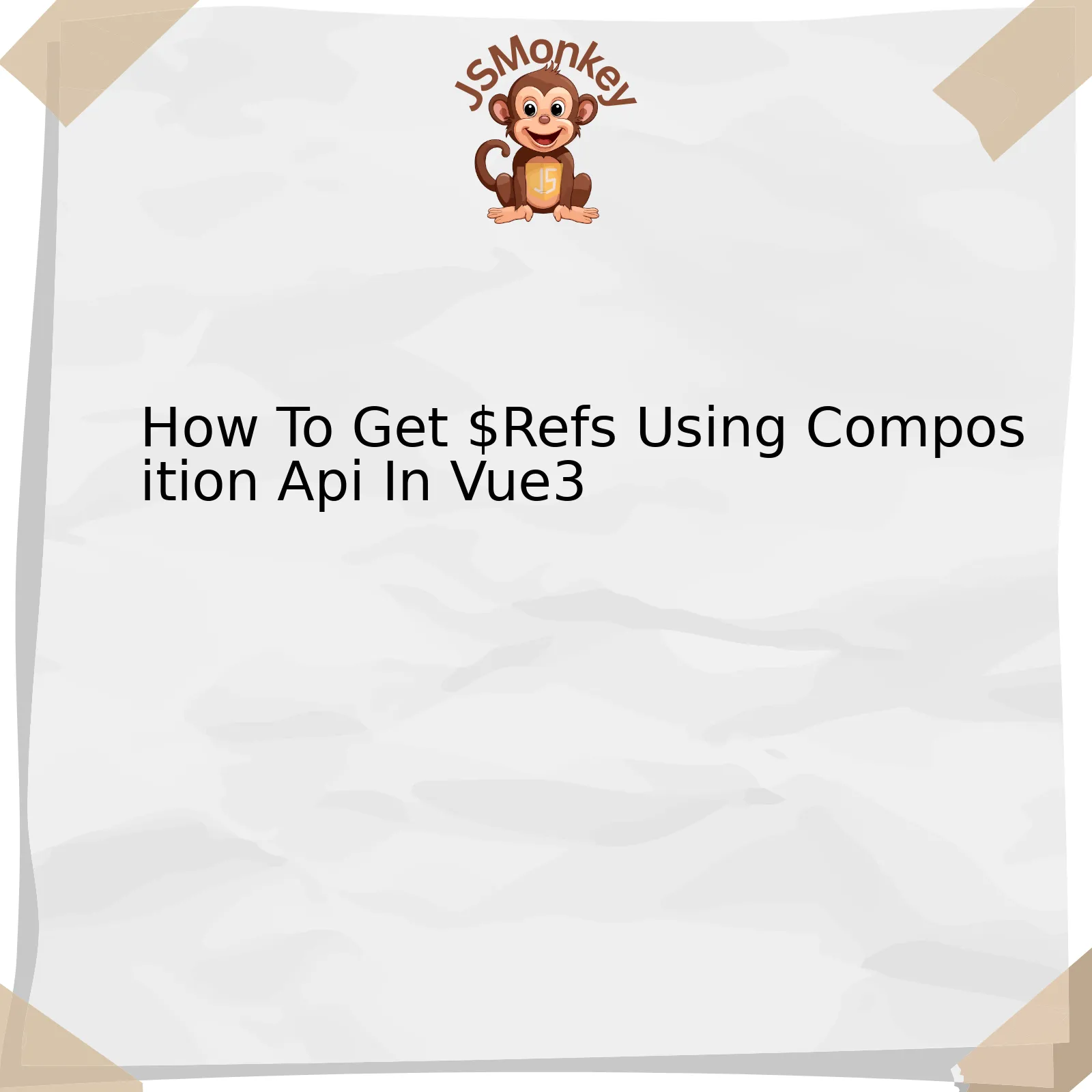
Getting `$refs` using the composition API in Vue3 is quite straightforward. Firstly, it’s important to understand that `$refs` are used in Vue to access DOM elements directly.
Steps | Description |
---|---|
Define a Ref | We need to define a `ref` to attach to the DOM element we want to access. |
Attach the Ref to DOM | Once the `ref` has been created, we can then use v-bind directive to bind it with the specific DOM element. |
Access the Ref | We can easily access this `ref` inside the setup function. |
To make more sense of the table above, here are the steps in more detail:
– Define a ref: In Vue3, `ref` is a reactive reference to a value which we can grab by importing `ref` from `vue`. Here’s how you can define it:
import { ref } from 'vue' const myRef = ref(null)
– Attach the `ref` to DOM: Now, to connect the created `ref` with our target DOM element, we need to use the `v-bind` directive within our template.
<div v-bind:ref="myRef"></div>
– Access the `ref`: After binding the `ref` with the DOM, it becomes easy to access it anywhere within setup block.
console.log(myRef.value)
Notice that, unlike Vue2, here we use `myRef.value` to access the underlying DOM element instead of `this.$refs.myRef`.
As Brendan Eich, creator of JavaScript has once stated, “Always bet on JavaScript.”. This especially resonates with the Vue3 composition API as it greatly expands the possibilities within the framework.
When using `$refs`, make sure that you are using them for things like manipulating the DOM or integrating with 3rd party libraries. They should not be used for data-flow purposes in your application, as Vue’s reactivity system provides ways to keep track and manipulate your data in a more structured way.
Leveraging Composition API for $refs in Vue3
One of the significant upgrades introduced with Vue 3 is the Composition API, a set of additive, function-based APIs that allow flexible composition of component logic. One common use case in Vue projects involves accessing DOM elements or child components using `$refs`. Understanding how to utilize `$refs` within the new Composition API framework can be of considerable advantage.
The `$refs` property in Vue allows us to directly access the DOM elements or child components. In Vue 3’s Composition API, we need to leverage `Ref` to create mutable reactive references. The process may appear slightly different from what you might be used to in the Options API.
Firstly, let’s consider how we’d originally use `$refs` in the Options API:
html
javascript
export default {
mounted() {
console.log(this.$refs.myDiv.innerText); // ‘Hello world’
},
};
In the snippet above, we define a ref “myDiv” on a div element and subsequently access it in the `mounted()` lifecycle hook.
When leveraging the Composition API, our approach becomes slightly different:
html
javascript
import { ref, onMounted } from “vue”;
export default {
setup() {
const myDiv = ref(null);
onMounted(() => {
console.log(myDiv.value.innerText); // ‘Hello world’
});
return {
myDiv
};
},
};
With the Composition API, we first initialize `myDiv` as a `ref` with the initial value `null`. In the `setup()` method, we then use the lifecycle hook `onMounted()` (equivalent to `mounted()` in Options API). Here, the `myDiv` ref is directly logged to the console. It’s important to note that when we use `ref`, we need to add `.value` to obtain the reference value.
As Bill Gates once said, “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.” This is true for these changes and updates introduced with Vue3 and the Composition API. As developers, it’s up to us to seamlessly integrate this new API into our development practices, making it the new ‘part of everyday life’ for coding.
For further reading on refs in Vue 3 Composition API, click here .
Strategies for Accessing $refs Using the Composition API
Understanding and effectively utilizing the component-oriented architecture of Vue.js can significantly enhance your application’s performance and maintainability. One feature that aids in this endeavor is `$refs` or references in Vue.js, especially as it relates to the newly introduced Composition API in Vue3.
The `$refs` object is a valuable tool within Vue’s arsenal, providing direct access to DOM elements or child components. In older versions of Vue, i.e., Vue2, you could easily access `$refs` within the `mounted()` lifecycle hook. However, with the introduction of the Composition API in Vue3, handling `$refs` has slightly changed.
Let’s start by understanding how to get `$refs` using the Composition API in Vue3:
html
In the example above, Vue3 uses the `ref()` function from vue package to create reactive references to DOM elements. Note that we are using `value` property of each `ref()` here. This is because `ref()` wrapped data should be accessed using `.value`.
However, some strategies could boost the process of accessing `$refs` better with the Composition API:
– **Reactivity**: Utilize Vue’s reactivity system to reactively provide data to your `$refs`. This means rather than defining your refs as reactive data using `ref()`, you can directly use them in the template, and access them in setup method using `isRef`.
– **Composable functions**: Write composable functions to encapsulate logic related to `$refs`. Composables are a great part of Composition API that can be used to abstract logic about common tasks such as reactivity, computed properties or directly manipulating `$refs` objects.
– **Lifecycle hooks**: Properly utilize lifecycle hooks such as `onMounted` or `onUpdated` for handling references. This ensures that the DOM element or the child component is ready to be accessed.
As Peter Drucker said: “Efficiency is doing things right; effectiveness is doing the right thing”. Understanding and implementing these strategies could make your usage of Vue3 and its Composition API both efficient and effective – ensuring you’re doing things right and also using the right solution.
Optimal Use of $refs with Vue3’s Composition API
The Composition API in Vue 3 has brought forth a host of advantages to the Vue.js framework. It allows developers to encapsulate bits of business logic in composable functions, making them easily shareable across components. One component that often requires special treatment in the Vue.js universe is $refs.
Understanding `$refs` in Vue.js:
A ref (`$ref`) is a reference to a DOM element or a child component within a Vue.js application. It provides a way to manipulate DOM elements or call methods on child components without relying heavily on JavaScript.
Use | Vue.js Implementation |
---|---|
DOM Element Reference | Directly access for manipulation – similar to querying via `document.querySelector`. |
Child Component Reference | Call methods or access data from child components directly. |
Utilizing `$refs` with the Composition API:
In Vue 3’s Composition API, `$refs` are defined similarly as in the Options API; However, they must be wrapped in a `ref()` function. Referencing a DOM element within the template could look like this:
html
<template>
<div ref=”myRef”>Hello Vue 3</div>
</template>
<script>
import { ref, onMounted } from ‘vue’;
export default {
setup() {
const myRef = ref(null);
onMounted(() => {
console.log(myRef.value); // Logs:
});
return {};
}
};
</script>
By accessing `$refs` within the `onMounted()` hook, we ensure that the DOM node is available when accessed. The `.value` property must be used to access the underlying reference.
“Understanding references ($refs) and the Composition API in Vue.js will unlock new levels of interactivity in your Vue applications.”
A beneficial approach when using `$refs` with the Composition API in Vue 3 is to limit their usage to only when absolutely necessary; They should not be used as a crutch to every problem but seen as a tool for unique challenges that cannot be solved in a reactive way.
For more comprehensive information, check out the Vue 3 Documentation on the Composition API.
The Role of Composition Api in Managing $refs within Vue3
The Composition API, introduced in Vue 3, provides a new and efficient system to manage $refs within the application. The overall roles of this API that contribute to the efficient handling of $refs essentially revolve around enhancing code quality, improving readability while structuring larger-scale applications, and offering a more flexible way for code reusability.
However, when it comes to getting $refs using the Composition API in Vue3, the specifics are somewhat different from the traditional Vue.js approach. The general steps are as follows:
– First, import ‘ref’ from Vue.
– Next, create a ref inside your setup method.
– Bind this ref to an element in your template section.
– Finally, you can access this ref inside your setup function.
This process is contributed by Composition API’s central concept which is “everything is a function”. This changes the way we think about organizing state and logic in our components. When $refs are used with Composition API, they provide direct access to child components, allowing for more direct interaction.
To illustrate this, consider a modal example:
import { ref } from 'vue'; ... setup() { const modalRef = ref(null); const openModal = () => { modalRef.value.show(); }; return { modalRef, openModal }; }
In your template section then, you simply bind modalRef to your modal component:
In the above example, modalRef provides a reference to the MyModal instance, enabling the parent component to directly call methods on it i.e., show().
Exactly quoted by Charlie Jeppsson, a leading expert on front-end development, “Even though developers have now been given a powerful tool at their disposal with the Composition API, good judgement should be utilized in terms of its application. Excessively complex or unnecessarily indirect code can result from misuse.” Thus, while Composition API offers brilliant flexibility and control, it should be reasonably used to avoid complications.
For detailed understanding you can refer this Vue3 Documentation.
In receptiveness to the need for simpler, more maintainable code, Vue 3’s Composition API emphasizes instance-specific composition and recyclability. This programming paradigm shift propounds a focused and flexible application structure. The proper targeting of $refs within this architecture occurs using ‘ref()’ and ‘toRefs()’. Vue enthusiasts will find that employing these methods increases productivity, readability, and consequently the overall quality of their software projects.
Reinforcing these techniques in your toolkit empowers developers to encapsulate functionality or state logic into reusable code snippets called composable functions. Then you can simply import these functions into the components that require them. This process contributes to modularising your codebase, making it easier to reason about complex scenarios since different pieces of functionality are siloed away from each other.
When you need to directly interact with DOMs or child components, leverage Vue’s $refs object to keep track of that element you would like to reference. Keep in mind, however, that their usage should ideally be minimal as the Vue.js philosophy encourages developers to think in terms of data-driven Uis rather than imperative commands.
import { ref } from "vue"; export default { setup(){ const inputElement = ref(null); return { inputElement, } }, }
Here, we have declared an inputElement ref by calling the `ref()` function from vue. Once assigned to a DOM element with the `ref` attribute, this will be used as point of reference.
Undoubtedly, mastery of any new development technology involves continuous learning. Vue.js, with its smooth assimilability and growing popularity among developers worldwide, is no exception. If you are eager to deepen your comprehension of Vue.js, I recommend exploring additional official documentation on the Composition API. In the words of Alan Turing, “We can only see a short distance ahead, but we can see plenty there that needs to be done”, and it all starts by taking that next step in your journey.
Weaving these Vue.js concepts and best practices into your daily workflow, you’ll be writing cleaner, more efficient, and scalable code in no time!