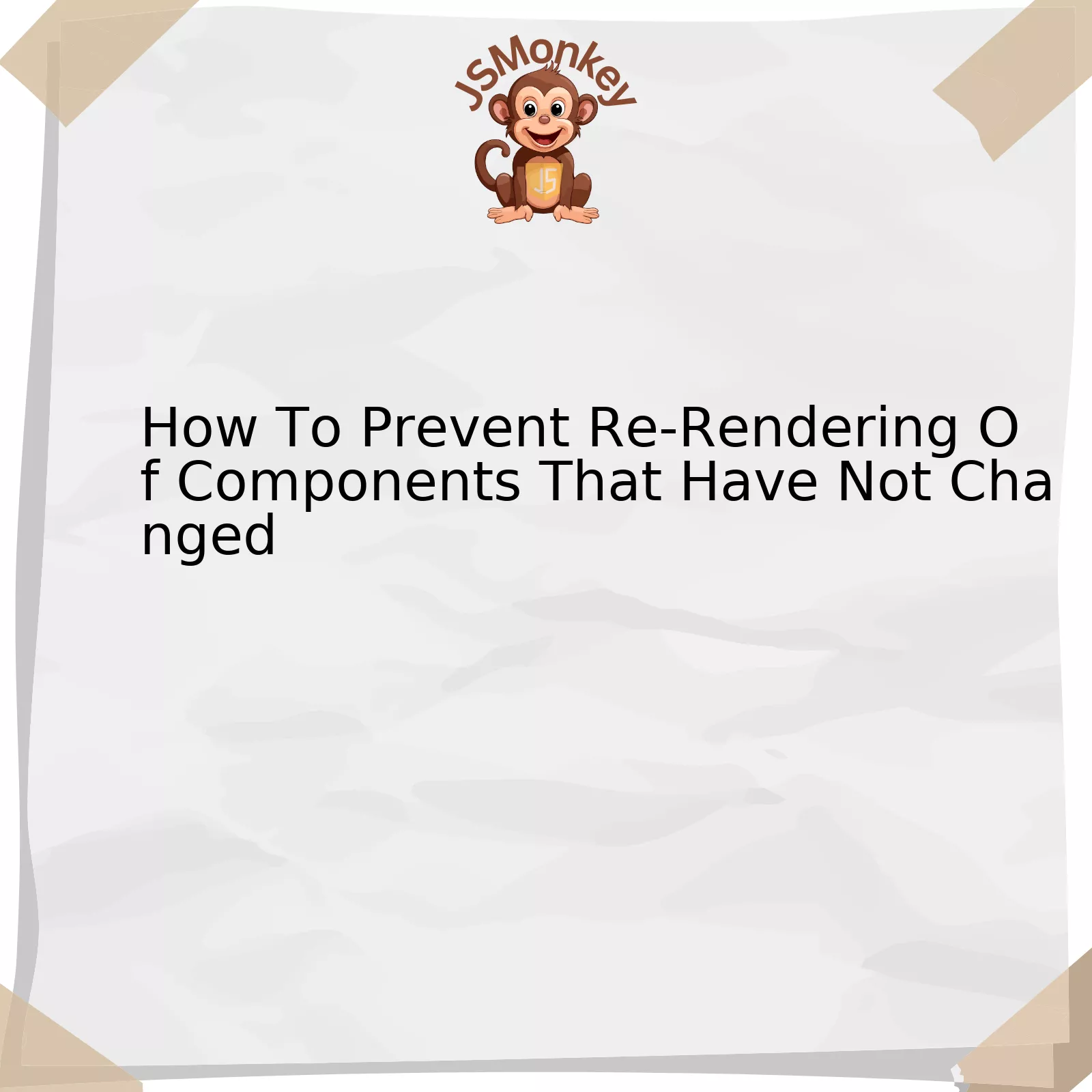
When it comes to optimizing a JavaScript application, specifically one that is built with a modern framework like React, avoiding unnecessary re-renders of components is key. This forms part of the core concepts in any efficient JavaScript codebase. Thankfully, various strategies can help a developer prevent unwanted re-rendering. A visual representation detailing some preventative methods is as follows:
<table> <tr> <th>Method</th> <th>Description</th> </tr> <tr> <td>ShouldComponentUpdate</td> <td>A lifecycle method in which you can add logic to decide if a component should re-render or not.</td> </tr> <tr> <td>React.PureComponent</td> <td>A type of component that only re-renders when there's a shallow difference in state or props.</td> </tr> <tr> <td>React.memo()</td> <td>It memoizes a component, preventing re-renders when prop changes do not reflect on the output.</td> </tr> <tr> <td>useMemo() Hook</td> <td>A hook to avoid expensive calculations on each render unless specific inputs have changed.</td> </tr> <tr> <td>useCallback() Hook</td> <td>Returns a memoized version of the callback that only changes if one of the dependencies has changed.</td> </tr> </table>
The table depicts five different strategies to avoid unnecessary re-renders in React.
– The ShouldComponentUpdate method gives developer control over whether a component should re-render by specifying conditions in which it should do so. However, caution is needed as improper use can lead to subtle bugs.
– The React.PureComponent does a shallow check for prop and state change and therefore, only re-renders when it detects a difference in its state or props.
– React.memo() wraps functional components and optimises them by preventing re-render, especially when prop changes do not impact the output of the render method.
– useMemo() Hook retains the expensive computation result and returns it during subsequent re-renders unless the specified inputs change. This avoids unnecessary computations thereby improving performance.
– Lastly, the useCallback() Hook returns a memoized function, that only updates if its dependencies change, avoiding potential excessive operations.
These methods can significantly enhance the performance of your app by reducing wasteful re-rendering activities. While the prevention of re-renders is crucial, we must also ensure that it doesn’t lead to stale UI or introduce complex patterns into our codebase.
As Steve Jobs once said, “Simple can be harder than complex…But it’s worth it in the end because once you get there, you can move mountains.”source Maintaining this simplicity while optimising the performance of our JavaScript applications is the goal we should all aim for.
Leveraging React.memo for Component Optimization
The React.memo function is a higher order component which allows us to optimize our functional components to prevent unnecessary renders. Optimization of component rendering in React can be instrumental for boosting the performance of your application.
React.memo is used primarily to wrap functional components for optimization purposes. Its primary role is to memorize the previous rendering’s output and prevent needless re-renderings by comparing the present and past props. With React.memo, components that have not changed will not be re-rendered during an update, ultimately optimizing overall performance.
For instance, imagine we have a component called
MyComponent
that receives props. Without any optimizations, anytime the parent component updates (which changes the props),
MyComponent
will be re-rendered even if the properties that it depends on did not change.
Using React.memo might look like this:
const MyComponent = ({ propA }) => { // Component logic here... return ( <div> I'm a memoized component that only depends upon propA! </div> ); }; export default React.memo(MyComponent);
In this example,
MyComponent
will only be rerendered if
propA
has changed.
Do keep in mind, using React.memo when unnecessary or inefficiently could become costly. Memoization comes with its own trade-off: while it helps avoid expensive calculations by storing the previously computed result, it also adds overhead as it needs to compare the old and new props.
As stated by Donald Knuth, “Premature optimization is the root of all evil”. When leveraging React.memo, we should apply it judiciously and not overuse it. It may seem tempting to wrap all components with React.memo to prevent unnecessary renders, but remember that memoization isn’t cost-free. It can both give your application a performance boost when used correctly and incur a memory cost when abused.
Refer to the official React documentation to learn more about [React.memo](https://reactjs.org/docs/react-api.html#reactmemo) function.
Understanding Pure Components in React
React and PureComponent share an important objective; to optimize rendering behavior, making applications faster and more reliable. In the core concept of React, all components re-render when a state or prop change occurs. This frequent re-rendering might seem harmless but primarily affects the overall performance of the app. When it comes to solving this performance issue, React offers PureComponent.
A
PureComponent
is a type of component offered by React which brings in a built-in shouldComponentUpdate method, for the purpose of performance optimization. The
shouldComponentUpdate
method determines whether a re-render is needed or not, saving unnecessary renders.
The critical difference between Component and PureComponent lies in their default implementation of shouldComponentUpdate method.
Component | PureComponent |
---|---|
For components that extend from React.Component, the shouldComponentUpdate lifecycle method returns true by default. | For a PureComponent, the shouldComponentUpdate method has a shallow comparison mechanism for props and state changes. |
With this functionality, if the state or props for a PureComponent have not changed, then React will skip rendering that particular component. It’s as simple as not calling its render function, hence optimizing the performance by avoiding unnecessary DOM operations.
Here’s an implementation example:
import React, { PureComponent } from 'react'; class ExampleComponent extends PureComponent { render() { return ( //...render logic ) } }
In this code snippet, ExampleComponent extends PureComponent instead of Component, and takes advantage of the pure-render-mixin provided with React out-of-the-box.
However, it’s essential to note that PureComponent performs a shallow comparison on state and props. If these contain complex data structures, it may produce false-negatives for deeper differences. If the kind of data your component is dealing with has nested data structures then you most likely should use a deep comparison library or, in certain scenarios, resort to using your custom logic inside shouldComponentUpdate.
As Dan Abramov, co-creator of Redux and part of React team at Facebook beautifully quoted,
“Write code as if it is executed only once and then it’s cached.”
This aligns very well when we think about use case of PureComponent, where we aim to write our render methods so that they won’t have side effects and render output can be re-used if inputs are same.
You see, understanding PureComponents and implementing them judiciously can help developers solve possible optimization issues arising from unnecessary re-renders. This way, a perfectly optimized React application is built, offering an intuitive end-user experience.
Employing shouldComponentUpdate for Re-render Prevention
The `shouldComponentUpdate` lifecycle method in React can be cleverly used to enhance the performance of a large application by preventing unnecessary re-renderings of components that have not changed. This fits seamlessly into an analytical portrayal of how to avoid superfluous component re-rendering to foster faster, more efficient real-time web apps.
React uses a mechanism known as reconciliation to update and render components when changes happen in the data model. However, this can sometimes waste computational resources if components are re-rendered though their prop and state data remain the same. Here’s where the magic of `shouldComponentUpdate` comes into play.
class CustomizedComponent extends React.Component { shouldComponentUpdate(nextProps, nextState) { return nextProps.value !== this.props.value; } render() { return <div>{this.props.value}</div>; } }
In the above code snippet, the `shouldComponentUpdate` function helps bypass the render operation when there’s no change in the value of props of `CustomizedComponent`. It only invokes a new rendering process when `nextProps.value` differs from `this.props.value`.
This is ‘deterministic rendering,’ as outlined by Adrian Holovaty, a renowned technologist: “Deterministic rendering means less bugs, dependable performance, and fewer headaches down the road.”
However, it’s crucial to apply `shouldComponentUpdate` thoughtfully. While it saves unnecessary rendering, inappropriate use could lead to bugs or outdated user interfaces. It’s discouraged to use `shouldComponentUpdate` while working with the Redux library or when you intend to implement PureComponent because they already incorporate similar mechanisms for performance optimization.
On another note, in functional components, React provides a similar hook called `React.memo`, which wraps around the component and performs a shallow comparison to prevent needless re-renderings.
The idea behind these practices is not just about stopping needless re-rendering but also is about creating more efficient, reliable, and faster web apps. By discerning what needs an update and what doesn’t, we ensure that the browser’s computational resources are used optimally.
Want to delve deeper? Check out the official React documentation on `shouldComponentUpdate` located here: React Optimizing Performance Instructions.
React’s Virtual DOM and Reconciliation Process
React, a prominent JavaScript library often utilized for building user interfaces specifically for single-page applications, implements a unique mechanism known as the Virtual DOM that notably enhances application performance. Comprehending the operation of the Virtual DOM and its reconciliation process lays the foundation for understanding how to stop unnecessary re-rendering components — a key strategy for optimizing app performance.
The primary concept of the Virtual DOM is it is an abstract form of the actual (Real) DOM. React builds this lightweight copy of the real DOM in memory and uses it to compute what transformations need to be applied without directly manipulating the real DOM. Essentially, every time state or props change, instead of updating the real DOM right away, React creates a new Virtual DOM and compares it to the old version. The process of comparison is known as Reconciliation.
A vital attribute of the reconciliation process comes in the form of “diffing,” where React strategically determines which parts of the virtual DOM have changed compared to the previous instance before concluding what precise updates require to be rendered on the real DOM.
Yet, achieving optimal efficiency when working with React depends significantly not only on understanding the mechanics of the Virtual DOM and reconciliation but importantly, deploying ways to prevent needless re-renders of unchanged components. One preferred method would involve utilizing a core feature of React called `shouldComponentUpdate()`. This lifecycle method allows control over whether a component should re-render or not by returning ‘true’ or ‘false’. For instance:
html
shouldComponentUpdate(nextProps, nextState){ if(this.props.contact === nextProps.contact && this.state.status === nextState.status){ return false; } else { return true; } }
In this code snippet, `shouldComponentUpdate()` performs a check to determine whether prevState and nextProps differ from their current state and prop values. If no difference exists, then the component does not proceed to render again, thus improving performance.
Another notable effectual technique to prevent unnecessary re-renders is Pure Components. A PureComponent performs a shallow comparison on the state and props, and if no changes are detected, it prevents a rerender. This can significantly improve performance in heavy render applications. However, bitwise caution must be taken while using PureComponent as the shallow prop and state comparison might lead to missed updates when deep properties change.
An additional optimization strategy is the decorous use of React’s `React.memo()`. `React.memo()` is a higher order component that memorizes the output of a function component and then returns this output if given the same inputs again, thus avoiding needless rendering. Applying `React.memo()` could look like this:
html
const MyComponent = React.memo(function MyComponent(props) { /* only rerenders if props change */ });
Here, `React.memo()` is wrapping around our component, which informs React to memorize and cache the component’s rendered output and then skip needless re-rendering whenever identical props are received.
As Paul Graham, co-founder of Y Combinator, remarked, “In programming, as in many fields, the hard part isn’t solving problems, but deciding what problems to solve.” By discerning React’s Virtual DOM intricacies and reconciliation process, developers prescribe themselves with powerful tools to keep their applications performant by skillfully averting needless component re-rendering. Remember, knowing when not to work is as important as knowing when to.
For additional expansive understanding about React’s Reconciliation, check out React’s official documentation.
In light of this engaging and in-depth exploration on preventing unnecessary re-rendering of components in Javascript, here are the key takeaways:
– Utilizing React’s PureComponent or `React.memo()` function can effectively avoid unnecessary re-renderings as they perform shallow comparison of props and state. They ensure that a component only re-renders when actual changes occur in its state or props.
javascript
class MyComponent extends React.PureComponent {
// Your code here
}
// OR
const MyComponent = React.memo(function MyComponent(props) {
// Your code here
});
– Additionally, optimizing the use of callbacks and event handlers can play an instrumental role in reducing superfluous re-renderings. Defining event handlers inside a component often triggers needless re-renders. A probable solution could be implementing callbacks outside the render method or employing `useCallback` Hook.
javascript
function Parent({ onClick }) {
return
}
// Callback defined outside the render method
function handleClick() {
console.log(‘Clicked’);
}
ReactDOM.render(
// OR Using `useCallback` Hook
function Parent() {
const handleClick = useCallback(() => {
console.log(‘Clicked’);
}, []);
return
}
ReactDOM.render(
– The usage of Redux, despite being outside the scope of React itself, also offers ways to prevent unnecessary re-renderings by using Reselect library to create memoized selectors [Reselect Library](https://redux.js.org/usage/deriving-data-selectors). This enables state changes to bypass components that don’t need updating, leading to optimized performance.
As Bill Gates once stated, “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency.” It’s in this spirit that understanding and applying these strategies can yield significant improvements in your applications’ performance. Remember, preventing needless rendering matters not simply for maintaining clean code, but can have tangible enhancements on user experience as well.