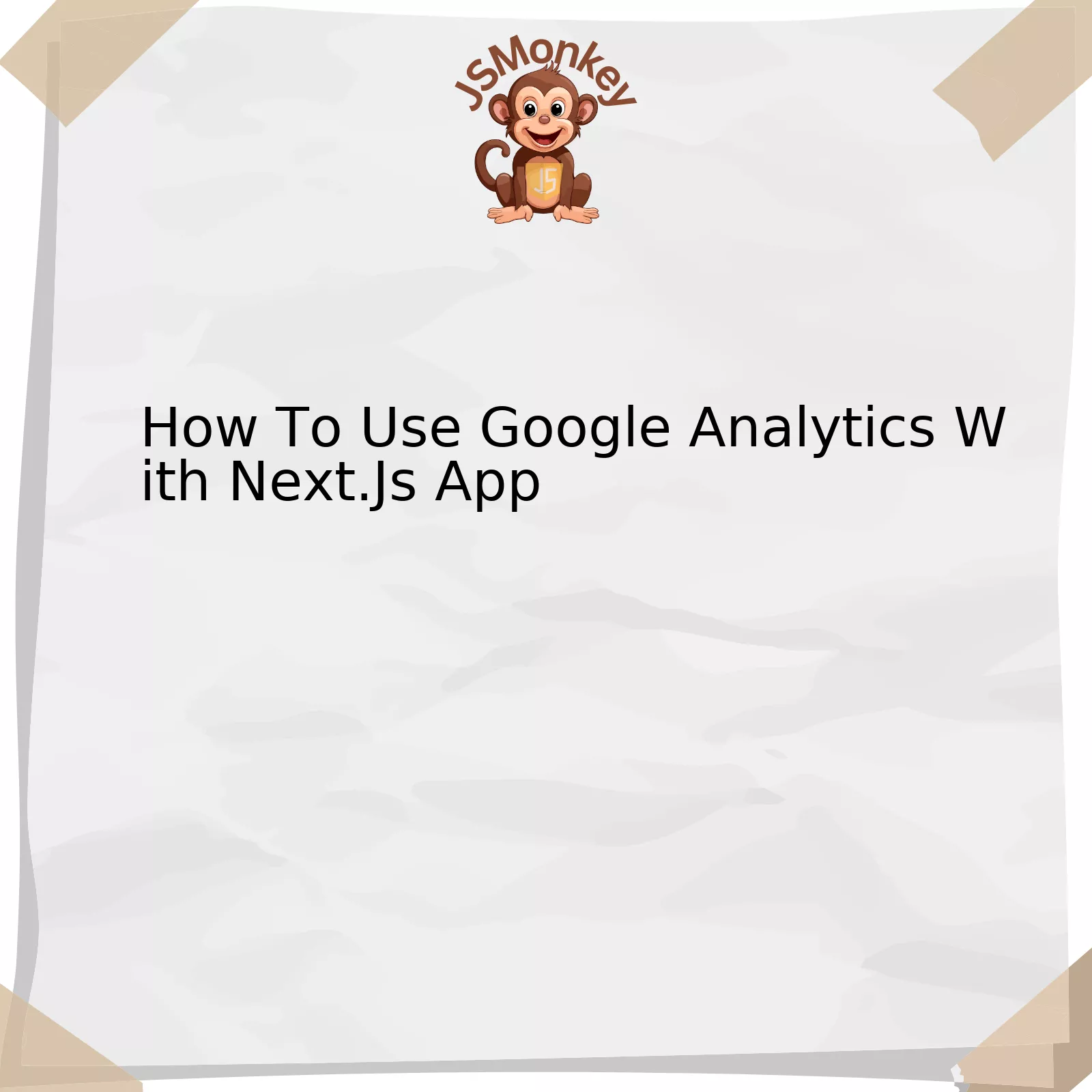
To incorporate Google Analytics with your Next.JS application, you can follow these steps as outlined in the table:
Step | Description |
---|---|
Create a Google Analytics Account | The first step involves setting up a Google Analytics account where all the website’s traffic data will be collected. |
Set Up a New Property in Google Analytics | Setting up a new property initiates the tracking process. Here, you’ll specify the Next.js app you want to track, and GA will provide the unique tracking ID for your website. |
Add the GA Tracking ID to Your Next.js Website | Incorporate the tracking ID into your Next.js application by inserting it into a tracking snippet, which will be placed in the Head component of your pages. |
Analyze Traffic Data | Once set up, you can start to analyze the incoming traffic data in your Google Analytics dashboard. |
The procedure can be further unwrapped to shed more insight:
1. When starting with Google Analytics, each user is required to set up an account on the analytics platform. This allows Google to consolidate essential data metrics relating to your website engagement.
2. After creating an account, a new “property” has to be established for every exclusive site that needs monitoring. The term ‘property’ refers to specific web-resource related to our Next.js application. Upon configuration, Google provides a unique ID called the Tracking ID.
3. This tracking ID plays a significant role in observing user interactions on your Next.js application. To implement this, Google shares a tracking snippet that is to be manually inserted into the header section of each webpage you intend to gather data from.
4. Once the tracking ID has been integrated successfully with your Next.js app, Google Analytics starts to collect and assess performance data. This proffers invaluable insights regarding crucial user metrics like demographic details, session durations, bounce rates, amongst others.
As renowned computer scientist Grace Hopper once said, “The most important thing about a technology is how it changes people.”
This brilliant quote sums up the use of Google Analytics with a NextJS application, it’s not just about integrating a technology or tool, but understanding how it helps create better experiences for users, thus driving positive change.source
Utilizing Google Analytics for Improved Next.Js Application Insights
In the realm of modern web development, Next.js has emerged as a potent tool for building highly efficient and SEO-friendly applications. It combines the capabilities of React, JavaScript, and Node.js, enabling developers to create scalable, server-side rendering (SSR) and statically generated web projects. However, building these applications requires developers to be in tune with their audience for optimization purposes. One of the most reliable ways to gain productive insights about users is by incorporating Google Analytics into your Next.Js application.
Google Analytics provides detailed statistics about website traffic, user behavior, and performance metrics that assist developers in understanding their audience better, thereby making crucial decisions regarding app improvements or modifications. Harsha Jeswani, a seasoned tech lead at an AI and ML technology company iterates, “Tracking user interaction gives you improved perspective on which areas of your site are doing well and where there is need for improvement.”
To connect Google Analytics with your Next.js app, there are steps you’d generally want to follow:
– Obtain your Google Analytics Tracking ID: Create a Google Analytics account if you don’t have one already. Once done, collect your unique tracking ID by going to Admin > Property Settings.
– Install google-analytics library: Your Next.js project will need the `react-ga` (React Google Analytics) library which can be installed using npm (`npm install react-ga`) or yarn (`yarn add react-ga`).
– Initialize Google Analytics: In the `utils` directory of your Next.js project, create a new file (say `Analytics.js`). Use the `initialize()` method from `react-ga` to initialize Google Analytics with your unique tracking ID.
import ReactGA from 'react-ga'; export const initGA = () => { ReactGA.initialize('Your-Google-Analytics-Tracking-ID'); };
It’s important to note that you should be initializing Google Analytics only once. An ideal way to ensure this is by initializing it within `componentDidMount()` lifecycle method or `useEffect` hook in your main component.
– Implement Page Views Tracking: Use the `pageview()` method from `react-ga` to track individual page views.
export const logPageView = () => { ReactGA.set({ page: window.location.pathname }); ReactGA.pageview(window.location.pathname); };
Invoke this function in your main component’s `componentDidMount()` lifecycle method or `useEffect` hook, anytime the route changes.
With such integration, you can glean useful insights about user interactions, and optimize your Next.js application accordingly.
You’ll find other ways to enable Google Analytics on your Next.js application on sites like Next.js Documentation and forums like StackOverflow.
Incorporating Google Analytics into your Next.js application not only augments your understanding of user behaviors but also provides crucial data which helps in optimizing the overall application performance for an improved user experience.
It’s aptly quoted by Bill Gates, “If you can measure it, you can manage it.” Therefore, leveraging such tools to understand your metrics better should always be a top priority while developing web applications.
Deep Dive: Setting up Google Analytics in a Next.Js App
Integrating Google Analytics into your Next.js applications allows you to make data-driven decisions. This integration provides the vital metrics about the behaviour of your users when visiting your web application.
You can start with installing the necessary package, `react-ga`, which is a lightweight Google Analytics module for React (which also works well with Next.js). To install `react-ga` via npm, use the following command:
npm install react-ga
Once installed, set up an analytics utility file that handles the logic related to Google Analytics:
// utils/analytics.js import ReactGA from 'react-ga' export const GA_TRACKING_ID = 'your_tracking_id' // This is your GA Tracking ID // https://developers.google.com/analytics/devguides/collection/gtagjs/pages export const pageview = (url) => { ReactGA.set({ page: url }); ReactGA.pageview(url); } // https://developers.google.com/analytics/devguides/collection/gtagjs/events export const event = ({ category, action, label }) => { ReactGA.event({ category, action, label }) }
The `event` function will help send events that occur on your application to your Google Analytics dashboard. These events could include things like button clicks, form submissions, among others.
Next up is integrating the Google Analytics library in the main application file. In `_app.js` file of Next.js, we can insert below code:
import { useEffect } from 'react'; import Router from 'next/router' import * as ga from '../utils/analytics' const MyApp = () => { useEffect(() => { const handleRouteChange = (url) => { ga.pageview(url) } Router.events.on('routeChangeComplete', handleRouteChange) return () => { Router.events.off('routeChangeComplete', handleRouteChange) } }, []) return ( /* Your app render */ ) }
This effectively tells Next.js to send a pageview event to Google Analytics every time the route changes, i.e., when a user navigates to a different page in your application.
Remember to replace `’your_tracking_id’` with a valid GA tracking ID. It can be found in your Google Analytics account.
Keeping these points into consideration will make sure that your application’s integration with Google Analytics in Next.js is achieved correctly and all necessary metrics are tracked effortlessly.
As famously quoted by Dijkstra:
“Program testing can show the presence of bugs, but never their absence.”
Just as testing is important in development, gauging user interaction is critical for understanding user behavior and making improvements. Google Analytics plays a crucial role in helping us achieve exactly that. This combined with a powerful framework like Next.js can enable developers to build very efficient, scalable and user-friendly applications. You can find more information [here](https://developers.google.com/analytics/devguides/collection/gtagjs/pages).
Exploiting User Metrics: The Power of Google Analytics in Your Next.js Project
Google Analytics is a remarkably potent tool for developers and site owners to utilize, giving valuable insights into user behavior on your website. When it comes to using it with Next.js App, this integration can help you understand your users better, enhance their web experience, and ultimately, drive your business metrics.
Integration of Google Analytics with Next.js is brought about by installing the `react-ga` package, which simplifies connecting your Next.js app with Google Analytics:
npm install react-ga
Initiating Google Analytics calls is facilitated at the application `/pages/_app.js` file where you can populate global information about the website for every page:
html
import ReactGA from ‘react-ga’;
ReactGA.initialize(‘Your-Unique-ID’); //Replace ‘Your-Unique-ID’ with your Google Analytics Tracking ID
…
Navigation events also need to be tracked for each route change. Within the router events in Next.js, you will use `routeChangeComplete` as an indicator for when a different page is loaded.
html
import Router from ‘next/router’;
Router.events.on(‘routeChangeComplete’, (url) => ReactGA.pageview(url))
With this mechanism in place, Google Analytics gains broad visibility into your webpage’s performance and visitors’ activity, presenting you with detailed information such as:
• User demographics: Locations, age groups, gender, interests, and more.
• Webpage interaction: Pages per session, average session duration, bounce rate, and percent of new sessions.
• Audience behavior: New versus returning visitors, frequencies, recencies, and engagement details.
• Flow visualization: Navigation path users take through your website
Steve Jobs once noted, “Design is not just what it looks like and feels like. Design is how it works.” By leveraging Google Analytics within your Next.js project, it extends your comprehension of how users interact with your work, enabling you to enhance your website’s design and functionality. This augmented user experience leads to increased conversions, customer satisfaction, and repeat visits.
In integrating Google Analytics into the Next.js app project ecosystem, developers receive insights that can be leveraged to make strategic, informed decisions concerning their website’s performance. You’re no longer relying solely on your perceptions of what works but making adjustments based on objective data.
For more detailed instructions about this integration, take a look at [Google Developers’ comprehensive guide](https://developers.google.com/analytics).
Overall, Google Analytics is an invaluable tool for developers using the Next.js app system, working to create websites that capture and hold user attention while delivering a robust ROI by understanding not just what visitors do on their site, but why they do it. With Google Analytics, you’re better equipped to deliver an optimized web experience that aligns with your audience’s needs while driving business growth.
Enhancing Web Performance: Integrating Google Analytics and Next.js
Google Analytics, a comprehensive web analytics service offered by Google, is typically used to track and analyze website traffic. On the other hand, Next.js, one of the most popular React frameworks, is renowned for its capability to create highly efficient websites with server-side rendering.
To enhance web performance, it’s prudent to integrate Google Analytics with your Next.js app. Here’s why:
1. Insightful Data: By implementing this integration, you gain access to valuable user data. This means you can observe how users interact with your application in real-time – which pages they visit most, how much time they spend on each page, where your traffic is coming from, and more. This allows you to tailor your web application to better suit user needs, ultimately enhancing performance.
2. Page Load Efficiency: When correctly integrated, Google Analytics does not affect the loading speed of your Next.js app. Since Google Analytics operates asynchronously, it won’t slow down your page rendering; instead, it provides valuable insights while keeping your web performance optimized.
The first step in integrating Google Analytics with your Next.js app involves setting up Google Analytics. After creating a new property on Google Analytics, you obtain a tracking ID. This identifier is what links your application to your Google Analytics account, allowing the two to communicate.
Next, in your Next.js application, install a package called ‘next-ga’, designed specifically to debug and deploy apps with Google Analytics integration easily. Using
npm
, the command for installation would be:
npm install next-ga
To wrap your Next.js app for Google Analytics:
import Router from ‘next/router’; import withGA from "next-ga"; function MyApp({ Component, pageProps }) { return & lt;Component {...pageProps} /> } export default withGA("GA_TRACKING_ID", Router)(MyApp);
Replace ‘GA_TRACKING_ID’ with your Google Analytics Tracking ID. This provides an overarching layer for the integration between Next.js and Google Analytics.
Evaluate trends, monitor user engagement, and optimize your web application’s design and functionality by combining the powerful functionalities of Google Analytics with the robust structure of a Next.js app.
As Anthony Goldbloom, CEO of Kaggle emphasized, “We are entering an era where being able to command AI will become an essential skill…“. In our context, using Analytical tools like Google Analytics integrated with modern frameworks like Next.js indeed become an essential skill set to extract impactful insights about the application usage patterns, thereby facilitating more informed development decisions.(source)
Remember, data-driven enhancement strategies combined with modern toolkits can help maintain high web performance while delivering optimal user experiences.
In this era of web development, integrating your Next.js application with Google Analytics is a pivotal move to understand your website traffic and user behavior. One might wonder why this integration is necessary, but the benefits are innumerable.
• Spot trends and adjust strategy: By integrating Google Analytics with your Next.js App, you’re placing yourself ahead of the curve by gaining insights into critical data about your users. This allows you to identify patterns, spot emerging trends, and alter your engagement strategies accordingly.
• Improved User Experience: By understanding how users interact with your app, you can essentially work towards providing a better user experience based on your findings.
• Data-driven decisions: The union of Next.js and Google analytics not only present solid numerical data but also arm developers with the power of making informed, impactful decisions that cater to their target audience.
However, despite these compelling reasons, incorporating Google Analytics into a Next.Js app may pose as a perplexing challenge for many developers. Fear not! It’s simpler than you think.
Steps | Descriptions |
---|---|
Installation | Start by adding Google Analytics library to your project by installing the React Google Analytics module. |
Setting up Google Analytics | For this, you’ll need a tracking ID which can obtained from the Google Analytics account. Insert it in the appropriate place in your code. |
Implementing Google Analytics in Next.Js | Define some routes and pageviews to track the desired user activity on your App. For simplicity, leverage ‘useEffect’ hook for this process. |
Note: The precise code for installation and implementation can be found on Google Analytics’ official documentation. Google analytics Documentation Reference
To nudge you on a quoting note, as Grace Hopper – a computer scientist and US Navy Admiral known for her pivotal work in developing early programming languages – once said, “The most important thing I’ve accomplished, other than building the compiler, is training young people. They come to me, you know, and say, ‘Do you think we can do this?’ ‘I know you can do it’”. In the context of weaving Google Analytics into your Next.js app, remember that it’s a challenging yet achievable goal.
Exploring the tableau of analyzing user behavior metrics with Google Analytics in a Next.js app, we hope you’re inspired to deep dive into implementing this integration. It not only illuminates key user insights but also equips developers to use these findings to deliver an optimized user experience. The fusion of these platforms will unequivocally spur a paradigm shift in how you view data-driven decisions in the realm of web development.