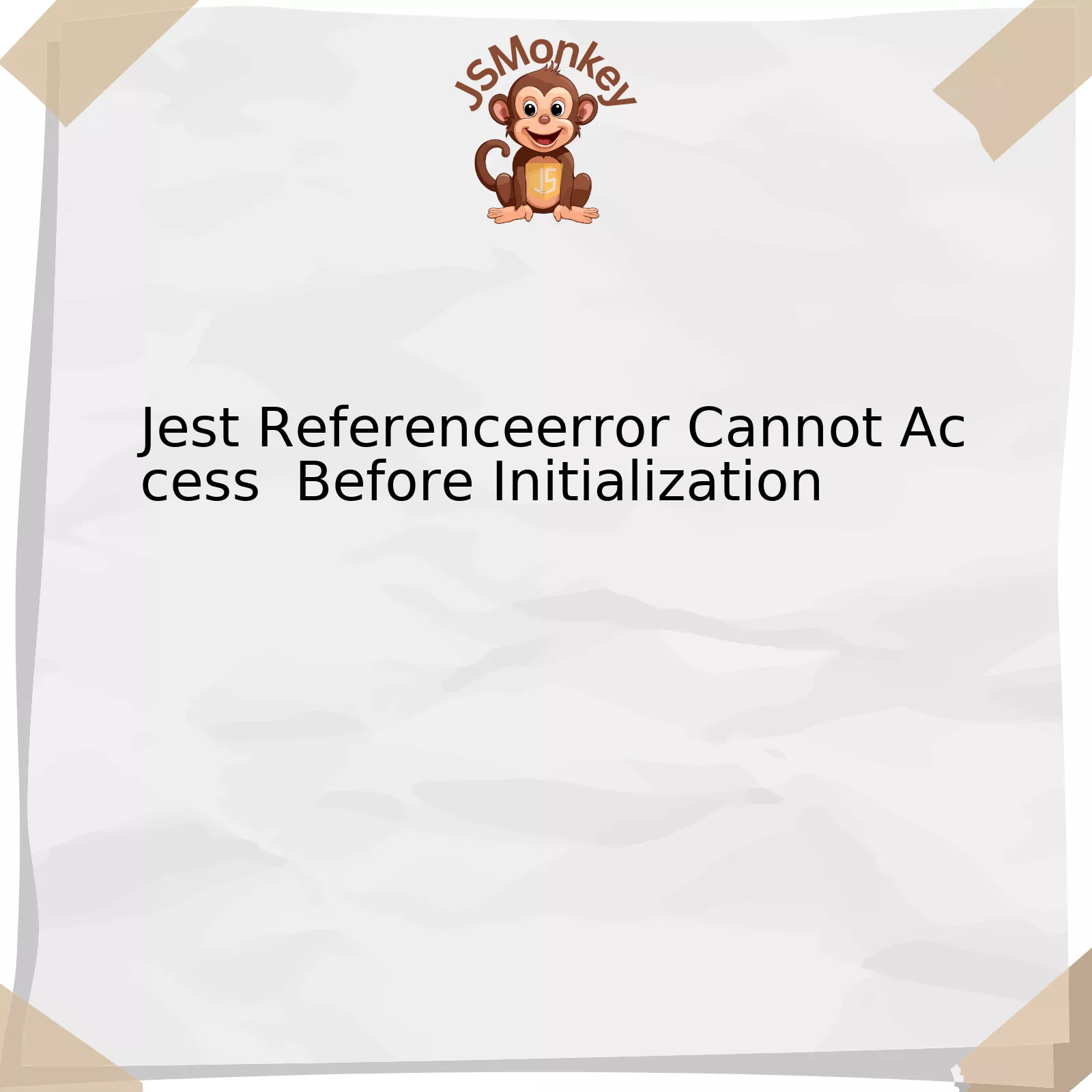
The error “Jest Referenceerror Cannot Access Before Initialization” is a common issue that developers encounter while unit testing with Jest. This error generally occurs when you attempt to utilize a variable before it has been defined or initialized causing the Jest framework throw this error.
Understanding the underlying concept of hoisting and the mechanisms of JavaScript’s Temporal Dead Zone can provide invaluable insight into why such an error may occur during unit testing scenarios within Jest.
Here, let’s break down the components concerning this issue via an HTML construct:
Term | Description |
---|---|
Jest | A delightful JavaScript Testing Framework with a focus on simplicity. |
ReferenceError | An error in JavaScript that occurs when you try to use a variable that does not exist. |
Cannot access before initialization | A specific type of ReferenceError that happens when a variable is accessed before it is defined due to the concept of hoisting. |
The Jest testing framework is popular because of its emphasis on simplicity and enabling easier debugging processes. However, like any tools, knowing the inner workings of JavaScript helps avoid pitfalls such as encountering a `ReferenceError`.
ReferenceError is one of standard built-in error types in JavaScript. It gets thrown when trying to dereference a variable that hasn’t been declared. In our case here, it’s more specific, indicating that there’s an attempt to access a variable before initialization.
This usually results from a concept known as “hoisting”. Hoisting in JavaScript moves variable and function declarations to the top of their containing scope during the compile phase. However, it’s critical to note that although the declarations are hoisted, the initializations are not.
Overstepping this boundary into what’s called the “Temporal Dead Zone”, a state where variables are considered declared but not yet initialized, triggers the error “Cannot access ‘variableName’ before initialization”.
To mitigate such an error, ensure you’ve properly initialized your variables before using them in your test cases. JavaScript, especially when used with Jest, demands a keen understanding of its behaviour to effectively exploit its testing capabilities.
Modern JavaScript explained by Kyle Simpson also advises:
> Be aware of hoisting mechanism in JavaScript. While it can be beneficial, inadvertently accessing a variable before initialization due to hoisting can lead to bugs hard to debug. Always initialize your variables appropriately.
Understanding ‘ReferenceError: Cannot Access Before Initialization’ in Jest
The error message ‘ReferenceError: Cannot Access Before Initialization’ in Jest means that you are trying to use a variable before it was initialized. This issue often arises due to the use of `let` or `const` with hoisting in JavaScript. Variables declared with `let` and `const` are hoisted to the top of their containing scope like `var`, However, with a subtle difference known as temporal dead zone – a period where they cannot be accessed until they’ve been declared.
For instance, consider this code:
html
console.log(myVariable); let myVariable = 'test';
In this case, ‘myVariable’ would throw out the error because the variable is being defined after the call.
Breaking down the “Cannot Access
– `ReferenceError`: This shows that a reference is made to something undefined in the environment.
– `Cannot Access Before Initialization`: This signals the attempt to access a variable which hasn’t been initialized.
In terms of solving this issue, there are several methods depending on the situation:
1. The first solution would be reordering the code so that the variable initialization happens before it’s used.
2. Another potential fix can be changing the `let` or `const` declaration into a `var`. This will solve the problem if you want the variable to be function-scoped rather than block-scoped.
Note though, these solutions will greatly depend on your application requirements and architecture.
Understanding the nature of ES6 variables and their hoisting behaviors is crucial for effective utilization of Jest for Unit Testing. To delve deeper into these concepts, refer to the ECMAScript 6 Official documentation [link] and Jest’s documentation on using matchers [link].
Incorporating the wisdom of Douglas Crockford, considered by many as the godfather of JavaScript, “Programming is the act of construction, the large scale creation of function and functionality.”
Coding can be as much of an art as it is a science. Use these principles and your intuition to interpret error messages and improve code performance.
Digging Deeper into Scope and Hoisting In JavaScript Testing With Jest
Understanding the concepts of scope and hoisting in JavaScript is crucial for avoiding errors such as Jest `ReferenceError: Cannot access ‘variable’ before initialization`. This error occurs primarily due to hoisting – a unique behavior in JavaScript where variable declarations (not initializations) are moved to the top of their enclosing scope.
To start, we need to grasp what scope is in programming specifically for JavaScript. In a nutshell, scope determines the accessibility or visibility of variables, functions, and objects in some particular part of your code during runtime. In other words, it defines the portion of the code where a variable or a function can be accessed.
There are two types of scope in JavaScript:
- Global Scope: Variables declared outside of a block
- Local Scope: Variables declared inside a block or function
Next, let’s understand hoisting. This is a JavaScript mechanism where variables and function declarations are moved to the top of their containing scope before the code has been executed. Important thing to note here is that only the declarations are hoisted, not initializations.
When writing tests in Jest, scoping issues may arise from asynchronous testing patterns, especially when paired with the hoisting of variables. As an example, if you declare a variable within a test case and subsequently reference it in a beforeEach block, Jest will throw a `ReferenceError` because, due to hoisting, the `beforeEach` block is run before the variable declaration occurs.
Here is an example to illustrate this:
let myVar; beforeEach(() => { console.log(myVar); // ReferenceError: Cannot access 'myVar' before initialization }); test('A Test example', () => { myVar = 'Hello, Jest!'; });
In order to prevent these kinds of errors, adhere to the following recommendations:
- Declare and initialize variables at the beginning of your code or function.
- Be cognizant of where you are declaring variables within asynchronous test blocks.
- Use `let` and `const` which are block scoped and would help to minimize hoisting related issues.
As Bill Gates once remarked, “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency. The second is that automation applied to an inefficient operation will magnify the inefficiency.” So it’s essential to keep our JavaScript usage optimized and efficient by understanding and effectively using concepts such as scoping and hoisting.
Further details about scoping and hoisting can be found on this MDN Web Docs page.
Note: This is only one possible cause for the ReferenceError in Jest. If issues persist, additional debugging may be necessary to identify the root cause, as other factors (such as third-party library usage) could be causing the error.
Fixing the ‘Cannot Access Before Initialization’ Error In Your Jest Code
When using Jest, a common problem developers often encounter is the “Cannot Access Before Initialization” error. This widespread issue generally arises due to temporal dead zone constraints with JavaScript’s new block scoping constructs:
let
and
const
.
While developers familiarize themselves with Jest testing, an unwelcome “Jest Referenceerror Cannot Access Before Initialization” might occur regularly. This error primarily stems from the precise order in which JavaScript initializes variables and how Jest handles scopes.
Consider an analogy by Kyle Simpson, the author of ‘You Don’t Know JS’, to better grasp these challenging concepts: “JavaScript engines think about code much like Einstein thought about time: not as a single, continuous, left-to-right execution flow, but rather as a more complex, jumbled set of events that are influenced by temporal relationships.”
To mitigate the ‘Cannot Access Before Initialization’ error in Jest code efficiently:
– Revisit your declaration sequence: Ensure that you’re not accessing a variable prior to its initialization. In JavaScript, the hosting feature allows us to use a variable before its declaration only when declared using `var`. However, this is not the case for `let` and `const`.
html
Table: Difference between var, let, and const
Type | Hoisting | Block Scope |
---|---|---|
var |
Yes (initialized with undefined) | No |
let |
No (Temporal Dead Zone) | Yes |
const |
No (Temporal Dead Zone) | Yes |
– Avoid using `let` and `const` in global scope: Due to the Temporal Dead Zone, it leads to ReferenceError if you try using these before their declaration. Hence, for global variables, it’s best recommended to go with `var`.
– Set a variable’s value before using it in Jest mock function: It may seem trivial but can be a lifesaver. In Jest, remember to set a variable’s value before mocking a function with that variable.
– Follow correct order of operations: To prevent improper initializations leading to “Cannot Access Before Initialization” errors, carefully follow the order of test writing – setup, execute, verify, and teardown.
– Know the limitations: Jest testing is exceptionally efficient yet bound by JavaScript’s inherent limitations and constraints. Recognizing these boundaries allows us to write better, error-free code.
Threading away unsightly initialization errors, we tread towards mastering Jest testing. The work doesn’t end here; rather, this practice develops into greater erudition as we explore deeper, echoing the words of Edsger W. Dijkstra: “Perfecting oneself is as much unlearning as it is learning.”
References:
– Jest Documentation
– Exploring ES6 Variables
Working with Variables: Avoiding Reference Errors While Using Javascript’s Jest Framework
The failure of a test in Jest due to the error: “ReferenceError: Cannot access ‘variable’ before initialization” could be down to variable hoisting in JavaScript, strict temporal dead zone rules surrounding let and const, or issues associated with asynchronous nature of tests in Jest framework.
Understanding Variable Hoisting
JavaScript allows for Variable Hoisting, which means that any variable declared is considered to be declared at the top of its scope regardless of where the declaration was initially made. While hoisting can provide more flexibility, it can also lead to unintended reference errors if variables are used before they’ve been properly initialized.
Consider an example:
console.log(someVar); var someVar = 5;
In this code snippet, the variable `someVar` will be hoisted top of its scope, so technically the variable has been declared when console.log statement tries to log it. But at this point, JavaScript only knows about the existence of `someVar`, it doesn’t know about its assigned value, thus resulting into ‘undefined’.
Temporal Dead Zone and Reference Errors
let
and
const
declarations in JavaScript have something called a Temporal Dead Zone (TDZ). When a block-scoped variable is hoisted, it enters a TDZ from the block start to the declaration, during which any reference to the variable results in a reference error.
This effectively prevents variables from being accessed until their declarations are encountered while executing the script, and in turn helps reduce bugs by enforcing more predictable behavior in your code.
Asynchronicity in Jest
The Jest testing framework extensively uses asynchronous operations. In such settings, you may encounter reference errors if you attempt to access a variable before it’s been properly initialized.
Consider the following example:
let a; beforeEach(async () => { a = await SomeAsyncFunction(); }); test('example test', () => { console.log(a); });
If `SomeAsyncFunction` doesn’t resolve before the test runs, `a` could potentially still be `undefined`, causing a reference error. In these cases, you would need to ensure your asynchronous code has correctly resolved before executing dependent tests.
Therefore, to avoid ‘Cannot access Variable Before Initialization’ error in Jest framework:
– Understand and correctly handle JavaScript’s hoisting of variables.
– Keep the Temporal Dead Zone of `let` and `const` in mind to ensure variables aren’t accessed before declarations.
– Handle asynchronicity in Jest by ensuring that async operations have properly resolved before accessing variables they assign to.
Lastly, I remember this quote from Martin Fowler, a renowned software developer, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand”. This helps us remember the importance of writing clear and understandable code, hence avoiding such errors which can otherwise be avoided with proper knowledge of language semantics and syntaxes.
When dealing with Jest and encountering the
ReferenceError: Cannot access 'variable' before initialization
, it’s critical to understand the underlying cause of this issue, which typically pertains to JavaScript’s concept known as temporal dead zone (TDZ). This error essentially means that a variable is being called or utilized before its declaration, and in the realm of testing with Jest, such an occurrence can often lead to false results or failures in your test suites.
Solving Jest ReferenceError |
---|
One way to remedy this is by ensuring your variables are properly initialized before you attempt to reference them. This includes proof-checking for any asynchronous code where variables may not have been fully resolved at the time they’re being accessed. |
You can also revisit your code block scopes and make sure you’re not attempting to call a variable outside its scope. It’s key to remember that constants, let and class definitions in ES6 all have block-level scope. |
Lastly, taking advantage of Jest’s setup and teardown methods like
beforeEach() and afterEach() to initialize variables before tests run can be a good strategy to prevent such errors from occurring. |
Given that this error pertains directly to native JavaScript behavior, when encountered while using Jest, it’s paramount to reinforce the foundations of your JavaScript knowledge.
As stated by Addy Osmani, a well-known JavaScript developer, “Write code every day. Your coding skills will improve. Work on projects after work until it becomes second nature.”
With the aforementioned insights, developers can efficiently debug and find their way around the Jest
ReferenceError: Cannot access 'variable' before initialization
, ensuring a smoother unit testing experience, maximizing productivity, and ensuring high-quality code.