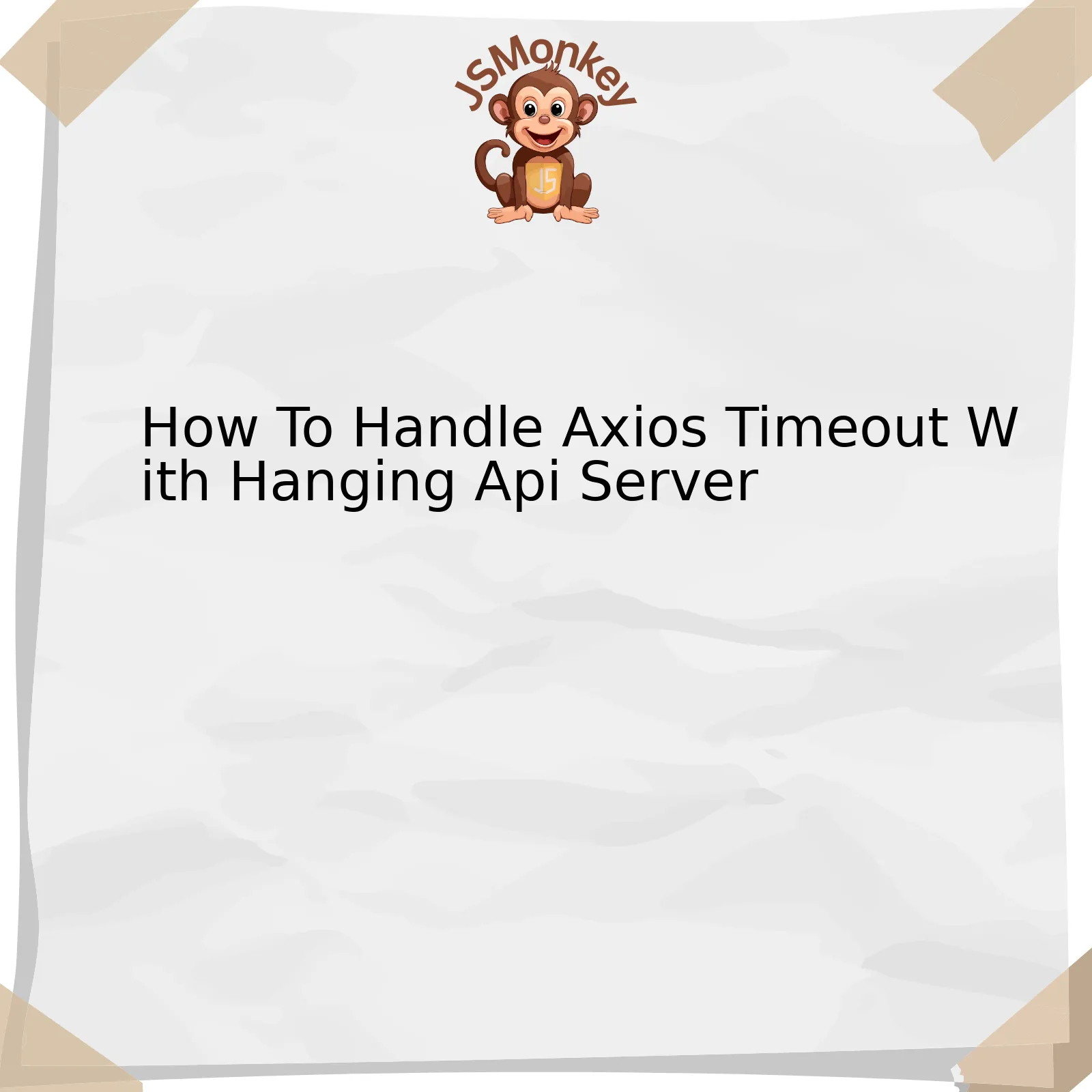
With Axios, an HTTP library for Javascript applications, the issue of handling timeouts with a hanging API server can occur from time to time. Server response time can often exceed the expected wait-time due to various reasons, resulting in the applications experience delays and sometimes, total failure.
The steps to handle such issues with Axios’s Timeout feature can be summarized as follows:
Action | Description |
---|---|
Initialize Axios Instance | Create a new Axios instance using the
axios.create() method. The base URL, timeout limit, headers and other parameters can be defined in this instance. |
Set Timeout Limit | The
timeout attribute should be defined within the Axios instance. This attribute specifies the number of milliseconds before the request times out. |
Axios Request | Make the requests to the server through a specified endpoint. If the server response exceeds the timeout limit, Axios throws a “Network Error”. |
Error Handling | If the request promises fails, we catch it with a call to
.catch() . We then include error-handling logic that can determine if the error was due to a timeout or some other occurrence. |
Retries (Optional) | In certain cases, one might want to retry the request after failure possibly after a delay or under certain conditions. This can be implemented by making another Axios request in the error-handling section. |
The steps outlined above will equip you with the means to manage API server delays more effectively and efficiently. Enforcing timeout limits through Axios helps control how your application handles delays, allowing for leaner, more user-friendly applications. Further customizability is possible, such as conditional retries on failure, providing additional control over the interaction with external APIs.
As stated by Linus Torvalds, “Most good programmers do programming not because they expect to get paid or get adulation by the public, but because it is fun to program.”
Likewise, facing challenges such as handling timeouts with a hanging API server might seem daunting at first, but remember, they are part of the journey in any coding venture, which ultimately makes you a better developer.
For more detailed instructions, you may refer to Axios’s official GitHub page.
Understanding the Role of Axios in API Interaction
Axios is one of the most prominent tools in modern web development that aids developers when dealing with API interaction. It is a lightweight, promise-based HTTP client renowned for its capability to run on both browser and server. The reasons for utilizing Axios are manifold:
Defining Axios’ role appropriately underscores our exploration into one of its crucial functions – handling timeouts with a hanging API server.
Imagine a scenario wherein your JavaScript application makes an API request using Axios, but the server hangs or stops responding due to an issue. This situation could exponentially increase the waiting time leading to a poor user experience. Hence, Axios provides the `timeout` attribute to overcome this challenge. Here’s how you set it:
axios.get('your_API_endpoint', { timeout: 5000 })
Here, the `timeout` attribute specifies the number of milliseconds before the request gets aborted. In this case, if the response isn’t received within 5000 milliseconds (5 seconds), Axios will abort the request.
However, merely setting the timeout doesn’t solve the problem completely. We must also efficiently handle the error thrown by Axios to ensure users do not experience unexpected application behavior.
Error Handling
When a timeout occurs, Axios throws an error which can be caught and handled within a ‘catch’ block as shown below:
axios.get('your_API_endpoint', { timeout: 5000 }) .catch(function (error) { if (error.code === 'ECONNABORTED') { console.error('Request timed out!') } });
In the catch block, an error object is returned. If the error code property equals ‘ECONNABORTED’, it denotes that the request was aborted – likely due to a timeout.
The usage of Axios for API interaction, especially its capability to handle timeouts with a hanging server API, empowers developers to build effective applications. This ensures delivering optimal user experience even in unfavorable network situations. As Aubrey Johnson once said, “JavaScript isn’t a part-time job (anymore).” [source] Thus, effectively optimizing tools like Axios is a requisite when dealing with JavaScript for a full-fledged outcome.
Insights into Timeout Issues with Hanging API Server
Promises, Axios library timeout features and the nature of event-driven programming in JavaScript bring forward certain complexities when dealing with hanging API servers.
Hanging API Server | Solution with Axios Timeout |
---|---|
An API server is said to ‘hang’ when it leaves a client’s request unattended indefinitely, due to unintentional irregularities like code bugs, overwhelming traffic or network issues. | Axios is a promise-based HTTP client for JavaScript designed to work both on the browser and Node.js platform, which means, it allows programmers to handle asynchronous operations effectively by setting timeouts at your discretion. |
A common issue with hanging API servers is that they don’t complete the request-response cycle within the time limit you have set. This may leave your application in stasis, consuming system resources without serving any purpose. To solve this problem, we use Axios’ request time-out features.
Here is an example utilizing Axios requesting a resource from an API endpoint:
html
const axios = require(‘axios’).default;
const fetchData = async () => {
try {
const response = await axios.get(‘YOUR_URL’, { timeout: 5000 }); // Set your desired timeout here.
console.log(response.data);
} catch (error) {
if (error.code === ‘ECONNABORTED’) {
console.error(‘Request timed out!’);
} else {
console.error(error.message);
}
}
};
fetchData();
In the above piece of code, a timeout period of 5000ms (or 5 seconds) has been assigned, meaning, if the API server hangs or fails to respond within 5 seconds of receiving the request, Axios will abort the connection and control will be transferred to the error handler block.
However, as it’s been paraphrased by many tech enthusiasts like Craig Buckler in an insightful quote “A developer’s problem-solving ability is not just fixing bugs, but about finding the best ways to fix them.” Hence, we need to ensure that appropriate error handling mechanisms are put in place.
Consider incorporating error handlers to catch any other exceptions. This could include logging of such occurances, retries for failover systems or providing a helpful user interface message to keep the user informed of the situation at hand.
Moreover, it is important to note that setting a global timeout in Axios might not meet the requirements of all your API calls. Some routes may require more processing time than specified in the set global timeout, resulting in timeouts. Thus, it is advised you handle each axios API call separately, giving each its own timeout value based on bespoke requirements.
Axios provides us with means to customize configuration for individual requests while making API calls. This gives developers enough flexibility and control over various API endpoints according to their needs.
API Hanging can prove challenging especially when serving real-time services. Managing it with Axios’ timeout feature, utilized aptly, could enhance resilience and responsiveness of our applications significantly with limited resources. Remembering that proper implementation, valuable planning and ample testing are essential steps towards gaining insights into timeout issues and tackling them mindfully while working with hanging API servers.
Effective Strategies for Handling Axios Timeout
Axios, a promise-based HTTP client for the browser and node.js, plays an important role in JavaScript development. When your API server is hanging, Axios offers you ways to deal with this challenge using timeout strategies. Here are some effective methods:
Setting a Timeout:
You can define request timeout in milliseconds in Axios configuration. It makes sure that your server doesn’t hang forever and fail after a specified time span.
axios.create({timeout: 5000});
Error Handling:
Axios embraces the paradigm of promises, making error handling less cumbersome. Catch blocks allow us to handle a network error or any other problems that lead to promise rejection.
axios.get('https://api.mywebsite.com') .catch(function (error) { if (error.response) { console.log(error.response.data); } else if (error.request) { console.log(error.request); } else { console.log('Error', error.message); } console.log(error.config); });
Retry Failed Requests:
There can be situations where retrying the failed request might yield success. For this, libraries such as axios-retry provides remarkable utility.
const axios = require('axios'); const axiosRetry = require('axios-retry'); axiosRetry(axios, { retries: 3 }); axios.get('https://api.mywebsite.com/thedata')
As Linus Torvalds once said, “Bad programmers worry about the code. Good programmers worry about data structures and their relationships.” So try to control the http client instead of letting it control your code. Whether setting a specific timeout or implementing complex mechanisms to handle or retry failed requests, a little consideration goes a long way in ensuring a smooth user experience. As developers, we should always strive to build resilient systems that can gracefully handle unexpected circumstances.
Troubleshooting Tips: Enhancing Axios Performance with Hanging API Servers
For handling Axios timeout caused by hanging API Servers, we can utilize a strategic approach that concentrates on enhancing performance and accessibility. Here, the primary focus is to ensure our applications dependent on APIs do not stall as a result of server latencies or temporary unavailability.
Axios Timeout Handling
An Axios request configuration provides an attribute known as ‘timeout’, a parameter representing milliseconds before the HTTP request is aborted. If an API server is taking longer than the allotted time, this prevents prolonged waiting through:
axios({ url: 'https://api-domain.xyz/request', method: 'get', timeout: 5000 }) .catch(function (error) { if (error.code === 'ECONNABORTED') { console.error('Request timed-out'); } });
The above code snippet sets a timeout of 5000 milliseconds (5 seconds). Therefore, if the server does not respond within this timeframe, Axios cancels the request and returns an ‘ECONNABORTED’ error.
Loosening-Up Retry Methods
In the event of an Axios request timing out, implementing a retry mechanism helps increase success rate by making several attempts until a response is received. Advanced retry libraries such as axios-retry, amplify the ability to automate this process with robust configurations and options.
const axios = require('axios'); const axiosRetry = require('axios-retry'); axiosRetry(axios, { retries: 3 }); axios({ url: 'https://api-domain.xyz/request', method: 'get', timeout: 5000 }) .then(function (response) { console.log(response); }) .catch(function (error) { if (error.code === 'ECONNABORTED') { console.error('Request timed-out'); } });
In the above example, the Axios request is retried up to three times if it fails due to a network error or timeouts.
Rate Limiting and Back-Off Strategies
Another approach that can boost performance when dealing with hanging API Server involves throttling and/or rate limiting. These strategies mitigate failure by reducing the number of requests being sent in a given timeframe, hence minimizing server load and subsequent response time.
As quoted by Donald Knuth, “premature optimization is the root of all evil”. Rather than optimistically sending requests and hoping for quick responses, adopting and implementing these solutions allows us to defensively optimize our applications such that they are resilient to outages, slowdowns, and latencies from external servers.
Overstepping the challenges posed by the hanging API server in using Axios is not a Herculean task. A solid understanding of Axios methods, coupled with insightful configuration of timeout and error-catching procedures ensures effective handling.
By setting a sensible timeout period on the Axios request, one can avoid getting stuck on an API call indefinitely. Adjusting these parameters as per your specific application’s needs will contribute to enhancement of overall responsiveness and user experience.
Axios allows precise handling of HTTP requests in JavaScript through provision of promise-based approach, enabling smoother execution flow. The right application of these promises allows for careful management of API calls, particularly when handling crucial data reception from servers.
An optimal way to handle this situation would be:
axios({ method: 'get', url: '/api/v1/data', timeout: 5000 // Wait for 5 seconds }) .then(function(response) { console.log(response); }) .catch(function(error) { if (error.code == 'ECONNABORTED') { console.error('A timeout happend on url', error.config.url); } });
This snippet demonstrates an ideal timeout setting along with a relevant catch procedure. By recognizing the ‘ECONNABORTED’ error code, we ensure that a network-related abort doesn’t disrupt our application’s flow and cloud users’ experience.
In return, this optimizes the loading time resulting in better SEO performance. To reiterate Steve Jobs; “Technology is nothing. What’s important is that you have faith in people, that they’re basically good and smart. And if you give them tools, they’ll do wonderful things with them.”
Here are a few fundamental strategies to keep in mind:
– Through Axios’ provision of interceptors, potentially timeout-inducing issues can be pre-emptively addressed even before the request is sent or after a response has been received.
– Managing errors effectively through use of the ‘catch’ block is vital to cauterizing potentially erroneous scenarios.
– Keeping timeouts reasonable; while shorter timeouts could lead to request abandonment due to server unresponsiveness, longer timeouts could negatively affect user experience.
Taking these into account, handling Axios timeout with hanging API server becomes a manageable task that improves your application’s SEO and overall efficiency. Explore more on beginning your journey with Axios.