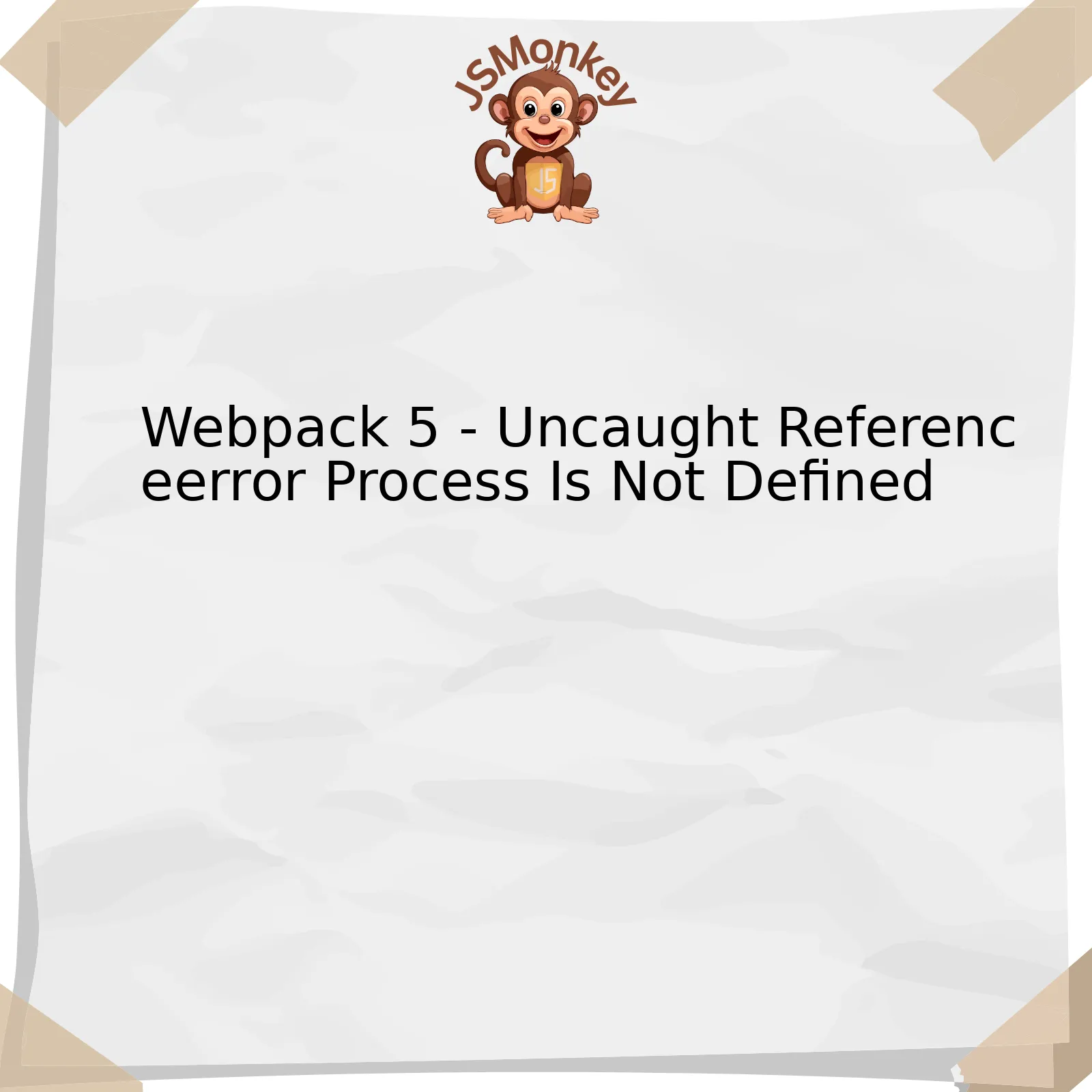
Let’s delve into the issue of ‘Uncaught Referenceerror Process Is Not Defined’ which you may encounter when transitioning from Webpack 4 to its newer version, Webpack 5.
Components | Webpack 4 | Webpack 5 |
---|---|---|
Polyfills | Automatically included | Not included by default |
‘process’ global keyword | Available out of the box | Undefined unless manually set up |
This table gives us a snapshot of the primary discrepancies between the two editions of Webpack that could lead to the said error. In Webpack 4, predefined polyfills for Node.js global variables like ‘process’ were part of its core setup. Thus, developers could readily use these keywords in their codebase without any configuration effort.
However, Webpack 5 has changed this approach with a new focus on not including such polyfills and global objects by default. This shift is part of an ongoing intention to deliver clean browser-compatible code and avoid bloating the build with unnecessary Node.js modules.
For developers who are upgrading their projects to Webpack 5 and utilizing the ‘process’ keyword in their code, this situation can pose quite a challenge. Without the automatic polyfills, the global ‘process’ will be undefined – resulting in the error ‘Uncaught ReferenceError: process is not defined’.
The solution to this issue lies in explicitly setting up these polyfills if they are required by your project. You can define a ‘polyfills.js’ file in your project and redirect Webpack to this file by adding it to the ‘entry’ property of the Webpack configuration file.
Here’s an example of how you can fix this problem in code:
// polyfills.js global.process = { env: { NODE_ENV: 'production' } };
By doing so, you tell Webpack that the global ‘process’ and its environments should be manually defined, thereby dealing with the ‘Uncaught ReferenceError: process is not defined’ error.
As Larry Wall, the original author of the Perl programming language, opined:
> “The three chief virtues of a programmer are: Laziness, Impatience, and Hubris.”
By understanding the evolving choices made by Webpack 5, developers will find better ways to maintain their code, putting into practice Wall’s virtues of effective and agile development.
For more information on adjusting your coding for Webpack 5, HTMLPurger provides a detailed guide.
Understanding the Webpack 5: Uncaught ReferenceError Process Is Not Defined
Unraveling the issue of “Uncaught ReferenceError: Process Is Not Defined” with Webpack 5 requires a thoughtful analysis that delves into essential areas of JavaScript development, Node.js, and bundling libraries like Webpack. In this discourse, I will highlight the core reasons for this common error message and propose efficient ways to tackle it.
To comprehend the problem, we first need to understand the role that
process
plays within our applications. Primarily utilized inside Node.js environments,
process
is a global object that essentially provides access to system-related functionalities, such as the application’s environment variables through
process.env
. However, in a browser context that employs Webpack, the
process
object does not exist in its usual Node.js form, which necessitates an alternative approach.
Approximating at a causal perspective, “Uncaught ReferenceError: Process Is Not Defined” typically manifests when your JavaScript code anticipates a Node.js-like environment where the
process
object would be available globally. This anticipation could exist implicitly or explicitly. Particularly, certain modules or libraries might heedlessly draw from
process.env
with the presupposition that they execute in a Node.js ambiance.(source)
Subsequently, when Webpack builds such applications for the browser, it stumbles upon the
process
or
process.env
references and attempts to offer shims. Nonetheless, Webpack 5 has taken a step back from providing automatic “polyfills,” leading to instances of “Uncaught ReferenceError: Process Is Not Defined.” Herein, some developers may encounter unforeseen issues, despite their code running fine on older versions of Webpack.
Essentially, the solution is to manually instruct Webpack on how to handle the
process
variable whenever it comes across it. The popular method for achieving this is through the use of the DefinePlugin, which replaces specified strings in your code with assigned values during compile-time. The following code extract offers a useful illustration:
const webpack = require('webpack'); module.exports = { // ... plugins: [ new webpack.DefinePlugin({ process: 'process/browser', }), ], };
This snippet tells Webpack to replace all
process
references with
process/browser
when preparing the bundle for the browser. Once again, bear in mind that
process/browser
does not entail end-to-end API compatibility with the real Node.js
process
but, majorly, it resolves “undefined” issues while working with common libraries.
Industry expert Addy Osmani beautifully illustrates the concept, stating, “Understanding your tools at a fundamental level is key to being able to effectively troubleshoot them.”
Gradually navigating through these technical terrains, we can derive nuanced insights in using modern development toolkits and judiciously maintain compatibility for resilient JavaScript applications.(source) By elaborately understanding the nature of our environments, we can skilfully evade errors such as the “Uncaught ReferenceError: Process Is Not Defined” in Webpack 5.
Debugging Techniques for ‘Process is not defined’ error in Webpack 5
When it comes to confronting a `Process is not defined` error in Webpack 5, understanding the causes and suitable debugging techniques becomes imperative. This particular issue could be part of the broader problem usually presented as ‘Webpack 5 – Uncaught Referenceerror Process Is Not Defined’. A more detailed exploration can further clarify this issue.
Webpack 5 omits inclusion of the
process
module automatically into built bundles, unlike its predecessor version, Webpack 4. So, when your code references the `process` object without defining it first, an ‘Uncaught ReferenceError: Process is not defined’ gets thrown.
Debugging Techniques:
Techinque | Description |
---|---|
Environment Definition Plugin: | This tool replaces free variables in modules with a static value during compile time. It can help webpack 5 recognize the process object whenever it’s referenced in the code. Adding the Environment Plugin can be your first step towards overriding ‘process not defined’ error. Here’s a code snippet for reference:
plugins: [ new webpack.EnvironmentPlugin({ NODE_ENV: 'development', // use 'development' unless process.env.NODE_ENV is defined DEBUG: false }) ] |
Code Modification: | If there are only a few instances in your code where the ‘process’ object is being used, it might be worthwhile to modify those specific areas by checking if the process object exists before using it within a function. Make sure to use fallback values or actions if the process is undefined. This technique ensures that the functionality doesn’t break in absence of ‘process’. |
Use Define Plugin: | It’s a built-in webpack plugin that allows you to create global constants which can be configured at compile time. This could potentially solve the issue by defining ‘process.env’ variable globally in your webpack configuration file, like:
new webpack.DefinePlugin({ 'process.env': JSON.stringify(dotenv.parsed) }) |
In resolving this issue, focusing on retaining your site performance is paramount as the solution must not involve bringing back the whole node.js polyfill into the bundle. As William E. Wheeler said, “Good code is its own best documentation. As you’re about to add a comment, ask yourself, ‘How can I improve the code so that this comment isn’t needed?’” Aim for writing clear and concise code, implementing only necessary changes to work around the error. (source)
Browser Compatibility issues linked with ‘Process is not defined’
While delving into JavaScript and its numerous development frameworks, it’s not uncommon to encounter an error like ‘Uncaught Referenceerror: Process is Not Defined’. Specifically, with the introduction of Webpack 5, several developers have reported experiencing this issue more frequently. Let’s unpack what this means and how to resolve it.
In essence, when you come across ‘Uncaught Referenceerror: Process is Not Defined’, it implies that your browser is trying to access the
process
object which isn’t defined within the client-side JavaScript environment. It’s coming from Node.js where
process
is a global node variable that exposes information about the current state of the Node process containing the plurality of system variables.
Webpack, for much of its lifecycle, has included polyfills for certain node.js globals and modules to seamlessly transit to the browser. However, Webpack 5 as the “latest and greatest” version introduced a break in this convention. The new management policy decided to stop including various Node.js core modules and globals polyfills automatically, including the famously used
process
. Since browsers don’t define a
process
object naturally (like Node.js does) – hence the error message.
Let’s dive into the solution:
A common approach to resolve these issues in Webpack 5 is to configure your webpack settings manually. This modification can be done within the
webpack.config.js
file by adding the
ProvidePlugin
.
Include this snippet in your configuration file:
javascript
plugins: [
new webpack.ProvidePlugin({
process: ‘process/browser’,
}),
],
The
ProvidePlugin
here is telling Webpack to include the equivalent browser version of the
process
whenever it comes across it in your code. Here, it maps the undeclared
process
variable to the equivalent browser version from the ‘process/browser’ module, essentially creating a replacement strategy.
Once you’ve made the necessary changes in your configuration file and saved it, rerun your build command. The ‘Uncaught Referenceerror: Process is not Defined’ error should no longer be present.
Remember the wise words of Linus Torvalds, creator of Linux and Git, “Good programmers know what to write. Great ones know what to rewrite (and reuse)“. So, ensure that finding solutions to errors should be seen as an opportunity for learning and growth rather than a setback.
Mitigating the Webpack 5 ‘Uncaught Referenceerror Process Is Not Defined’: Best Practices
The error “Uncaught ReferenceError: process is not defined” in Webpack 5 may result from a significant breaking change introduced with the version upgrade. In comparison to its predecessor, Webpack 5 no longer includes node.js polyfills automatically. This change aims to make the tool more suitable for frontend development, as most of these polyfills are unneeded and add unnecessary weight to the bundle.
Though it might seem like a setback, there are strategies to mitigate this problem and ensure your project runs smoothly. These practices can also improve the overall efficiency of your codebase. Let’s delve more deeply into them:
Webpack’s EnvironmentPlugin
One effective practice involves utilizing Webpack’s EnvironmentPlugin to create global constants which can be configured at compile time.
const webpack = require('webpack'); module.exports = { ... plugins: [ new webpack.EnvironmentPlugin(['NODE_ENV']) ] }
In this example, ‘NODE_ENV’ is added to EnvironmentPlugin. It replaces every instance of `process.env.NODE_ENV` in your source code with the stringified value from `process.env`.
ProvidePlugin
Another strategy is using Webpack’s ProvidePlugin to automatically load modules without having to import or require them explicitly.
const webpack = require('webpack'); module.exports = { ... plugins: [ new webpack.ProvidePlugin({ process: 'process/browser', }) ] }
Here, the ProvidePlugin is adding a pseudo `process` object provided by the shim package `process/browser`, which could be installed via npm or yarn as a bare minimum replacement for node.js ‘process’ object.
Fallback Feature
You can also use Webpack 5’s resolve.alias to define alternative packages. Consider an example where you are trying to use a package in the browser that depends on built-in node.js modules:
module.exports = { resolve: { fallback: { "process": require.resolve("process/browser") } }, }
In this setup, we use the ‘fallback’ property to replace the `process` package by a browser-compatible alternative.
For more on this topic, refer to the official Webpack 5 documentation about basic configuration as well as these mitigation practices.
As Bill Gates once said, “The computer was born to solve problems that did not exist before.” While upgrading to Webpack 5 may initially cause some issues due to changes in default behavior, adapting your configurations and understanding why these changes were made unlocks new possibilities for optimization, performance improvements, and more efficient builds.
Webpack 5 has undoubtedly transformed and simplified the way JavaScript applications bundle dependencies. However, unique error messages such as “Uncaught ReferenceError: process is not defined” have been a common issue faced by many developers.
The underpinning cause of this problem is that Webpack 5 doesn’t include ‘process’ polyfill to emulate Node.js global variables by default, contrary to its predecessors. The objective behind this shift in functionality was to trim down the final bundle size and proffer developers more control over the inclusion of specific polyfills.
This unexpected error can impede development flow, but worry not – there are numerous solutions available:
- Using the DefinePlugin in Webpack’s configuration file, you can provide your own process or process.env.
- You may also consider installing the ‘process’ npm package and then integrate it into your code where necessary.
new webpack.DefinePlugin({ 'process.env.NODE_ENV': JSON.stringify('production') })
It’s encouraging to note that, despite these minor hiccups, Webpack 5 remains an indispensable tool for JavaScript application development which aims to streamline the bundling mechanism in an increasingly intricate ecosystem. It further represents an evolutionary step towards performance optimization and greater developer control.
Although these changes lead to some initial confusion as pointed out by [Jeremy Sachs on his blog](https://xes.cx/archive/2021/07/docs-webpack-js-org-guides-migration-5-md.html), they ultimately indicate Webpack’s move towards favoring a fully browser-compatible configuration over implicit Node.js shims.
“Computers themselves, and software yet to be developed, will revolutionize the way we learn.” – Steve Jobs