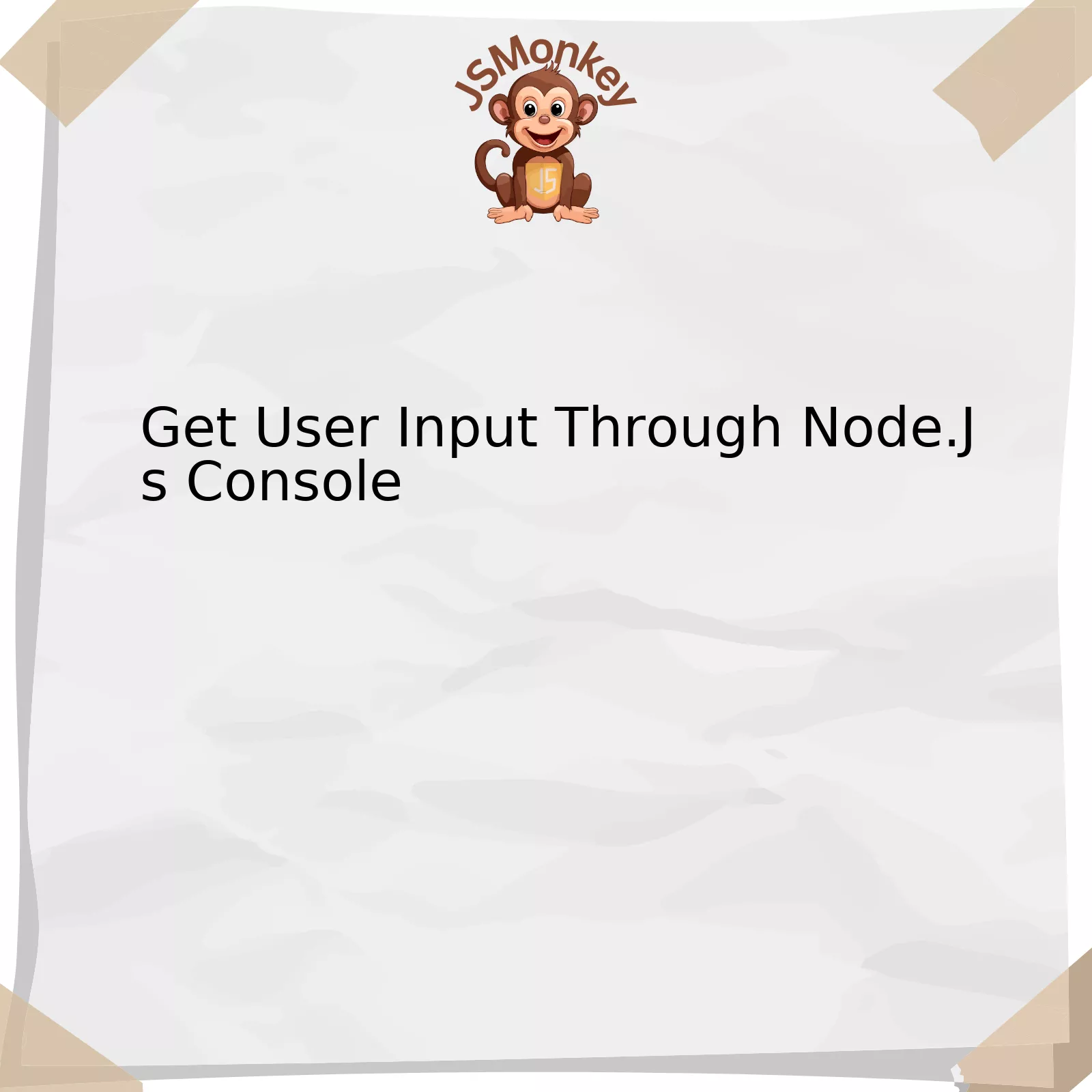
The process of gathering user input through Node.js console involves several interactive steps. Let’s illustrate these steps systematically without referencing it as an HTML table but as a structural outline:
Phase | Tools Involved | Description |
---|---|---|
Initialization |
Node.js, process object |
Launch the interaction with the user via Node.js runtime environment. Utilize the inherent ‘process’ object. |
Prompting |
console.log function |
Use the console.log method for instructing or querying the user to provide their requisite input. |
Capture |
process.stdin |
To access real-time user input and make data capturing possible, employ the ‘process.stdin’ feature. |
Data Output |
process.stdout |
‘process.stdout’ utility is employed to facilitate output, post the processing of raw user input data. |
Frequently applying this method allows user input to be fetched from the console in a Node.js application. It enables developers to write back-end scripts that can interact directly with users, increasing the flexibility and versatility of your JavaScript applications.
Initiating the application in the Node.js runtime environment, the ‘process’ object, which provides information about, and control over, the current Node.js process, is inherently used.
A console.log function is utilized to direct the user towards input by asking questions or instructing them. This function prints to stdout with newline and multiple arguments can be used with `console.log(object [, object, …])`.
Capturing of data becomes possible through ‘process.stdin’, which returns a stream connected to stdin (fd 0). It is paused by default, so one must call `process.stdin.resume()` to read from it. Note that calling `process.stdin.resume()` itself would switch the input stream into “old” mode.
On completion of the user’s input, ‘process.stdout’ writes out the processed output. Constructed from the underlying system standard output (‘stdio’) just like `process.stdin` is built over standard input (‘stdio’). `process.stdout` provides functions pertaining to a duplex stream, apart from exception for that it cannot be closed and does not emit the ‘finish’ event.
It’s noteworthy to quote Brendan Eich, the creator of JavaScript: “Always bet on JavaScript.” This holds especially when you consider this fascinating feature of Node.js that lets you interactively communicate with users right through your console. We can vividly see how JavaScript and its ecosystems have evolved over time to make web development more engaging, interactive, and effective.
Leveraging Node.Js for Effective User Input
Leveraging Node.js for retrieving effective user input is a common practice among JavaScript developers. It allows dynamic interaction with users, making web applications more engaging and user-centered.
The `readline` module in Node.js can be used to acquire user inputs directly from the console. This built-in module wraps stdin and stdout interfaces into an interface that is more simplistic and easier to use.
Here’s an example:
html
const readline = require('readline'); const rl = readline.createInterface({ input: process.stdin, output: process.stdout }); rl.question('Please enter your name: ', (answer) => { console.log(`Hello, ${answer}!`); rl.close(); });
In the aforementioned code:
– The functionality begins by requiring the `readline` module.
– An interface is set up through which Node.js will interact with the user by reading lines from stdin (standard input – typically the user’s keyboard) and writing out to stdout (standard output – usually the console window).
– By invoking the `question()` method, we pose a question to the user on the console. The user’s response is then captured as an argument to our callback function.
– Eventually, `rl.close()` is called to close the readline interface.
A famous quote from Bill Gates springs to mind on this topic – “The computer was born to solve problems that did not exist before” – and with Node.JS, we can certainly tailor how we get our user inputs in a swift and effective manner, opening the door for innovative approaches towards problem-solving.
Always remember — in programming, effectiveness relies heavily on the developer’s ability to optimize and cleanly leverage available technology and tools.
There are many comprehensive guides and tutorials on leveraging Node.js effectively like [TutorialsTeacher] to further delve into this topic. Learning and adapting to new techniques is crucial in the rapidly evolving realm of technology. Node.js is a powerful tool, and when used correctly, it can substantially enhance user interaction experiences on your web applications.
Exploring Process.stdin: The Built-in Method for User Input in Node.js
For web developers seeking to interact with users via terminal or command-line interfaces, Node.js provides a straightforward solution: the built-in Process.stdin method. This is a readable stream that represents keyboard input; it’s part of Node.js’ core process module.
If you’re wondering how this translates to getting user input via the Node.js console, keep in mind that interacting through the console is at heart an operation conducted in the terminal. For this reason, Process.stdin is a relevant resource for your cause.
Role and Functionality of Process.stdin
The first thing we need to stress about Process.stdin is its nature as a readable stream from the “process” main object. A ‘stream’ can be distilled into an abstraction of any collection of data. Streams simplify handling data, whether the source comes from the keyboard, a file, network communication or even another application.
-
stdin
: The standard input (stdin) property of “process” object brings forward data from the terminal with Process.stdin.
The implementation looks akin to:
process.stdin.setEncoding('utf8'); process.stdin.on('readable', () => { const chunk = process.stdin.read(); if (chunk !== null) { process.stdout.write(`data : ${chunk}`); }; }); process.stdin.on('end', () => { process.stdout.write('end'); });
This code effectively pushes us towards two key operations: reading from and writing to the stream, which facilitates two-way interaction via the console.
Process.stdin and User Input on Node.js Console
How does all this translate to getting user input on the Node.js console? Quite simply. Not only are streams ideal for moving large quantities of data, but they also make asynchronous I/O possible. The latter being invaluable when it comes to responsive, user interactive applications. Additionally, you can write to the console using process.stdout.write instead of console.log.
Consequently, we can infer that Process.stdin is a viable tool for:
- Developing command-line interfaces
- Building text-based games
- Making time-tracking applications…
Process.stdin gives programmers straightforward access to user input, streamlining interactivity through the console in Node.js applications.
In the words of Douglas Crockford, “JavaScript is the world’s most misunderstood programming language. But once developers understand it, they begin to see its power” (source). The more we explore features such as Process.stdin, the more versatile our JavaScript (and by extension Node.js) toolkit becomes, letting us build more dynamic and interactive applications.
Digging Deeper into Readline Module of Node.js for Enhanced User Interactions
The Readline module of Node.js is a built-in toolset that provides an interface for reading data from a readable stream, such as the process.stdin, line by line. It is this characteristic that makes it a preferred alternative when it comes to acquiring user input through the Node.js console.
const readline = require('readline').createInterface({ input: process.stdin, output: process.stdout }) readline.question('Who are you?', name=>{ console.log(`Welcome ${name}`); readline.close(); })
The above script opens the door to an interactive conversation with the user via Node.js console, asking the user’s name and then returning a welcome message.
From a higher perspective, here is what happens:
– The
readline
module is imported and an interface is set up, binding with
process.stdin
for reading in data and likewise,
process.stdout
for outputting data.
– The
readline.question()
method then puts out the question “Who are you?” waiting patiently for the user to respond.
– On receiving the input, the introduced callback function fires and the welcome message gets logged into the console including the user’s name.
– Finally, the
readline.close()
method ends the readline process.
To further comprehend the applicability of this topic, let’s consider an additional benefit of the Readline module – event-driven nature.
Driven by events, the Readline module not only allows reading of data line by line but also triggers events on every new line, or upon completion or termination of the readline instance. For example:
readline.on('line', (input) => { console.log(`Received: ${input}`); });
The given code snippet listens for ‘line’ event and every time a user inputs data finishing with the return or enter key, the ‘line’ event triggers and logs the received data.
“Code is like humor. When you have to explain it, it’s bad.” – Cory House
Remember, better user interactions lead to enriching user experience and ultimately, that’s what we are aiming for. Proper streamlining of Readline built-in Node.js library can undeniably be a tool to help achieve this objective. [1] [2].
Handling Advanced Terminal Techniques with Node.js
The prowess of Node.js in handling advanced terminal techniques is quite powerful. This logically extends to garnering user input through the Node.js console, a feature that can be impactful for developers seeking to take advantage of interactive CLI applications or scripts.
When it comes to receiving user input through the Node.js console, this process hinges upon utilizing one of Node’s core modules –
process
. The
process
module provides a way of accessing the system’s information such as environment variables, reading data from the terminal, and writing data to the terminal.
For instance, let’s consider interaction with the users directly from the command line. An example of this could be retrieving a user’s name:
process.stdin.on('data', (data) => { const name = data.toString().trim(); process.stdout.write(`Hello ${name}!\n`); });
In the above code snippet, we are listening for ‘data’ event on
process.stdin
, which gets triggered whenever a user types something on the console and presses the enter key. We then convert the Buffer data into a string, trim off any extra spaces, use that as the ‘name’, and write it back to the console through
process.stdout
.
Further expanding on this foundation, you can also handle more advanced terminal techniques such as:
- Readline Module: Node.js has a built-in readline module. It provides an interface for reading data from a readable stream (like process.stdin) one line at a time. Here’s how you could use it:
const readline = require('readline').createInterface({ input: process.stdin, output: process.stdout }); readline.question('Please enter your full name: ', name => { console.log(`Hello ${name}`); readline.close(); });
By employing the
readline
module, you can ask questions and get answers from the terminal, making it possible to have more interactive console applications.
- Commander Module: If you’re venturing into creating complex CLI tools, consider using libraries like commander. It simplifies your work by helping handle command-line options, user prompts, colorizing output, and more in a more elegant way.
“Programs must be written for people to read and only incidentally for machines to execute.” – Hal Abelson. This quote is often applicable to situations where we develop programs (like in Node.js) that rely on user interaction. Careful crafting of these interactive experiences can assist in producing code that is both user-friendly and easily understandable by other developers.
Node.js provides powerful functionality for getting user input from the console, which involves two primary tools: The JavaScript readline module and the process object.
Understanding these tools aids in programming a more interactive command line application. Through a few lines of code with the readline module, we can transform our Node.js scripts into fully interactive applications.
The
readline
module has several essential methods, like:
–
readline.createInterface()
: Creates a new readline instance
–
readline.question()
: Presents a query to the user
–
interface.close()
: Closes the underlying input stream, signaling the program that it’s done receiving input
html
var readline = require(‘readline’);
var userinput = readline.createInterface({
input: process.stdin,
output: process.stdout
});
userinput.question(‘What is your name?’, function(answer) {
console.log(Hello , + answer);
userinput.close();
});
The process object on the other hand, gives an interface connected with information and control over the current Node.js process. It helps us gather any data passed in prior to script execution – an important feature when considering customization via a command line interface.
So, acquiring user input through Node.js console essentially enhances the dynamic interaction of applications leading to more efficient software. Moreover, it enables developers to build more user-friendly interfaces. Steve Jobs once said, “Design is not just what it looks like and feels like. Design is how it works.” This principle can be directly applied to designing programs and applications that we build using Node.js. By focusing on getting user input through console, we can design applications that work in harmony with the user’s needs and expectations.
For additional insights, consider checking resources such as the official Node.js documentation.