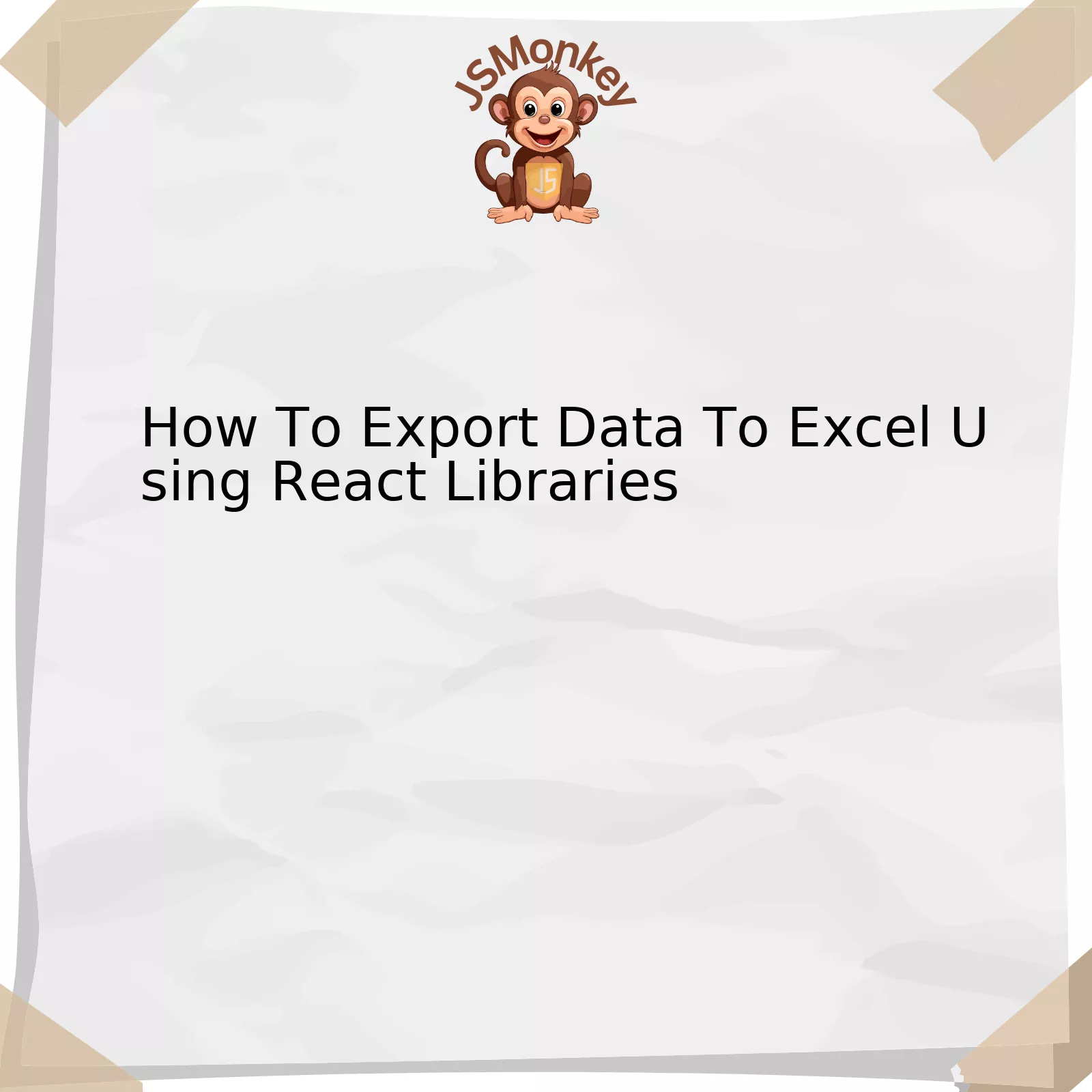
Exporting data to Excel using React libraries involves leveraging key libraries such as XLSX and FileSaver. A significant part of the process centers around formatting and configuring the dataset for a seamless export operation before initiating the download action.
First, let’s lay out the overall process visually using an HTML table:
Step | Action |
1 | Installation of required libraries (XLSX and FileSaver) |
2 | Data configuration |
3 | Conversion of data to worksheet format |
4 | Workbook creation and assigning of worksheets |
5 | Initiation of Download |
Now, let’s delve into each step in detail.
Step 1: Installation of Required Libraries
Install the necessary libraries – XLSX and FileSaver, using npm or yarn. These libraries are crucial in any data export functionality setup.
npm install xlsx file-saver or yarn add xlsx file-saver
Step 2: Data Configuration
Data to be exported should be organized in an array of objects where each object represents a row in the final excel file and properties of it indicating cells. Reference
Step 3: Conversion of Data to Worksheet Format
Using the XLSX library, data is converted into a worksheet format using function `xlsx.utils.json_to_sheet(myDataArr)`.
Step 4: Workbook Creation and Assigning Worksheets
A new workbook is created using `xlsx.utils.book_new()`. The formatted worksheet is then assigned to this workbook with `xlsx.utils.book_append_sheet(myWorkbook, myWorksheet, ‘MySheet’)`.
Step 5: Initiation of Download
Finally, the data download is initiated using the FileSaver library function `saveAs(blob, fileName)`, to complete the export process.
Paul Graham once said – “In programming, the hard part isn’t solving problems, but deciding what problems to solve.” And when it comes to exporting coded data, knowing the what and how of the problem at hand, is key to efficiently utilizing resources such as libraries like React to solve it.
Understanding the Process: Exporting Data to Excel with React Libraries
Let’s understand the process of data export into an Excel file using React libraries, a highly popular practice for businesses aiming at data analysis and reporting. We’ll dive into some popular libraries used for this functionality and how the whole setup works in the context of a React application.
Before diving into specific library details, it’s important to comprehend why the task of exporting data to Excel is so valuable. Excel, being a tool widely accessible and universally utilized, provides a common platform where non-technical personnel can analyze business data without needing assistance from web tools or developer teams. It’s accessibility and universal usage emphasize its importance.
Now let’s examine how a React application enables data export to excel:
1. A commonly used library for this task is
xlsx/react-js
, specifically designed to handle Excel file formats like XLSX. The features offered by this library include reading data from an existing workbook, creating new workbooks, and adding worksheets to workbooks, thus offering a myriad of ways to interact with Excel data.
2. The use of the
xlsx.write()
method allows for writing (or exporting) workbooks previously assembled in memory to physical files, and saving them in XLSX format. The command line utility accomodates data conversion tasks also.
The following example demonstrates basic implementation:
html
const ws = XLSX.utils.json_to_sheet(data);
const wb = XLSX.utils.book_new();
XLSX.utils.book_append_sheet(wb, ws, “Sheet1”);
XLSX.writeFile(wb, fileName);
3. For converting JSON data into spreadsheet formulas, cell addresses, and datatypes, the
xlsx.utils.json_to_sheet
function ensures efficient communication between your JavaScript data and Excel.
4. Another powerful library you might consider is
FileSaver.js
. Specifically designed for creating files on the client-side, it enables your application to dispatch data in various formats including Excel.
For example:
html
var XLSX = require(‘xlsx’);
var FileSaver = require(‘file-saver’);
var wb = XLSX.utils.book_new();
XLSX.writeFile(wb, ‘NameOfFile.xlsx’);
FileSaver.saveAs(blob, fileName+”.xlsx”);
Above, we see a process where workbook is created and then shipped off as an Excel file. The
FileSaver
API saves these generated files on the client’s machine.
Remember, as Mark Zuckerberg once said, “The biggest risk is not taking any risk… In a world that changing really quickly, the only strategy that is guaranteed to fail is not taking risks.” The same applies when opting to export data to excel using React Libraries. Understanding and implementing new libraries may feel daunting, but not venturing into new solutions can stifle progress. In the ever-changing world of web development, leveraging these stepping stones lead to practical and efficient results. Source
This framework should provide you with a comprehensive understanding of how to export data to Excel using React libraries, breaking down why the task is significant and outlining how one might tackle it. Whether utilizing `xlsx` or `FileSaver.js`, you can capitalize on these tools to augment your React applications.
Key Considerations when Choosing a React Library for Data Export
To boost the effectiveness, precision, and efficiency of data export operations in React applications, selecting an optimal React library is paramount. The following key considerations offer valuable guidance when choosing a React library for data export:
Efficiency and Performance
The selected library should enhance performance by enabling prompt and efficient data manipulation and export processes. Libraries like react-data-export excellently serve this purpose as it provides a simple and effective way of exporting data to Excel.
Implementation Code:
To illustrate, look at this simple code snippet:
import React from "react"; import * as ReactDataExport from "react-data-export"; const dataArray = [{name: 'John', age: 30}, {name: 'Jane', age: 28}]; const ExcelFile = ReactDataExport.ExcelFile; const ExcelSheet = ReactDataExport.ExcelFile.ExcelSheet; const ExcelColumn = ReactDataExport.ExcelFile.ExcelColumn; function App() { return (Download Data To Excel}> ); } export default App;
Compatibility
Ensure that the chosen library is compatible with the existing technology stack of your application. It should work well alongside other libraries, frameworks, tools, or software packages implemented within your system. By choosing a compatible React library for data export, it enhances overall development execution and minimizes the likelihood of software conflicts or issues.
Compliance with ES6 and React Standards
React is based around ES6 standards and principles, hence the selected library should comply with these stipulations. ES6 compatibility enhances functionality, making your code cleaner and more efficient.
Ease of Use
An ideal React library for data export should be user-friendly, allowing simple implementation even for developers who are not highly proficient in JavaScript or React. Excellent documentation and a vibrant community for support vastly improve ease of use.
Popularity and Community Support
A popular library generally implies widespread usage which often results in well-tested stability. Abundant examples and resources would be available online, contributing significantly to problem-solving during its use.
Remember this quote from Robert C. Martin, a renowned software engineer, “The best design is the one that allows for change”. Similarly, when choosing a library, it’s best to pick one that aligns with your current needs yet accommodates room for future modifications.
Step-by-Step Guide: Implementing Data exportation with Selected React Library
Implementing data exportation with a selected React library, particularly for exporting data to Excel, can be accelerated using npm packages such as ‘xlsx’ or ‘react-data-export’. The steps below break down the process:
Environment Setup and Library Installation
First, set up your environment. Assuming you already have Node.js installed on your system, create a new React application, navigate to your project directory, and install the necessary libraries.
npm create-react-app data_exportation cd data_exportation npm install xlsx file-saver react-bootstrap bootstrap
Create a New Component
Next, create a new React component ‘ExportReactCSV.js’ in the ‘src’ folder with the following code:
import React from 'react'; import * as FileSaver from 'file-saver'; import * as XLSX from 'xlsx'; const ExportReactCSV = ({csvData, fileName}) => { const fileType = 'application/vnd.openxmlformats-officedocument.spreadsheetml.sheet;charset=UTF-8'; const fileExtension = '.xlsx'; const exportToCSV = (csvData, fileName) => { const ws = XLSX.utils.json_to_sheet(csvData); const wb = { Sheets: { 'data': ws }, SheetNames: ['data'] }; const excelBuffer = XLSX.write(wb, { bookType: 'xlsx', type: 'array' }); const data = new Blob([excelBuffer], {type: fileType}); FileSaver.saveAs(data, fileName + fileExtension); } return ( ) } export default ExportReactCSV;
This component creates a button that upon clicking, triggers the function ‘exportToCSV’ to convert JSON data into Excel format and download it.
Using the Component
Now, utilize this component in your main application. Implement an array of JSON objects (your data) and call the ‘ExportReactCSV’ component with ‘csvData’ as your source data and ‘fileName’ as your preferred filename for the exported Excel file.
import React from "react"; import ExportReactCSV from './ExportReactCSV'; const App = () => { const customers = [ {firstname : "Lidiya", lastname : "Fox", email : "lidiyafox0@gmail.com"}, {firstname : "Milka", lastname : "Crasteau", email : "milka.c@gmail.com"}, {firstname : "Jasper", lastname : "Zapata", email : "Zapata.J@zoho.com"} ] const fileName = "Customers"; return (); } export default App;List of Customers
The above steps serve as a guided approach in implementing data exportation using React libraries.
“Your assumptions are your windows on the world. Scrub them off every once in a while, or the light won’t come in.” —Isaac Asimov. This quote rings true in technology. It is vital to occasionally reassess our understanding and approaches, seeking innovative solutions like using React libraries to simplify tasks such as exporting data to Excel.
For additional insight into these react libraries, refer to the official documentation here: (xlsx, file-saver, react-bootstrap).
Troubleshooting Common Issues in Data Export to Excel using React Libraries
Data export to Excel is a popular operation when it comes to data management in web applications, and certain React libraries are specially tailored for this function. However, developers might experience some common issues while implementing this feature leveraging React libraries. Below are three typical scenarios where problems can occur during data export to Excel and potential solutions.
1.Issue: Corrupted Excel Files
You may find that the Excel files you’ve exported are corrupted or not opening properly once downloaded. This could be due to incorrect or incompatible file formats, unsuitable library methods, or problems with data structures.
exportToExcel = () => { const workbook = new ExcelJS.Workbook(); // other codes workbook.xlsx.writeBuffer().then((data) => { const blob = new Blob([data], { type: 'application/vnd.openxmlformats-officedocument.spreadsheetml.sheet' }) saveAs(blob, 'filename.xslx'); }); };
The
Blob
is designed for handling binary data arrays. The
saveAs
from FileSaver.js is a solution used to save the Blob as an Excel file. This method saves the corresponding buffer array directly into the local machine without any corruption.
2. Issue: Incorrect/Incomplete Data in Excel File
Another common issue encountered is finding data inaccuracies or incomplete entries in the exported Excel file. This typically occurs due to an incorrect understanding of arrays and object data types in JavaScript.
const handleExportAndDownload = async ()=> { // Extracts fields from objects and creates headers const data = [...] // Headers and rest of the data fill the Excel sheet var ws = XLSX.utils.json_to_sheet(data) // Create New Workbook var wb = XLSX.utils.book_new() XLSX.utils.book_append_sheet(wb, ws, "App report") // Writes Excel File XLSX.writeFile(wb, 'app_report.xlsx') }
Here, the
json_to_sheet
function from the xlsx library is used to convert JSON data into an array of arrays. It ensures every cell in the data grid aligns correctly with corresponding Excel cells.
3. Issue: Slow Export Speed for Large Data Sets
If you’re working with large datasets, the exporting process may be slow. This is typically due to inefficient handling of large datasets by libraries or methods being used.
You could address this issue by optimizing your method of parsing data into the Excel file using streams or pagination of data, reducing the load handled at a given time.
As Jeff Atwood, the co-founder of Stack Overflow, says: “We should forget about small efficiencies, say about 97% of the time: premature optimization is the root of all evil”. Nonetheless, in scenarios like handling large datasets, keeping performance in mind becomes pivotal during implementation and provides optimal user experience.
Remember to ensure that the chosen React library can handle your requirements efficiently while taking care of common errors and exceptions.
Creating an Excel document from data in your React application can be achieved through various React libraries with ease. For a seamless experience, valuable suggestions would be to choose correctly between libraries such as `xlsx` and `react-data-export`, depending on the complexity of your dataset and user requirements.
The `xlsx` library offers versatility for both client-side and server-side usage, allowing you to work with larger datasets while ensuring extra features like styling, formatting and manipulating worksheets comprehensively. As seen in the following code snippet:
<code>
const wb = XLSX.utils.book_new();
// Creates excel worksheet
const ws = XLSX.utils.json_to_sheet(data);
// Append workbook
XLSX.utils.book_append_sheet(wb, ws, ‘Sheet1’);
// Generate downloadable file URL
const url = window.URL.createObjectURL(new Blob([s2ab(XLSSure)], {type: “application/octet-stream”}));
</code>
On the other hand, `react-data-export` brings about a more streamlined approach specifically tailored for React applications. It provides a clean interface for rapid exporting functionality. This library excels in situations where you need a quick setup and basic Excel output without multiple fine-grained controls which a more full-featured library like `xlsx` provides:
jsx
Reference: npmjs.com
In the words of computer scientist Donald Knuth, “Premature optimization is the root of all evil.” Therefore, when considering which library to use for exporting data to Excel in your React application, instead of obsessing over choosing the “best” one, it is more useful to consider which best meets your specific needs and constraints for a given project. Remember that there’s no one-size-fits-all solution; what might work best in one scenario may not be the most suitable in another. This way, you can ensure a balance between effective functionality and performance optimization.