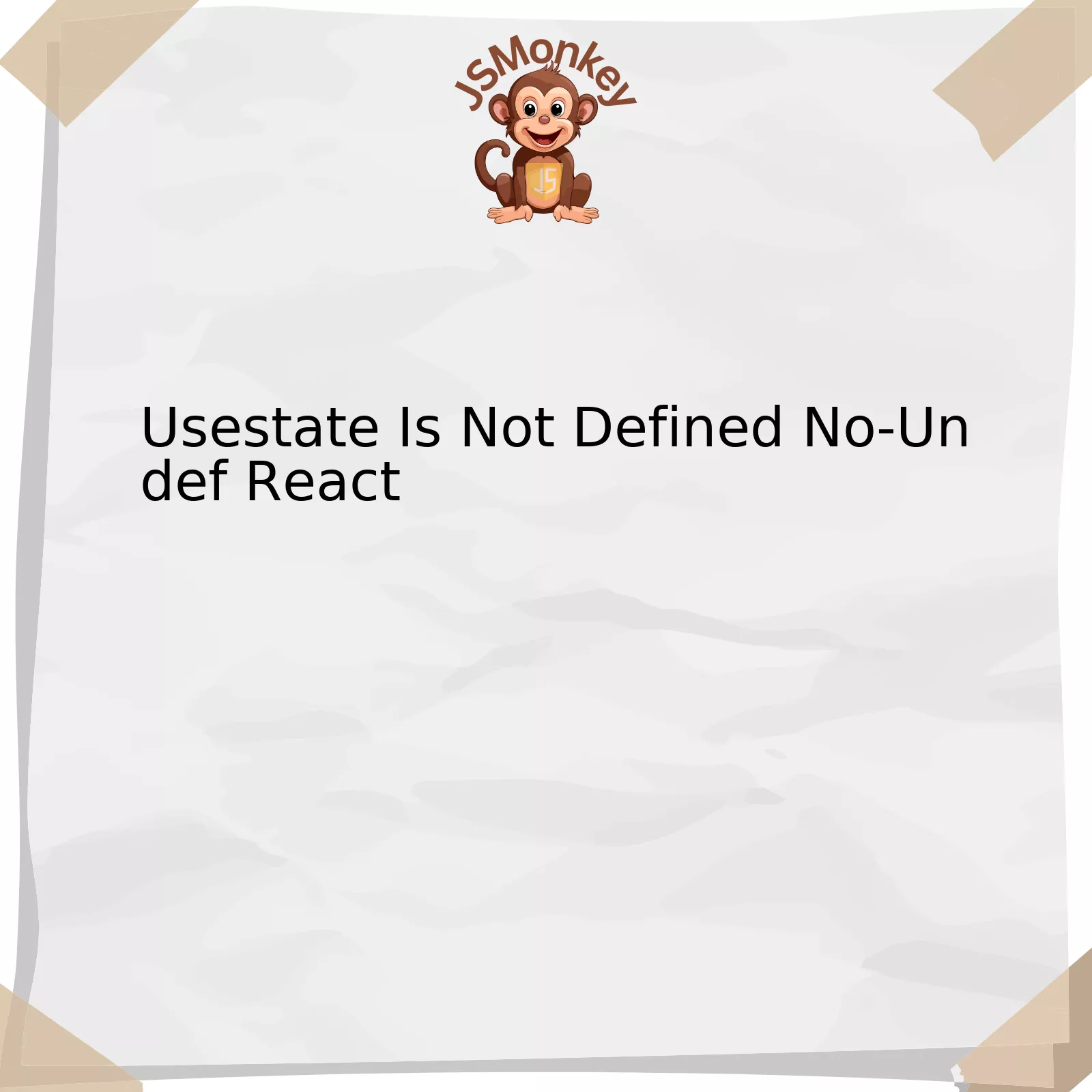
First off, the `useState` is not defined no-undef error in React can occur when you’re attempting to utilize the useState React Hook without the necessary import statement. This error simply means that the code can’t find the definition of `useState` which is crucial for managing and manipulating state data within functional components.
Let’s break down the typical reasons this error can happen:
Issue | Description |
---|---|
`useState` function was not imported |
This issue occurs when your program doesn’t have a line importing the useState function from React library. |
Incorrect or misspelled import statement for useState |
In case you incorrectly spelled ‘useState’ or got the casing wrong (e.g., `UseState`, `usestate`), JS is case sensitive and thus the interpreter will not be able to recognize the function. |
React version doesn't support Hooks |
If you are working with a version of React that predates anything around or below 16.8, it does not support Hooks, including `useState`. |
To resolve these problems, there are several approaches:
– Importing the useState hook: Make sure to add the appropriate import statement at the top of your React file where useState is being used. It should look like this:
jsx
import React, { useState } from ‘react’;
– Using correct syntax: Always use `useState`, JavaScript and by extension, React is case sensitive. ‘useState’ doesn’t equal `UseState` or `usestate`.
– Updating your version of React: If you are on a React version lower than 16.8 and you want to use Hooks, you have to update your version of React to the latest edition.
To paraphrase renowned computer scientist Donald Knuth: “Understanding programming languages isn’t about what you can find in the manual, it’s about what you can leave out.” This ideal rings true with the `useState` hook — understanding not just how to use it but also how to effectively avoid and resolve issues like ‘not defined no-undef’ error contributes to a smoother coding experience.
Understanding the “UseState is Not Defined No-Undef” Error in React
The error “useState is not defined no-undef” that might surface in React development environment typically means that the useState hook isn’t recognized or imported correctly into your React component.
useState
is categorized as a built-in function in React to manage local state in a functional component. Not executing the correct importation steps for this function will likely lead to the “useState is not defined no-undef” error message.
Let’s analyze the problem and its available solutions:
Situational Analysis:
Here, we can start with the basic implementation of
useState
inside a functional component:
function App() { const [value, setValue] = useState(initialState); //... }
If you get an error saying “useState is not defined no-undef” while trying to use it even in this way, it stems from useState not being imported from the ‘react’ library at the top of your file.
Possible Solutions:
• Importing
useState
at the beginning of your file. The correct procedure of importing this hook is directly from the ‘react’ library like shown below:
import React, { useState } from 'react';
By using this import statement,
useState
should be defined in your file now and can be utilized in your components.
• It could also transpire from typographical errors or casing discrepancies in useState. Remember it is not UseState or USESTATE but
useState
, JavaScript is case-sensitive.
According to software developer Dave Rupert,
“typos are the leading cause of software defects” [source]. This demonstrates how one small oversight can introduce bugs into your code, hence insure to double-check your spelling while coding.
Outcomes:
Hence, the error “useState is not defined no-undef” in React typically transpires when there is a problem involving the importation or proper usage of the useState hook. By executing an accurate importing syntax and recognizing JavaScript’s case-sensitivity, this error can be easily resolved.
Always requirement to ensure that all hooks and other components you intend to use are correctly imported and spelled in order to maintain a smooth and efficient development process.
Common Causes for “UseState is Not Defined No-Undef” Issue in React
The “useState is not defined no-undef” error in React commonly arises from a few key issues.
Comprehending these errors require an understanding of useState, which is a critical aspect of Hooks within the context of functional components in React.js. useState manages local state within a component, allows you to initialize a variable, and track its changes over time.
Error Source | Description |
---|---|
useState importation mistake |
If
useState was not imported correctly at the top of your component file from React library, it can cause this issue. Your import statement should look like: import React, { useState } from 'react'; |
Misspelled
useState |
Typographical mistakes such as misspelling useState transitioning to UseState or USESTATE which are not recognized by javascript language because it’s case-sensitive. |
Incorrect use of
useState |
Troubles may arise from using the function incorrectly. As an instance, if called outside the function scope where it has been defined. The recommended way of invoking useState is within the primary body of a functional component. |
To mitigate this frequently occurring problem, always ensure that the `useState` is imported correctly from the React package. Besides, regulate your typing accuracy to eliminate possibilities of confusion due to small typographical discrepancies, and keep your code tidy and ordered, aligning with best practices. Lastly, be attentive to where you’re calling useState to make sure it’s within the functional component body.
If these problems persist, consider accessing online developer resources and community platforms such as Stack Overflow, where many developers usually share similar issues, you might find valuable insights or solutions pertinent to your problem.
“It’s fine to celebrate success, but it is more important to heed the lessons of failure.” — Bill Gates on troubleshooting and iteration. It reminds us that encountering problems like “useState is not defined no-undef” is part of the learning process in mastering React.js or any other programming language.
Effective Solutions to Fix “UseState not Defined No-Undef” Error in React
The “UseState not defined no-undef” error in React is a common issue that developers often encounter when working with the built-in useState Hook. It typically signals that the way useState is invoked or imported is incorrect. Identifying the occurrence of this particular error is central and exploring practical solutions will tap into enhancing ReactJS development understanding, while focusing around ‘UseState Is Not Defined No-Undef React’ context.
Firstly, understand that
useState
must be imported from the ‘react’ module before it can be utilized. Forgetting to perform this necessary process might seem like a reasonably simple oversight, but it’s a critical action that can easily result in the highlighted error message. Below is an accurate way to import useState:
import React, { useState } from "react";
To clarify: in the above code segment,
{ useState }
is extracted from the ‘react’ bundle. This approach indicates you’re extracting a specific piece of the ‘react’ package, which in this case, happens to be useState.
Next, another plausible reason can be typos or spelling mistakes. Given how sensitive coding syntax can be, these seemingly trivial mistakes can end up causing significant issues such as our undefined error. Ensure that “useState” is accurately spelled, and proper casing (camel case) has been used.
Furthermore, sometimes misplacement of
useState
may lead to the error. Please note that Hooks, including
useState
, should always be called at the top level of your React functions – this ensures they are executed in the same order every time the component renders, thereby preserving the state correctly.
Lastly, installing old versions of ‘react’ or ‘react-dom’ that do not support hooks can cause the error. Uninstalling the outdated packages and then reinstalling the current versions can fix the problem. This can be done as follows:
npm uninstall react react-dom npm install react react-dom
As cited by Lautaro Carmona, a senior developer at Globant ― “Success in JavaScript, and particularly with its frameworks such as React, frequently hinges upon identifying symptoms of code failure and understanding how to swiftly rectify them.”1 Embracing this mindset will steadily improve your coding capabilities over time.
Error Type | Possible Explanation | Solution |
---|---|---|
“UseState not defined no-undef” | useState is not imported correctly from ‘react’ | Import useState using
import React, { useState } from "react"; |
“UseState not defined no-undef” | Typos or Misnaming | Ensure useState is accurately spelled with proper casing |
“UseState not defined no-undef” | Misplacement of useState inside React components | Always call Hooks at the top level of your React functions |
“UseState not defined no-undef” | Old versions of ‘react’ or ‘react-dom’ | Upgrade to latest versions of React and ReactDOM that support hooks |
Fixing such errors in applying useState function can enhance the functionality and stability of your application. Consequently, you can create more effective and dynamic user interfaces using React Hooks.
Preventing Future Incidence of “UseState Not Defined No-Undef” Error in React
The “UseState is not defined no-undef” error can typically surface when we are working with React’s Hooks feature, and particularly when useState has not been defined correctly. Though this might initially appear as a daunting issue, preventing future instances of it from happening can be achieved through following a set of best practices in specifying your application’s components.
Here’s how to prevent future incidence of the “UseState not defined No-Undef” error in React:
Action | Description |
---|---|
Importing useState correctly | The first step to prevent this error is to make sure that useState is imported from ‘react’ at the top of each component file. The standard convention is
import React, { useState } from 'react' , hence ensuring that React knows the origin of useState. |
Avoiding typos and syntax errors | Misnaming or incorrectly spelling useState can also trigger the no-undef error. Therefore, always cross-check useState declarations for any typographical errors. |
Maintaining version consistency | The useState Hook was introduced in React 16.8. So, discrepancy across different versions of the library can generate an undefined error. It’s important to regularize the React versions being used in both development and production environments. |
Enforcing ESLint rules | “no-undef” error comes from the ESLint. By ensuring all variables are declared properly you not only dodge this error but also enhance the quality of your code. So, adopting appropriate ESLint rules will contribute significantly towards prevention of this error. |
Apart from the remedies listed above, you could also delve into finer concepts in React. To quote JavaScript and React enthusiast Kent C. Dodds, “Understanding the nuances of how context works with Hooks can help prevent a whole world of bugs”. Therefore, it wouldn’t hurt to further explore How to use React context effectively.
By following these recommendations, you’ll not only rectify the ‘useState is not defined no-undef’ error but also improve the overall consistency and quality of your codebase. This Node.js example below shows how useState should be properly imported:
//File: MyComponent.js import React, { useState } from 'react'; function MyComponent() { const [value, setValue] = useState(0); // rest of your code ... } export default MyComponent;
This way you avoid the “no-undef” error by explicitly defining `useState`. It is important to remember that these best practices are designed to maintain code consistency across teams and projects – so adopting them early on is recommended.
In discussing “Usestate Is Not Defined No-Undef React” issue, a common quagmire encountered by JavaScript developers when using the useState hook in their React projects, three primary aspects emerge that need to be brought into focus.
The first and foremost factor that needs to be addressed is understanding what exactly the error message “Usestate Is Not Defined No-Undef” signifies. In essence, this error crops up whenever JavaScript encounters an undefined variable. Specifically, with respect to React development, such an occurrence can be traced back to a failed import of the `useState` Hook.
Example: If your code appears as follows –
function App() { const [state, setState] = Usestate(false); ... }
It’s crucial to remember that JavaScript is case sensitive – ‘Usestate’ (with capital U) is not recognized by JavaScript whereas ‘useState’ is.
Secondly, ensuring proper import procedure is key in eliminating this error message. The useState hook should be imported from ‘react’ library in the following way.
import React, { useState } from 'react';
Lastly, if you ensure all variables are defined before they’re used, this error can be avoided altogether. As Erich Gamma once said, “Specify everything explicit in your code, leave nothing to chances”. Although mere elimination of typographical errors may seem insignificant, it can oftentimes make the difference between smooth execution and bumpy debugging experience (source).
These steps underscore the fundamental ways to tackle the “Usestate Is Not Defined No-Undef React” error issue, thereby creating seamless and optimized user experiences in your React applications.