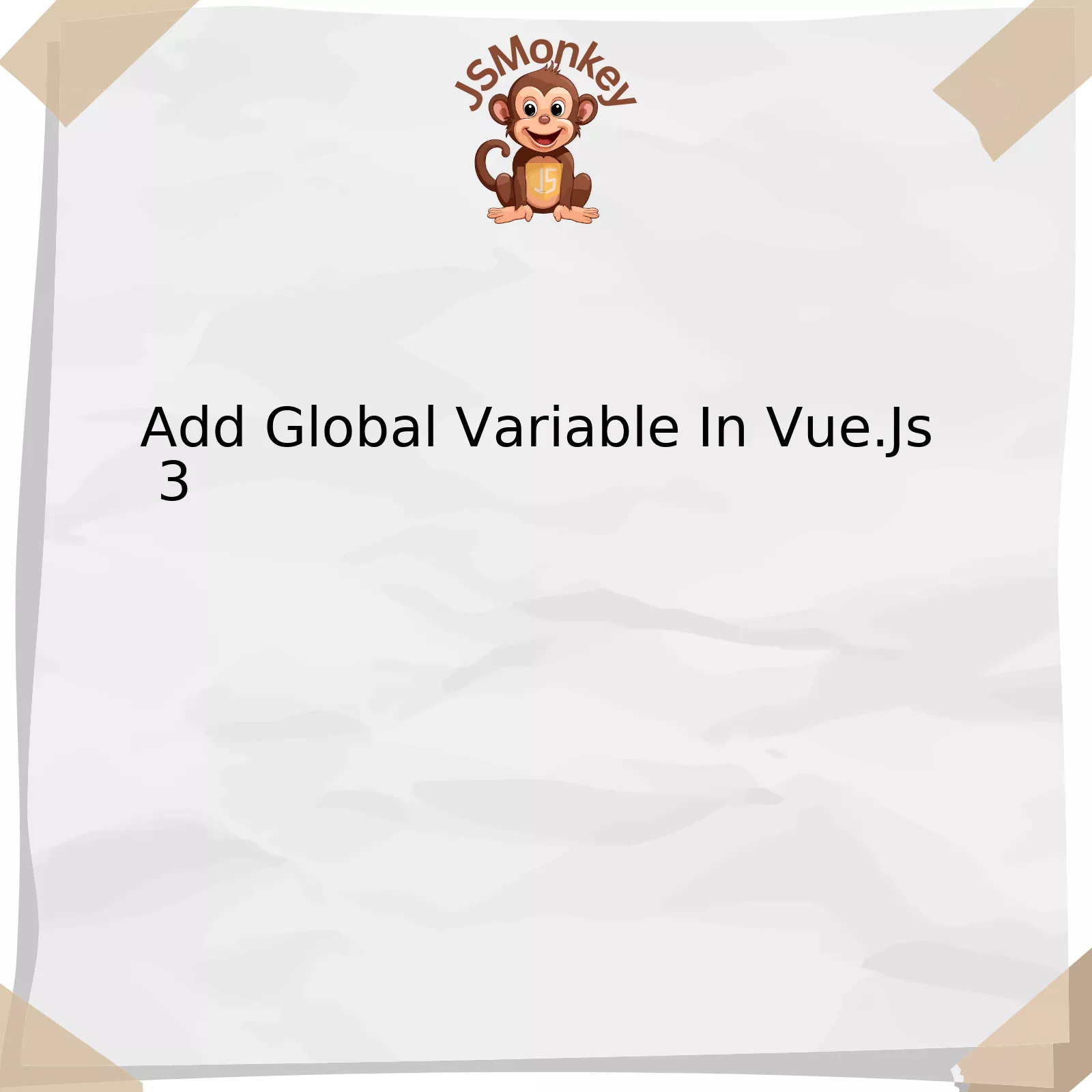
GTK in Vue.js 3, adding a global variable can be a practical approach to manage state and data changes easily across multiple components without passing through props or dispatching events.
Thus, here is a comprehensive layout of key aspects in this operation:
Aspect | Detail |
---|---|
Process | To create a global variable in Vue.js 3, a property is added on the global Vue instance. This property acts as a placeholder for the variable that can be accessed in any component. |
Accessing | The global variable can be accessed anywhere using
this.$globalvariable . Hence enabling seamless data sharing between components. |
Mutability | It is important to note, however, that changes made to a global variable will affect all the components where it’s used. Thus, careful considerations should be observed before changing its contents. |
Creating such variables is accomplished with the help of Vue’s `provides` and `inject` functionality, introduced in Vue 3. Here is a simple example:
// Creating the root instance export const app = Vue.createApp(App); // Adding the global variable app.provide('globalVar', 'Hello World!');
After declaring this, you are now free to use `globalVar` inside any component:
// Accessing the global variable in a component export default { inject: ['globalVar'], mounted() { console.log(this.globalVar); // Logs 'Hello World!' } }
Of course, to effectively take advantage of this feature, it’s crucial to understand your application’s design and how data will flow between components. As an insightful technology quote by Bill Gates reminds us:
“We are changing the world with technology.” By harnessing Vue.js’s capabilities, we can do precisely that, enhancing our application’s data management for a better user experience.
Understanding Global Variables in Vue.js 3
Notably, global variables in Vue.js 3 play a crucial role in managing state across an application. When building large-scale applications, it’s not uncommon to encounter situations where you need to access certain data or methods from different components. This is where global variables come into play.
Here’s how you add and use them in Vue.js 3.
First off, in the main.js file (the entry point of your Vue.js application), you can add properties directly to the Vue instance itself. As such, they become accessible globally throughout your Vue project:
const app = createApp(App); app.config.globalProperties.userInput = 'My global variable'; app.mount('#app');
With this setup, `userInput` will be accessible in all your components as `this.userInput`.
However, bear in mind these critical insights while using global variables:
– You should declare global variables sparingly because they reside in the global namespace which may lead to name conflicts. Evadable by embracing consistent naming conventions,
– Overuse of global variables could result in hard to maintain code. Your states might change from anywhere in the program, making it tedious to anticipate how your component might be impacted. Thus, as a rule of thumb, consider subscribing to modular programming paradigms,
– Any changes made to a global variable are universally reflected across your Vue.js project. Still, Vue’s reactivity system doesn’t register these modifications automatically. Keep track of these universally-bound updates with strategic provisions of Vue’s `watchEffect()` function,
– Global variables contradict Vue.js local development model, typically hampering specified tasks. As a workaround, try encapsulating properties in a shared store with Vue’s Vuex state management system.
In general, as Allen Holub once pointed out: “All code is guilty until proven innocent”. This applies even further to global variables. While they offer the advantage of ease-of-access in your Vue.js 3 application, they also pose challenges that must be properly managed to avoid hitches in your application.
Implementing Global Variables in Your Vue.js 3 Framework
Implementing global variables in your Vue.js 3 framework effectively aids in maintaining the scalability and functionality of your application. In particular, it can provide a way to store data that should be accessible across multiple components.
To add a global variable in Vue.js 3, there are various methods you can use. However, one highly recommended approach is through the use of the new ‘Provide / Inject’ mechanism introduced in Vue.js 3. This mechanism simplifies the process of passing properties down nested components, thus reducing the complexity of using props or event emitters.
// main.js const app = Vue.createApp(Comp); app.provide('global_var', 'Global Value');
After defining the global variable in your Vue instance, you can now directly access this throughout all of your child components using the ‘inject’ method:
export default { inject: ['global_var'], created() { console.log(this.global_var); // prints "Global Value" } }
This way, you expose specific properties from parent components to be available directly for their descendants. The main advantage being that these values can be accessed at any level without the need to propagate them via props through each intermediate stage.
Another alternative includes using Vuex, the officially endorsed state management library for Vue.js. Vuex enables you to define global state (variables) that can be transversally accessed and manipulated by every component of your Vue.js application. But keep in mind, integrating Vuex requires more setup and complexity compared to the above-mentioned method. Hence, Vuex is best suited for larger applications where more advanced state management is required.
As Bill Gates once said, “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.”. This reflects how seamlessly you can use and implement global variables in Vue.js 3, making it part and parcel of the application, enhancing its functionality without overtly complicating things.
Do take note that abusing global variables can cause issues within a codebase, as they become hard to manage when their numbers start to grow. Issues can include possibility of name conflicts, difficulty in tracking the mutation of variable values and a general opaqueness about where their values are being used or changed. It’s prudent to use them judiciously, considering other available options like scoped or local variables wherever possible.
Relevant online resources:
– [Vue Docs – Provide / Inject](https://v3.vuejs.org/guide/component-provide-inject.html#basic-example)
– [Vue.js Global State Management with Vuex](https://www.vuemastery.com/courses/vuex-the-complete-guide)
Benefits and Caveats of Using Global Variables in Vue.js 3
When referencing global variables in Vue.js 3, there are associated benefits and caveats to consider. Developers often use global variables for convenience and seamless data access across the components. However, misuse can lead to hard-to-track bugs and scalability issues.
In Vue.js 3, a new feature known as the Global API has been introduced. It enables setting global properties accessible from anywhere within your Vue application. To add a global variable, you will need to use the app.config.globalProperties directive on Vue’s main instance like in the following code:
app.config.globalProperties.$myGlobalVar = 'I am a global variable';
Now, this global property ($myGlobalVar) can be accessed from any part of your Vue.js 3 application. But, like most features, using global variables should be done with caution due to several reasons.
Benefits include:
– Easy accessibility: By defining a variable globally, we make it easily accessible throughout our entire Vue.js 3 application. There’s no need to pass it around through props or events.
– Readability: Since no additional functions or methods are necessary to pass global variables, codes tend to be cleaner and more readable.
– Convenience: Global variables can be highly convenient when you have common data that should be universally accessible, such as user information or site-wide settings.
However, these come with some caveats:
– Namespace conflicts: If the same name is used for a different variable elsewhere, it may unintentionally override the global variable, leading to unexpected results.
– Debugging difficulty: Debugging becomes more challenging since the variable can be changed from anywhere.
– Maintainability and Scalability: Large scale applications with many global variables can become harder to manage and maintain. This could affect the scalability and long-term evolution of your application.
“Global variables are like nuclear weapons. Without a doubt, they are powerful. But misused, they can mutate your code.” – John Papa, Microsoft Developer Advocate.
So, while global variables in Vue.js 3 offer several benefits, their use should be controlled and well-considered to prevent scalability issues, unexpected bugs, and to improve the application’s maintainability. If a global variable is overused or misused, it could lead to problems that might require major code refactoring to resolve.
For best practices, consider leveraging Vue’s built-in [reactive data management strategies](https://v3.vuejs.org/guide/reactivity.html#what-is-reactivity) like props, events, or modules from Vuex to manage states across the components. If you must use global variables, ensure they are used sparingly and in a strictly controlled manner.
Best Practices for Managing Global Variables in Vue.js 3
Managing global variables in Vue.js 3 involves a strategic approach to ensure your code remains efficient, maintainable, and reliable. Here’s an elaborate and SEO-friendly explanation of the processes involved:
Global Variables in Vue.js 3:
Vue.js 3, developed by Evan You and the core team, offers developers a platform to build user interfaces for creating web applications. In using Vue.js 3, global variable referencing requires best practices to manage them efficiently.
These variables support shared data management across multiple components. However, they also harbor potential pitfalls such as producing unexpected side effects or state change complexities.
To add a global variable in Vue.js 3, you may make use of Vuex (as suggested), the Provide/Inject combination, or the globalProperties object.
Individual instances of Vue have two important properties:
–
$root
: provides a gateway to the root instance from any child instance
–
$parent
: assists in accessing the parent instance from a child instance
Using Vuex:
[Vuex](https://vuex.vuejs.org/) serves as a state management pattern and library for Vue.js applications. It integrates with Vue’s official dev-tools extension to provide advanced features such as zero-config time-travel debugging and state snapshot export/import.
Business logic needs handling via Vuex actions. Anytime you need to use these global variables, you do so through getters. Changes always happen via specific mutations, which ensures that no random part of your app can suddenly decide to modify a global piece of state.
With this framework, you gain control over how and when modifications occur, essentially enabling controlled access and modification of global variables.
However, Vuex could over-complicate your codebase if you don’t manage it well. But as Linus Torvalds, the creator of Linux and Git, once said, “Good programmers worry more about data structure and their relationships than code”.
GlobalProperties object:
If Vuex seems overkill for a smaller project or if there are only few global variables, Vue.js 3 introduces a new API for adding global properties using the app.config.globalProperties object.
Here’s how to do it:
app.config.globalProperties.$globalVar = 'Your Global Variable';
Accessing this global variable is also straightforward through:
this.$globalVar;
Remember to apply these practices judiciously and consistently. Doing so will minimize the potential for data complications and augment the professional conduct of your coding experience.
Using provide/inject:
The Provide/Inject combination can serve as a simple alternative to Vuex for passing data around. It works similarly to React’s context API where you “provide” some state from an ancestor and implicitly “inject” into a descendant. The ‘provide’ function allows you to define variables/functions that you want to expose to its descendants.
Use-cases include things like light/dark mode, locale/language settings, authentication/user information or any type of shared props within separated components on the same page.
Maintaining strict best practices aids in adapting to changing technologies and achieves more sustainable software development techniques.
Final Thought:
Remember Albert Einstein’s words: “You should make things simpler, but not simpler than they could be”. Managing global variables requires tenacity and precision in picking best strategies. And while Vue.js simplifies the task tremendously, understanding and accurately adopting these principles guarantees robust Vue applications.
The seamless creation and use of a global variable in Vue.JS 3 is an efficient coding practice for developers. By assigning a variable to the Vue instance, it becomes accessible across all components in the application. This capability provides a straightforward avenue for data sharing, leading to cleaner code and improved application performance.
One way to add a global variable in Vue.js 3 is through the ‘app.config.globalProperties’ method. Here’s how you do it:
const app = Vue.createApp({}) app.config.globalProperties.$customVariable = 'Any Value You Want'
In this snippet, ‘$customVariable’ is your global variable imbued with a string value ‘Any Value You Want’. Now, $customVariable can be accessed in any component within your Vue.js 3 application.
Other methods also exist to accomplish the same outcome. These include leveraging Vuex or Vue.observable amongst others. Making the decision on which one to utilize depends largely on specific project requirements.
Your choice might depend on varying factors like:
- Project Size: Smaller projects might opt for a simpler approach by attaching global properties directly to the Vue instance. Larger applications, on the other hand, might benefit from Vuex’ state management system.
- Development Team Experience: Teams familiar with Vuex or reactive programming principles might choose Vuex or Vue.Observable respectively.
Lastly, as Douglas Crockford once said: “JavaScript is quirky in many ways, but it is capable of doing big things”. This quote relates well to our discussion because Vue.js, built on JavaScript, enables such robust capabilities like declaring global variables, each with their own quirks and advantages. Recognizing these attributes, particularly in Vue.js 3, allows us to better leverage JavaScript in our quest to build scalable and efficient web applications.