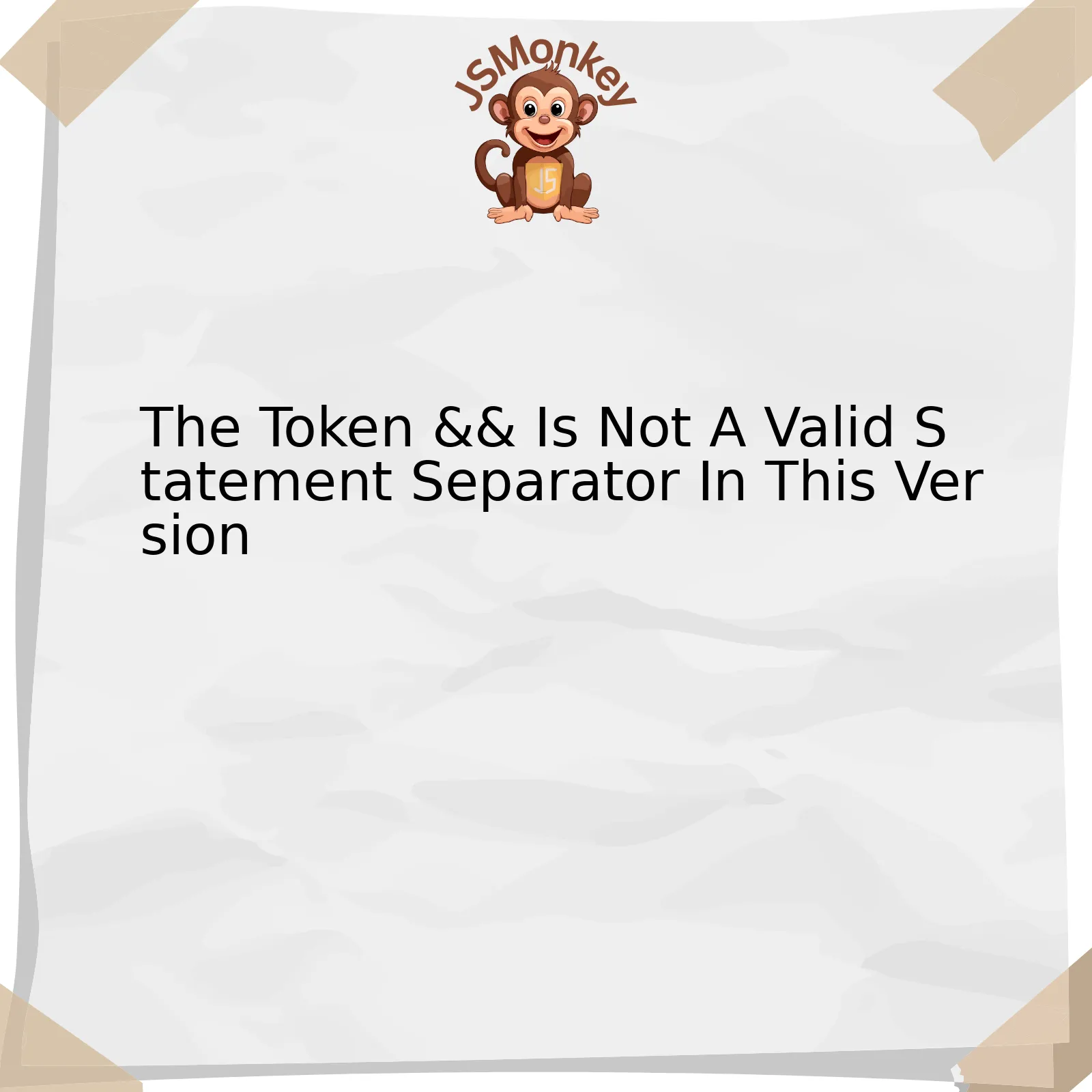
The token ‘&&’ is traditionally recognized as a logical operator in JavaScript, which allows the execution of two conditions. However, it isn’t viewed as a valid statement separator within numerous programming language versions.
Here’s an lucid table representation for an accurate understanding:
Symbol | Name | Usage | Role in JavaScript |
---|---|---|---|
&& | Logical AND Operator | Evaluating two conditions | Javascript uses ‘&&’ to evaluate two expressions and return a boolean value. |
Using the “&&” token as a statement separator could potentially cause errors in your program. Instead, you should consider using a semicolon “;” as a statement separator in most programming contexts. This difference can seem subtle but is crucial to maintain code correctness and readability.
An example of correct usage in JavaScript would be:
let x = 10; let y = 20; if (x > 5 && y > 10) { console.log('Both conditions are true'); }
This code checks to see if both conditions (“x > 5” and “y > 10”) are true – if they are, it prints out “Both conditions are true”. The ‘&&’ is working as a logical operator here, not a statement separator.
As Steve McConnell mentioned in his book, Code Complete: “Good code is its own best documentation.” It’s essential to break down our code into manageable chunks or statements, separated by semicolons, each performing a specific task.
When learning how to code, it’s good to remember that although certain symbols may look similar and even share some functionality across contexts (like “&&” being used as a logical operator), they can perform different functions based on the language and context in which they’re used.
Understanding The Role of Token && in Statement Separation
The conjunction operator “&&” is widely recognized in the realms of JavaScript. However, when associated with separating statements, misconceptions may occur because this operator does not serve as a valid statement separator traditionally.
To thoroughly comprehend the use and implementation of the “&&” operator inside the context of the JavaScript scripting language, one needs to understand its purpose precisely. In JavaScript, the “&&” operator performs a function called logical conjunction, which essentially means it determines if both sides of the expression are true. If so, and only then, the overall result is considered true.
Consider the following example:
var x = 10; var y = 20; if (x < 15 && y > 15) { console.log('Both conditions are true'); }
Here, “&&” signifies a logical AND operator. The statement within the “if” condition will only execute if both operands – x < 15 and y > 15 – return true. Therefore, the role of “&&” herein is to bind together two condition checking operations and not to separate them.
On using “&&” as a statement separator in JavaScript, there could be some confusion because it does not function as a conventional “statement separator” in JavaScript programming practices. In traditional syntax, different statements are separated by semicolons (;), not by the logical AND operator (&&).
However, we can often witness the ‘&&’ operator used as part of conditional (ternary) operations or within script elements in HTML where multiple JavaScript commands need to be executed based on certain conditions.
A common misconception arises from using && in an operation such as command-line scripts, batch files, or shell scripts where && is used to chain together commands, executing each command only if the previous one has succeeded. Nonetheless, taking this concept into JavaScript might lead to incorrect interpretations, as JavaScript does not consider && a true statement separator.
Bill Gates once said, “I choose a lazy person to do a hard job. Because a lazy person will find an easy way to do it.” And while this quote may seem out of context, it encompasses the spirit of coding and programmers’ constant endeavor to find shortcuts or simpler pathways. But, it’s essential to ensure that these simplified pathways, like using “&&” as a statement separator, are valid and don’t lead to complications in the code.
To maximize code readability and to fall in line with industry best practices, it’s crucial to use the established conventions when writing code. Therefore, “&&” should be used for its proper purpose – as a logical conjunction operator, and semicolon (;) should be employed for separating different JavaScript statements.[source]
Common Issues with Invalid Use of Token &&
The issue you’re confronting revolves around the “&&” symbol–commonly known as a logical AND operator in JavaScript. It’s intended to execute part of certain JavaScript code only if two conditions are satisfied simultaneously.
However, it seems you might have been attempting to use the “&&” operator as a statement separator, which is not allowed in the current version you are using and thus creates invalid token issues.
Concept of && Operator
The && operator is typically employed in conditional statements for assessing whether two conditions are both true. The code to the right of the ‘&&’ operator is only evaluated when the left side condition augments to true.
if (condition1 && condition2) { // Executes some code when both conditions are true }
Rationale Behind Invalid Usage Issue
Using “&&” as a statement separator, similar to “;” or “,”, is indeed synonymous with incorrect syntax, resulting in Non-Separator error messages. JavaScript doesn’t regard ‘&&’ as a command separator; instead, it’s primarily used within control structures.
Consider these correct example:
var result = (age > 18 && name == 'John') ? 'Access granted' : 'Access denied';
Solutions to Parse Error
To resolve this, one may need to consider the context of where various tokens are being utilised, ensuring command delimiting is correctly achieved with appropriate separators like “;” or “,”.
Reflections from Experts:
As sited by Douglas Crockford, a well-known JavaScript expert: “Programming JavaScript requires understanding how to structure the decision-making process so that program functionality aligns with the desired result”. In essence, one must recognize how and where to implement operators such as &&, avoiding misapplication that could lead to parsing errors.
Differentiation: Valid and Invalid Statement Separators
Understanding the nuances of JavaScript syntax is essential for efficient programming. Among these intricacies are statement separators, used in nearly all programming languages to separate sequences of statements. In JavaScript, two common statement separators are the semicolon (;) and comma (,). However, one should note that not all characters traditionally used as separators or delimiters in other languages necessarily perform the same function in JavaScript.
For instance, when it comes to the token &&, it is important to understand its role as a logical operator rather than a statement separator. While some developers may attempt to utilize && as a statement separator, it is not a valid substituent for the semicolon or comma within the context of this language.
Let’s look at an example:
let flag = true; let count = 10; if(flag && count > 5){ console.log('Condition met'); }
In the example above, ‘&&’ operates as a logical AND operator. This script checks if both ‘flag’ is true and ‘count’ is greater than 5. If both conditions are satisfied, ‘Condition met’ will be printed to the console.
Below is how statement separators like ‘;’ and ‘,’ are typically used in JavaScript:
let x = 25; // Semicolon separates the declaration from the next statement let y = 10, z = 30; // Comma separates multiple variable declarations
Contrary to this, using &&
as a replacement leads to misuse due to its functionality as a logical operator, not a statement separator.
As stated by Douglas Crockford, a JavaScript authority: “Programming isn’t about what you know; it’s about what you can figure out.”[^1^]. Auspiciously, understanding these syntactical constraints equips us with the analytical toolset required to write more robust and maintainable code.
[^1^]: (source)
Fixing Version Compatibility: Working Around the Token && Issue
Working around the token `&&` issue primarily involves understanding that in certain versions of JavaScript, this operator is not recognized as a valid statement separator. This can result in cryptic error messages and program crashes.
The common usage of `&&` is Logical AND where it can be used to exit out of execution if some condition is not met. But improper usage might lead to issues around version compatibility. Hence, to maintain the code efficiently and effectively, developers should consider alternatives that maintain the functionality without violating the constraints of their coding environment.
For example, one might use conditional (ternary) operators which resemble `if-else` statements. An analog statement to `condition && action();` is:
condition ? action() : null
Besides, a simple `if-else` construct can also be used to replace the `&&` token, while maintaining the original logical flow of your code. This can ensure the same functionality with improved clarity in older, non-compatible versions of JavaScript or different versions of browsers.
It’s important to understand that dealing with programming challenges isn’t just about finding a solution, but also about understanding why that problem occurred in the first place. So, when you’re dealing with version-compatibility issues, such as the ‘Token && Is Not A Valid Statement Separator In This Version’, make sure you understand the cause behind this error and act accordingly.
Understanding these nuances will not only help in effective debugging, but also in writing cleaner and more maintainable code. As the famous quote by Brian Kernighan goes, “Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.”
Further reading: [JavaScript Operators](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Expressions_and_Operators), [Conditional (Ternary) Operator](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Conditional_Operator), [if…else Statement](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/if…else)
Before proceeding to delineate this topic, it’s essential to understand the nature and use of the double ampersand (&&) in JavaScript. This token is primarily used as a logical AND operator that returns true if both operands are true. However, it cannot function as a valid statement separator like semicolon (;).
Diving into the JavaScript ecosystem, programmers often encounter different signs or symbolisms that represent distinct functions within the language. The understanding of these symbols contributes to creating more refined and error-free code. But at times, these functions may be misinterpreted, causing confusion and unexpected errors, such as using the && token incorrectly.
The interpretation of ‘&&’ as a statement separator can be a common mistake among developers new to JavaScript or those coming from a different programming language background. While a semicolon (;) or newline naturally separates statements in JavaScript, mistakenly utilizing ‘&&’ as a separator can lead to runtime errors, as the JavaScript interpreter does not recognize ‘&&’ as signifying the end of one statement and the start of another.
Rather, in JavaScript context, ‘&&’ serves as a logical operator predominantly used for executing conditional operations. JavaScript applies short-circuit evaluation using the following two principles: truthy and falsy values, optimizing its execution to improve performance. If the first operand is falsy, JavaScript won’t check the second operand due to its inherent “short-circuiting” behavior.
The erroneous perception regarding ‘&&’ as a statement separator might arise from confounding the syntax with some shell scripting languages where ‘&&’ is indeed utilized to chain commands together.
As identified, misunderstanding JavaScript tokens can result in programming errors or inefficient code practices. It emphasizes the importance of appropriate and continuous learning, especially considering the ever-evolving panorama of web development technologies.
To further delve into the intricate details of understanding JavaScript operators, consult online documentation and resources like MDN Web Docs. Furthermore, adopting best coding practices, including write-up efficient JavaScript code, is fundamental to mitigating these types of errors.
As Rita Mae Brown famously quoted, “Good judgment comes from experience, and experience comes from bad judgment.” It’s an essential lesson in programming: making errors, understanding them, and improving upon them leads to successful coding experiences in the future.