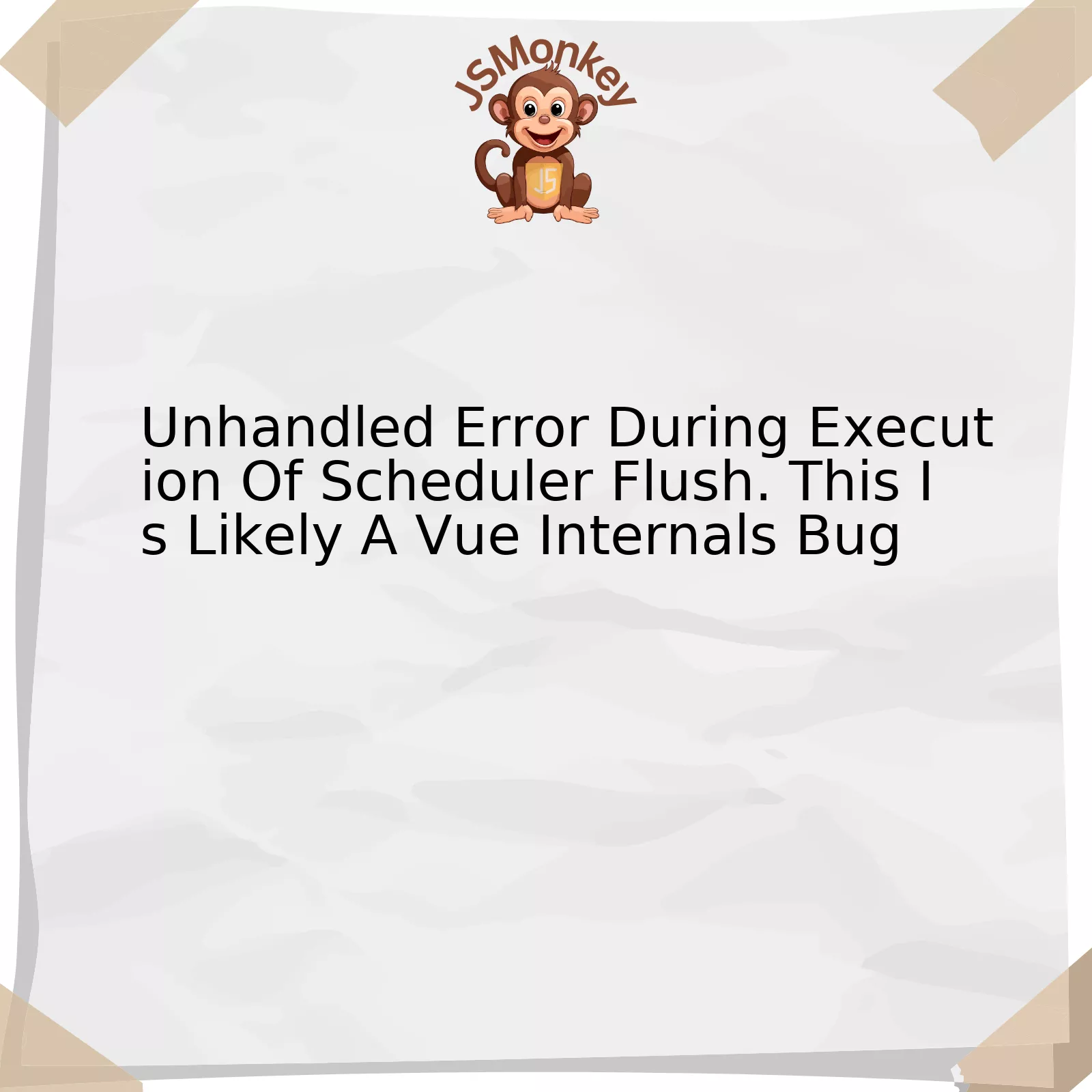
Errors | Potential Causes | Solution Path |
---|---|---|
“Unhandled Error During Execution Of Scheduler Flush” | Vue internals error, occurring from Vue.js platform | Debugging, upgrading Vue.js to the latest stable version |
“Unhandled Error During Execution Of Scheduler Flush” is an error commonly encountered in Vue.js. It products a notification that there’s an uncaught or unmanaged error during the execution of scheduler flush. Developers understand this as an unexpected issue that occurred within the Vue internals which is likely due to a bug with the framework itself. When these errors appear, the exact cause can be varied and hence proper debugging is crucial.
Here are some tactics employed by developers:
1) Thorough Debugging: Assessing the console log for any additional error messages or indicators that might hint at what could be causing the disposition.
2) Isolate Problem Components: Recognizing if the error message happens globally across all components or it’s specific to only a few of them may help isolate the problematic code.
3) Updating Vue.js: Frequently, resolving such errors involves updating Vue.js to the latest stable release. This ensures any known internal bugs have already been addressed and fixed by the Vue.js team.
4) Checking dependencies: This includes examining plugins used in the project. Sometimes, incompatible versions between Vue.js and its plugins generate unpredictable internal errors.
5) Seeking help: If nothing above helps, seeking assistance on platforms like StackOverflow, or reporting the issue on the official Vue.js GitHub repository can be helpful.
A relevant quote here is one from Brian Kernighan: “Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.”
This statement stresses the importance of writing maintainable and readable code while developing, which will eventually ease the debugging process. Given that Vue.js prides itself on its simplicity and ease of use, encountering an “Unhandled Error During Execution Of Scheduler Flush” brings up the challenge to constructively embrace debugging as advised by Kernighan.
const app = new Vue({ el: '#app', data: { message: 'Hello Vue!' } })
This sample code represents how simple Vue.js intends for creating applications to be. Yet under certain circumstances, complexities might still arise leading to errors such as “Unhandled error during execution of scheduler flush,” reminding developers that debugging is equally as important as coding, even in simpler frameworks such as Vue.js.
You may find a comprehensive guide on handling errors and debugging in Vue from the official Vue.js guide.
Diagnosing the Scheduler Flush Error in Vue
The error message of “Unhandled Error During Execution Of Scheduler Flush. This Is Likely A Vue Internals Bug” explicitly implies that you have run into a problem during the rendering stage of a Vue instance, wherein the system has been unable to suitably process updates to the Vue components.
Fundamentally, Vue.js operates on a component-based architecture. Each component in this ecosystem is handled by a scheduler. This ‘scheduler’ queues and manages the rendering of these components to ensure efficient rendering.
Understanding the Problem
An ‘Unhandled Error During Execution Of Scheduler Flush’ often means there’s a discrepancy occurring inside the lifecycle methods such as
mounted
,
beforeUpdate
, or
updated
. If an exception occurs during these function executions, but is not caught adequately, it leads to this error.
Possible Causes & Debugging
Finding the root cause of this issue involves debugging your code thoroughly, focusing particularly on:
Vuex Store: | If you are using Vuex for state management check all your mutations or actions. |
---|---|
External Packages: | They may be out-of-date or have unreported bugs. Cross-check the version compatibility with Vue and update them if necessary. |
Promises: | Unresolved Promises may also lead to this error. Ensure they’re resolved successfully. |
Bear in mind that the goal is to find any operation that suspends and resumes asynchronously.
Alan Turing once said, “We can only see a short distance ahead, but we can see plenty there that needs to be done.” Hence, why we need to patiently comb through the web of code, understanding and rectifying any potential pitfalls.
Solutions
1. Add a
.catch
clause: Make sure that all asynchronous tasks have a
.catch
block to handle any exceptions and incapacitate their propagation up to the global level.
Example:
axios.get(url) .then((response) => this.data = response.data) .catch((error) => console.error('Axios error', error));
2. Use Vue.config.errorHandler: For all uncaught errors in lifecycle methods, use
Vue.config.errorHandler
.
Example:
Vue.config.errorHandler = (err, vm, info) => console.log(`Error: ${err.toString()}\\nInfo: ${info}`);
3. Finally, it’s plausible that the bug is not visible to vue-loader in Single File Components. Switching to runtime-only mode can elucidate such hidden issues.
As an enduring solution, consider keeping up with Vue updates and continually testing apps across different environments before deployment.
Reference:
Vue JS Error Handling
Decoding ‘Unhandled Error During Execution of Scheduler Flush’
When working with Vue, the error message “Unhandled Error During Execution of Scheduler Flush” can surface occasionally. It often hints at an internal bug within Vue’s event scheduler. Specifically, this issue emerges when there’s a discrepancy in the execution of scheduled tasks or updates in a Vue application.
To analyze and decode this error, let’s focus on Vue’s core mechanisms and how its event scheduler contributes to this problem:
• **Vue’s Reactivity System:** Vue employs a reactivity system to observe and react to data changes. When any registered dependency modifies, Vue strategically updates only those sections of the DOM which rely on that particular data.
• **Scheduler Role:** The Vue scheduler holds the primary role in managing these updates. It queues them and ensures they execute efficiently without redundant renders or wasteful efforts.
• **Errors During Scheduling:** ‘Unhandled Error During Execution of Scheduler Flush’ arises due to unforeseen issues during the scheduling process. These problems could include the inability to monitor dependencies, disruptions during task queuing, or failures in executing updates.
If you come across such obstacles, here are some troubleshooting steps:
1. Update Vue: Check if your Vue and its associated libraries (Vuex, Vue-router, etc.) versions are the latest. If they are not, consider updating them as many bugs usually get fixed with every new release.
2. Clean Reinstallation: Try reinstalling all the npm packages, as sometimes certain package versions may encounter compatibility issues.
npm uninstallnpm install
3. Debug your code: Run your app in a development environment and use Vue Devtools extension. This tool provides real-time insight into your Vue application and facilitates in-depth debugging.
As Bill Gates remarkably mentioned, “The computer was born to solve problems that did not exist before” [1](https://www.brainyquote.com/quotes/bill_gates_383863). Issues like ‘Unhandled Error During Execution Of Scheduler Flush’ can seem daunting at first. Yet, as you dive deeper into the framework’s internals, you not only solve the problem but also gain a more profound understanding of Vue’s functioning.
Understanding the Use Case Scenarios: The Vue Internals Bug
The message “Unhandled error during execution of scheduler flush. This is likely a Vue internals bug” can appear when working with Vue.js, which typically signifies an issue within the core library’s mechanisms. Let’s delve into understanding the Vue internals bug by discussing the use-case scenarios related to this specific error.
In Vue.js, the implementation of the Virtual DOM and the reactivity system are intricate parts of the internals. The “scheduler flush” process is also one part of this. Notably, when data changes in a Vue application, the scheduler will perform tasks like:
– Updating the component.
– Running lifecycle hooks.
– Triggering the reactive dependencies.
When an unforeseen or unhandled error happens during this sequence (the “scheduler flush”), this results in the error message – “Unhandled error during execution of scheduler flush. This is likely a Vue internals bug”.
Optically, these errors usually appear due to:
– Exceptions inside lifecycle hooks: If there’s an error or exception inside a lifecycle hook managed by Vue’s scheduler, it might lead to this error.
– Non-compliant handling of asynchronous operations: JavaScript relies heavily on asynchronous processing. If your Vue components have problematic async operations, then this error might surface.
– Incorrect handling of computed properties: Computed properties within Vue are automatically cached based on its dependencies. A bug here might trigger the aforementioned error.
To debug such errors:
1.
console.trace()
: You might want to place this in your lifecycle hooks or watchers to see where the execution stops because of the error.
2. Propagate errors in Promises: Make sure to propagate errors in Promises using
.catch()
or try-catch blocks with async-await syntax.
3. Use Vue.config.errorHandler: This lets you take control over Vue’s built-in error handling mechanism at a global scale.
When Vue.js throws “Unhandled error during execution of scheduler flush. This is likely a Vue internals bug”, it actually means there’s something going wrong in your own code. It’s a sort of misphrasing which indicates that the problem is ‘likely’ inside Vue, when in reality it’s with the way Vue is being utilized.
Remember the quote from Edsger W. Dijkstra: “Program testing can be used to show the presence of bugs, but never to show their absence”. It highlights how vital it is for developers to understand the internal workings of the tools they use each day and ensure they are implementing them correctly.
You can refer to Vue.js official documentation here for more details.
Effective Strategies to Resolve and Prevent Vue Internal Bugs
Vue.js, although one of the top JavaScript frameworks famed for its simplicity, flexibility, and efficiency can sometimes come with its own set of challenges, just like any other software. One such challenge can be the unhandled error during the execution of a scheduler flush, which is commonly an indication of a bug in Vue internals.
Understanding This Error
This error usually points to an issue within the Vue.js application that you’re developing rather than the Vue.js library itself. It may happen due to different reasons which include but are not limited to improper data handling, running asynchronous operations without proper error handling or a component lifecycle misuse. However, uncovering and fixing the exact cause may take more systematic debugging methods.
– “Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.” – Brian Kernighan
Solution Strategies
- Adequate Input Validation: Always ensure that your application validates all inputs before they are processed. Improper input validation can result in various unexpected scenarios leading to bugs.
- Error Handling: When dealing with asynchronous operations – use catch blocks, fundamentals of Promise based functions – to handle errors properly. This could help prevent undefined behaviors and give more insight on where things might go wrong.
- Use Well-Tested Libraries: Libraries that are tested thoroughly and maintained up-to-date significantly reduce the risk of runtime errors. Unmaintained libraries can clash with new JavaScript standards or Vue internals, which may lead to unexpected bugs.
<template> <input v-model="name"> </template> <script> export default { data() { return { name: '' } }, watch: { name(newName) { this.name = newName.trim(); } } } </script>
fetchData().then(response => { this.data = response; }).catch(error => { console.log('An error occurred:', error); });
Prevention Strategies
- Regular Update: It’s key to keep your Vue.js version updated. The Vue community frequently launches updates with bug fixes, as well as new feature enhancements, thus mitigating likelihoods of encountering internal bugs.
- Effective Testing: Implementing regular unit tests and end-end-tests using tools like Jest and Cypress help catch issues at earlier stages, preventing the occurrence of such errors in production.
// Using Jest for a simple unit test test('adds 1 + 2 to equal 3', () => { expect(sum(1, 2)).toBe(3); });
- Detailed Logging: Detailed logging provides valuable feedback when debugging. Consider using a logging package that allows different logging levels with contextual metadata collection, providing insightful information when an error occurs.
Reference: Official Vue.js Documentation
Observing the “Unhandled Error During Execution Of Scheduler Flush. This Is Likely A Vue Internals Bug,” it is essential to delve into its anatomy, resolution strategies, and precautions.
Anatomy of the issue
This occurrence in Vue.js involves an unhandled error during the execution of scheduler flush, which at a glance, suggests an internal deficiency within the Vue framework’s infrastructure. Mistakes can occur when synchronizing asynchronous tasks tied to updating the DOM, causing inefficiencies and potentially game-stopping errors.
Strategies for Resolution
To begin with, ensure that your development toolkit is updated comprehensively– both Vue.js versions along with all dependant libraries. These updates often incorporate bug fixes which may resolve this issue.
Furthermore, managing the project’s dependencies astutely becomes crucial. It may be expedient to isolate and test separate modules or components of your code. Using unit testing methods with tools like Jest or Mocha can streamline this process and help eliminate the error source.
Lastly, the Vue community support system and forums are benefit-rich resources where developers share their experiences and solutions related to similar issues. Exploring these platforms can provide useful insights.
Precautions and Best Practices
Being conscientious about coding practices helps reduce the probability of such issues reoccurring. Here are some valuable recommendations:
– Ensure callbacks completion: Asynchronous tasks could remain unresolved, leading to situations where Vue struggles to sync operations and causes errors.
– Judicious use of timers: Flameout conditions can occur if component flushing overlaps with timer-based operations.
– Catch and Handle Errors: Using mechanisms like
try/catch
blocks allows you to pragmatically handle detected exceptions.
As Dijkstra once said, “Testing shows the presence, not the absence of bugs”. Going forward, always remember that no software is ever entirely devoid of bugs. Developing an understanding of how to navigate and rectify such challenges will continuously improve your developer skills, reducing the likelihood of encountering an “Unhandled Error During Execution Of Scheduler Flush” within Vue.js. Error Handling in Vue.js