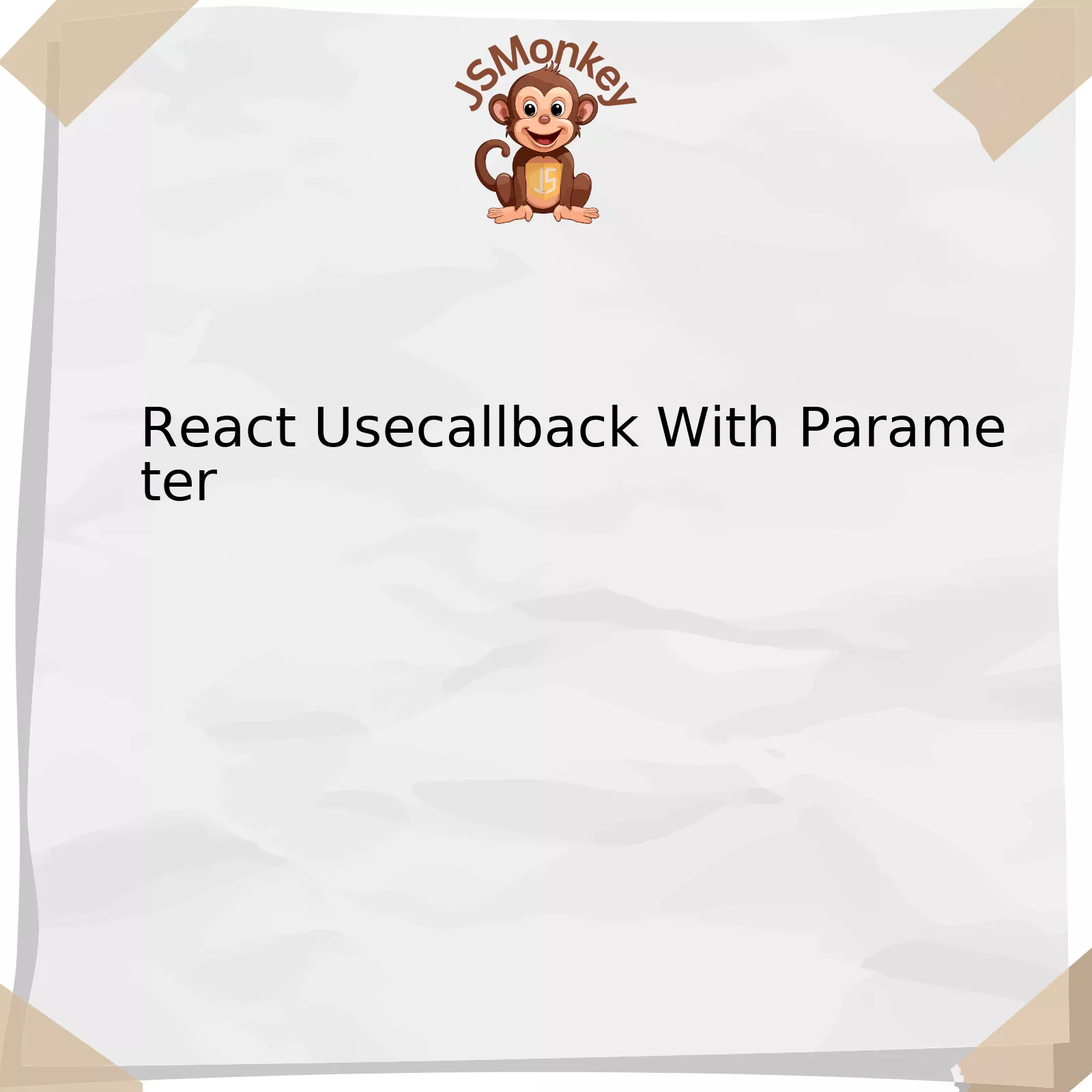
React’s `useCallback` is a frequently utilized hook in the development landscape. This hook is particularly useful for optimizing performance in React applications by preventing unnecessary re-renders.
Let us delve into an elaboration of `useCallback` with Parameters:
html
Hook | Description | Parameter |
---|---|---|
useCallback |
Preserves the identity of a function across render cycles | A function and a dependency array |
The `useCallback` hook returns a memoized version of a function that only changes if one of its dependencies has changed. It’s primarily used when passing callbacks to optimized child components that rely on reference equality to prevent unnecessary renders (Memo, useCallback, useMemo).
The parameters to `useCallback` are:
– The first parameter is a user-defined function.
– The second parameter is a dependency array.
Here’s a simple usage scenario. Suppose we have a parent component which renders an expensive-to-calculate Child component that takes a callback prop.
html
By wrapping our callback function inside `useCallback`, we’re stipulating that we only want the callback function to change (and thus trigger an expensive recalculation of the `Child` component) when the values of either `a` or `b` change.
When using `useCallback`, remember that it is not a guarantee of performance boost, but a tool to be used judiciously where necessary. Premature optimization can lead to difficult to read source code without significant gains.
As Donald Knuth, highly regarded computer scientist, once said; “Premature optimization is the root of all evil.”
A very important aspect to remember about `useCallback` (like other hooks) is that it should always be used at the top level of your React function. This ensures that it’s called in the same order during each re-render, adhering to React’s Hooks rules.
For more information or in-depth understanding, look into [
React' documentation
](https://reactjs.org/docs/hooks-reference.html#usecallback).
Understanding the Use of Parameters in React’s useCallback
Diving into the depths of React hooks, we come across React’s useCallback function. This hook proves extremely helpful when optimizing component performance in React applications. Below, we will focus specifically on understanding the usage of parameters within this hook.
Firstly, let’s examine what useCallback essentially does:
It returns a memoized version of the callback function that only changes if one of its specified dependencies has changed. This optimization technique is beneficial to prevent unnecessary re-renders.
The main syntax for `useCallback` is:
const memoizedCallBack = useCallback( () => { someFunction(a, b), }, [a, b], );
In this, the ‘a’ and ‘b’ are parameters or dependencies, which when altered, result in the hook triggering a rememoization of the function.
To keep it centered around ‘React useCallback With Parameter’, let us take an example:
const MyComponent = ({list, setList}) => { const addItemToList = useCallback( (newItem) => { setList([...list, newItem]); }, [setList] ); return ( //... ) }
In this code snippet, `newItem` acts as the parameter required by our useCallback functional component. Whenever `addItemToList` is invoked with a new item, previous list items are preserved due to the spread operator(one of JavaScript ES6’s features), and the new item gets appended, all while avoiding any redundant re-renderings.
Do note that since ‘list’ is not included as a dependency, whenever the ‘list’ changes, addItemToList doesn’t change – leading to potentially stale data.
As Sefika Ilkin Serbetcioglu stated[1]:
_”Understanding Hooks in React is essential to write clean, optimized and structured code.”_
Ultimately, achieving that optimal balance of useCallback’s dependencies is crucial. Using this hook effectively impacts overall application performance positively – a primary concern when developing scalable front-end applications.
– Never declare functions inside the body of components.
– Keep the function’s parameters completely independent from useCallback’s dependency array.
For more guidance regarding hooks and advanced topics around React, you can explore React’s official documentation.
[1] Serbetcioglu, Sefika Ilkin. “The Power of Hook: React.” Hacker Noon(2020).
Maximizing Efficient Rendering: A Deep Dive into React’s useCallback
Delving into your query on “Maximizing Efficient Rendering: A Deep Dive into React’s useCallback Keeping it Relevant to: React useCallBack With Parameter”, let’s step into the intricacies of React’s `useCallback` with parameters, which is an astounding feature of this JavaScript library that helps to enhance the efficiency of rendering.
React’s `useCallback` is a hook which is leveraged in order to return a memoized version of the callback function. This essentially means that this function will not change unless one or more of its dependencies have been altered. This is highly beneficial when it comes to preventing re-renders and thus improving the performance.
Let’s take a look at a typical usage of `useCallback` with a parameter:
jsx
import { useState, useCallback } from ‘react’;
function Example() {
const [count, setCount] = useState(0);
const increment = useCallback((incrementValue) => {
setCount(count + incrementValue);
}, [count]);
return (
{count}
);
}
export default Example;
In the code snippet above, `useCallback` is functioning to ensure that the `increment` function is consistently returning the same instance unless its dependency (`count`) changes.
This practice receives recognition due to the fact that it results in less re-rendering, meaning that the components that integrate the `increment` function are more efficient. According to David Walsh, respected JavaScript developer and Mozilla evangelist, “The key to achieving maximum efficient rendering with React lies in understanding the power of specific hooks such as `useCallback`. It’s all about accurately determining dependencies and using memoization effectively.”
However, be aware that `useCallback` doesn’t entirely abolish needless rerenders. The real source of such unnecessary renders is usually inadequate data flow or unoptimized state management within a React application. So, while `useCallback` can play an important role in reducing unnecessary rendering, it’s only one tool among many and should be used judiciously.
For a further deep dive into React’s `useCallback`, expert explanations on the memoization of callback functions, and several riveting examples, check out their official documentation.
Delving Into Parameter Passing with the useCallback Hook
The `useCallback` hook in React is no doubt a potent tool, widely used for performance optimization. One of the key features of this hook that often elicits great interest and still leaves some room for further exploration, is its inherent support for parameter passing.
The `useCallback` hook refers to a react hook which returns a memoized version of the callback function that only changes if one of the dependencies has changed. Its syntax can be succinctly represented as:
const memoizedCallback = useCallback( () => { doSomething(a, b); }, [a, b], );
In the preceding code block,
a
and
b
denote the parameters the useCallback hook depends on and will recompute whenever any of these parameters alter.
How then does `useCallback` handle parameter passing, and why is there always ardent curiosity regarding its execution?
The truth is simple; when a function is passed as a prop and this function is changed, it will cause the component to re-render – sabotaging the bid to achieve performance optimization. At times, it may also result into unnecessary renders due to the inappropriate management of dependencies within the callback function.
The `useCallback` hook bridges this gap by providing an optimal approach to parameter passing – It allows us to specify an array of values that the memoized value of the callback function depends on. You should know that the function wraps itself around any parameter we pass into it from within its closure.
By employing the `useCallback` hook properly, your application will eliminate unnecessary renders, reduce the time taken for each render cycle, perform at an improved speed, and offer a more desirable user experience, thereby allowing you to build highly efficient applications.
For example:
const memoizedCallback = useCallback( (param1, param2) => { return param1 + param2; }, [], );
In the above code snippet, `memoizedCallback` is a memoized function that gets new values when either `param1` or `param2` changes, therefore, keeping your component performance optimized.
Remember that “Good, better, best. Never let it rest. ‘Til your good is better and your better is best.” – [St. Jerome](https://www.brainyquote.com/quotes/st_jerome_209353), so always strive for optimization in your React applications using these advanced hooks like `useCallback`.
To better comprehend the inner workings of the `useCallback` hook with respect to parameter passing, you may want to [deep dive into the official react documentation](https://reactjs.org/docs/hooks-reference.html#usecallback).
An important note is not to indulge in over-optimizations as well, as mentioned by Donald Knuth, “Premature optimization is the root of all evil.” So, while useCallback can certainly be helpful in enhancing performance, it is crucial to use it judiciously.
Reactivity Enhanced: Practical Examples of React’s useCallback With Parameters
Understanding the useCallback Hook in React with parameters opens doors to creating efficient, reactive applications that minimize extraneous renderings and function creations. This is a critical aspect of application performance optimization, particularly in more extensive, more complex applications.
React’s
useCallback
hook returns a memoized version of a specified function that changes only if any dependencies change.1 If, for example, you have a component that receives a function as a child or uses a prop, each time the parent component renders, it will create a new instance of that function. However, by leveraging the
useCallback
hook, you can ensure that unnecessary re-renders don’t occur. Instead, only changes in dependency values instigate the recreation of these functions.
Let’s analyze an illustrative example:
html
const ParentComponent = () => {
const calculatedValue = expensiveCalculations();
const memoizedCallback = useCallback(() => {
return “Value is ” + calculatedValue;
}, [calculatedValue]);
return
};
In this snippet above, we instantiate
useCallback
with a function and a dependency array, which includes
calculatedValue
. The useCallback hook will return a memoized version of the function that only changes if
calculatedValue
does2.
Thereby, we are safeguarding against unnecessary computations and enhancing reactivity by ensuring rerenders and recalculations only when there are relevant value alterations among the dependencies.
One fascinating perspective on using hooks like
useCallback
comes from Sophie Alpert, a core engineer at Facebook who has contributed heavily to React. She stated: “Hooks let you always use functions instead of having to constantly switch between functions, classes, higher-order components, and render props.”3. It succinctly encapsulates the flexibility that comes with hooks and how they amplify our ability to create efficient code.
Harnessing the power of `React’s useCallback` with parameters propels an application’s performance to new heights. Why so? This is largely due to the fact that it limits unnecessary re-renders, leading to an efficient and smooth user interface while maintaining crystal clear code readability.
Let’s elucidate this by taking a simple scenario where we may need to pass parameters to `useCallback`. Consider you have a large list of items that users can select and each selection triggers a state update. Using `React’s useCallback` here would be highly beneficial as it would prevent needless re-rendering when the user selects an item on the list.
Examining from aspect of code, suppose `onChange` event handler is attached to each of these items like,
const handleClick = (id) => { setSelectedID(id); };
Now if we employ `useCallback`, we could do something similar like so,
const handleClick = useCallback((id) => { setSelectedID(id); }, []);
Understandably, the parameter ‘id’ isn’t in the dependency array but it doesn’t have to be. Simply put, `useCallback` will create a persistent version of the function that has access to latest variables from its scope. So the above statement reduces unnecessary re-renderings by avoiding creation of new functions at each render.
Eminent computer scientist Donald Knuth once declared, “Premature optimization is root cause of all evil”. This holds a lot of significance for React developers, who might incline towards optimizing every part of their application without justifiable need. While `React’s useCallback` with parameters undeniably gives a commendable performance boost, it’s advisable to incorporate this only when confronting issues like slow renders for complex UIs or jank.
To delve deeper into how `React’s useCallback` with parameters aids in improving performance, React’s official documentation can serve as an enriching resource.
Hence, making judicious use of `React’s useCallback` hook with parameters by understanding its nuanced behaviour can help developers yield a remarkable user experience while conservely utilizing computational resources. This is an exemplary manifestation of React’s distinctive and user-friendly approach to solving contemporary programming challenges.