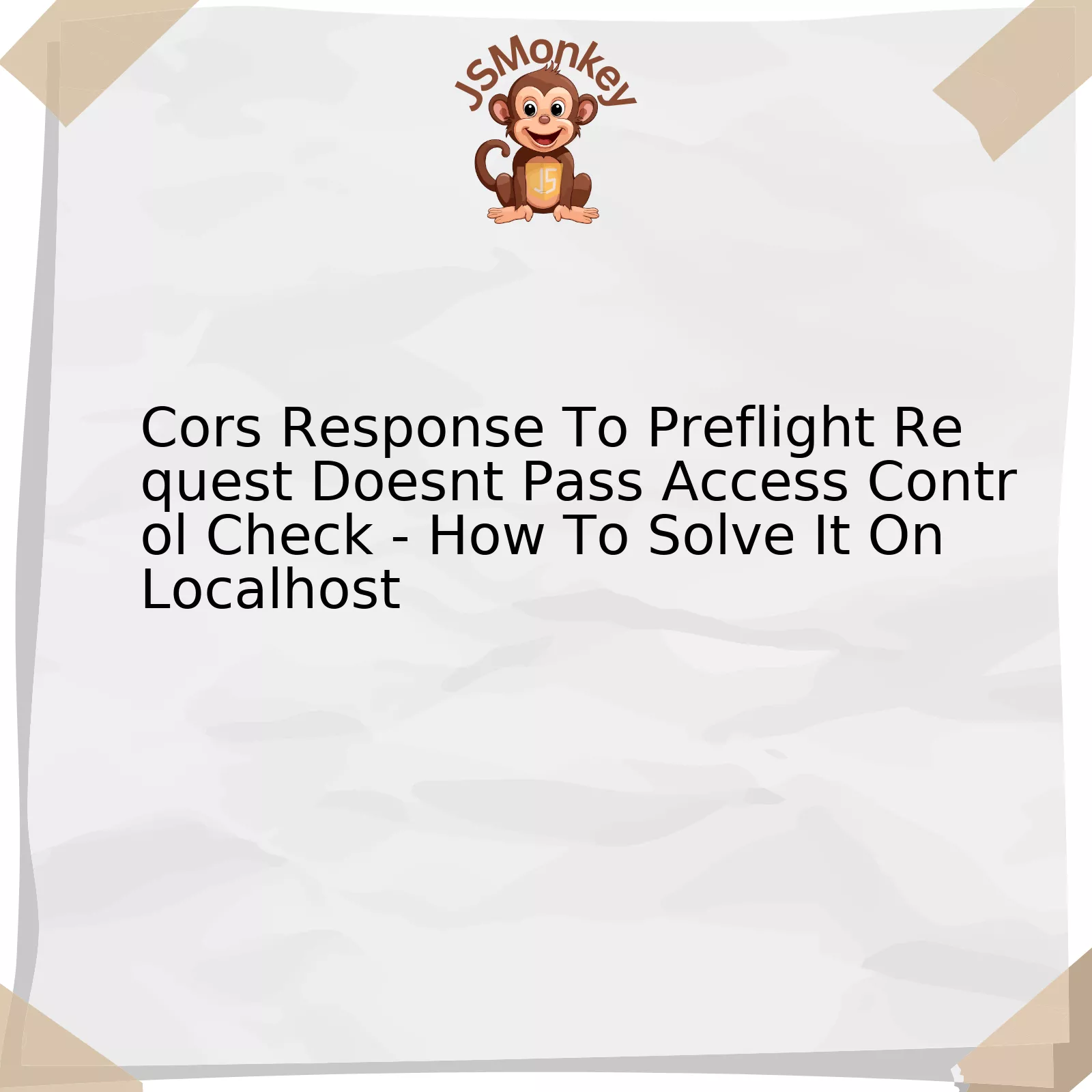
One of the common challenges encountered in web development is the
CORS response to preflight request doesn't pass access control check
error. CORS stands for Cross-Origin Resource Sharing, which is a protocol that enables scripts running on a browser client to interact with resources from a different origin. Dealing with such an issue can be daunting but don’t worry, it can be solved effectively especially if you’re working on localhost. Let’s delve into this in more depth using a structured layout.
Error Type | Description | Solution |
---|---|---|
CORS response to preflight request doesn’t pass access control check. | This error happens when the server declines a preflight request sent by the browser while trying to send an HTTP request to a different domain/origin. The cause is mainly due to the server not returning the required CORS headers in its response or returning them with incorrect values. This impedes controlled cross-origin communication between localhost and the server. | To resolve this, make sure to configure your server to include the correct CORS headers in the response, notably the
Access-Control-Allow-Origin header should match the origin of the calling JavaScript code. On localhost, you can set it to * (which allows all origins) or specifically to the origin of your localhost ( http://localhost:your_port ). The server settings will depend on the technology stack being used, so refer to the relevant documentation to get precise instructions. |
The outlined information elaborates on the nature of the “CORS response to preflight request doesn’t pass access control check” error and a viable solution to tackle it, particularly on the localhost. However, it’s crucial to note that a slight variation in configuration settings might be required based on the technology stack and server being used.
To quote Mark Zuckerberg, “The biggest risk is not taking any risk… In a world that’s changing really quickly, the only strategy that is guaranteed to fail is not taking risks.” This applies even more so in the coding world. Step out of your comfort zone, experiment with different solutions and most importantly, learn from your mistakes. With patience and persistence, mastering CORS and similar complex concepts can become second nature.
For further information and resources regarding CORS and its role in web development, this article on Mozilla MDN provides an extensive overview.
Please remember to replace ‘your_port’ with your actual localhost port number when implementing these solutions. Strictly speaking, these solutions aren’t a one-size-fits-all answer due to variances in coding language, development environments, and server configurations – hence, amending it as necessary is essential.
Troubleshooting CORS Response to Preflight Request Issues on Localhost
Troubleshooting Cross-Origin Resource Sharing (CORS) issues tends to be a common occurrence for developers as it pertains to handling requests between different origins, i.e., protocols, domains or ports. When we are considering the specific issue of
CORS response to preflight request doesn't pass access control check
, it indicates an error in handling of the CORS policy by the server receiving the request from the localhost.
Before proceeding, let’s quickly underline what is a preflight request. It’s a mechanism within the CORS protocol that checks with the server if it’s okay to send the actual request. The browser automatically sends a preflight request when:
– The HTTP verb is `PUT`, `DELETE`, `CONNECT`, `OPTIONS`, `TRACE` or `PATCH`.
– The request is `POST` and the `Content-Type` header is set to something other than `application/x-www-form-urlencoded`, `multipart/form-data` or `text/plain`.
Now let’s look at how to solve this issue step by step. This detailed analysis aimed at solving this situation includes ++implementing appropriate headers++ and ++active use of middleware++ like express.js
When a request runs afoul of the CORS policy in your localhost environment, one major reason can be the missing access control headers in the response. These headers provide information for the client about which requests are allowed.
Implementing Appropriate Headers: A straightforward method is to configure the server so that it responds with correct CORS headers. You can add the following response headers:
html
Access-Control-Allow-Origin: http://localhost:xxxx
Access-Control-Allow-Methods: POST, GET, OPTIONS, DELETE
Access-Control-Allow-Headers: Content-Type
Access-Control-Allow-Credentials: true
This would allow requests from your localhost on specified port (replace xxxx with your localhost port) and permit certain methods like `GET`, `POST`, `OPTIONS`, and `DELETE`. Adding the `Access-Control-Allow-Credentials` header with a value of `true` allows requests to be made using cookies, authorization headers or TLS client certificates.
Middleware Use: If you’re using Node.js with Express, one way to solve this issue is by making use of middleware packages such as `cors`. Here’s an example of how to implement it:
html
const cors = require(‘cors’)
app.use(cors())
The `app.use(cors())` middleware enables all CORS requests. However, for security reasons, we are advised not to leave our server open to all origins in production environments. We would therefore adjust the configuration in a production environment to specify which origins are permitted.
Careful consideration and proper implementation of these steps can help mitigate issues regarding
CORS response to preflight request doesn't pass access control check
. Still, always consider your application’s requirements when setting up your CORS policy.source
As Steve Jobs once articulated, “Design is not just what it looks like and feels like. Design is how it works”. This sentiment certainly rings true when dealing with intricate server-to-client policy design, as reflected by CORS implementation. Accurate coding practices – much like effective design – can result in systems that “just work”, improving efficiency, interoperability, and user experience.source
Understanding and Addressing “Access Control Check” Errors
When working with web applications in a local environment, it is quite common to run into Cross-Origin Resource Sharing (CORS) errors such as “Access-Control-Allow-Origin” or “The CORS response to preflight request doesn’t pass access control checks”. To tackle these errors effectively, one needs to understand both the reason behind the occurrence and ways to address them adequately.
Let’s begin by examining the roots of these errors. They are primarily triggered because browsers enforce a policy known as the “Same-Origin Policy”, which restricts how a document or script loaded from one origin can interact with a resource from another origin. This is crucial for security purposes: it prevents malicious scripts on one page from obtaining access to sensitive data on another web page.
However, the Same-Origin Policy would also severely limit the potential and capabilities of the web if taken in absolute terms. Consequently, we have CORS – a mechanism that enables many cross-origin requests, while still providing the possibility for servers to specify who can access their assets. It operates based on HTTP headers that indicate which origins are permitted.
“The key to cracking CORS-related errors lies within its nature itself – understanding how to manipulate its HTTP headers.” – John Resig, American software engineer and creator of jQuery.
This brings us into addressing those dreaded CORS errors:
1. Running the project over HTTPS: Security features like CORS are often relaxed when both requestor and requested resources communicate over a secure handshake (HTTPS). If you configure your local server to use HTTPS, this problem might just disappear.
2. Utilize a proxy service: Essentially, make a request to your own server (which will not be restricted due to being the same origin), and let your server forward that request to its actual destination.
3. Configure your Server Correctly: The optimal solution is configuring your server properly. Upon receiving preflight requests (HTTP OPTIONS method), your server should respond with appropriate CORS related headers. Those like
Access-Control-Allow-Origin
,
Access-Control-Allow-Methods
, and
Access-Control-Allow-Headers
.
For instance, to allow all domains:
html
Access-Control-Allow-Origin: *
Note: Replace ‘*’ with a specific URL or URLs to limit accessibility strictly to those URLs.
4. Middleware tool for local testing: There are middleware tools available like `cors-anywhere` which can be added to your local server while you’re developing and testing. This reduces time troubleshooting CORS error in the local development environment and focuses more on actual code development.
5. Utilizing browser extensions: There are many browser extensions, such as ‘MOESIF ORIGIN & CORS CHANGER’, that will allow CORS requests sending from your local browser.
Remember: While these solutions should take care of CORS issues, use them judiciously. Loosening CORS security could expose your application to various potential attacks if used in production environments. It’s good practice to keep these development workarounds separate from your production code.
Further Readings:
MDN – Cross-Origin Resource Sharing. A comprehensive read about CORS that includes detailing preflight requests.
W3C – CORS. Details about the official CORS specification.
Implementing Solutions for CORS Response Access Issues on Localhost
Cross-origin resource sharing (CORS) is a mechanism that allows many resources (e.g., fonts, JavaScript, etc.) on a webpage to be requested from another domain outside the domain from which the resource originated. It’s a handy feature for service integration, but it can sometimes lead to access control issues. Understanding and resolving such problems could make the development process much easier, especially on local servers.
Let’s delve into the common CORS issue “Response to preflight request doesn’t pass access control check,” and the steps you can perform on your localhost server to fix this.
Understanding Preflight Requests
Preflight requests are sent before the actual request to check the server’s CORS policy if it’s safe to send the real request or not. Browsers automatically make this decision based on certain criteria, like:
- If the request method is `GET`, `HEAD` or `POST`.
- If the `Content-Type` header has been set to application/x-www-form-urlencoded, multipart/form-data, or text/plain.
For instance, requests that don’t meet these criteria, browsers send a preflight OPTIONS request. If the server responds affirmatively and approves, only then the browser sends the actual request.
CORS Response to Preflight Request Doesn’t Pass Access Control Check:
If you see an error in your console saying “CORS response to preflight request doesn’t pass access control check”, it means that the web page has tried to access a response for cross-origin resource sharing (CORS) request that the server did not allow.
In most cases, this happens when trying to consume APIs or any other web services from different domains. The server might not be configured to accept requests from your localhost.
Below is an example of what the error message may look like:
Error: Request header field Content-Type is not allowed by Access-Control-Allow-Headers in preflight response.
How to Solve it on local Host?
You can opt for several methods to solve this issue on your localhost server depending on the scripting language and server you are using.
Allow All Origins:
One of the first things you can do is to modify your server configuration to allow all origins by adding ‘Access-Control-Allow-Origin’ as ‘*’ in the HTTP headers. But remember, this should only be done during development as it could pose a security risk if done in a production environment. For example:
Access-Control-Allow-Origin: *
Specify Localhost:
To mitigate potential risks, you can explicitly specify which origin is allowed. Here, we’re granting access only to our local server at port 8080:
Access-Control-Allow-Origin: http://localhost:8080
Setting Server Headers:
For Node.js with Express server, for instance, you can choose to use CORS middleware package that simplifies the process of setting CORS headers:
var express = require('express') var cors = require('cors') var app = express() app.use(cors()) // Your routes go here
Remember, most of these solutions involve changing configurations on the server. Thus, proper testing and ensuring a secure production environment should always be maintained.
As Jeff Atwood, co-founder of StackOverflow, said, “You’re responsible for making sure your code works—it’s not some QA person’s job, and not your customers’ job, either.”
For more in-depth information, visit MDN web docs.
Advanced Techniques in Resolving Preflight Request Challenges
Cross-Origin Resource Sharing (CORS) is a security measure enacted by Web Browsers to prevent access to resources residing in a domain different from the requesting source. To cater to this, HTTP responses support an
Access-Control-Allow-Origin
header that specifies which origins are allowed.
When testing on your localhost, you might encounter a CORS preflight error message such as: “Response to preflight request doesn’t pass access control check.” This error occurs when the server’s response does not agree with the permissions configured within the CORS header.
To solve this problem, let’s explore various advanced techniques:
Serving Appropriate Response Headers
Built-in Express.js middleware can help handle CORS issues. Where
app
is your express app instance, just before defining your routes, drop in:
app.use(cors())
This will add correct CORS headers to your responses, allowing all origins.
Allow Preflight Caching
Additionally, reducing preflight requests via preflight caching helps to put unnecessary traffic under check. Achieve this by setting
Access-Control-Max-Age
on your server.
Localhost Proxy
Another intriguing technique involves creating a proxy server on your localhost. Packages like http-proxy-middleware on npm can be quite handy for generating proxy middleware, and its setup combines well with most front-end development servers such as Webpack Dev Server or BrowserSync.
As Bill Gates once said, “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.” The same applies to resolving preflight request challenges – these solutions integrate seamlessly into your codebase, ensuring that server response passes the access control check more efficiently and unnoticeably.
NOTE: Because each approach alters server configuration, always ensure that changes are in harmony with your app’s security requirements. When resolving preflight request issues locally, remember to adjust configurations as required when deploying to a different environment.
The Cross-Origin Resource Sharing (CORS) mechanism can sometimes give developers headaches when they encounter the problem of failure to pass access control checks during preflight requests, especially on localhost. This issue primarily arises due to deficient backend configuration that inadvertently disallows communication with a different origin.
Examining the root cause of this problem, it is necessary to understand the role CORS plays in web security. Most importantly, it serves as a safeguard against potential attacks by controlling who can interact with resources on a web page. When your browser makes a request that necessitates a preflight check, and the response from the server lacks the required ‘Access-Control-Allow-Origin’ header or isn’t configured correctly, the browser denies access with a CORS error.
Here’s how you solve this on localhost:
app.use(function(req, res, next) { res.header("Access-Control-Allow-Origin", "*"); res.header("Access-Control-Allow-Methods", "GET, PUT, POST, DELETE"); res.header("Access-Control-Allow-Headers", "Content-Type"); next(); });
This segment of code signifies setting up middleware that adds the required ‘Access-Control-Allow-‘ headers to every incoming request. As such, your server welcomes any cross-origin request and fits constant interaction scenarios while development on localhost.
To stay more secure during production, replace ‘*’ with your production site’s URL:
res.header("Access-Control-Allow-Origin", "http://your-web-url.com");
Furthermore, remember to allow all needed HTTP methods through ‘Access-Control-Allow-Methods’.
Lastly, we can’t ignore the advice of Ward Cunningham, the developer of the first wiki. He said, “You know you are working on clean code when each routine you read turns out to be pretty much what you expected.” Just like that, a smooth development process ensures efficiency and productivity.
These alterations should typically rectify the CORS response issues during localhost development. Apply these adjustments cautiously to ensure you enhance, rather than risk web security.
When all is said and done, remember that the CORS policy exists for a reason. Even though it may seem like an inconvenience during localhost development, manipulating it irresponsibly in a live environment can expose your platform to risks. Ensure all changes respect the essence of Web Security.
For further information on handling CORS, visit the MDN Web Docs.