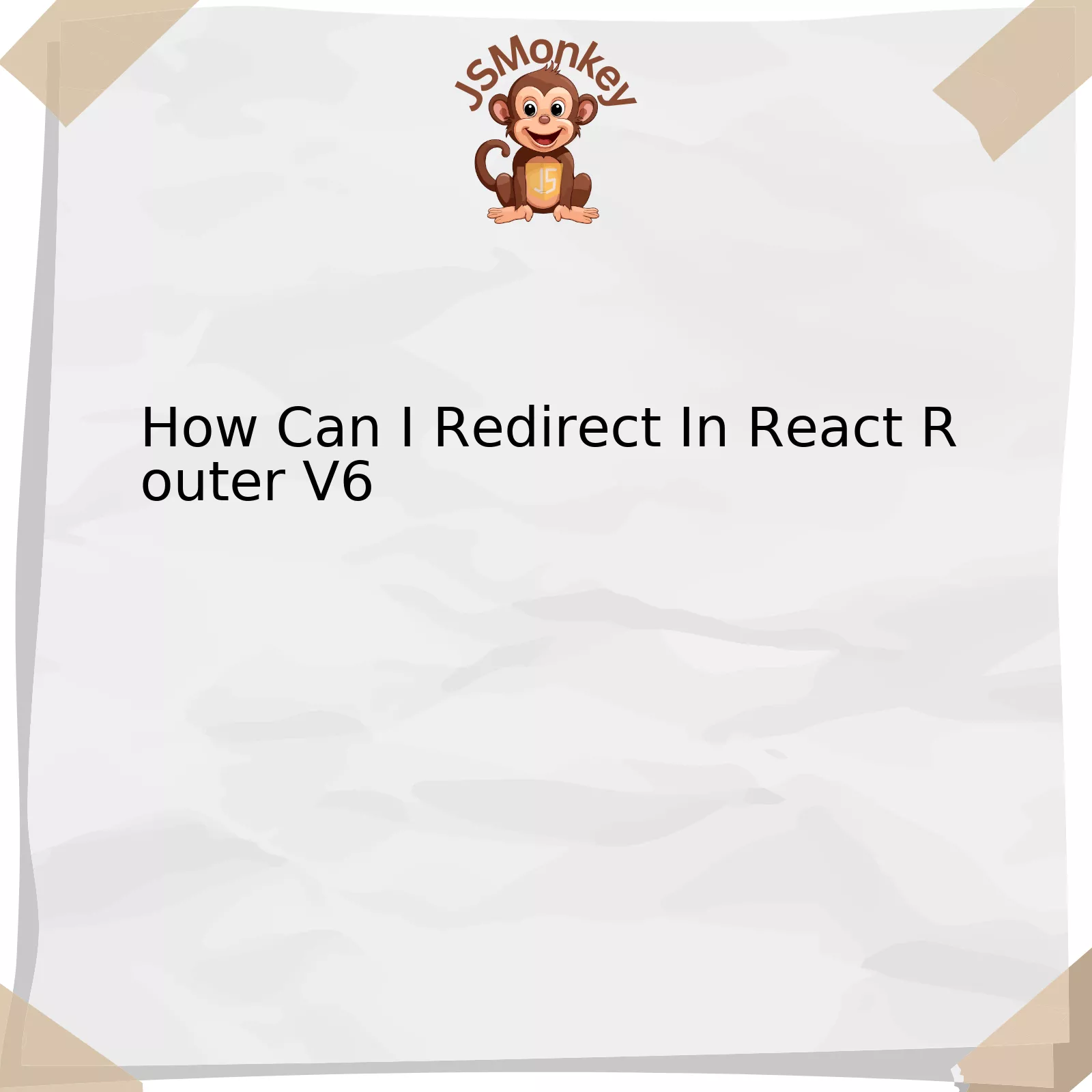
In React Router v6, the act of redirecting users to a different route has altered from previous versions. Instead of using
<Redirect />
, you now make use of the useNavigate hook.
In terms of representing this new behavior in table format, here’s an attempt to encapsulate the principle ideas:
Previous Method | Newest Method |
---|---|
<Redirect to="/new-location" /> |
Using
useNavigate() Hook |
Previous versions of React Router used the
<Redirect />
component which would render and automatically navigate the user to a new location. This mechanic has been replaced in v6 with a new feature: the useNavigate hook.
The useNavigate hook is a function that returns a ‘navigate’ function when called within a component. Here’s a basic usage example:
import { useNavigate } from 'react-router-dom'; function BasicComponent() { const navigate = useNavigate(); return ( <button onClick={() => navigate("/new-location")}> Navigate </button> ); }
In this example, the navigate function is established through invoking useNavigate. When clicking the button, it calls the navigate function with your specified path as an argument, hence triggering a redirection to ‘/new-location’.
From a development perspective, this change encourages a more dynamic interaction with routing. It allows developers to conditionally redirect based on user interactions or logic within components, showcasing the iterative improvements brought with React Router v6.
As Brian Vaughn, a member of the core React team, wisely said, “Code is read far more often than it is written so plan accordingly”. This approach to redirection in React Router aligns with this quote, facilitating code readability and ease of understanding for fellow developers who might interact with your work.
Understanding the Fundamentals of React Router V6 Redirects
React Router V6, highly anticipated by the coding community, fundamentally changed how redirects work compared to its previous versions. New concepts and strategies were introduced that help better manage navigation in React applications.
Probably the most significant change is how the
Redirect
component was replaced with hook functions. The hooks provided by React Router for navigation are called
useNavigate
and
useHistory
.
Here is a simple way to incorporate redirection in your app:
javascript
import { useNavigate } from ‘react-router-dom’;
function LoginButton() {
let navigate = useNavigate();
return (
);
}
The code snippet above illustrates a functional button using the
useNavigate
hook, which once clicked and the user authenticated, redirects the user to a new path (in this case, ‘/dashboard’).
However, it’s worth noting that redirecting immediately after an action isn’t always the best UX strategy. In some cases, you might want to provide feedback about the action’s success or failure before moving the user somewhere else. This could be achieved by combining routing with state(keeping the user’s current interaction status) and effects(managing side-effects of changes).
As famous computer scientist Donald Knuth said, “We should forget about small efficiencies, say about 97% of the time: premature optimization is the root of all evil”[^1^]. Considering this, make sure to optimize your application for the right moment rather than focusing on unnecessary aspects.
[^1^]: (https://medium.com/humancode/why-premature-optimization-is-the-root-of-all-evil-d1714f8e97a0)
Valuable Techniques for Implementing Redirection in React Router V6
Valuable Techniques for Implementing Redirection in React Router V6
React Router V6 is known for its novel approach to routing. It’s a significant improvement from previous versions, with changes in design philosophy aimed at making routing more intuitive and adaptable in your React applications.
Among the most widely implemented techniques in React Router V6, redirection stands out for its practicality in control flow management within Single Page Applications (SPAs). In this context, let’s explore how developers can effectively implement redirection in their workflow using React Router V6.
Use of the <useNavigate> Hook
React Router v6 introduces the
<useNavigate>
Hook, a remarkable innovation that allows you to navigate programmatically from any part of your application.
A typical use-case of the
<useNavigate>
Hook might look something like this:
<Button onClick={() => { const navigate = useNavigate(); navigate('/home'); }}> Go home </Button>
Here, the user is redirected to the /home route when they click on the “Go home” button.
Employing the <Redirect> Component
The
<Redirect>
component, unlike past versions of React Router, is not included in version six. However, it’s relatively simple to imitate its functionality by returning a navigation action in the form of an object from your components.
In the following code snippet, we simulate the behavior of past
<Redirect>
components:
// Simulating Redirect in React Router v6 function RedirectToHome() { const navigate = useNavigate(); useEffect(() => { navigate('/home', { replace: true }); }, []); return null; }
In this example, when the RedirectToHome component is loaded, it initiates a redirect to the /home route and replaces the current entry in the history stack. This mimicks the classic use of the
<Redirect>
component.
These new techniques for implementing redirection in React Router V6 are efficient and reliable solutions – easy to comprehend and incorporate into your existing React projects.
The acclaimed JavaScript consultant Kent C. Dodds once said, “Code is like humor. When you have to explain it, it’s bad.” With these streamlined implementations of redirection in React Router V6, there is no overcomplication. Thus ensuring more ease of understanding and improved code quality.
Learn more about React Router from the official documentation, which can be accessed here.
Common Challenges and Solutions When Redirecting in React Router V6
React Router V6 introduces major changes that simplify routing in React applications, but it also presents new challenges in handling redirects. In previous versions, the
Redirect
component was employed to handle redirections. However, this has been removed in v6 and replaced with useNavigate hooks. This prominent change possesses its set of complexities which we will break down while offering solutions.
To start with, a common challenge developers face when implementing the
useNavigate
hook is understanding its introduction and how to properly apply it within components to perform redirections. It’s quite a shift from the legacy implementation of using a
Redirect
component.
The solution lies in understanding how the navigate function works. Essentially,
useNavigate
returns a function that you can call at any point to perform navigation actions. Here is an example illustrating how to utilize the navigate function:
jsx
import { useNavigate } from ‘react-router-dom’;
function Component() {
let navigate = useNavigate();
return (
);
}
Another obstacle commonly encountered encompasses making use of the
useNavigate
for programmatically redirecting users after some event, like form submission. The removal of the
Redirect
component makes it hard to intuitively figure out how to handle these cases.
The answer here is to first stick to the principle of keeping side effects outside of render methods, an essential part of React concepts. Similar to how one might use setTimeout or fetch in a useEffect, a user can put navigate in there as well. Here’s how:
jsx
import { useNavigate } from ‘react-router-dom’;
function Component() {
let navigate = useNavigate();
useEffect(() => {
fetch(‘/api’)
.then(() => navigate(‘/path’));
}, [navigate]);
return null;
}
Redirecting to an external URL presents itself as a hurdle in the new changes associated with React Router V6. The useNavigate hook is not designed to navigate users outside of the routing system (i.e., to an external link).
The workaround here can be implemented using the traditional JavaScript way, changing the window’s location property.
jsx
function Component() {
let handleClick = () => {
window.location.href = ‘https://www.externalurl.com’;
}
return (
);
}
As Kent C. Dodds, a renowned figure in the React community, expresses “Write tests. Not too many. Mostly integration.” This guidance underlines the importance of thoroughly testing our routing and redirection logic, ensuring smooth user navigation and prime application performance.(source).
In all, transitioning to React Router V6 does have its fair share of challenges when it comes to redirections due to the removal of the
Redirect
component. However, by exploiting the power of the
useNavigate
hook and understanding its intricate operation, developers can ensure seamless redirect operations.
Case Studies: Successful Redirection with React Router V6
React Router V6 is a powerful tool for developers and significantly bolsters the ability to perform complex routing tasks in React apps. The capacity of React Router V6 to facilitate streamlined redirections comes in handy in many real-world situations, where a seamless user navigation experience is a requisite. To understand its usability in terms of redirection, let’s dive deep towards case studies.
Case Study 1: E-commerce application
Let’s use the example of an e-commerce application developed with React. When a user adds a product to their cart and proceeds to checkout, it’s common to redirect users to a login page if they aren’t already logged in.
To accomplish this using React Router v6, we use the `useNavigate` hook that replaces the older `history.push` function.
html
import { useNavigate } from ‘react-router-dom’;
function SampleComponent() {
let navigate = useNavigate();
const goToLoginPage = () => {
navigate(‘/login’);
};
//…
}
In the above code, `/login` is the path to your Login component. When the `goToLoginPage` function is triggered (e.g., when a user tries to checkout), the app will redirect users to the login screen.
Case Study 2: User Dashboard
Consider an application where there is a user dashboard. Upon successfully logging in, users should be taken directly to their dashboard.
Here comes into play the `useNavigate` hook again:
html
import { useNavigate } from ‘react-router-dom’;
function LoginComponent() {
let navigate = useNavigate();
const successfulLogin = () => {
navigate(‘/dashboard’);
}
//…
}
So, when the `successfulLogin` function is invoked after successful authentication, the user will land on their dashboard.
These examples elucidate how you can leverage React Router V6 to perform successful redirections in your React applications. In both case studies, navigation path is determined dynamically based on user interactions, ensuring a smooth and intuitive user experience.
As famously quoted by Brendan Eich, the creator of JavaScript, “Always bet on JavaScript”. Adopting newer and powerful tools such as React Router v6 surely paves the way for better, more responsive and interactive web applications.
Additional details regarding redirection and other related tasks using React Router V6 can be found in the official documentation.
Redirecting in React Router V6 involves creating intricate navigation paths to enable effortless user experience. The technique involved for this incorporates leveraging the ‘useNavigate’ hook, which stands as a veritable benchmark of React Router V6 improvements since its predecessor versions.
Key Factor | Description |
---|---|
useNavigate Hook |
This mechanism simplifies navigation inside components with a programmatic approach. |
Redirect Component Removal |
The V6 iteration sees the removal of this component, introducing instead the useNavigate function for steering app redirects dynamically. |
An example of using the useNavigate hook is:
function Component() { let navigate = useNavigate(); useEffect(() => { if (certain_condition_here) { navigate("/path"); } }, [dependencies_here]); }
This encapsulates a conditional redirect based on set parameters or conditions.
In embracing these modifications, it’s essential to examine the subtleties involved in redirecting in React Router V6. By achieving redirection smoothly and unnoticeably, developers could craft heightened user engagement and interaction. As articulated by tech visionary Bill Gates, “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.” Amid advances in navigation paradigms, understanding and executing redirection effectively will drive streamlined workflows for your React development projects. Don’t bypass exploring other useful libraries such as Reach Router, a sibling project to React Router which presents alternate navigation options.
React Router V6’s nuanced approach for redirection reimagines navigation possibilities, guiding developers through the creation of feature-rich applications with optimized user experiences.