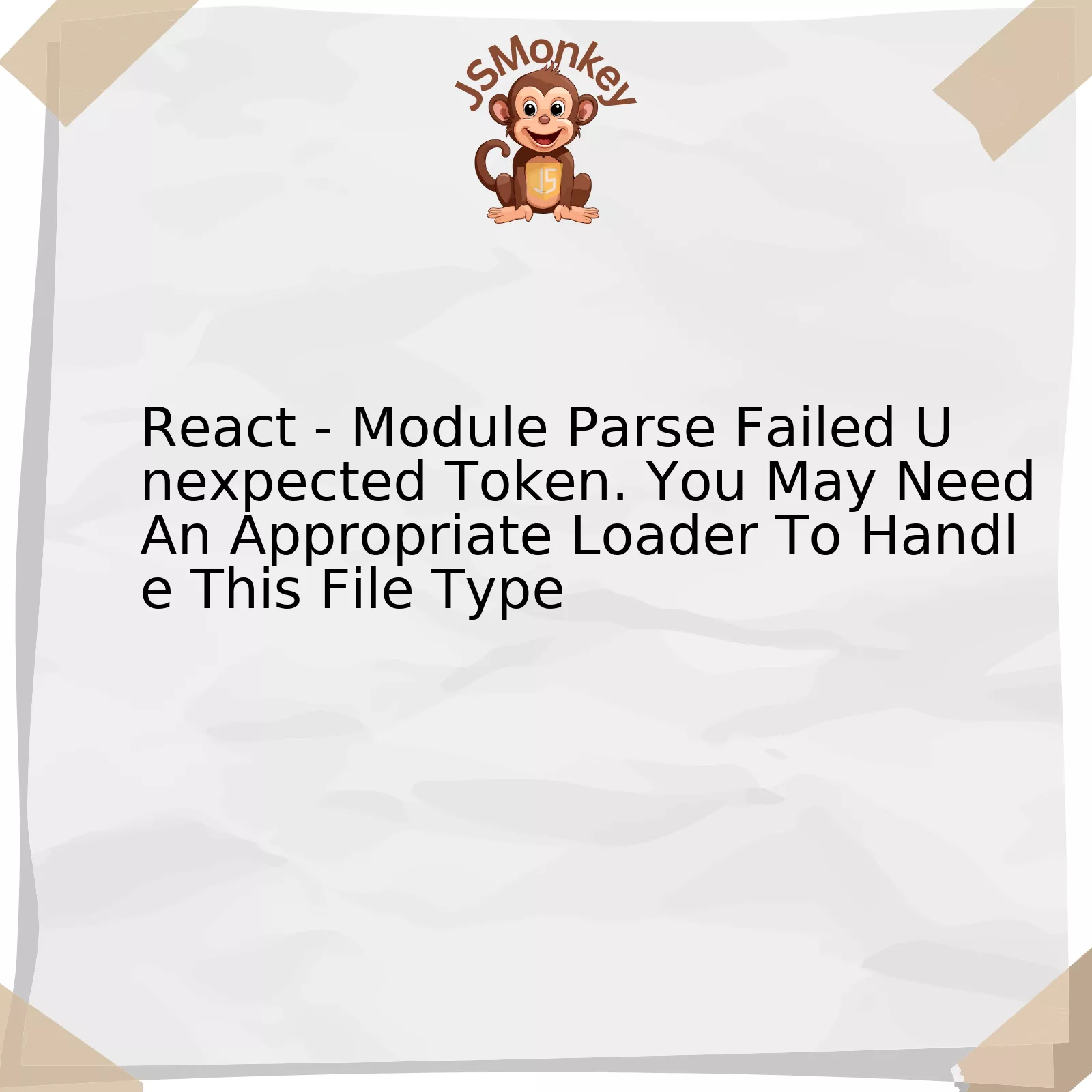
React, a popular JavaScript library for building user interfaces, is often subject to errors such as “Module Parse Failed: Unexpected Token.” This error typically arises when there is incorrect configuration of loaders in Webpack, a powerful tool that bundles JavaScript files for usage in a browser.
The issue primarily revolves around transpiling, or the process of converting source code from one language to another. In the context of React and Webpack, we are referring specifically to the conversion of modern JavaScript (ES6+) into ES5, the form of JavaScript most broadly compatible with browsers.
Error | Module parse failed: Unexpected token You may need an appropriate loader to handle this file type. |
---|---|
Root Cause | Inappropriate configuration of loaders in Webpack resulting in unsuccessful transpilation |
Solution | Proper configuration of Babel with Webpack to ensure successful transpilation of modern JavaScript into ES5 |
Babel is a widely employed transpiler which can convert modern JavaScript code into backward-compatible versions of JS code. It is essentially a compiler that transforms newer syntax into older syntax, while also allowing the usage of next generation JavaScript today.
To fix the ‘Module Parse Failed: Unexpected Token’ error, Babel must be configured correctly with Webpack. Webpack uses Babel through a ‘loader’, in this case babel-loader, which is responsible for loading the JavaScript files to be bundled.
By configuring babel-loader properly within the webpack.config.js file, you’re better positioned to curb the occurrence of this error:
module.exports = { module: { rules: [ { test: /\.(js|jsx)$/, exclude: /node_modules/, use: { loader: "babel-loader" } } ] } };
As Karl Popper, a renown philosopher once said, “We learn from our mistakes.” Similarly, encountering and troubleshooting these errors not only safeguards the robustness of your application but also enhances your competency as a JavaScript developer. While technology is ever-evolving, we constantly need to improve our understanding and knowledge to keep up with it. This error in the context of React serves as an inevitable example.
Understanding “React – Module Parse Failed Unexpected Token” Errors
Taking a closer examination at the error message “React – Module Parse Failed Unexpected Token. You May Need An Appropriate Loader To Handle This File Type”, it is clear that it emerges during the build process where your project code is being transformed and bundled by module bundlers like Webpack.
It is crucial to understand how the build process works to navigate such issues effectively. When you install react and configure webpack, there are several steps involved.
– First off, your JavaScript files go through transpiling process to convert them into browser-compatible JavaScript with the aid of Babel.
– Next, all the browser-compatible files get bundled together into one larger file with the help of Webpack to allow easy loading from the server.
Remember, the major purpose of using loaders in webpack configuration is to instruct your build tool on how to deal with different types of files. It could be stylesheets, fonts, images or JSX. Consequently, when Webpack encounters a ‘require()’ or ‘import’, it uses appropriate loader as per the configuration file.
Now, let’s relate this to your error: “Module parse failed unexpected token. You may need an appropriate loader to handle this file type.” This can be translated as : Webpack is encountering a piece of code or syntax it doesn’t recognize and hence, unable to conduct properly.
Here is a hypothetical situation:
You are adding an object spread operator {…obj} in a component without having babel-preset-react-app in your react project. The reason for this type of error to crop up is because the object spread (or rest) operator was added to JavaScript specification only after ES6 and thus should be complied correctly before being recognized by the browsers.
To solve this problem, check and verify if you have correct loaders installed and configured. For instance, to handle JSX syntax which is specific to React js, you might require Babel-Loader. Here is how you add a loader in your webpack config:
module: { rules: [ { test: /\.js$/, exclude: /(node_modules)/, use: { loader: 'babel-loader', options: { presets: ['@babel/preset-env', '@babel/react'] } } }, ] }
This instructs Webpack to use babel-loader for all JavaScript files. In simple words, it tells Webpack – “Hey, when you see a file with .js extension, use the Babel Loader to transform it into code browsers can understand.”
As Tom Dale, a software engineer rightly said, “If you think good architecture is expensive, try bad architecture.” Always ensure appropriate loaders are being used to avoid build issues and maintain well architected code.
Here’s more on using loaders with webpack. Here’s more on setting up Babel with React.
Key Reasons Behind Parsing Failure in React Modules
The error message “React – Module parse failed unexpected token. You may need an appropriate loader to handle this file type” is a common encounter in the React development environment. This issue could be a result of several factors, which we’ll dissect in depth:
– Incorrect File Type / Syntax Error: The phenomenon occurs mainly when you have an un-recognized or unsupported file type in your module. This could also mean that there’s a syntax error within your files where the Webpack fail to interpret or compile.
For instance, using import/export syntax in Node.js (which doesn’t support ES6 modules by default) can provoke such anomalies. Here’s how it typically looks when importing a component into your application:
import React from 'react'; import SomeCompnent from './SomeComponent';
– Incompatible or Missing Babel Loader Configuration: Babel helps in transpiling our JavaScript code from one standard to another (typically from ES6+ to ES5). In setting up Babel with Webpack, you include and configure Babel loaders. If Webpack encounters a non-JavaScript file or if these loaders are badly configured or missing, then “unexpected token” errors can occur.
To rectify this problem, ensure to install babel-loader and @babel/core via npm, and then correctly configure your webpack.config.js file to include test and exclude properties correctly. This prevents parsing node_modules and only interprets actual JavaScript files.
– Obsolete Dependencies: The versions of Babel or Webpack you’re using might be outdated relative to the other tools and libraries in your tech stack. It’s recommended to crosscheck and ensure all dependencies are up-to-date, functioning well together and compatible with each other.
– Absence of .babelrc file or Incorrect configuration: This configuration file is used to describe how the compiling/transpiling tool should operate. If it’s missing or not properly configured, Webpack and Babel might refuse to communicate properly, resulting in inability to parse through your JS files.
Bill Gates once said, “The most amazing thing about software is that it’s really all artificial. You can define your world.” Indeed, we play around with different technologies and tools like React, Webpack and Babel to create our applications. However, sometimes, things don‘t always align leading to challenges as seen above but with an understanding of what causes these issues and how to resolve them, we swiftly fix things to keep the wheel spinning.
For more information on this issue, find a detailed explanation here.
Effective Solutions for Handling File Types in React
React, one of the most popular JavaScript libraries in web development today, allows developers to build complex user interfaces effectively and efficiently. However, a common hurdle that developers may encounter when utilizing this library pertains to handling different file types. You could easily find yourself facing a module parse error stating “Unexpected token: You may need an appropriate loader to handle this file type.”
This error typically occurs when webpack, the static module bundler for modern JavaScript applications, can’t recognize a specific file type because it lacks an appropriate loader for parsing that type of file.
Resolving The Issue
To remedy this, you should update your webpack configurations with loaders suitable for the specific file types you’re attempting to utilize.
- Firstly, start by locating the configuration file
webpack.config.js
.
- Once you’ve located the config file, take a closer look at your module rules. Here, you’d define which extensions webpack is apt to handle.
Below is an example of how you can set the rules:
module.exports = { module: { rules: [ { test: /\.(js|jsx)$/, exclude: /node_modules/, loader: "babel-loader" }, { test: /\.css$/, use: ["style-loader", "css-loader"] } ], }, };
In this example, webpack has been configured to work with two loaders: ‘babel-loader’ and ‘css-loader’. These loaders respectively handle JavaScript and CSS files whereas excluding any from ‘node_modules’.
- The
babel-loader
transpiles JavaScript files using Babel and webpack.
- The
css-loader
interprets @import and url() like import/require() and will resolve them, and the
style-loader
injects CSS into the DOM.
This configuration helps webpack to handle JavaScript and CSS content elegantly without throwing a module parse error.
In addition, if you’re dealing with other unconventional file types (SVG, PNG, etc.), it would be necessary to add appropriate loaders:
module.exports = { module: { rules: [ { test: /\.(png|jpg|jpeg)$/, use: ['file-loader'] }, { test: /\.svg$/, use: ['svg-inline-loader']} ] }, };
With this configuration, webpack can now seamlessly process images and SVGs.
Discovering the appropriate loader to handle certain files stands crucial in enhancing your React development experience. While the task might initially seem daunting, learning how to accurately configure them could save considerable time and effort down the line.
Robert C. Martin, famously known as Uncle Bob, who is an American software engineer and author, once said, “A good programmer looks both ways before crossing a one-way street.” This wisdom applies perfectly to our discussion here, where ensuring you have the right tools (or loaders) for the job will ensure a smooth development process.
For further reading, visit official webpack documentation on loaders.
Enhancing Your Code: Appropriate Loaders to Resolve Unexpected Tokens in React
When encountering the error message “React – Module parse failed unexpected token. You may need an appropriate loader to handle this file type”, it often indicates that your Webpack configuration does not have the requisite loader to parse specific file types involved in your React application. In essence, Webpack needs ‘loaders’ to understand and transfigure certain file types into valid modules that can be added to the dependency graph.
Let’s dissect this error and propose solutions:
Why Do We Need Loaders?
On a foundational level, Webpack understands JavaScript and JSON files effortlessly. However, when it comes to other file types utilized in a modern day React project (like JSX, CSS, or even images), it needs extra sets of instructions to convert these files into usable modules.Webpack Docs
Here are some recommended loaders:
– Babel-loader: Used to transpile ES6 and JSX code into plain vanilla JavaScript that browsers can understand.
– Style-loader, CSS-loader: These work together for incorporating CSS styles into your JavaScript components.
– URL-loader, File-loader: Allow you to import files, such as images, into your code.
Solving the ‘Unexpected Token’ Error
Providing the right instruction to webpack using a loader might solve the issue. Below is an example of how you can set up a loader for Babel within the `module.rules` field of your Webpack config:
{ test: /\.(js|jsx)$/, exclude: /(node_modules|bower_components)/, use: { loader: "babel-loader", options: { presets: ["@babel/env"] } } }
To better appreciate the role each loader plays:
– The “test” property tells Webpack to apply the loader to any ‘js’ or ‘jsx’ files.
– The “exclude” property instructs Webpack to bypass certain directories.
– The “use” field indicates the loader to use, in this case, Babel.
As stated by Addy Osmani: “First do it, then do it right, then do it better.” While this error might seem intimidating at first, understanding the ‘why’ behind the application of these loaders will enable you to write more efficient code, and resolves such issues faster. Remember to focus on ‘doing it right’ and enhancing your application’s performance with the appropriate set of tools, including loaders.
The “React – Module Parse Failed Unexpected Token. You May Need An Appropriate Loader To Handle This File Type” error is a fairly common one that developers encounter when working with React JS. It typically arises when the Webpack compiler can’t interpret specific file types due to a missing or improper configuration of loaders. As compiled JavaScript and JSX are not natively understood by browsers, they need to be transcompiled into something which the browsers can interpret.
The term “loader” in this error essentially refers to the dependency or package like Babel loader, which parses and compiles your JavaScript or JSX files to browser-understandable code.
Consider an example: let’s say you’re trying to import a CSS file into your JavaScript module, but Webpack isn’t configured to handle CSS file types. The resulting error could look something like this:
Error in ./src/app.css Module parse failed: /{your_project_path}/src/app.css Unexpected token (1:0) You may need an appropriate loader to handle this file type.
A simple solution would be to install the style-loader and css-loader packages via npm and then update the Webpack’s config file accordingly:
{ test: /\.css$/, use: [ 'style-loader', 'css-loader' ] }
This essentially tells Webpack to use the defined loaders whenever it encounters a file with a .css extension.
Error messages such as “Module parse failed: Unexpected token. You may need an appropriate loader to handle this file type,” serve as important indicators that there may be a gap in your tooling configuration. Notably, these errors make developers more adept at configuring their development environment while also making them understand the intricate interconnectedness of various tools in modern web development.
An insightful quote from Mark Zuckerberg, the co-founder of Facebook (the company behind React), encapsulates this well: “Our philosophy is that we care about people first.”
This quote embodies the spirit of JavaScript developers worldwide, tirelessly debugging and resolving issues such as these to help build digital products, which are, at the end of the day, for people.
For starters in the field, a crucial resource that can provide assistance when a similar problem arises would be Babel Loader documentation. It contains information on how to setup Babel with your project and thus prevent or solve parsing problems within React.