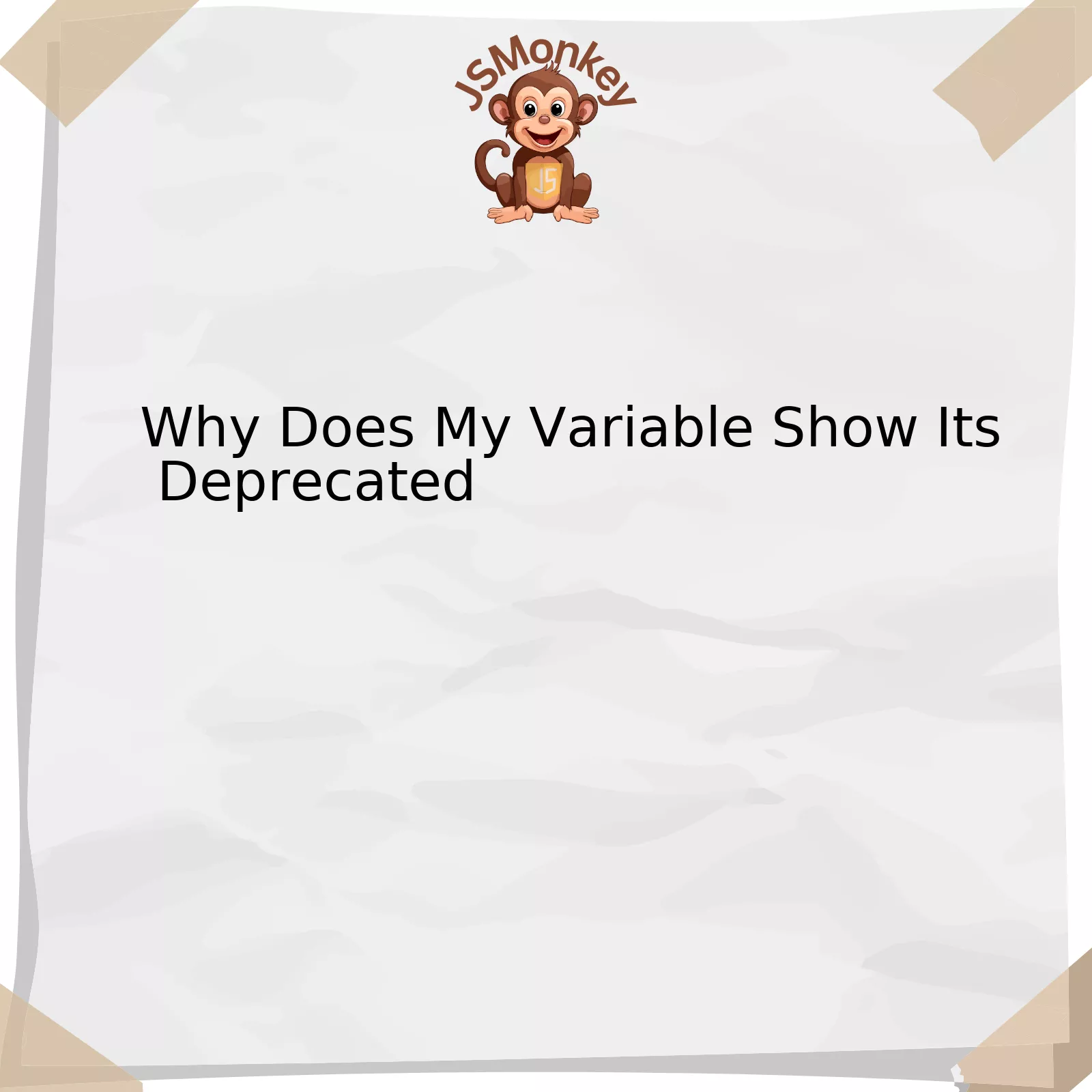
Possible Causes | Implications | Solutions |
---|---|---|
Outdated Javascript Syntax | The variable might be deprecated due to utilizing outdated JavaScript syntax that has been superseded by newer and more efficient methods. | Remediate the issue by updating your codebase using the latest ECMAScript (ES) standard. |
Deprecated API/Library Usage | This commonly happens when a variable is tied up with APIs or libraries which are no longer supported. | Aim for replacing the deprecated API or library calls with its updated version or an altogether different but updated library solving your purpose. |
Lack of Updates in the Underlying Platform | Your variable may appear as deprecated due to lack of timely updates on the underlying platform. | Ensure to regularly update your environment, infrastructure, package dependencies ensuring they’re all of the most up-to-date version. |
For a better understanding, take the first row that talks about outdated JavaScript syntax. Variables can be considered deprecated if they make use of old JavaScript syntax or methods which have since been updated or replaced. Mostly Javascript developers prefer using enhanced features given under ECMAScript 6 (also known as ES6), ES7 standards because these versions brought a manifold of advanced ways to define variables more reliably like `let` and `const`.
Moving on to the second possible cause, deprecated API/library usage. You may have defined your variable using an API or library that is no longer in use or has been updated. For instance, the
substr()
method in JavaScript was widely used for string manipulations but later got deprecated and recommended to use
substring()
or
slice()
.
Taking a look at the last cause, which is lack of timely updates on the underlying platform where your Javascript code runs. Sometimes, the execution environment might have changed without you being aware, leading some variables to be flagged as deprecated. In this regard, regular monitoring and maintenance of your infrastructure and service dependencies is a key factor.
To quote Edsger W. Dijkstra – “Program testing can be used to show the presence of bugs, but never to show their absence!”, always perform regular audits and tests across your application to ensure no deprecated elements exist. Keeping your codebase up-to-date with the latest syntaxes, libraries/APIs, and platform versions will minimize the risk of facing deprecated variables. It can also provide enhanced features, improved performance, and security patches.
Understanding the Concept of Deprecated Variables
The term deprecated in software development or more specifically in JavaScript, refers to a practice where the language authors discourage the use of certain features or functions. Essentially, this means an aspect of the code that, even though currently usable, may be removed or altered significantly in future updates of the language.
When you come across a variable in your code that’s showing as deprecated, it is signaling towards the discontinuation or discouragement of use in future versions. The main reasons why variables are deprecated include:
- Presence of better methods for achieving the same functionality.
- Security risks concerning the use of these variables.
- Improvement in language efficiency and optimization.
Despite this, you might wonder why the language developers don’t just entirely remove these deprecated features immediately. This allows a transition period during which developers can gradually phase out the use of these features from their codebases.
For instance in Node.js, there was a depreciation warning for Buffers. They launched the Buffer API years ago when JavaScript had no mechanism for reading or manipulating binary data. However, as JavaScript evolved, new alternatives like
Buffer.alloc()
,
Buffer.from()
came into scenario which was considered safer.
Bill Gates once said, “Software innovation, like almost every other kind of innovation, requires the ability to collaborate and share ideas with other people.”
In order to keep this spirit of collaboration and continuous improvement, it’s essential to stay updated with these changes, adapt to them and refactor our code accordingly. When you see a deprecation warning, check the documentation or search online for modern ways to achieve the same functionality. If the warning doesn’t contain enough detail about what to do, Stack Overflow might have a thread on the subject. In addition, consider using tools like JSHint or ESLint – which can help catch these issues during the development process.
While dealing with deprecated variables might seem troublesome at times, it’s an integral part of ensuring software safety, efficacy, and future compatibility. Therefore, understanding them is vital to successful, enduring JavaScript coding.
Common Causes and Solutions: Deprecation Warnings in Programming
Understanding why a variable shows as deprecated involves exploring the complex world of software lifecycle management and its impact on programming. Deprecation warnings in programming are usually issued when a part of the language or library is considered legacy, no longer maintained or better alternatives have been recommended by the creators for future use. This concept applies to JavaScript too, where a variable can become deprecated.
Causes
Firstly, it’s helpful to recognize the common causes associated with deprecation warnings:
Cause | Description |
---|---|
The advent of new features | Programming gradually evolves. A newer, potentially more efficient, alternative may make an older feature or variable irrelevant. |
Issues with the original feature | A feature might be deprecated if it presents performance issues, bugs, vulnerabilities, or lacks certain capabilities. |
Improved standards and practices | As programming paradigms shift, certain variables and methodologies may not adhere to these newer standards, leading to their deprecation. |
Based on the above scenarios, your variable could show as being deprecated due to any (or possibly all) of these reasons.
Solutions
Deprecation doesn’t mean immediate removal. It’s merely a signal that developers should start transitioning away from the deprecated elements. Here’s how you can approach a solution:
– Update Your Code: Upon receiving a deprecation warning, explore the documentation or community forums for alternative variables or approaches introduced by the creators of the language or library. Adapt your code to these new features which eventually makes your code more compatible with future versions of the platform or language.
For example, if a variable `A` is deprecated and the creators recommend using a new variable `B`, you can replace instances in your code where `A` was used with `B`.
let A = value; // Deprecated
to
let B = value; // Recommended alternative
– Staying Updated with Changes: Make sure to keep track of the updates for the tools and languages being used. Sign up for newsletters, follow relevant blogs, participate in related forums, etc. Also, regular auditing of dependencies can help in identifying and addressing any deprecated elements quickly.
As Robert C. Martin, a prominent figure in software development, said, “The only way to make the deadline—the only way to go fast—is to keep the code as clean as possible at all times.” By updating and continuously improving your codebase, deprecation warnings can be avoided and quality code can be maintained.
Ultimately, deprecation is a key aspect of software evolution. Understanding how to anticipate, manage, and navigate deprecations efficiently is a crucial skill for any successful javascript developer.
Tips to Debug Deprecated Variable Errors
Debugging deprecated variable errors is a critical skill to master as a JavaScript developer. These errors pinpoint variables in your code that have been replaced by new features or practices.
Deprecation is not an error in itself; it is more of a warning from the development community advising against the usage of certain features. The considerations regarding the deprecation of a variable and the possible implication in our code can be better apprehended with the following points:
Understanding Deprecation
Firstly, it’s important to clarify why a variable would show as deprecated. Deprecation warnings usually occur because:
- The feature was phased out due to better alternatives.
- The feature was disapproved due to impact on performance and efficiency.
- The usage was not up to standard coding conventions and best practices.
When these variables are not updated in the code base, they might lead to inefficiencies, bugs, or even failure in the future when no longer supported.
Use Developer Tools Effectively
Developer tools, especially console logs and breakpoints, are vital resources for identifying deprecated variables. Console logs give insights into the behavior of your application, while breakpoints allow you to pause the execution of your script at a particular point and inspect the variables manually.Mozilla’s debugger documentations is a good resource to get started with if you’re not accustomed to these methods.
Employ Linters & Static Code Analysis
Linters like ESLint, are static code analyzers that can check your code for anti-patterns and deprecated features used before runtime, helping an average programmer avoid subtle programming errors, filling gaps that may not be detected by compilers or interpreters, and enforcing uniformity.
Looking Up Documentation
Once you’ve found the deprecated variable, a great practice is to look them up in the language’s official documentation or on MDN Web Docs. This will provide you with information about the best replacement for the deprecated variable and how to use it optimally.
As Jim Highsmith, one of the original authors of the Agile Manifesto, says “Good code is its own best documentation.” It is imperative that we adhere to this principle and keep our code reliable, extensible and maintainable.
Effectively Updating Your Codebase for Non-Deprecated Variables
Updating your codebase effectively to accommodate for non-deprecated variables, and consequently shunning deprecated variables, is of utmost importance in maintaining the functionality as well as efficiency of your applications. This task becomes even more crucial when dealing with peculiar cases where the variables show up as deprecated in usual circumstances.
Let’s first understand what a deprecated variable is in terms of JavaScript programming language. A variable might be flagged as deprecated when it’s designated for removal in the future updates of the language. If the system informs you that your variable is deprecated, it means that either you are using an outdated syntax or you are utilizing a method or property that might not be available in future releases.
In such situations, frequently reviewing and adjusting your codebase could prevent potential breakdowns of your application due to discontinued support for outdated functionalities.
Process for Updating Your Code
Here are some steps you might want to consider to ensure your codebase stays free from deprecated variables:
1. Keep Track of Updates: Keeping informed about any new developments concerning the language of your codebase is key to staying ahead of deprecations.
2. Identify Deprecated Variables: Use a modern IDE (Integrated Development Environment) that will highlight deprecated variables directly in your code.
3. Use Updated Standards: Aim to use the latest standards of JavaScript (like ECMA2015 and beyond), which tend to deprecate less variables and methods.
4. Refactoring: Periodically refactor your code to replace depreciated functions or methods with their newer counterparts.
5. Automating the Process: You may employ tools like linters that will automate this whole process by instantly flagging deprecated functions or variables.
Now, coming to exact problem you have, i.e., “Why Does My Variable Show Its Deprecated”, there might be a couple of reasons behind this:
1. Outdated Syntax: You are likely using syntax that has been deprecated in the newer versions of JavaScript.
2. Deprecated Function or Method: The variable that you have declared might be linked to a method or function whose usage has been deprecated.
In both cases, a quick Googling with something like “JavaScript <variable/method> deprecated reason” should lead you to the answer. Once you identify what exactly is triggering the deprecation warning, you can look for its modern counterpart and refactor your code accordingly.
To put it simply, suppose you are using
var
which might be flagged as deprecated in some contexts. You would replace instances of
var
with let or const like so:
//Old, possibly deprecated usage var variableName = 'Hello World'; //Updated usage let variableName ='Hello World';
Robert C. Martin, a renowned software engineer and author, once said, “”Indeed, the ratio of time spent reading versus writing is well over 10 to 1. We are constantly reading old code as part of the effort to write new code. …[Therefore,] making it easy to read makes it easier to write.”” This quote perfectly sums up why keeping your codebase up-to-date and free from deprecated variables is vital not only for your application’s overall health but also for aiding your development process.
While updating your codebase, always aim for readability and understandability. Your future self (and other developers) will thank you for this consideration.”
Scripting in JavaScript offers vast and dynamic possibilities. Nevertheless, when confronted with the message that your variable is deprecated, it may leave you scratching your head. If we dig deeper, deprecation essentially serves as an alert signifying a particular syntax or function coding method is considered outdated or to be phased out in future updates of the language.
The root cause of this issue often lies in the evolution of JavaScript over time. As newer ECMAScript versions are released, some functions or methods from older releases become ineffective or inefficient. When a specific feature gets the label “deprecated”, the language runners are signaling that there’s likely a more efficient alternative now available.
There are multiple reasons why variables might be deprecated:
- Improvements in code efficiency: With each new version of ECMAScript (the standard for JavaScript), enhancements to the language lead to more efficient coding practices.
- Enhanced security features: Deprecated functionalities are sometimes replaced with more secure alternatives.
- Alignment with modern programming trends: At times, traditional ways of doing things might get removed to align with contemporary trends and practices in web development.
It’s important not to ignore these deprecation messages because most likely, they will stop working in future versions of JS, leading to potential breaks in your script.
Fixing deprecations essentially involves moving away from using deprecated techniques and adopting the new recommended ones. Browsers will continue to support deprecated features for a limited time frame but will eventually stop them, resulting in non-functional scripts. It requires consistent effort on the part of the developer to stay updated with latest coding standard changes and best practices.
One example could be the use of ‘var’ keyword which was the standard way to define variables in old-time JavaScript. However, post ES6 release, ‘let’ and ‘const’ keywords were introduced to provide more control over variable scope, thereby marking ‘var’ as deprecated.
var deprecatedVariable = "This is deprecated";
let newVariable = "This is the new way of declaring variables";
To ensure your work stays relevant and efficient, embracing these changes is vital. It also helps avoid unexpected bugs or issues when browsers stop supporting deprecated functionalities. As Douglas Crockford, a well-known JavaScript developer and author, said, “JavaScript is the world’s most misunderstood programming language, but JavaScript is not a defective design.” It’s constant evolution to improve itself reflects this sentiment.
For further information, the Mozilla JavaScript documentation is always a reliable source.
Don’t see deprecation as an issue, embrace it as an opportunity for improvement.