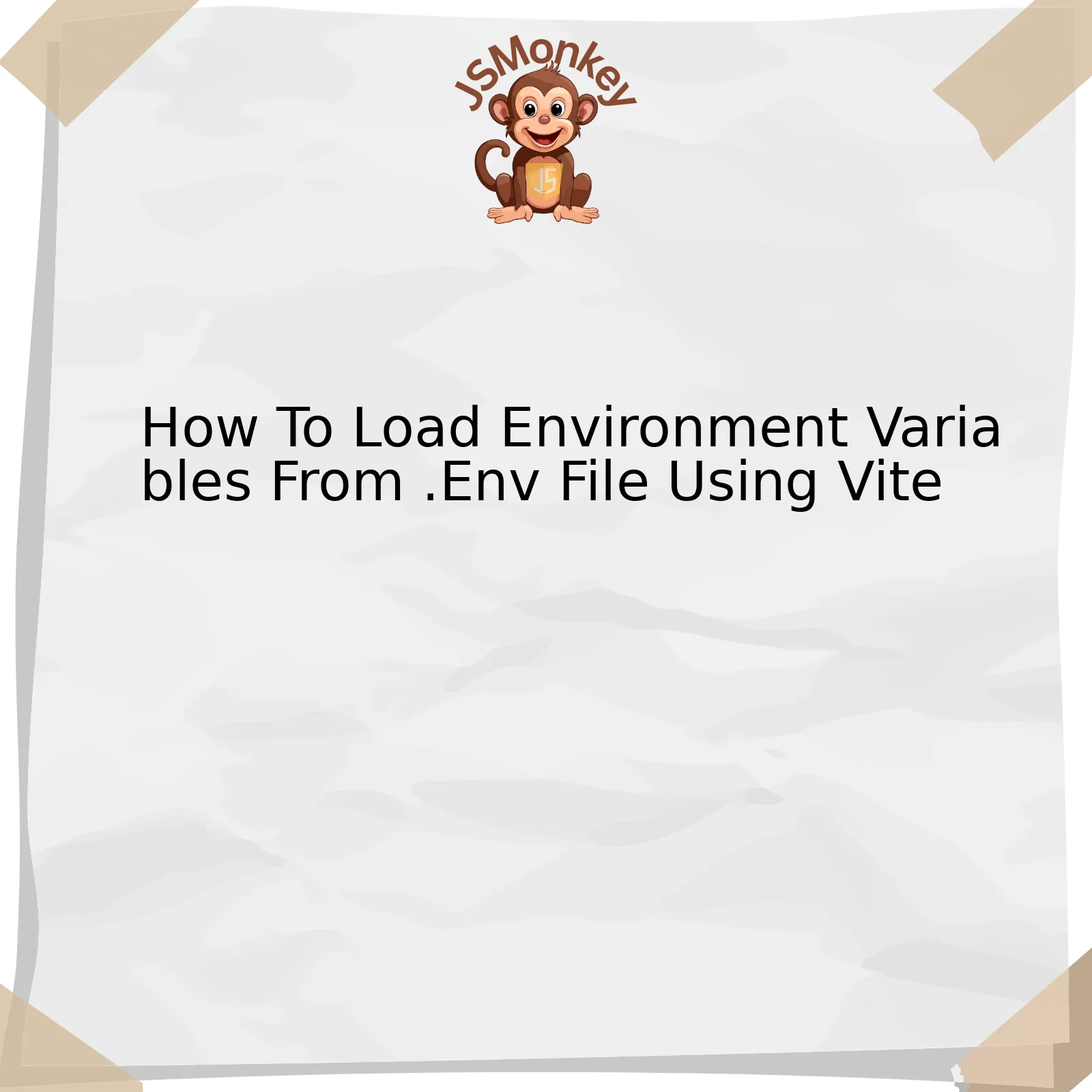
The Vite JavaScript tool allows you to fluently load environment variables from a .env file. The actions involved are quite simple and direct, and can be efficiently accomplished if the steps below are adhered to:
Step | Action |
---|---|
Create .env File | In your project root directory, first, create a .env file that will hold your environment variables.
touch .env |
Add Environment Variables | Open the .env file in any text editor and start defining your environment variables.
VITE_APP_TITLE=MyApp VITE_API_KEY=123456 Remember, all variable keys in the .env file must be prefixed with VITE_ to be imported into your Vite application. |
Access in Vite Application | In your Vite application, access the environment variables using process.env.
console.log(process.env.VITE_APP_TITLE); // 'MyApp' |
Once these steps have been followed, your Vite application should be set and ready to utilize the environment variables loaded from the .env file.
It’s worth highlighting that Vite embraces the novel concept of import.meta.env for handling environmental ties within its build system, thus providing an effective alternative to the conventional process.env used by tools like Webpack.
This significant feature plays out beneficially for loading environment variables as it assists developers to smoothly manage varying development setups and deployment configurations in a straightforward manner, without having to cycle through complex setup routines. Do remember to prefix your .env variables with “VITE_” in order to gain access to them.
Every developer has their own unique toolkit and workflow; but when it comes to managing sensitive data, we all have one thing in common: “It’s never a good idea to put secrets directly into the code,” as noted by famed technology author and founding engineer at Scalyr, Steve Newman. With tools like Vite, you can keep those secrets safe and sound in your .env file, away from prying eyes looking to take advantage of exposed confidential information.
Leveraging Vite for Loading Environment Variables
Loading environment variables from a .env file is a best practice in application development that allows you to keep sensitive information, such as API keys or database user credentials, separate from your codebase. This makes your application more secure and easier to manage since changes to the environment do not affect the underlying application logic.
Leveraging Vite, a build tool created by Evan You, offers us a efficient way to load environment variables from .env files. Let’s delve into how it can be achieved:
This process occurs in three main steps:
Create .env File:
Create a new file titled “.env” in your project root. This file will house all environment variables; for instance, an API key might be stored as follows:
API_KEY=MY_API_KEY
Access The Variables in Vite:
Here’s the interesting part; Vite automatically loads environment variables prefixed with VITE_. To use our previously-defined API key, it would need to be renamed to
VITE_API_KEY
in the .env file.
Utilize the Variables within Your Code:
Vite makes these environment-specific settings available on the
import.meta.env
object. Like other properties of this object, our API key can be referenced with
import.meta.env.VITE_API_KEY
.
Step | Create .env file | Access the Variables in Vite | Utilize the Variables within Your Code |
---|---|---|---|
Example | API_KEY=MY_API_KEY | Rename to VITE_API_KEY | Reference with import.meta.env.VITE_API_KEY |
In the words of Linus Torvalds, “Most good programmers do programming not because they expect to get paid or get adulation by the public, but because it is fun to program.” Loading environment variables in such an efficient manner not only secures your application’s sensitive data but also adds to the After all, coding should be enjoyable as well as functional. Vite, with its streamlined approach, assists us in achieving both.
To note, keep in mind that if the codebase needs to be shared then you might want to replace the actual values in .env with dummy data or add .env to .gitignore so it’s not committed to Git. Always remember the golden rule of application development: never commit sensitive data.
Remember too that while Vite makes accessing environment variables easy from our JavaScript and TypeScript files, this won’t work in our HTML files. In those cases, we would need use Vite’s built-in templating system. Finally, bear in mind these loaded variables are made available during runtime, hence won’t be available during Node.js scripts execution like vite.config.ts.
Understanding the Role of .env File in Vite
The `.env` file plays a vital role in managing the various environments in which your Vite applications run. This is especially useful when working with a tool like Vite, as it helps to standardize and streamline configuration between different instances (like development, testing, production, etc).
Understanding the power of `.env` files courtesy of `dotenv`-related technologies that parses these files to make environment variables available within your application, let’s delve into how you can load environment variables from a `.env` file using Vite.
Loading Environment Variables using Vite
Often we would require certain configurations to change based on our environment. For example, the API endpoint might be different for the development and the production environment. We then use Vite’s special handling of environmental variables.
Vite has built-in support for loading environment variables from `.env` files in your project root. It utilizes a combination of the project root, mode, and a `.env` extension to define the name of the `.env` file.
For example, suppose you have a development mode. The file will be named `.env.development`. If no mode is specified, Vite defaults to the `development` mode. These environment variables are loaded at the start up of your Vite service.
A hypothetical example follows:
// .env.development file VITE_APP_TITLE=My App (Dev)
In the above code, `VITE_APP_TITLE` is now accessible in both the server and client side of your Vite application via `import.meta.env.` as seen below:
console.log(import.meta.env.VITE_APP_TITLE) // Outputs: 'My App (Dev)'
It’s important to note that only variables prefixed with `VITE_` are exposed to your Vite application.
Securing .env
Considering the sensitive nature of environment variables, it’s essential to keep them secure. One method is to add `.env*` in your `.gitignore` file to prevent inadvertent upload of confidential data to your repository.
To wrap up, making use of Vite’s powerful environment handling along with the `.env` file is an excellent way to manage multiple setups, ensuring smooth transition and error-free execution. As Jeff Atwood, co-founder of Stack Overflow says: Any application that can be written in JavaScript, will eventually be written in JavaScript.
So why not take advantage of all tools at our disposal to make the process more efficient and enjoyable?
I would also like to suggest visiting the official [Vite documentation](https://vitejs.dev/guide/env-and-mode.html) on Environment Variables for a more in-depth look.
Dissecting the Process: Loading .Env File Using Vite
Diving into the process of loading environment variables from a `.env` file using [Vite](https://vitejs.dev/) unfolds exciting facets of this modular and light-weight JavaScript-oriented web development build tool.
Starting at the fundamental level, an environment variable is a dynamic-named value that changes the way various applications behave on a user’s computer. Developers often stuff environment variables into a `.env` file, hence making these accessible to their software.
//.env file DB_HOST=localhost DB_USER=root DB_PASS=s1mpl3
In Vite, making use of these environment variables becomes efficacious due to its built-in support for loading them. Following are the steps involving how to load these variables:
– Establishing a new `.env` file in your project root.
– Popping variables into this file, like `VITE_APP_Title=My_Vite_App`.
Note: Prefix them with `VITE_`. It allows them to be accessed in client source code. Server-side counterparts will need to prefix the key with `VITE_` to expose a variable to Vite’s server context and the browser.
// .env file VITE_APP_TITLE=My Awesome Vite App
– Making use of these variables in your project as `import.meta.env.VITE_APP_Title`.
console.log(import.meta.env.VITE_APP_TITLE); // Outputs: My Awesome Vite App
To conclude, every time you run your Vite application (`npm run dev`), Vite reads data from `.env` file and tucks those variables into `import.meta.env`.
In words of Jeff Atwood, “Coding with unclear future requirements is like walking through mud, both slow and messy”, so understandably, having a clear understanding of environments gives us a clean start. This process not only ensures readability but also minimizes potential misconfigurations in diverse development stages.
Lastly, being cautious with .env files is necessary. Ignoring them in .gitignore will rule out the inadvertent commit of confidential data into the Github repository.
/ .gitignore .env
In this rapidly evolving technological world, making use of tools like Vite that streamline developers’ work is an astute step. It empowers them to build faster and efficient apps with ease, without having to worry about configuring environment variables. With Vite, loading `.env` variables becomes a breeze, hence fuss-free coding!
Error Solutions and Best Practices When Implementing Environment Variables with Vite
When interacting with Vite, managing environment variables can require attention to detail and adherence to best practices. Particularly when attempting to load these variables from a .env file, there may be potential for errors if not implemented properly. However, working around these issues can be achieved intelligently and satisfactorily with the right approach.
Environment variables in Vite, similar to other tools, are instanced that store data outside your code that can be switched out without altering your application’s source code. It enhances security and portability of your application. However, by loading them from a .env file, you must consider the file’s location, naming convention and syntax correctness to prevent potential pitfalls.
Understanding how Vite works with .env files is crucial to overcoming any implementation difficulties. Let’s take a look at some Errors that might occur during the implementation and talk about best practices.
Error Solutions & Troubleshooting Techniques
Misspelled Variable Names:
One common error encountered is misspelling the variable names in your .env file or where it’s used. They may lead to “reference error”, To avoid it.
– Double-check your variable names.
Empty Variables:
Another potential issue arises if a variable is declared within your .env file, but not assigned a value. This could lead to potential results such as ‘undefined’. To avoid those situations:
– Always assign values to your variables.
Sensitive Information Disclosure:
Unintentionally committing the .env file to version control systems such as GitHub can disclose sensitive information. To avoid accidental commits
– Include .env in your .gitignore file to prevent this.
Passing Environment Variables to Client:
With Vite, any variable named VITE_* in .env will be exposed to your client-side JavaScript. If not careful, this could unknowingly expose sensitive server-side data. Therefore,
– Be cautious of what you name your environment variables.
Best Practices
Some recommended practices when working with .env and Vite includes:
– Namespace your variables: It’s advised to prefix all environment variables with ‘VITE_’ as required by Vite. It allows Vite to pass those variables into your client bundle.
VITE_APP_TITLE=SOMETHING
– Use Default Variables: Defining default values for variables is an excellent safety practice. This can be done using
process.env.VARIABLE_NAME || 'default value'
– Keep it Private: To prevent unintentional exposure of credentials, use a .env.local file for sensitive data. Make sure you include .env.local in your .gitignore as well.
– Consistency: Maintain consistent names for the same types of data across different environments. Don’t call it DB_USERNAME in one place and DB_USER elsewhere.
Remembering what [Edsger Dijkstra](https://www.brainyquote.com/quotes/edsger_dijkstra_138681) once pointed out about the nature of computation – “Computer Science is no more about computers than astronomy is about telescopes” – keep in mind that ensuring the efficient performance of a line of code matters far more than writing it does, and this is especially true when it comes to environment variable implementation in Vite.
The process of loading environment variables from a .env file using Vite encourages ease and efficiency in application development. By enabling developers to securely manage and access sensitive data, Vite has effectively optimized the usage of environment variables.
Discovering the methods for loading such variables involves creating a .env file at the root level of your project. Remember, syntax is key. Each variable should be prefixed with “VITE_”. An example of how this may look is:
VITE_APP_TITLE=My App
Running commands like “npm run dev” (Node.js Package manager) ensures environment variables are loaded within Vite. Indeed, as specified in the Vite documentation (Vite Environment And Mode) , these variables become accessible on the special ‘import.meta.env’ object in our Javascript.
However, there’s a cautionary note to handling environment variables. Developers need to ensure such data doesn’t get exposed within client-side code, especially when it contains sensitive information, making security a paramount concern while advanced applications evolve. As Edsger W.Dijkstra, a famous Dutch systems scientist perfectly encapsulates, “Simplicity is prerequisite for reliability.”
Subsequently, Vite provides an excellent solution to managing environment variables. Facilitating accurate utilisation within our projects, it minimises the risk of divulging sensitive information. Thus, importing a .env file in Vite significantly contributes to improving user experience, boosts the development process’ security, and permits robustness amidst fast-evolving technical advancements.