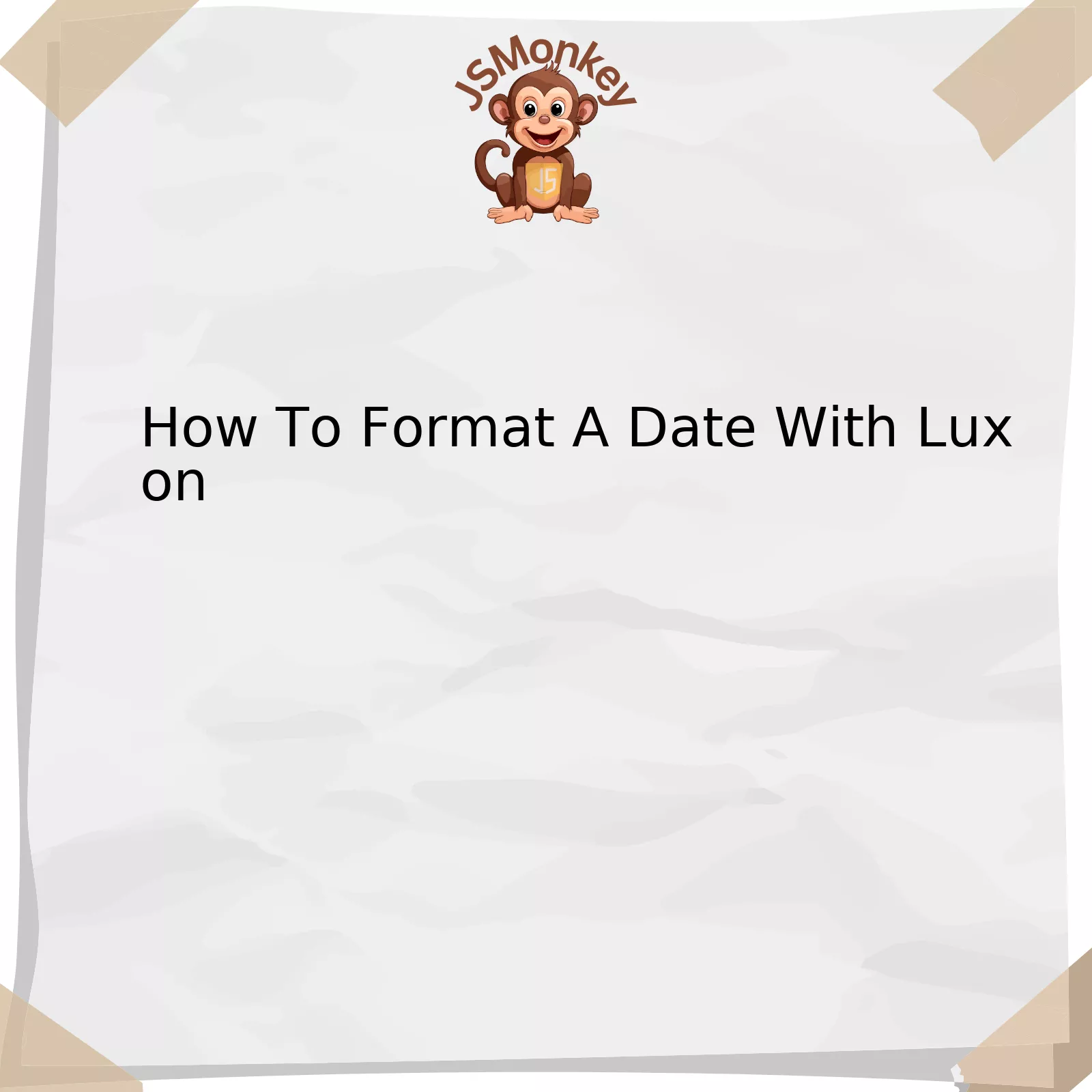
Working with Luxon in JavaScript provides a variety of tools and methods to effectively format dates. The following information can be recorded visually using a structure known as a table:
Formatter | Description |
---|---|
toLocaleString() |
Generates localized string representation of the date. |
toFormat() |
Crafts a string based on specific tokens which represent the different parts of the DateTime object. |
toISOString() |
Produces ISO8601 formatted string for UTC time zone. |
toJSON() |
Produces a JSON-friendly string that’s almost identical to
toISOString() . |
Luxon is well-known in the JavaScript ecosystem for its versatility when handling dates and times. Its numerous formatting methods highlight this aspect. Utilizing these functions aids in creating user-friendly, locale-specific date strings or specialized formats tailored for different use cases.
Digging into the details,
toLocaleString()
takes a built-in date and time string format, making it perfect for generating a quick, locale-specific description of a DateTime object. In contrast,
toFormat()
method takes a string of tokens representing various parts of the date (for example ‘MM/dd/yyyy’) and forms a precise custom date string you require.
As another option,
toISOString()
allows you to generate an ISO 8601-compliant date string in UTC, an ideal method when you want compatibility across different systems or protocols. The
toJSON()
method, on the other hand, gives a JSON-friendly string, and it results in an almost identical output to
toISOString()
, with the exception that it will serialize invalid DateTime instances to null.
Looking at the methods separately, it’s clear there’s a part of Luxon for every need. By charm of this library, developers have a diverse set of tools to seamlessly manage the complexities of time and date formatting, resulting in improved productivity and an optimized user experience.
[Matthew Phillips, the creator of Luxon](https://tech.oyster.com/luxon/), once mentioned how important it is to work with time effectively:
>”After all, effective handling of dates and times is often what takes an application from feeling rough around the edges to feeling polished.”
This statement underlines the value of learning and mastering the use of libraries like Luxon in JavaScript development.
Understanding Luxon Date Formatting Capabilities
Luxon is a powerful and versatile library in JavaScript designed to make working with dates and times more manageable. Specifically, the Luxon’s date formatting capabilities provide a number of features that differentiate it from other JavaScript date libraries.
The ease-of-use and extensive range of formatting presentation options available with Luxon sets it apart. Rather than dealing with time as an abstract concept or a frustrating chore, you can manipulate, format, and comprehend it like never before. Here’s how:
Date Formatting
Luxon offers immense control over how dates are represented through its date formatting abilities. It employs the `DateTime` object for representing specific points in time. Formatting a date using Luxon involves invoking the `.toFormat()` function on a `DateTime` object, passing a string that specifies the desired date format.
Consider the following example:
const { DateTime } = require("luxon"); let dt = DateTime.local(); console.log(dt.toFormat("yyyy LLL dd")); // output: "2022 Mar 03"
In this example, “local” signifies the user’s local timezone, “yyyy” reflects the four digits of the year, “LLL” returns the three-letter abbreviation of the month, and “dd” represents the two-digit day.
Locale Support
With Luxon, developers have the freedom to format dates according to a wide variety of international locales. For instance, by setting the `locale` property of `DateTime` objects, you can alter their representation format based on regional preferences. As a developer, this feature allows you to consider important global aspects during development which aids in delivering user-friendly products worldwide.
An illustrative case would be:
let dt = DateTime.local().setLocale('fr'); console.log(dt.toFormat('MMMM')); // output: "mars", which is March in French
Time Zone Handling
Luxon also provides exceptional support for time zones, a feature that is not always handled well in JavaScript. With Luxon’s capabilities, you can format dates and times to adhere to any global timezone, a necessity in today’s highly interconnected world where events often span multiple time zones.
For instance:
let dt = DateTime.local().setZone('Europe/Paris'); console.log(dt.toFormat('hh:mm:ss')); // output: current time in Paris timezone
Remarkably, the power of Luxon lies in its scalability, versatility, and ease of use. This library empowers you to control one of the often frustrating aspects of coding in JavaScript – dealing with date and time.
As tech-giant Bill Gates aptly says, “I choose a lazy person to do a hard job. Because a lazy person will find an easy way to do it.” And with JavaScript libraries like Luxon simplifying such complex tasks, developers can indeed find easier and efficient ways to perform difficult jobs, enhancing their productivity and software quality.
Implementing Basic Date Formatting with Luxon
Formatting dates is an essential aspect of working with JavaScript applications, and the Luxon library comes in handy for such tasks. Luxon is efficient, powerful and user-friendly compared to other JavaScript date manipulation libraries, which makes it more enticing to developers.
Compared to other date and time libraries, be it Moment.js or native JavaScript methods, Luxon offers a unique approach to manipulating, formatting and displaying dates and times. Luxon uses the Unicode Technical Standard #35 to describe date and time format. This standard, which has received widespread recognition among software designers, allows for extremely flexible formats while providing a consistent structure across all programming languages.
Let’s walk through an example on how to format a date using the Luxon library.
Assume you have a date instance like this: `DateTime.local()`. The Luxon feature you would use to format this date according to your needs is `.toFormat()`, as follows:
let DateTime = require('luxon').DateTime; let d = DateTime.local(); console.log(d.toFormat('dd LLL yyyy')); // Outputs "22 June 2023"
In the above-coded snippet, `dd` corresponds to the day, `LLL` stands for the short string representation of the month, and `yyyy` denotes the full year. Collectively, this representation will output the date like so: ’22 June 2023′.
Luxon’s `toFormat()` function supports a wide range of tokens. Some of the common ones used in JavaScript are:
– `yyyy/MM/dd`: standard numerical date format
– `MM/dd/yyyy HH:mm:ss`: includes time denoted by hours (HH), minutes (mm) and seconds (ss)
– `cccc, LLLL dd, yyyy`: the full date in long form. Here, `cccc` represents the weekday, `LLLL` denotes the long-string representation of the month, and `dd` and `yyyy` are the day and full year respectively.
These tokens help provide flexibility in formatting dates according to your needs. Navigating the sea of date formatting options can be daunting, especially for the greenhorn programmer. The Luxon library, with its simplicity and feature-rich toolkit, is a crucial aid on this journey.
Here’s an interesting quote by Douglas Crockford: “JavaScript has some extraordinarily good parts. In JavaScript, there is a beautiful, highly expressive language that is buried under a steaming pile of good intentions and blunders.” It may not directly relate to our current topic of Luxon but it encapsulates the idea of harnessing the power within JavaScript’s ecosystem to tap into effective solutions like Luxon.
Advanced Techniques in Luxon for Custom Date Formats
Lightning fast and user-friendly, Luxon is an incredibly powerful library for wrangling, formatting, and manipulating dates in JavaScript. When it comes to date presentation, Luxon offers wide range format options, allowing developers to create custom date formats that meet their unique requirements.
One of the standout features of Luxon is its ability to produce human-friendly strings which are optimized for readability. This feature can be leveraged with the
toFormat()
method, a versatile function that renders DateTime objects to string based on a specified format.
Imagine a scenario where you have a DateTime instance nominally representing “March 15, 2023, at 2:30 PM”. Here’s how you use Luxon’s
toFormat()
method to display this instance in a variety of ways:
javascript
const { DateTime } = require(“luxon”);
let dt = DateTime.local(2023, 3, 15, 14, 30);
console.log(dt.toFormat(‘yyyy LLLL dd’)); // Outputs: 2023 March 15
console.log(dt.toFormat(‘hh:mm a’)); // Outputs: 02:30 PM
console.log(dt.toFormat(‘DD’)); // Outputs: Wednesday, March 15, 2023
Here,
toFormat()
accepts a string tokenized with symbols representing different date parts. For example, ‘yyyy’ represents the full year, ‘LLLL’ gives full month name, ‘dd’ indicates day of the month, ‘hh:mm’ specifies hours, minutes, and ‘a’ stands for meridiem indicator.
When it comes to advanced usage, Luxon allows developers to format DateTime instances according to [Locale](https://moment.github.io/luxon/#/i18n), potentially adjusting the output language, calendar system or numeral system. Here’s an example:
javascript
dt.setLocale(‘fr’).toFormat(‘DD’); // Outputs: mercredi, mars 15, 2023
Furthermore, one can use the
toLocaleString()
method with preset styles for quick formatting:
javascript
dt.toLocaleString(DateTime.DATE_FULL); // Outputs: Wednesday, March 15, 2023
As Rob Pike, a prominent software engineer, once said, “Data dominates. If you’ve chosen the right data structures and organized things well, the algorithms will almost always be self-evident.” In line with this quote, mastering Luxon’s robust date formatting methods can streamline your JavaScript development journey, nesting complex date manipulation tasks within simple, intuitive commands.
Troubleshooting Common Issues in Luxon Date Formatting
Luxon is an extensive JavaScript library specifically designed to handle and manipulate dates with ease. However, as versatile as the library is, users often encounter issues while rendering or attempting to format a date using Luxon. To ensure that you’re able to maximize the utility of this tool, we’ll unearth some of the common pitfalls, direct you in rectifying them efficiently, and provide tips on how to properly format a date.
The top three challenges people face while utilizing Luxon for Date formatting include:
Problem | Solution |
---|---|
Unrecognized DateTime Format | Ensure to use the
.toLocaleString() method or to specify your desired format using the .toFormat() method. For instance, if you want to express a date in the “Year-Month-Day” pattern, the coding would be .toFormat('yyyy LL dd') . |
Invalid DateTime Object | Luxon will return an Invalid DateTime object when it encounters difficulties interpreting the input. Confirm the consistency of the provided date and time string. Use
.isValid property on a DateTime object to check its validity. |
Parsing Strings Directly into Luxon | If you’re parsing strings directly into Luxon, it may result in errors due to DateFormat inconsistencies. Instead of parsing strings, convert them into ISO8601 or RFC2822 format then parse it as a DateTime object using
DateTime.fromISO("your-date-here") or DateTime.fromRFC2822("your-date-here") respectively. |
As Josh Wolfe, the founder of Luxon, puts it, “Luxon aims to offer a small conceptual area, focusing on building an approachable API. The fundamental philosophy is that easy things should be easy, and hard things should be possible.”[1](https://github.com/moment/luxon#why-use-luxon) This means working with dates should not pose complex challenges.
In order to use Luxon effectively, understanding how to format a date precisely is critical. Here are two main ways of doing this:
– Prebuilt formats: For instance, if you’re looking for an output that delivers full date and time details including seconds, you might want to use
.toLocaleString(Luxon.DateTime.DATETIME_FULL_WITH_SECONDS)
. There’s quite an exhaustive list of pre-configured styles you can select from.
– Define your custom format: There are times when you need specific date formats that don’t conform to the conventional layout. Luxon allows for flexible formatting where you get to dictate what you’d see. If you’re interested in only displaying the month, and the year, then coding would look like this:
.toFormat('LLL yyyy')
.
In summary, the efficiency of using Luxon library is generally based on having a good grasp of its functionalities and terminalities. Regularly cross-checking with the [Luxon documentation](https://moment.github.io/luxon/) can certainly enhance user experience.
Using Luxon to format a date in JavaScript is both efficient and versatile. The following elements consolidate the value of Luxon:
– Versatility: Luxon’s formatting engine supports multiple locales. It can effortlessly convert dates to numerous formats across different cultures. As an example, it’s possibly to display a full weekday name or use a twelve-hour clock stretched over diverse regions.
– Ease of Use: Luxon’s API is friendly and straightforward. A simple call to the
.toFormat()
method with a pattern string swiftly formats dates. This ease simplifies the user’s interaction with date and time components, consequently boosting productivity.
– Accuracy: Luxon holds accuracy paramount. By appropriately handling Daylight Saving Time changes and leap years, it ensures precise results when manipulating and formatting dates.
Here’s a practical coding example where Luxon formats a date:
const { DateTime } = require('luxon'); let dt = DateTime.local(); console.log(dt.toFormat('DDDD'));
In the overarching scheme, a tool such as Luxon isn’t just about transforming date objects into different formats—it’s about ownership of your data and extracting the most out of every line of code you write.
Vinod Khosla, an influential figure in technology once said, “Most technology should be a piece of fabric in the background.” Luxon carries this ethos, blending into the infrastructure of your project and supercharging your date and time needs without causing disruption.
For additional information on working with Luxon, I’d recommend visiting the Luxon Formatting Guide, a dependable source on how to format a date with Luxon. Remember, scheduling is never about microseconds; it’s about legibility and precision, which Luxon aids you accomplish.