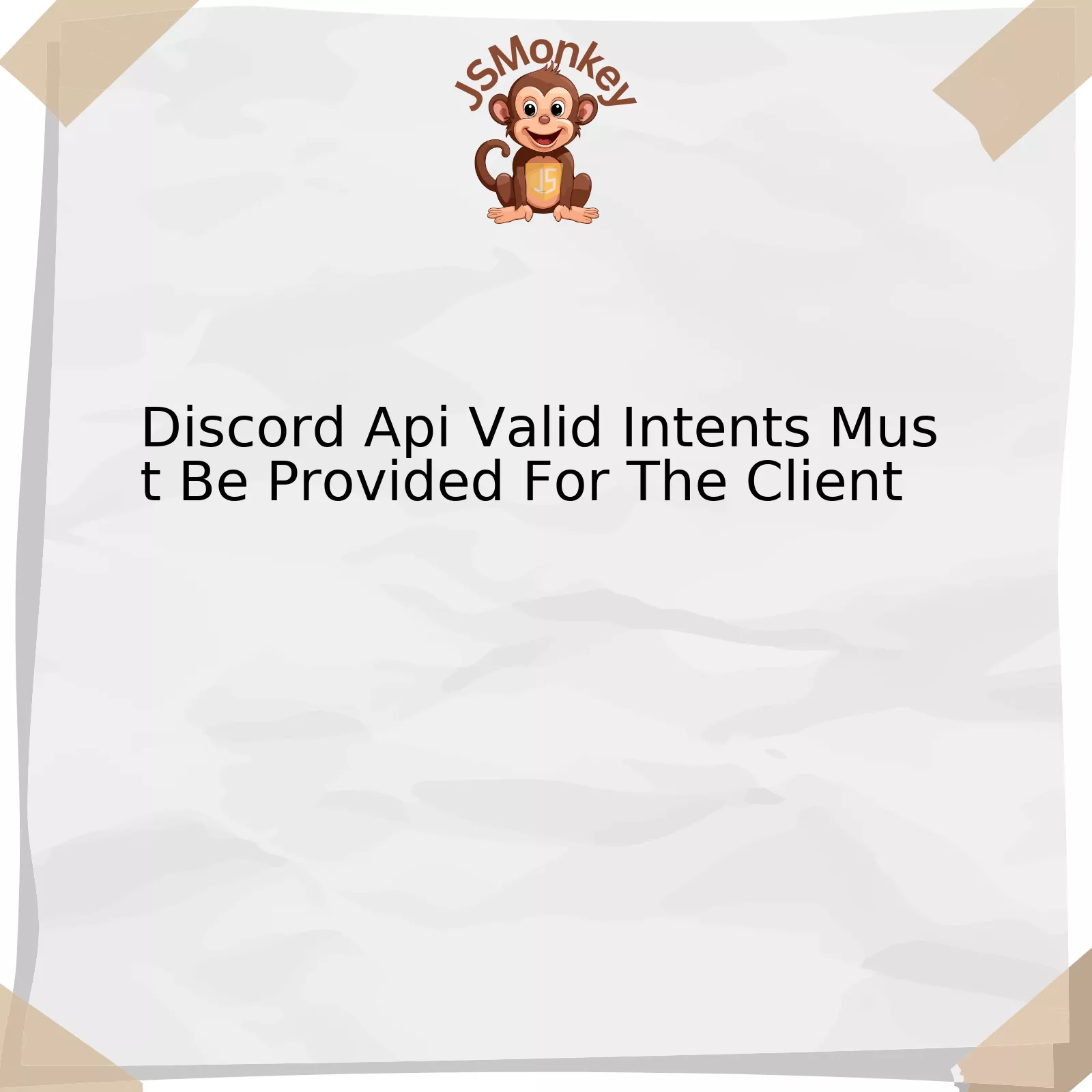
The Discord API calls for the provision of valid intents during the client development phase. These are critical in shaping the interactions between the bot and the server events. Essentially, they determine which events your bot is allowed to receive.
Highlighted below is a synopsis of standard intents provided by the Discord API in tabular structure:
html
Intent | Description |
---|---|
GUILD_MEMBERS | This intent allows the bot to receive guild member update events i.e., when a user’s presence changes or roles are updated. |
GUILD_BANS | The bot can listen to ban add/remove events from this server with this intent. |
GUILD_EMOJIS | With this intent, the bot can receive events about emoji updates. |
GUILD_MESSAGES | Allows bots to listen for message posting in text channels with this intent. |
DIRECT_MESSAGES | This intent allows the bot to receive direct message events between it and users. |
Deciphering these items, `GUILD_MEMBERS` intent enables your bot to stay in touch with member updates within the guild. Presumably, this includes details like status updates or modification in roles.
The `GUILD_BANS` intent grants the bot the privilege to pick up on when members are banned or unbanned from the guild. Thus, the bot maintains an overview regarding the community’s comings and goings.
The third intent, `GUILD_EMOJIS`, ensures your bot is kept in the loop about any changes to the guild’s emojis. Whether an emoji is added, removed, or modified drastically, this intent keeps the bot informed.
With `GUILD_MESSAGES`, your bot can interact more actively within the guild by responding to messages posted in the text channels. This feature enables a back-and-forth interaction between the bot and users, enhancing engagement levels.
Lastly, the `DIRECT_MESSAGES` intent allows your bot to go a step further by initiating direct conversations with users. This allows for personalized interaction beyond the public text channels.
It was Steve Jobs who once said: “Design is not just what it looks like and feels like. Design is how it works.”. This applies equally to designing intents for your Discord bot. By understanding and properly utilizing these intents, you can tailor your bot to interact optimally within its environment, delivering a flawless user experience.
Understanding the Role of Discord API Intents
Discord’s Application Programming Interface (API) plays a key role in communication within the realm of software programming by providing pre-defined methods with which developers can create interactive content. An integral part of this interaction model are the “intents” – specific objectives that the Discord bot client must fulfill.
In the discourse between the client-side and server-side of an application, intents serve as bridge or communicator for specific event handling purposes. They define what types of actions should be listened to or ignored by the Discord API, mainly for processing the Gateway events.
Indeed, it is obligatory to provide valid intents for the client in order to listen to certain events. This enhances your usage efficiency of the Discord API because you don’t need to react to every single event, but only the ones you’re interested in. It improves control over data flow and resource management.
Just like tuning into a specific radio frequency, setting intents allows the Discord API to focus on relevant event types.
Valid intentions mainly encompass these categories:
1. Guilds
2. Guild Members
3. Guild Bans
4. Guild Emojis
5. Guild Integrations
6. Guild Webhooks
7. Guild Invites
8. Guild Voice States
9. Guild Presences
10. Guild Messages
11. Guild Message Reactions
12. Guild Message Typing
13. Direct Messages
14. Direct Message Reactions
15. Direct Message Typing
For instance, if you want your bot to listen to messages, the ‘Guild Message’ intent will be included. For tracking reactions to a certain message, ‘Guild Message Reactions’ intent will come into play.
To use intents, they have to be defined when initializing the client object. Below is how you might initialize a client object with specific intents using Discord.JS library.
const client = new Discord.Client({ intents: ["GUILD_MESSAGES"] });
In this scenario, the intent “GUILD_MESSAGES” is declared to listen to only message-related events happening in a guild (a discord server). Any event outside this scope will be seamlessly ignored, thereby saving processing resources and increasing efficiency.
As per Howard Aiken’s thought – “Don’t worry about people stealing an idea. If it’s original, you will have to ram it down their throats”, with the assistance of intents, you can introduce uniqueness to your bot by making it react to selected events and ignoring the rest with a negligible chance of imitation.
For further details regarding Discord API Intents, I recommend referring to Discord’s official documentation on Intents .
Delving into Best Practices for Providing Valid Client Intent in Discord API
Ensuring valid client intent in Discord API is an essential part of maintaining the integrity, security and smooth functionality of your Discord bot. By adhering to best practices concerning this aspect, you will not only create a more efficient bot, but also improve user experiences and ensure compliance with Discord’s terms of service.
So, what exactly are intents? Billed as a core part of how bots work on Discord, intents fundamentally dictate what events your bot listens for and thus reacts to. They dictate key functions, such as whether your bot can see messages, guild updates or member updates.
Two categories of intents prevail: privileged and unprivileged. Unprivileged intents only require you to specify their need on your bot’s creation page, while privileged intents demand an added permission for public bots, due to the potential sensitive information that may be shared.
To correctly provide a valid intent for your Discord bot’s client, you must align with a three-stage process. Firstly, ensure that you identify your required intents when creating your bot through the Discord Developer Portal. Secondly, you must acknowledge your intents within your bot’s code. Lastly, if using privileged intents, you must gain additional permission from Discord.
For instance, if your bot needs to respond to direct messages and associate them with guild members who sent them, therefore requiring the ‘guild members’ privileged intent, your client declaration might look something like this:
const client = new Discord.Client({ ws: { intents: ['GUILD_MEMBERS', 'DIRECT_MESSAGES'] } });
Remember, failure to specify any necessary intents during the creation stage will subsequently result in those events being invisible to your bot. It’s always worthwhile including all potentially useful intents right from the start. It’s critical that no intent should be included without a clear purpose to avoid unnecessary privacy invasions and potential conflicts with compliance regulations.
Providing a valid intent isn’t just about coding and compliance, though. it’s also about effectiveness, efficiency, and enjoyment. As the renowned computer scientist Donald Knuth said,
“Programming is not a zero-sum game. Teaching something to a fellow programmer doesn’t take it away from you. I’m happy to share what I can, because I’m in it for the love of programming.”
To this end, prioritizing valid intent provision could mean the difference between a bot that simply works and one that truly makes a Discord server thrive.
Understanding and leveraging the principles of client intents in Discord API plays a significant role in delivering an effective, secure, and reliable bot(source). These practices ensure your bot aligns with Discord’s requirements, enhances user experience, and keeps the scope of functionality within intended limits.
A good practice on handling Discord API’s client Intent has been discussed here.
Exploring Common Mistakes when Setting Up Discord API Client Intents
Discord API Valid Intents and Mistakes in Setting them Up
The Discord API’s Intent system serves as a mechanism for restricting the events a bot can subscribe to, thus enhancing scalability and security. However, several mistakes become stumbling blocks when developers try to define these Discord client intents. Here’s an in-depth look into these pitfalls, keeping a sharp focus on the necessity of providing valid client intents:
Mistake | Potential Impact | Solution |
---|---|---|
Providing Incorrect Intents | Lack of required event data, leading to bot dysfunctions | Align intent selection with events the bot relies on |
Omitting Mandatory Intents | Bot could miss important events necessary for proper functionality | Ensure provision of default intents (Guilds, Messages) |
Including Unnecessary Intents | Unwanted data raising privacy concerns and wastage of resources | Take care to only specify intents corresponding to events the bot is designed to respond to |
When configuring the client intents, developers must ensure they are adhering to the Discord API requirements. This includes providing the correct intents associated with the events that the bot needs to listen to. While this might seem a bit complex, it all boils down to keenly understanding the bot’s needs and aligning them with the available intent categories.
A common mistake that developers make is providing incorrect intents. For example, if a developer defines a
GuildMemberAdd
or
MessageCreate
event but has not provided the right intent, the bot will lack the necessary data leading to dysfunctionality. The solution here is to carefully determine which events your bot relies on and aligning your intent selection accordingly.
Another blunder is omitting mandatory intents like the Guilds and Messages. Without these, your bot could miss important events that are fundamental for its functionalities. It’s imperative for developers to ensure they include the default intents unless they’re developing a bot that does not require them.
Finally, there’s the mistake of including unnecessary intents which can result in things like excess data being passed along, raising privacy concerns, or wasting computational resources since you’re listening for events your bot doesn’t need to respond to. To avoid this, one must be careful to specify only intents corresponding to events their bot is designed for.
The good news is, rectification of such mistakes revolves around the efficient use of the Discord API documentation as well as thorough testing of bot functionality throughout development stages.
Apart from handling mistakes, it’s also paramount to consider some best practices when dealing with Discord APIs client’s intent. Kent C. Dodds, a renowned JavaScript Software Engineer, once said: “Code is like humor. When you have to explain it, it’s bad.” This underscores the importance of writing clear, readable, and understandable code in terms of intent setup, thus circumventing potential configuration mistakes.
Effective Techniques to Troubleshoot Invalid Discord Client Intent Issues
When handling the Discord API, a possible pain point developers encounter lies in the need to provide valid intents for the client. This requirement is crucial because it determines the types of events your bot will be capable of receiving from the API. When you encounter an “Invalid Discord Client Intent” issue, this is your code essentially telling you that the intents you’ve specified don’t conform to what the Discord API recognizes or requires.
Discord
has, thus, introduced a system called ‘Intent Flags’ which are essentially binary switches that enable or disable different event groupings. These intent flags allow you to specify exactly which events you want your bot to receive source.
We’ll explore effective techniques to troubleshoot such issues, revolving around the central cause: improper setting of intent flags.
Confirm That All Required Intents Are Set
Ensure all the necessary intent flags have been set according to the functionality requirements of your bot. If a particular flag hasn’t been activated, associated events cannot be received. For instance, if your bot handles message reactions, but you haven’t applied the
MESSAGE_REACTION_ADD
intent, you will meet an invalid client intent issue.
Explicitly Define Default Intents
In many cases, having default intents set can help reduce the likelihood of invalid intent errors. Example of this may include
GUILD_MESSAGES
, and
GUILD_MESSAGE_REACTIONS
, among others. Explicitly set these in your client object when connecting to the API.
Error Specific Debugging
Reviewing error messages provides vital information about the kind of problem at hand. Analyzing the error code trace could pinpoint the specific intent causing trouble, speeding up the resolution process.
Ensure the Intent Flag Enumerations Are Correct
An often overlooked but crucial aspect of setting intents is using the correct intent flag enumerations. Typos or incorrect capitalization can lead to discord reading an inapplicable intent hereby resulting in a discord client intent error. Verifying spelling accuracy, as well as the use of the right characters, ensures you correctly set up your bot’s capabilities.
As Jeff Atwood has famously stated, “Coding is not ‘fun’, it’s technically and ethically complex.” Relatedly, troubleshooting invalid Discord Client Intent issues requires a blend of critical assessment and keen attention to details—a fitting tribute to the challenging (yet rewarding) nature of coding.
The key to successful integration with the Discord API relies heavily on providing valid intents for the client. The Discord Gateway, which fuels real-time updates, uses a concept known as ‘intents’ to regulate these events, and it’s mandatory to define them.
A quick recap about Discord Intents:
Intents | Functionalities |
---|---|
GUILD_MEMBERS | Track member joins, updates, or leaves |
GUILD_BANS | Track when a member is banned or unbanned |
GUILD_EMOJIS_AND_STICKERS | Detect emoji or sticker updates |
GUILD_INTEGRATIONS | Observe changes in guild integrations |
Failure to provide valid intents will prompt “405 Method Not Allowed” error because Discord now requires bots to explicitly declare the scope of their functionality by using these gateway intents. To resolve this, pass an array of desired intent strings when constructing the Client object.
For example:
const client = new Discord.Client({ ws: { intents: ['GUILDS', 'GUILD_MESSAGES'], } });
Not restricted to returning guild information only, bot developers could maximize their usage based on requirements ranging from tracking messages across different channels to monitoring guild bans and emojis/stickers. As Steve Jobs once said, “Design is not just what it looks like and feels like. Design is how it works.” The same applies to designing comprehensive bot functionalities using Discord API that perfectly cater to specific application needs.
For a detailed guide on using these friendly strings, refer to the Discord API Documentation. It provides invaluable insights on efficiently handling Intents and thereby leveraging the potential of Discord Bots to meet numerous requirements efficiently.
To wrap up, remembering to provide valid intents for the Client when working with the Discord API is not just recommended—it’s required. Whether you are building a bot to oversee a gaming guild or develop an engaging community platform, mastering the use of Intents will pave the way to tap into the real power of Discord’s ecosystem and create remarkable, interactive experiences.