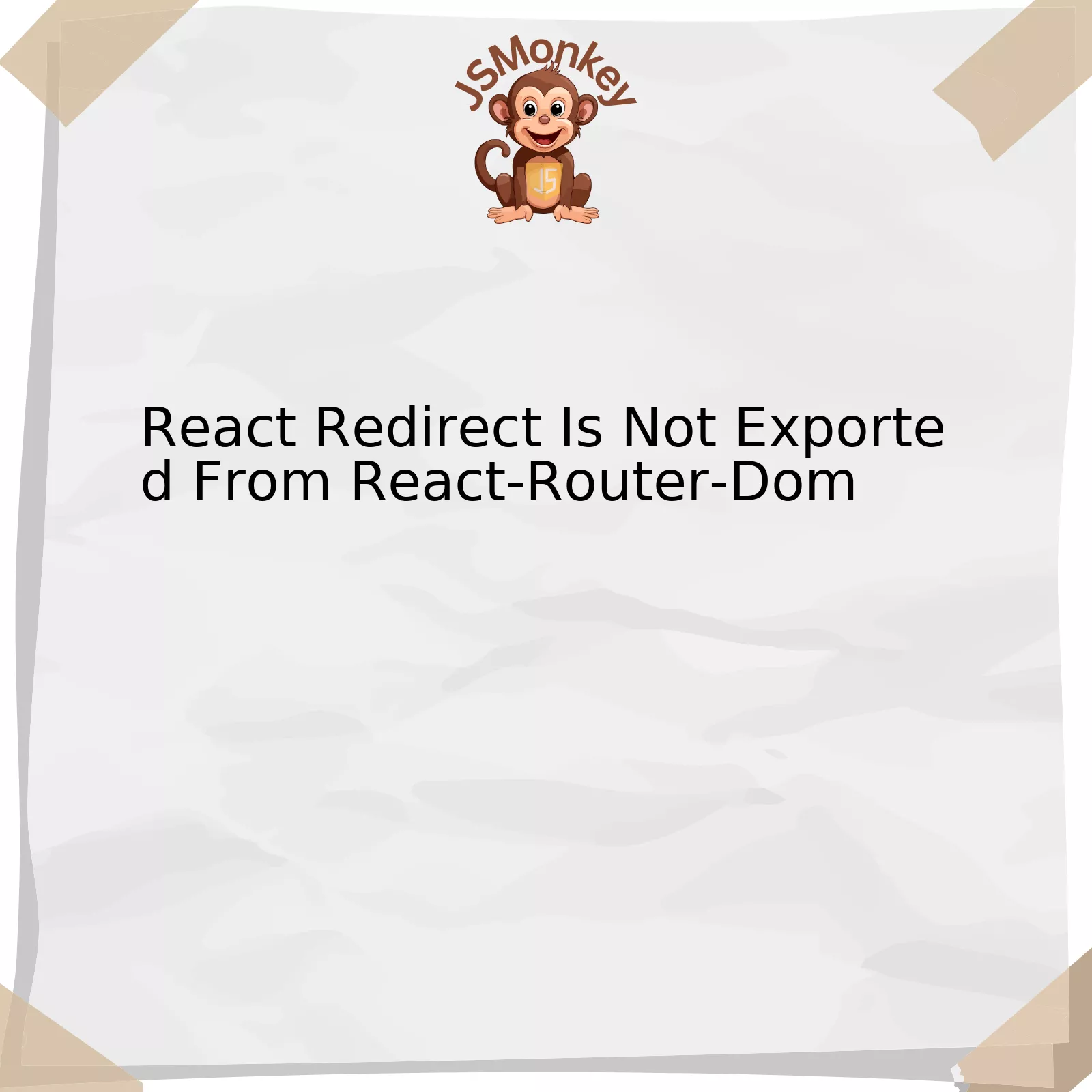
The obstacle you could be contending with is intricately connected to the alterations in the newer versions of `react-router-dom`. Specifically, these changes are firmly rooted from version 5 to version 6. The variant has seen some changes that could potentially clutch the traditional use. With that said, it’s important to grasp that `Redirect` is no longer a separate entity available for export directly from `react-router-dom`.
Take into consideration the following table:
Version | Exports |
---|---|
react-router-dom v5 |
Redirect |
react-router-dom v6 |
No Direct Redirect |
In essence, utilizing `Redirect` as formerly done in version 5 poses a problem due to the omission of distinct `Redirect` export in version 6.
Therefore, how might we address this dilemma?
Fundamentally, the solution resides within the entrails of the new alteration, we use the power of hooks; which in this specific case, would be to leverage `useNavigate`. It can be imported from ‘react-router-dom’ and is used to redirect to different routes.
Here’s an example:
import { useNavigate } from 'react-router-dom'; function Component() { let navigate = useNavigate(); return <button onClick={() => navigate('/path')}>Go to path</button>; }
Quoting Kyle Simpson, author of the You Don’t Know JS series: “Code is not just meant to be executed. Code is also a means of communication from a developer to both the machine and other developers.” This quote holds particularly true in this context. While the transition from the older `react-router-dom` version to the newer one changes how you write your code for redirection, it offers an enhanced method of communicating developers’ intentions clearly through its use of hooks.
This doesn’t mean `Redirect` has been entirely phased out. In fact, it still plays a role in the grand scheme of things as `useNavigate`, providing similar functionality but with a sense of distinctiveness that offers an improvement from what we’ve adored from version 5.
It’s important to stay updated with these changes, and also be patient with progressive transitions as frameworks continue to evolve, offering new ways to solve old problems. Always remember, coding is all about embracing change and evolution.
Understanding the Challenge: React Redirect Not Exported From ‘React-Router-Dom’
When working with React.js, a common issue that arises is the ‘React Redirect Not Exported from React-Router-Dom’ error message. This issue arises because the Redirect Module is no longer packaged with the primary distribution of the react-router-dom from version 5 onwards.
In effect, what this means for developers is:
- You can’t import the Redirect module using the old syntax:
import { Redirect } from 'react-router-dom'
.
- This unavailability could lead to confusion, especially if you’re accustomed to the older variants of react-router-dom.
The solution to this challenge is integrating the ‘useHistory’ Hook provided within the React-Router-Dom. In simple terms, you can substitute your current utility of the
Redirect
component with useHistory Hook to navigate programmatically.
Here’s how to do it:
First, import the useHistory hook at the start of your component:
import { useHistory } from "react-router-dom"
Then inside your function or class component create an instance of useHistory:
let history = useHistory();
Afterwards, use the push method on your history object to navigate to your desired path:
history.push('/new-path');
As John Von Neumann observed, progress in computer programming is made with the introduction of new and improved language features (like hooks in React). Indeed, these advancements tend to simplify code and enhance efficiency.
However, understanding these changes during migration from older versions could be tough. The key is knowing when the modules were introduced or deprecated. Aspiring to stay ahead with the most recent versions of frameworks and libraries would certainly help mitigate these sorts of issues in future. For example, the API and feature updates in newer versions are excellently documented on the official React Router website. This is a key resource for understanding shifts between different versions of libraries and frameworks.
Dealing with `React-Router-Dom` Post Redirection Issues
In order to understand the issue in question, “React Redirect is not exported from React-Router-Dom,” it’s important to dissect the components involved and why such an issue might arise. The primary reason for this error message could largely be due to the misinterpretation or misuse of certain functionalities within the React-Router-Dom library.
React-Router-Dom is a well-established, flexible, and efficient third-party routing solution in the universe of the React library. A common feature of this library is redirection – navigating users from one page or path to another dynamically.
`
The most striking change has been the replacement of `
Old way – Not Anymore Supported:
html
New way – Using useHistory hook:
html
const history = useHistory();
history.push(‘/new/path’);
This notorious error “React Redirect is not exported from React-Router-Dom” often originates when developers stick to deprecated or unsupported features. As seen above, `
Now, if you were using `
html
{isAuthenticated ?
With these intricate details regarding the navigation functionalities of `React-Router-Dom`, it becomes fundamentally easy to address the aforementioned error and substitute `
As Donald Knuth, a computer scientist and professor emeritus at Stanford University rightly put it – “The real problem is that programmers have spent far too much time worrying about efficiency in the wrong places and at the wrong times; Premature optimization is the root of all evil (or at least most of it) in programming.” This applies perfectly well here. Pivoting swiftly with library updates brings eminent benefits in maintaining efficient code and preventing unexpected bugs such as the “React Redirect is not exported” issue.
For additional insights into these changes, I would recommend visiting the official documentation on [React’s Github page](https://github.com/ReactTraining/react-router).
Exploring Alternatives to React Redirect from ‘React-Router-Dom’
When it comes to managing redirects in a React application, ‘react-router-dom’ is often the go-to library. However, starting with version 5.1, the dedicated ‘Redirect’ component has been deprecated and not exported from ‘react-router-dom’. This change might be confusing or even frustrating for developers used to the ‘Redirect’ component, but alternatives are available within the framework.
The primary option to execute a redirect now is the ‘useHistory()’ hook provided by ‘react-router-dom’. It provides the history instance that you may use to navigate.
Here’s an example of how we may use this:
html
import { useHistory } from ‘react-router-dom’;
function ExampleComponent() {
const history = useHistory();
function handleOnClick() {
history.push(‘/new-url’);
}
return (
);
}
In this code snippet, `useHistory` exposes the `history` object. A click event on the button triggers a method which invokes `history.push`, effectively redirecting the user to ‘/new-url’.
Another alternative to react’s `Redirect` is programmatically navigating using react router’s `` component as shown below:
html
import { Link } from ‘react-router-dom’;
function ExampleComponent() {
return (
Take me to new url
);
}
In this piece of code, we’re using the `` component provided by ‘react-router-dom’, which renders a navigation link to a new location.
As Bill Gates once said, “I choose a lazy person to do a hard job. Because a lazy person will find an easy way to do it.” What he meant was that we always need to find easier and simpler ways to achieve our goals. In coding, this can be translated to finding and using alternatives when the main option is no longer available.
To sum it up, even though the ‘Redirect’ component is no longer being exported from the newer ‘react-router-dom’ versions, thanks to the ‘useHistory()’ hook and other built-in features like the `` component going forward shouldn’t be a struggle.
Solving the Problem of React Redirect Being Unexportable from ‘React-Router-Dom’
The issue of ‘React Redirect’ not being exported from ‘react-router-dom’ is quite common among developers using React’s routing library. This problem resides in the shift between v4/v5 towards v6 where they have replaced redirect with useNavigate functionality.
Here are the key points you need to take into account while dealing with this situation:
1. Study Version Differences: Before we delve into the solutions, here is an essential fact about how things have evolved:
- React Router v4/v5 – Made use of
Redirect
component for redirections.
- React Router v6 – Replaced
Redirect
with
navigate()
function which belongs to
useNavigate
hook.
Therefore, if you come across an error like “‘redirect’ is not exported from ‘react-router-dom'”, it’s likely due to the difference between the versions you’re using and the version your code or tutorial was based on.
2. Adopting V6 Syntax: To resolve the confusion at its root, we must adapt our code to conform to the new syntax and methodology introduced with v6. The
Redirect
component does not exist anymore, but fear not. Instead of using
, now you will use the navigate function from useNavigate hook, like so:
let navigate = useNavigate();
navigate("/path");
Remember, timely updating your skills, knowledge and codebase according to the new versions is the best practice recommended by industry veterans.
3. Familiarizing New Contextual Routing: With React Router v6, a lot has changed including a stronger emphasis on relative routes and links, and ‘less magic’ context. The creators have served us something more powerful with a contextual routing mechanism. So, the practice from now on should be to understand and get comfortable with this new feature.
Take a look at the detailed guide [here](https://reactrouter.com/docs/en/v6/upgrading-5-to-6).
“As software developers, our job is to make informed decisions about the tools and libraries we choose to depend on.” – Kent C. Dodds. Becoming savvy on React Router means overcoming its learning curve, version differences being one of them, which will lead you to write more efficient and manageable code in your React applications.
In exploring the realm of ‘React Redirect not exported from React-router-dom’, it is essential to comprehend that this occurs primarily when the developer mistakenly utilizes obsolete versions of packages or imports the redirect component in an incorrect method.
The primary cause revolves around adjustments made in the version V5 and subsequently to version V6 of react-router-dom. Prior to version V5, the ‘Redirect’ was a part of the package but from version 5 onwards, it was removed. The removal has marked an important shift in how the react-router-dom functions. Developers should now use the ‘useNavigate’ hook for redirection instead of ‘Redirect’.
Let’s delve into the ideal solution for addressing the issue:
import { useNavigate } from "react-router-dom"; function Example() { const navigate = useNavigate(); return ( ); }
Presenting the ‘useNavigate’ hook available via ‘react-router-dom’, as shown above, eliminates the ‘React Redirect not exported’ problem extensively. Notice how ‘useNavigate’ replaces ‘Redirect’ to offer the same purpose.
As remarked by technologist Michael E. Gerber, learning is finding out what you already know, doing is demonstrating that you know it. Therefore, adapting to these changes, understanding the emerging tools, and staying updated make us better Javascript developers.
For more detailed information on how to utilize ‘useNavigate’, kindly visit the official React Router Documentation. An improved comprehension of these practices can vastly improve your user experience and proficiency in leveraging JavaScript’s powerful features within a web development context.