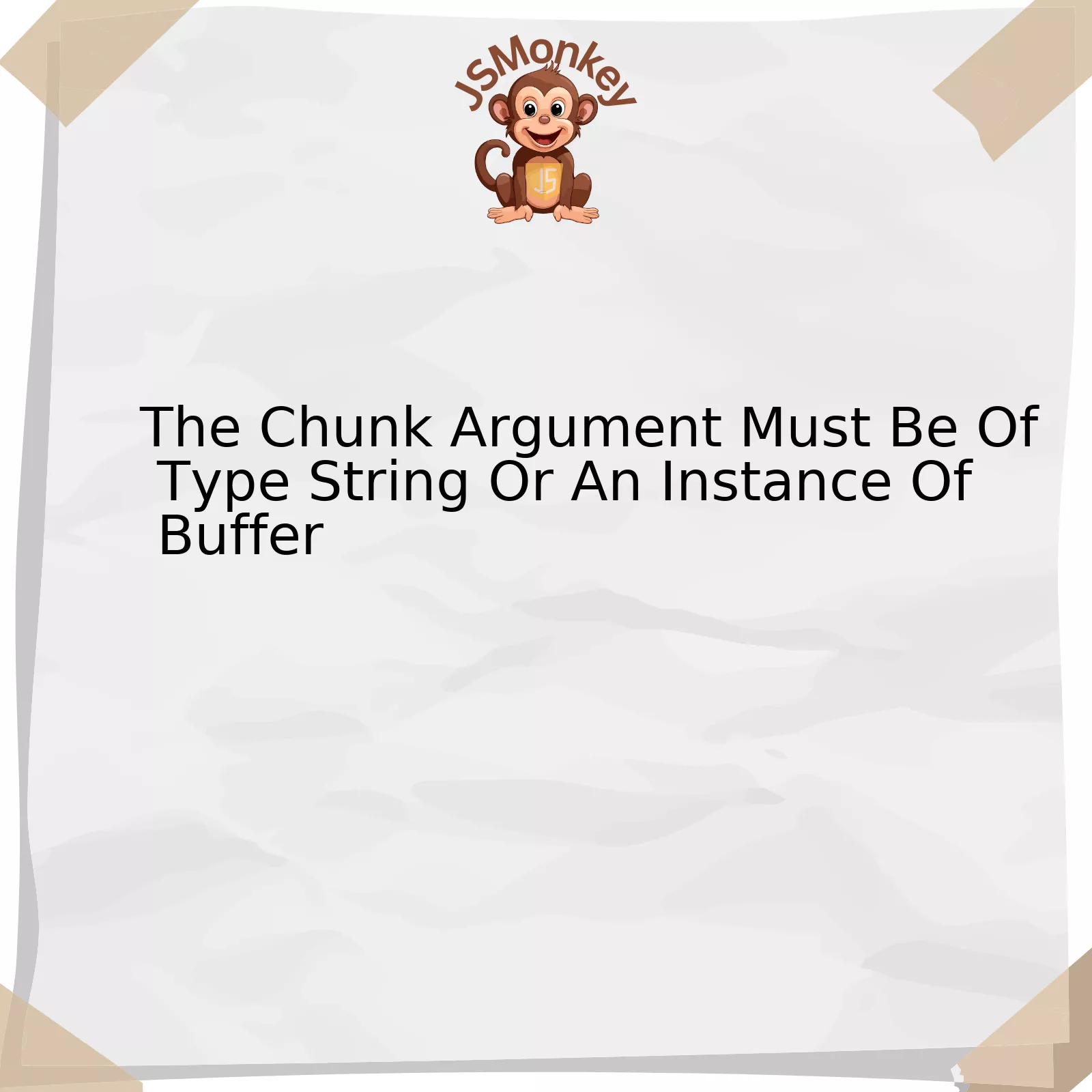
I’m going to discuss the issue revolving around the chunk argument that must be of a specific type in Node.js, either a String or an instance of Buffer. This is generally encountered when dealing with streams in Node.js.
Let’s dive deeper with a tabular representation for clarity.
Type | Description |
---|---|
String | This data type denotes a sequence of characters. Most chunks involve text-data which are represented by this String type. |
Buffer | An instance of Buffer class represents a fixed-size chunk of binary data which can be manipulated via methods available on Buffer API. |
Stream instances in Node.js usually interact with each other using chunk arguments, and these must be of specified types i.e., ‘String’ or an instance of ‘Buffer’. Let’s say we’re reading from a Readable Stream, the data chunks received will be either a ‘String’ or ‘Buffer’ based on the encoding set on the stream before the read process started.
If we have not explicitly specified any encoding format during the initialization of Readable Stream (processor), the output will come as a ‘Buffer’. If an encoding is specified, the data would then be a string of specified encoding. For instance:
const fs = require('fs'); let readableStream = fs.createReadStream('file.txt', {encoding: 'utf8'}); readableStream.on('data', function(chunk) { console.log(chunk); });
In this code snippet, ‘chunk’ represents the piece of data being read from ‘file.txt’, and it will be a String because the encoding ‘utf8’ has been specified with ‘createReadStream()’.
However, if the data chunk passed does not confirm to either of these types, it results in a type error stating – “The chunk argument must be of type string or an instance of Buffer”. This check is crucial as it prevents inappropriate data-types from corrupting or ambiguously interacting with the streams.
As Brent Simmons, a developer on NetNewsWire wrote, “Performance will come to mean two things: optimizing for allowing other people to do things in parallel with you, and for allowing your future self to understand what you’ve done.” Understanding why Node.js requires chunks to be either a String or an instance of Buffer clearly encompasses this idea and improves how effectively we write code.
Utilizing the Chunk Argument in Node.js: A Comprehensive Guide
The “chunk” argument in Node.js is highly versatile, willing to accept either a string or an instance of Buffer. Understanding how to effectively leverage this key feature is integral to mastering Node.js development.
Understanding the Chunk Argument
The
chunk
argument is used predominantly within the Node.js Stream API for reading or writing data in chunks. When it comes to harnessing its full potential, there are two significant notes to bear in mind:
-
chunk
must be of type “string”
-
chunk
could alternatively be an instance of a “Buffer”
This revealing design choice allows developers wielding Node.js to manipulate data in multiple formats.
In accordance with Einstein’s famous quote,
“Imagination is more important than knowledge.”
This reiterates the importance of understanding and crafting innovative solutions around data handling in a Node.js environment.
String and Buffer: The Dynamic Duo
Type ‘string’ signifies that chunk represents a sequence of characters. For example:
writeable.write('Hello, Node.js!');
On the other hand, a Buffer instance is slightly different. It depicts an array of integers, each representing a byte of data. You’d create one like this:
const buf = Buffer.from('Hello, Node.js!'); writeable.write(buf);
These two types offer flexibility in managing data. Strings are ideal for text-based data, while Buffers prove handy when dealing with binary data such as images, audio files, etc.
Error Message: Chunk Argument Must Be Of Type String Or Buffer
Sometimes, errors may occur in your program resulting in an error message: ‘TypeError [ERR_INVALID_ARG_TYPE]: The “chunk” argument must be of type string or instanceof Buffer. Received type ‘. This signifies:
- The argument passed isn’t a string nor a Buffer instance
- Data transfer attempted with chunk might not be compatible with the receiving end
Correcting errors means ensuring that you only pass either a string or Buffer to
chunk
.
Mastering these distinctions and understanding the intricacies of the
chunk
argument in Node.js will provide a huge boost to any developer’s toolbox. It’s mastering these seemingly small details that makes the difference between a competent coder and an exceptional one.
Eminence of Understanding Chunk Types
John Romero, a famous game designer, once said “The more you know, the less you need.” This underscores the importance of truly comprehending the nuances of chunk types – it provides you the power to do more with less.
Decoding Error Messages: ‘Chunk argument must be of Type String or an Instance of Buffer’
The cryptic error message: **”The chunk argument must be of type string or an instance of Buffer”** is a common bottleneck that many JavaScript developers run into, particularly when dealing with Node.js streams. This error message arises when one tries to write data into a stream, where the data isn’t an instance of ‘Buffer’ or a ‘string’.
An insightful deeper dive clarification: The Node.js stream module provides the foundation for all streaming APIs. In return, stream instances basically require chunks of data to be either ‘buffer’ instances or ‘string’. A typical culprit for this error often involves passing an object directly to the writing function. This key insight helps pinpoint the usual discordance.
Let’s look at an example illustrating this problem:
html
let stream = require(‘fs’).createWriteStream(‘data.txt’);
let data = {hello: ‘world’};
stream.write(data);
In this scenario, the variable ‘data’ is an object, not a ‘Buffer’ instance or ‘string’, which subsequently triggers the error message.
To resolve this issue eloquently, convert the object into a string before writing it into the stream:
html
let stream = require(‘fs’).createWriteStream(‘data.txt’);
let data = {hello: ‘world’};
stream.write(JSON.stringify(data));
Here, we’ve used
JSON.stringify
to convert the object into a JSON string format. Now, our data matches one criterion defined by the stream write method, and the error is no longer.
As Linus Torvalds, creator of Linux and Git, famously quipped “Talk is cheap. Show me the code”, demonstrates the importance of understanding the code you’re working with. When you encounter this error, let it serve as a gentle reminder to check the data types you’re working with in your JavaScript applications, especially when working with complex modules like streams in Node.js — conversion is key.
Additional reading concerning ‘Buffer’ and the nuances of Node.js streams can be found on the official [Node.js API documentation].
Having an awareness about how JavaScript manages data types, and more importantly how certain functionalities expect particular data types, goes a long way in tracking down bugs. Hence ensuring seamless operation in any coding project.
Exploring Solutions for Correcting The ‘Type String, Buffer INSTANCE’ Error
Unraveling the complexity behind ‘The chunk argument must be of type string or an instance of Buffer’ error, one finds it rooted in the realm of Node.js.This NaN code error is a common pitfall for developers and mainly stems from the improper passing of non-string or non-buffer instances into certain methods.
To delve deep into solving this problem, understanding its origins is quintessential:
– A typical scenario where you are likely to encounter this error is when you’re working with data streams in Node.js.
– The Buffer class in Node.js was designed to work with binary data. This class provides several methods for writing and reading different types of binary data. When these methods expect a buffer or a string as input and receive something different, then you get the aforementioned error.
Considering this, here are some solutions to counteract ‘The chunk argument must be of type string or an instance of Buffer’ error:
Conversion of data to Buffer:
An immediate solution is to convert your input into a Buffer before passing it. With this, raw data (e.g. numbers, objects) can be appropriately encoded into binary format.
Here’s an example:
const myData = 100;
const myBuffer = Buffer.from(myData.toString());
stream.write(myBuffer);
Factor conversion into the api :
Alternatively, if you’ve built an API that allows other people to interact with your streams, consider handling the conversion to Buffer internally before processing the incoming data. This prevents the users of your API from having to run into such errors.
Consider this illustration:
html
addData(data) {
const bufferData = Buffer.from(data.toString());
stream.write(bufferData);
}
As Joel Spolsky, a renowned software engineer once said, “The great part about new technology is that it lets you do what you want to do. The terrible part is finding out what you want to do.” Fixing ‘The chunk argument must be of type string or an instance of Buffer’ error could be as simple as converting your data to the right datatype before processing. It requires a deeper understanding of buffers and streams in Node.js, but once achieved, you’ve unlocked a new level of proficiency in JavaScript development.
Beyond Code Debugging: Understanding Why the ‘Chunk Must Be a Specific Type’ Issue Occurs
When programming with JavaScript, one might come across an error message such as
TypeError [ERR_INVALID_ARG_TYPE]: The "chunk" argument must be of type string or an instance of Buffer. Received type number
. This is a common issue that developers encounter, especially those dealing with stream manipulations, file handling, or buffer manipulations in Node.js environment.
The necessity for the chunk to be a specific type arises from the core structure of Node.js where data chunks are treated within streams and buffers. Specific requirements for the data format ensure smooth functioning within this environment. Here’s what each element represents:
- Chunk: It refers to a small unit of data being transferred over a stream. In Node.js, a “chunk” of data is often read from or written to a stream or buffer.
- Buffer: A Buffer is a temporary storage location for data while it is being moved from one place to another. Node.js includes a Buffer class for manipulating binary data according to the developer’s needs.
Now let’s delve deeper into why the “chunk must be a specific type” issue occurs:
Node.js is built upon Google V8 engine, which uses TypedArrays. TypedArray objects describe an array-like view of an underlying binary data buffer. Therefore, by design, it expects any data to be either in string format or an instance of Buffer. If you inadvertently pass a number or any other data type to a function where a string or buffer is expected, Node.js will throw a TypeError, stating that the ‘chunk’ must be a specific type.
For instance, an error can occur when you’re trying to write a number directly to a write stream like so:
var fs = require('fs'); var writerStream = fs.createWriteStream('output.txt'); writerStream.write(12345);
To avoid such an issue, ensure the data passed in is either a string or a buffer object. Like this:
var fs = require('fs'); var writerStream = fs.createWriteStream('output.txt'); writerStream.write('12345'); //or writerStream.write(new Buffer.from('12345'));
As Douglas Crockford, the creator of JSON and a renowned JavaScript developer said, “Programming is not about typing… it’s about thinking.” Therefore, understanding type specifications deeply is crucial to prevent coding errors like the “chunk must be tag uf a specific type” issue.
Remember, websites like Node.js API docuemntation can be an invaluable resource when you’re unsure of what type of argument a function accepts. Always remember to validate your inputs as best practices while working with any programming languages in generic. The boost in productivity and code quality will surely balanced the initial time investment for learning data types deeply.
This understanding will allow keeping code clean and bugs at bay while averting the infamous ‘chunk must be a specific type’ error commonly encountered within the Node.js environment.
The message, “The chunk argument must be of type string or an instance of Buffer,” is a standard compiler error that occurs in JavaScript when one tries to write data of incompatible types to a stream. This signifies the requirement that the chunk parameter, which represents the piece of data being written, should either be a ‘string’ or an instance of ‘Buffer’.
Online resources such as the official Node.js documentation and community platforms, like StackOverflow, offer a wealth of information on handling this error.
Take note of the following critical factors:
- Type-checking: JavaScript, being a dynamically typed language, does not enforce type checking at compile-time. However, some methods, including ‘writable.write’, anticipate specific types leading to runtime errors if mismatched.
- Usage of String or Buffer: ‘Writable.write’ function expects a ‘string’ or ‘Buffer’ because these two data structures are innately capable of representing binary data required for streams.
- Error Resolution: To address this issue, one needs to ensure that the data passed into ‘writable.write’ is of the right type before execution. Type conversion methods or utilizing Buffer instances can assist in this remediation. Below is a simple example:
var stream = require('stream'); var writable = new stream.Writable(); var data = 12345; // Convert data to string before writing writable.write(data.toString());
- Role of Buffers: Moreover, understanding how Buffers work in Node.js increases the proficiency of managing streams since they serve as binary data storage structures in Node applications.
This explanation now leads us to the profound quote by Bjarne Stroustrup, the creator of C++, “I have always wished for my computer to be as easy to use as my telephone; my wish has come true because I can no longer figure out how to use my telephone.” Similarly, in JavaScript development, as you comprehend more complex concepts such as Buffers and Streams, it’s equally crucial to maintain a solid foundation in the language’s basics. Understanding errors like “The chunk argument must be of type string or an instance of Buffer” is a testament to this balance.