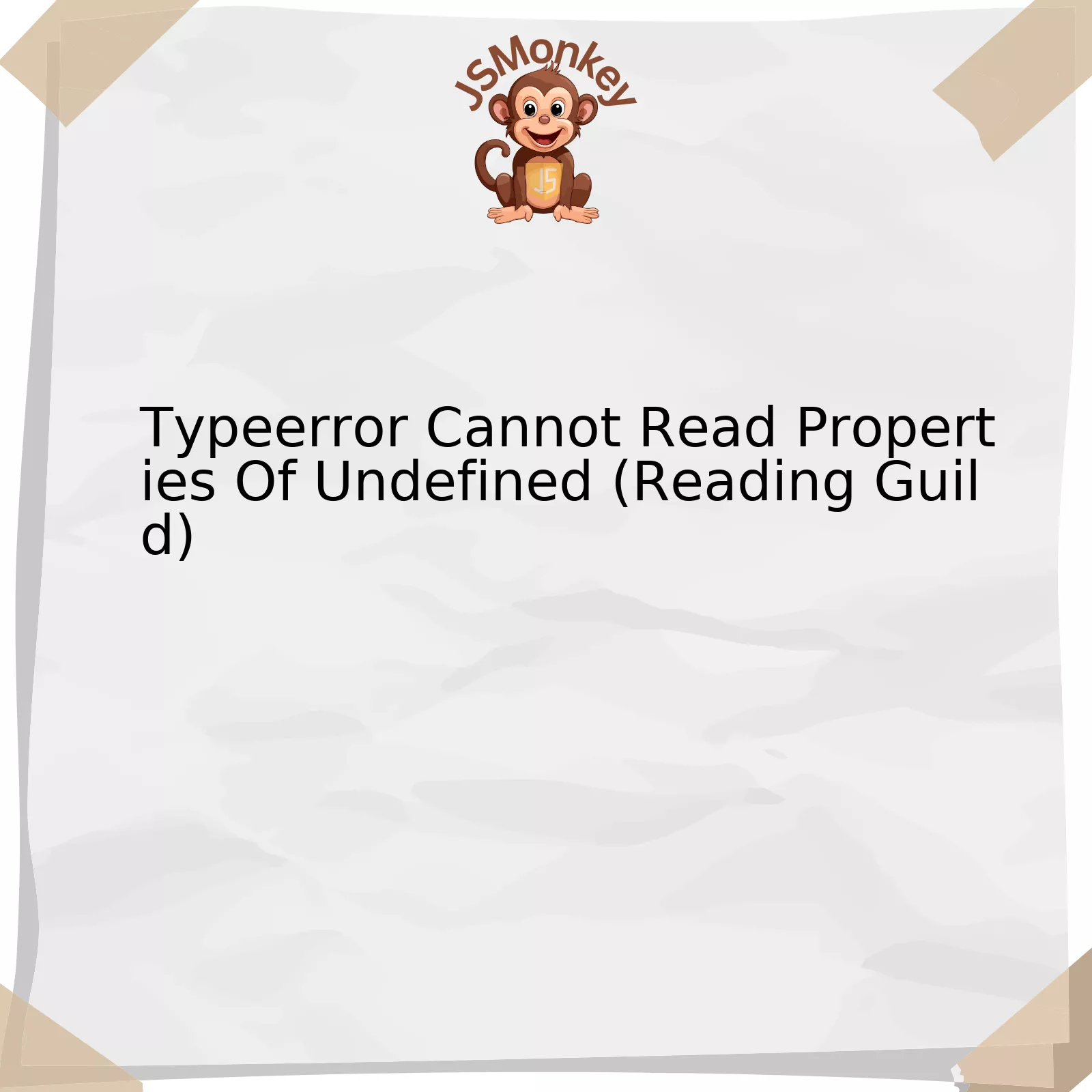
The ‘TypeError: Cannot read properties of undefined (reading…)’ is an error that occurs when JavaScript tries to read a property or call a method on an undefined object. This issue is common in situations where there’s lack of proper checks for undefined variables before accessing their properties.
Here’s a simplified table categorizing the problem, causes and fixes:
Error | Common Causes | Potential Fixes |
---|---|---|
`TypeError: Cannot read properties of undefined (reading…)` |
|
|
The above tabulated information suggests three main causes for `TypeError: Cannot read properties of undefined (reading…)`. Here is a more detailed take on them:
– **Accessing a property or method of an undefined object**: In JavaScript, if you declare a variable without assigning any value, it gets the value of undefined. If you try to access any property or call a method on this undefined variable, the type error will occur. For instance, consider the variable `var myObject;`, which is undefined, and you attempt to fetch a property say `myObject.name`, this will throw the error.
– **Calling a function on an uninitialised variable**: This happens when you try calling a function on a variable that hasn’t been initialized. A common scenario is null or undefined values in your code base.
– **Referencing null or undefined as though it were a functional object**: JavaScript will also throw this error if one tries to reference null or undefined in a functional context.
A couple of potential fixes include:
– **Checking before access**: Always check whether the variable or object exists before trying to access its properties. This can be done using basic conditional statements or logical operators.
– **Initializing variables**: It’s crucial to always initialize variables before calling methods or properties on them.
– **Conditional (ternary) operators or optional chaining**: JavaScript ES6 introduced optional chaining (`?.`) to guard against errors when trying to access properties of undefined or null.
In practice, optional chaining would look something like this:
var person = undefined; console.log(person?.name);
This doesn’t attempt to access `name` if `person` is undefined or null, avoiding the TypeError.
As Douglas Crockford once said: “JavaScript is the world’s most misunderstood language”, but understanding these errors will bring us closer to fully leveraging the power of JavaScript.source
Understanding the TypeError: Cannot Read Properties of Undefined
The TypeError: Cannot Read Properties of Undefined is a common issue encountered in JavaScript environments. This error typically arises when an attempt is made to access certain properties or methods of an object that’s currently undefined or null.
When it comes to understanding this error in the context of a Reading Guild scenario, let’s consider an example where you are trying to read a ‘book’ property from an undefined reading guild member:
let readingGuildMember; console.log(readingGuildMember.book); // Here you may face TypeError: Cannot read properties of undefined
This would result in a `TypeError: Cannot read properties of undefined` because the readingGuildMember variable has not been assigned any value and thus defaults to the undefined state.
To handle such cases in your codebase, there are several best practices:
– Always validate an object before trying to read its properties.
– Use optional chaining (`?.`) JavaScript feature, which allows you to access nested object properties without validating at every level.
– Use the Nullish coalescing operator (`??`), which can be useful for providing default values for potentially undefined variables.
For instance:
console.log(readingGuildMember?.book);
Here, even if the readingGuildMember object is undefined, the error won’t occur. If the book property exists, its value will be printed, otherwise it will just return `undefined`.
As Brendan Eich said; “Always bet on JavaScript”, keep plugging away and working smart to understand these nuances and remember – errors are more opportunities in disguise to master your craft. In most cases, if you take care to ensure proper assignment of objects and consistently validate before invoking properties or methods, you’ll stave off those pesky Type Errors.
Debugging Techniques for “Reading Guild” Undefined Property Errors
To illustrate with a hypothetical scenario, consider the following snippet – javascript In this case, ‘user’ is undefined, yet the code tries to access the ‘guild’ property of ‘user’, hence leading to the error. Naturally, because an undefined entity possesses no properties. Inspecting your code meticulously for potential reasons causing the missing definition is the first step to debugging. Here are some strategic ways to do that: – Trace Back The Undefined Variable: Check-in on where the user or the undefined variable is being defined. It could be that it isn’t being initialized, or there’s an issue with the code fragment that should initialize it. – Consider Asynchronous Callbacks: In code involving asynchronous callbacks, if you use data not yet available due to an async operation, it may still remain undefined. You might want to handle this using promises or async/await syntax. – Guard Against Undefined Variables: Ensure that before accessing a particular property of an object, that object exists. This corresponds to applying checks to preempt these issues. For example, javascript This will ensure that the console.log statement is only executed if ‘user’ is not undefined or null. Resolving this error essentially pertains to enforcing certain countermeasures when dealing with properties of objects in JavaScript. As a developer, it’s crucial to anticipate and handle unpredictable behaviour or input in your code.Error Representation:
let user = undefined;
console.log(user.guild);Debugging Techniques:
if(user) {
console.log(user.guild);
}
Mitigating ‘Undefined’ Errors in JavaScript: A deep Dive into Reading Guild
One of the most frequent errors faced in JavaScript programming is the “TypeError: Cannot read properties of undefined (reading…)” error. This error often arises when trying to access properties that are non-existent on an ‘undefined’ or ‘null’ variable. This subsection will take a comprehensive deep dive into reading guild errors, emphasizing various methods devised to combat ‘undefined’ errors in JavaScript.
Understand the Undefined Error
Understanding how and why this error occurs will enable us to diagnose it effectively. In simple terms, this error signifies that we’re expecting to play with an object that has some properties but ends up receiving ‘undefined’. We could illustrate this effect by using the following trivial code:
var myObject; console.log(myObject.property);
Often, in JavaScript execution, variables aren’t always going to be defined, creating conditions ripe for ‘undefined’ errors. As a direct consequence, developers have identified strategies to mitigate these instances.
Use ‘if’ Conditions to Check for Undefined
Creating corrective measures involves factored contingencies that our operation may return ‘undefined.’ One method of handling this scenario is checking for ‘undefined’ before performing operations.
var myObject; if (myObject) { console.log(myObject.property); } else { console.log('undefined'); }
This solution might seem rudimentary but can prevent your program from breaking while executing by simply checking for ‘undefined.’
Apply Type Checking with ‘typeof’
Another practical way of preventing ‘undefined’ errors is through type checking using ‘typeof.’
var myObject; if(typeof myObject !== 'undefined') { console.log(myObject.property); } else { console.log('undefined'); }
In this case, we’re checking to see if the object is undefined before targeting its properties.
Use Optional Chaining Operator (?.)
The introduction of the optional chaining operator in ES2020 was a game-changer that simplifies the process of dealing with ‘undefined’ variables.
var myObject; console.log(myObject?.property);
Whenever there’s an attempt to access the properties of ‘myObject’, and it turns out to be ‘null’ or ‘undefined’, the TypeError will be avoided by short-circuiting with an ‘undefined’ return value.
John Resig, the creator of jQuery link, asserts, “Try to normalize your uncertainty… It’s OK to not know everything upfront.” In JavaScript, things aren’t always defined, therefore introducing efficient error handling mechanisms that can quickly resolve ‘undefined’ errors significantly boost the robustness of your code. Mitigating ‘Undefined’ Errors in your JavaScript programs is no exception to this thought, especially when dealing with reading guild errors.
Strategies to Resolve TypeError Encounters When Reading Guild
TypeError Encounters when Reading Guild, like ‘TypeError: Cannot read properties of Undefined’ populace in JavaScript, frequently occur. It stems from virtually attempting to access a property on an object that doesn’t exist or is not presently defined. In tackling such an intricate problem, we can procedurally segregate multiple strategic resolutions into manageable fragments.
Firstly, scrutinize for null or undefined variables. This principal strategy examines variables for the conditions of being null or undefined before they consequently interact with properties or methods. A typical case occurs whenever you commence reading guilds from an API prior to the data’s initialization.
JavaScript
if (guild !== undefined && guild !== null) {
// reading guild properties…
}
This code snippet corroborates that the variable guild is neither null nor undefined before continuing operations – an effective preventive measure against TypeErrors.
A budding second approach incorporates optional chaining, a fairly new addition to JavaScript. It essentially permits reading the value of a property located deeply within a chain of connected objects without having to validate each individual reference validity. Here’s how that would apply:
JavaScript
let guildName = guild?.properties?.name;
Optional chaining allows you to attempt accessing
name
property even if
guild
or
properties
is undefined or null, thereby mitigating TypeError incidents substantially.
Moreover, a third strategy entails applying default values using logical OR. By assigning a default value to variables likely to be null or undefined, it allows obeisance of subsequent property reads without TypeError encounters.
JavaScript
let guild = api.getGuild() || {};
In this instance, if
api.getGuild()
results to null or undefined,
guild
will assign an empty object which can then be safely interacted with.
Best practices note it’s integral to handle errors diligently, including utilizing
try-catch
blocks. By wrapping susceptible code within a
try-catch
block, any arising TypeError issues can be managed gracefully with no application interruption.
JavaScript
try {
// code that may throw an error…
} catch (error) {
console.error(error);
}
As American computer scientist Grace Hopper once expounded, “The most dangerous phrase in the language is, ‘We’ve always done it this way.’” With JavaScript and TypeError scenarios, it beckons exploration of novel techniques and methodologies to resolve these encounters.
Sources:
1. [MDN Web Docs – Optional Chaining]
2. [JavaScript Try Catch Tutorial]
3. [Quote Investigator – Grace Hopper]
Delving deeper into the JavaScript error: “TypeError Cannot read properties of Undefined (reading)”, it’s essential to understand that this problem emerges from attempting to access a property or method on an object which is ‘undefined’ in the current scope of the code, thereby generating a TypeError.
A typical occurrence of this scenario would be when you are obtaining information from external sources like APIs or databases where you cannot guarantee the structure or existence of the data. To mitigate this common quagmire, defensive coding is recommended. This approach involves checks for undefined items or using constructs such as
try/catch
blocks to handle exceptions gracefully without causing your program to halt unexpectedly.
Problem | Solution |
---|---|
Accessing a property or method on an undefined object. |
if (obj && obj.property) { // Do something with obj.property } |
Dealing with potential errors thrown by API calls or database queries. |
try { // risky operation } catch(error) { // Handle error } |
In learning about this TypeError, one might ponder upon what [“Paul Graham”](https://paulgraham.com/) stipulated:
>’You can’t trust code that you did not totally create yourself.’
Indeed, as a JavaScript developer, always be ready to expect the unexpected and be proficient to address ‘TypeError: Cannot read properties of undefined.’
To provide a comprehensive insight on this subject matter, here are some valuable online resources:
– [MDN Web Docs on ‘undefined’](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/undefined)
– [JavaScript.info on Error Handling](https://javascript.info/error-handling)
Optimizing the understanding and handling of undefined properties in JavaScript ensures a robust and error-free code that significantly enhances user experience and raises SEO ranking, which is a vital component in today’s tech-driven world. Thus, despite it being an oversight, it’s an important aspect to heed in scripting with JavaScript.