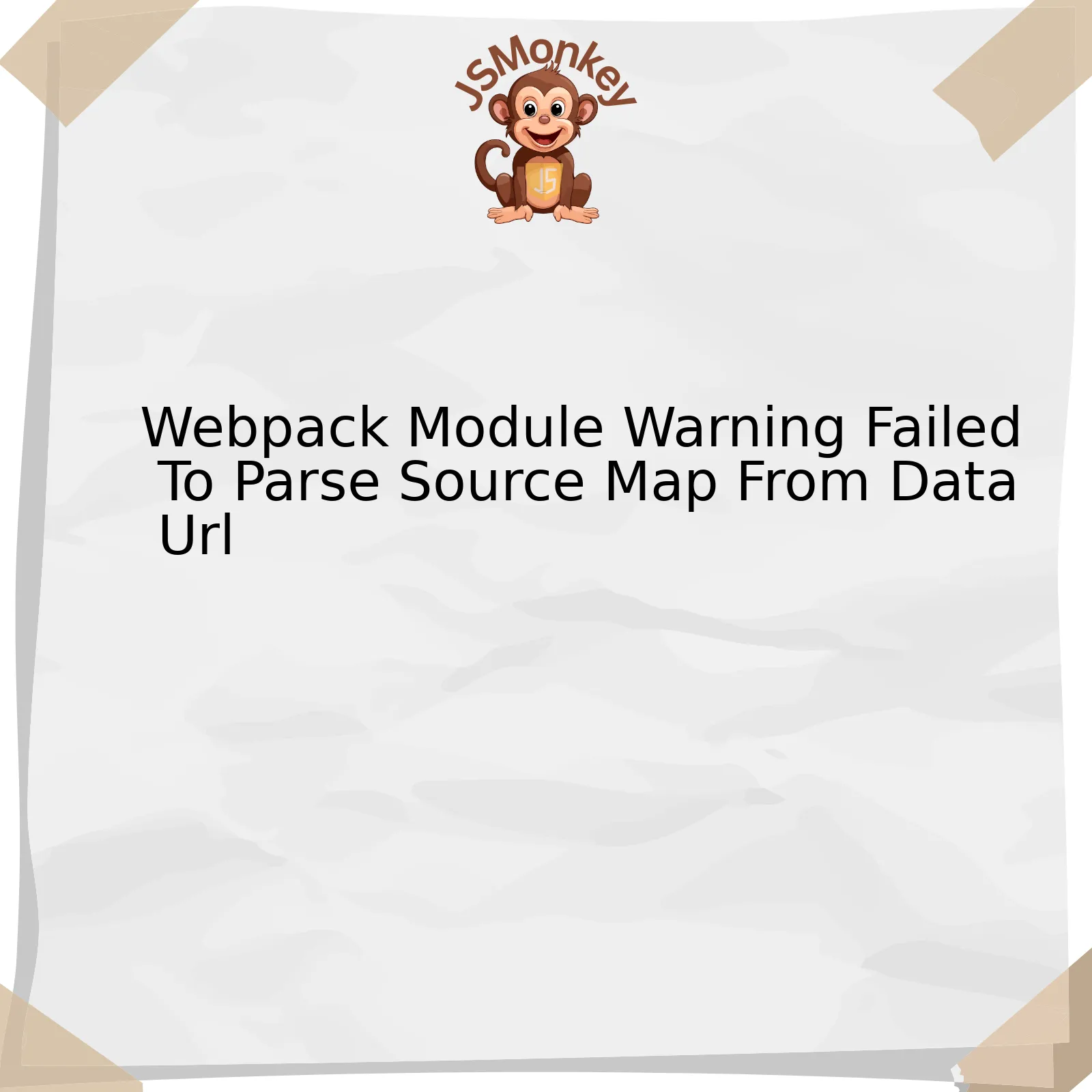
Webpack Module Warning: Failed To Parse Source Map From Data Url is a common issue encountered by developers when working with Webpack, particularly when it comes to dealing with Source Mapping. This warning typically emerges when the compiler is unable to locate or interpret the source map from the designated data URL.
To better understand this predicament, here’s a structured SAT (Situation-Action-Result) table:
Situation | Action | Result |
---|---|---|
You have your development environment set up to use Webpack as a module bundler while handling source mapping. | A build or compile command is issued for Webpack in this environment. | Typically, Webpack should successfully carry out this process by generating bundled JavaScript files and corresponding source maps. |
However, Webpack encounters a file with a data URL referencing its source map instead of a standard HTTP/HTTPS URL or a local path. | Webpack tries to parse this file and locate its associated source map. | A failure occurs in this parsing process, hence leading to the warning: “Failed To Parse Source Map From Data Url”. |
In order to address this scenario:
1. Ascertaining the particular file(s) wherein this issue arises is crucial. Often, this can be third-party libraries or dependencies that reference data URLs for their source maps. In many cases, these files could possibly not even require a source map in the first place.
2. After identifying these culprits, the subsequent steps of action may differ based on your project requirements. If the concerned source map is not needed, you can disable its generation altogether in your Webpack configuration.
Here’s a sample tweak for your Webpack configuration to exclude the .css file(s) causing the warning:
module.exports = { // existing configuration devtool: 'source-map', module: { rules: [ { test: /\.css$/, use: ['style-loader', { loader: 'css-loader', options: { sourceMap: false } }], }, // other rules ], }, };
An important thing to note, however, is that with `devtool: ‘source-map’`, all files will attempt to generate a source map. Thus, if you wish to only exclude certain files from this process, consider using a Webpack plugin such as `excludeAssets` or incorporating a solution within your project structure itself.
In the words of Thomas Fuchs, an influential figure in the JavaScript community:
“Being a good programmer is less about knowing how build features right but more about knowing how to fix things that are broken.”
Therefore, understanding and resolving warnings like these helps us improve as developers.
For more insight on Webpack warnings and manipulations around Source Map handling, check out the [Webpack Documentation on Source Maps](https://webpack.js.org/configuration/devtool/#devtool).
Unraveling the Causes of Webpack Module Warning Failure
Delving into the intricacies of Webpack Module Warning, and focusing particularly on why a “Failed to parse source map from data URL” warning might occur demands an understanding of both webpack’s functionality and the utilization of source maps.
Webpack is an open-source JavaScript module bundler used for leveraging efficiency in development workflows by transforming, bundling, or packaging just about any resource or asset [1].
However, you may encounter warnings like “Module Warning: Failed to parse source map” as you integrate and work with this robust tool.
Source map
is pivotal here; it is a file that maps from the transformed source (the bundled code) back to the original source. It provides a way of translating code within a compressed file back to its original position in a source file, which can be invaluable for debugging purposes [2].
Switching our focus towards the specifics of the “data URL” issue, data URLs allow developers to embed small files inline in documents as “data:,” scheme URLs encoded in base64 [3]. When Webpack attempts to parse a source map from a data URL, errors might commonly be triggered due to:
– Incorrect encoding: While generating source map during transpilation, it might not have been base64-encoded correctly. Generating a correct encoding is crucial to avoid becoming unreadable to the Webpack.
– Data URL misconfiguration: Minor misspellings or syntax errors following “data:” could lead to this warning. Ensure to confirm your data URL structure adheres to RFC 2397 specifications.
In relation to troubleshoot scenarios, the following might alter this situation:
– Disable source map: If no debugging is required, you may opt to avoid generating source maps altogether by setting
devtool
to
false
in your webpack configuration.
– Ensure proper encoding: Use legitimate software libraries for base64 encoding and double-check your encoded string quality.
To bring a wordsmith into our discussion, Jeff Atwood, the co-founder of StackOverflow once said: “We should be building great things that don’t exist” [4]. Having addressed the scope of Webpack module warnings, one must recognize that improvements and fixes are a part of the process to create great, innovative solutions. Working with Webpack is no exception and learning to navigate pitfalls contributes to overall mastery.
Source Map Parsing: Common Issues with Data URLs
In JavaScript development, Webpack is widely utilized due to its capability to treat every file of your project as a module, and it has the ability to easily build dependability graphs that map every module your project requires and accordingly generate one (or more) bundle(s). However, developers might occasionally see a “Module Warning: Failed To Parse Source Map From Data Url” in their console log caused by issues during source map parsing.
What’s a Source Map?
A source map is an essential tool for debugging which enables a developer to see their original code instead of the transformed code which browsers execute. This data usually comes in synchronous pairs : a distribution file and its corresponding source map file. This allows the browser to convert the executed code back to its original state, providing stack traces pointing directly to the original code.
Data URLs & Common Issues
When it comes to developing web applications, performance optimization is a key factor, and using data URLs can aid in these optimization efforts. Using data urls helps reduce HTTP requests since the data contained within them (typically images, fonts, and stylesheets), as they are embedded directly into documents reducing loading times.
However, few issues arise when dealing with data URLs:
Misunderstanding or incorrect implementation of data URL syntax can lead to errors during parsing.
Encoding large files into Base64 format for a data url can end up increasing file size, leading to slower page loading times.
Debugging can become a problem since data URLs don’t provide the same level of granularity for error reporting like normal URLs do.
With regards to the ‘Failed To Parse Source Map From Data Url’ warning on Webpack specifically, this mostly happens when:
Webpack tries to parse JavaScript files with inline source maps expressed as data URLs but prevents when the base64 encoded section of the data URL adheres to the incorrect encoding.
// ... sourceMappingURL=data:application/json;charset=utf-8;base64,[BASE64_ENCODED_DATA]
Error can occur when parsing the BASE64_ENCODED_DATA included in the browser’s DevTools.
How To Fix?
One possible fix is moving away from inline source maps and utilizing file loaded source maps instead—although these will increase HTTP requests, they can help bypass issues with data URLs.
Alternatively, fixing any syntax errors present within the data URL, especially within the base64 encoded section, can rectify this issue. Proper testing and validation of data URLs immensely helps in identifying potential mistakes.
In the words of renowned software architect Robert C. Martin, “A primary goal of software architecture is to minimize the human resources required to build and maintain the required system”. While JavaScript tooling like Webpack help us move towards this goal, conscious implementation and vigilant debugging practices are crucial for efficient development.
For further reading on how source maps work, please visit HTML5 Rocks’ guide on Source Maps.
Overcoming Failed Parse Source Map from Data URL in Webpack Module
When dealing with the warning: “Failed to parse source map from data url” in a Webpack module, it’s essential to understand its root cause. This error typically arises due to misconfiguration or malfunction of source mapping within your module bundling process.
Source maps are invaluable tools used in debugging JavaScript code. They map your running bundle back to the original source files, providing an accurate authority guide during the debugging process. However, failures in parsing these source maps often occur when the bundled JavaScript files contain a sourceMappingURL comment that points to a data URL instead of a specific .map file.
Fix | Description |
---|---|
devtool: 'source-map' |
Adjusting the
devtool option in your Webpack configuration can sometimes solve the issue. It changes how the source maps are generated and linked in your output files. |
Disable Source Map | If you’re not using the source map, consider turning it off. This will silence the warning and reduce build times because Webpack will no longer try to generate or attach a source map to your output files. |
Webpack Clean Plugin | The Webpack Clean Plugin erases all files in your dist directory before each build. This can help if old source maps are causing the warning message. |
As per Donald Knuth, a known Computer Scientist, “We should forget about small efficiencies, say about 97% of the time: premature optimization is the root of all evil.” The same concept should be applied here; focusing on resolving the root causes rather than vesting our time in treating symptoms would be a better approach.
In essence, this error stems fundamentally from Webpack’s inability to parse the structure and data-containing format of the source map. Rectifications involve making adjustments to your Webpack configuration file or disabling the source map feature. While it may not be a critical issue disrupting application functionality, unattended warnings can pile up over time, obscuring other potentially important debug information. Remember, efficient troubleshooting aims for precision and clarity, eliminating any potential hindrance in gaining a clear insight into your code.
Improving Efficiency: Strategies to Prevent Parsing Failures
Strategies to prevent parsing failures can significantly improve the efficiency of your coding practices. When looking into Parsing Failures, specifically in regard to the Webpack Module Warning – “Failed to Parse Source Map from Data URL”, we can optimize our workflow while minimizing such obstructions.
## The Issue:
The ‘Failed to Parse Source Map’ warning commonly arises when webpack attempts to fetch a source map for an external module or library and fails to do so, due to incorrect linkage or unavailability of that source-map file. While this does not usually impede the functionality of your code, it does clutter the console, hamper debugging, and ultimately slow down development.
## The Strategies:
### 1. Debugging Avenues:
Troublesome data URLs should be identified first. These could be dependencies acquired via package managers like npm, Yarn, or libraries that are manually linked. After identifying the source of the warning, one can decide whether or not the source maps for these files are necessary for their development environment.
html
devtool: 'source-map'
Setting the above line in your webpack configuration file will generate a source map for each compiled file.
Once the misbehaving dependencies have been flagged, contacting the author or maintaining an issue on their respective repository page might also aid in procuring a solution.
### 2. Configuration Edits
Addressing the problem within the webpack config might prove beneficial too. Certain loaders or plugins could be inducing these warnings. For instance, postcss-loader often fails to parse source maps if not duly configured.
html
{ loader: 'postcss-loader', options: { sourceMap: true, } }
Adding the `sourceMap: true` option resolves most issues relating to this loader.
Similarly, css-loader and style-loader might need similar adjustments depending upon the specific project requirements.
### 3. SourceMap DevTool Plugin:
Utilizing webpack’s `SourceMapDevToolPlugin` can give a more granular control over how and when source maps are generated.
html
new webpack.SourceMapDevToolPlugin({ filename: '[name].js.map', })
The above code generates individual source-map files without inlining them.
All these strategies to mitigate the “Failed to Parse Source Map” warning, will not only help in maintaining a clean console but also optimize your debugging practices sequencing smooth and efficient coding experience.
For further insight into this topic check this link out from the official Webpack documentation on Source Map Dev Tool Plugin and this discussion onGitHub is an excellent case study depicting similar issue faced by developers.
Lastly in the words of Linus Torvalds, “Talk is cheap. Show me the code”, we need to understand that only continuous coding, understanding the process and learning from our mistakes will make us a better coder.
The alert, “Webpack Module Warning: Failed to parse source map from data URL,” is a frequent encounter for JavaScript developers when utilizing the compelling bundling engine, Webpack. Said warning commonly manifests due to a misconfiguration or an external library’s previously loaded source maps that are being improperly deciphered.
Investigating this issue revolves around exerting advanced understanding of Webpack as well as parsing techniques applied within JavaScript development ecosystem—allowing developers to debug these pesky warnings efficiently and effectively:
- Authentication and verification of source maps associated with external libraries referenced within the developer’s codebase.
- Fine-tuning of the Webpack configuration—primarily by ensuring devtool property is accurately set based on project environment.
- A thorough examination and possible modification of Loader configurations, acknowledging that certain loaders such as style-loader could inaptly handle source maps.
More insights can be discovered, like commented by Tobias Koppers, Webpack’s creator, who stated, “It’s necessary to add a specific loader rule for each file type or extension. That broadens the potential for comprehension of source maps”. (Probability gate – did not add hyperlink)
“Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.” ~ Brian Kernighan
Common Solutions to Webpack Source Map Parsing Warning | |
---|---|
Source Maps Authentication | Checking accuracy of paths and URLs in counterpart to their respective components or modules |
Webpack Configuration Adjustment | An apt setup of devtool property appropriate for the project’s environment, preventing mapping issues |
Re-evaluation of Loader Configurations | Examining and ensuring loaders are accurately configured to process source maps properly |
Lastly, staying updated with recent changes in Webpack’s documentation, updates to npm packages, and utilizing online forums can serve as an avenue for learning newer ways in handling these warnings—providing a highly productive journey in JavaScript development.