it effectively.
• Use IDE plugins or linters like ESLint that warn you about these common mistakes before runtime. These tools significantly increase your code’s robustness and save investigating time by spotting probable errors during development.
Reconsidering Multiple Exports
Multiple exports in JavaScript provides flexibility. However, as coding becomes verbose and more susceptible to errors, adhering to the principle of ‘simpler is better’, using a singular, default export per module promotes cleanliness and minimizes error proneness.
References:
MDN Web Docs – Export
ESLint
Understanding and rectifying the error – ‘The requested module does not provide an export named default’ can indeed be a complex task. This particularly occurs when dealing with JavaScript ES6 modules where one tries to import a library that doesn’t have a default export. It’s crucial to note here that, in JavaScript, every file is considered a separate module and the variables and functions defined cannot be accessed directly from other files (or modules).
To address this issue we need to follow some critical steps:
– We should ensure that we are exporting components, objects, variables, or functions correctly by using `export default
`. - When importing, make sure to use the correct syntax: `import object from ''`. This assumes that the module you're importing has a default export. - If there is no default export, use `import * as object from ' '` which imports all named exports from the given module into the `object`. - Make certain your configurations are accurate especially with JavaScript bundling tools like Webpack, Parcel, Babel, among others. They generally handle imports/exports for you, permitting to seamlessly write ES6 code. Regarded as a cornerstone of JavaScript, Modules enhance reusability, maintainability and organization of code in a large-scale application. Bill Gates once said, "A great lathe operator commands several times the wage of an average lathe operator, but a great writer of software code is worth 10,000 times the price of an average software writer." Indeed, mastering nuances such as module exports—and the errors associated with it—plays into being a 'great writer'. Correct usage and understanding of JavaScript modules exports and imports is hence pivotal for any developer wishing to create efficient, robust, and scalable applications. References:
- MDN Web Docs - Error: import must declare an exported item
- Exploring JS: Details of importing and exporting in ES6
- The final syntax of ES6 modules